Daily Dose of DSA - Day 13
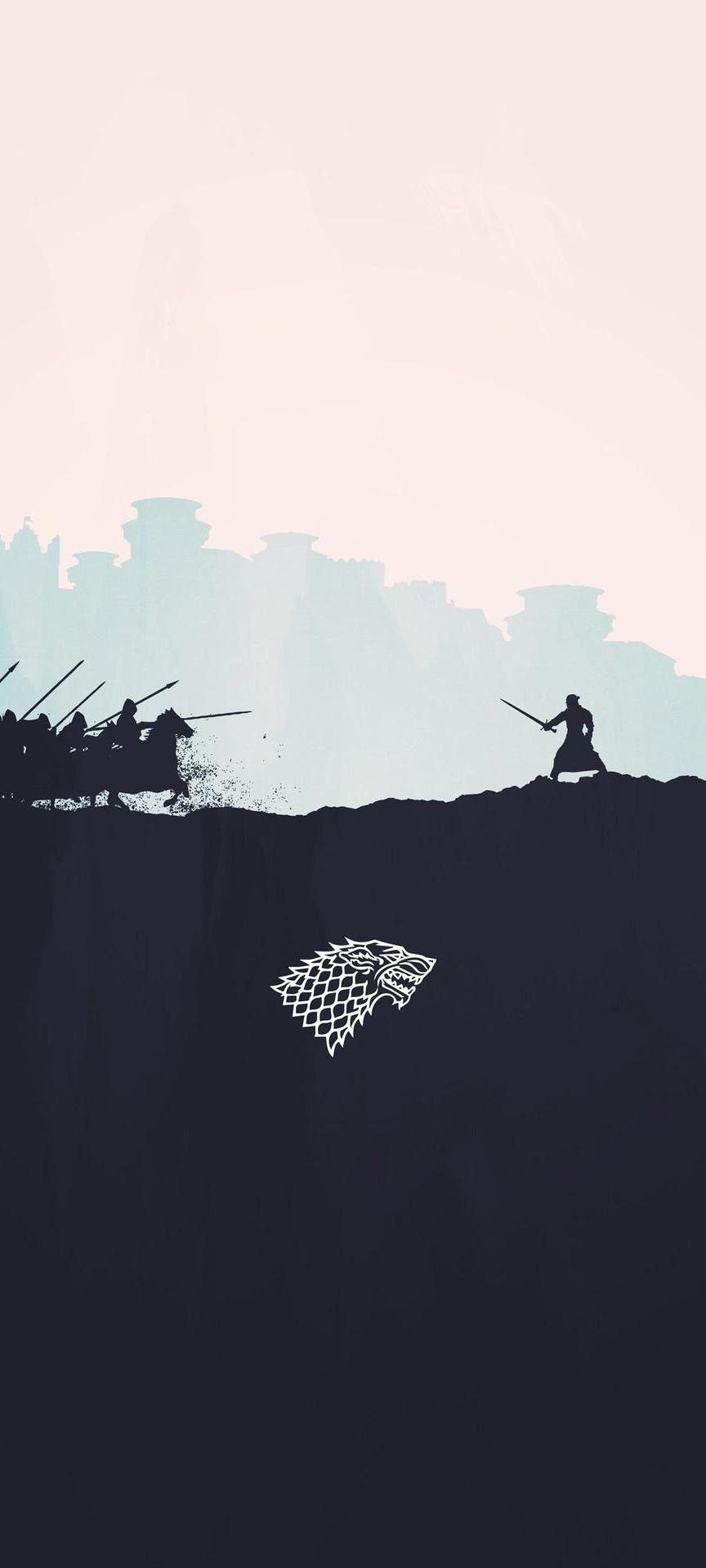
Table of contents
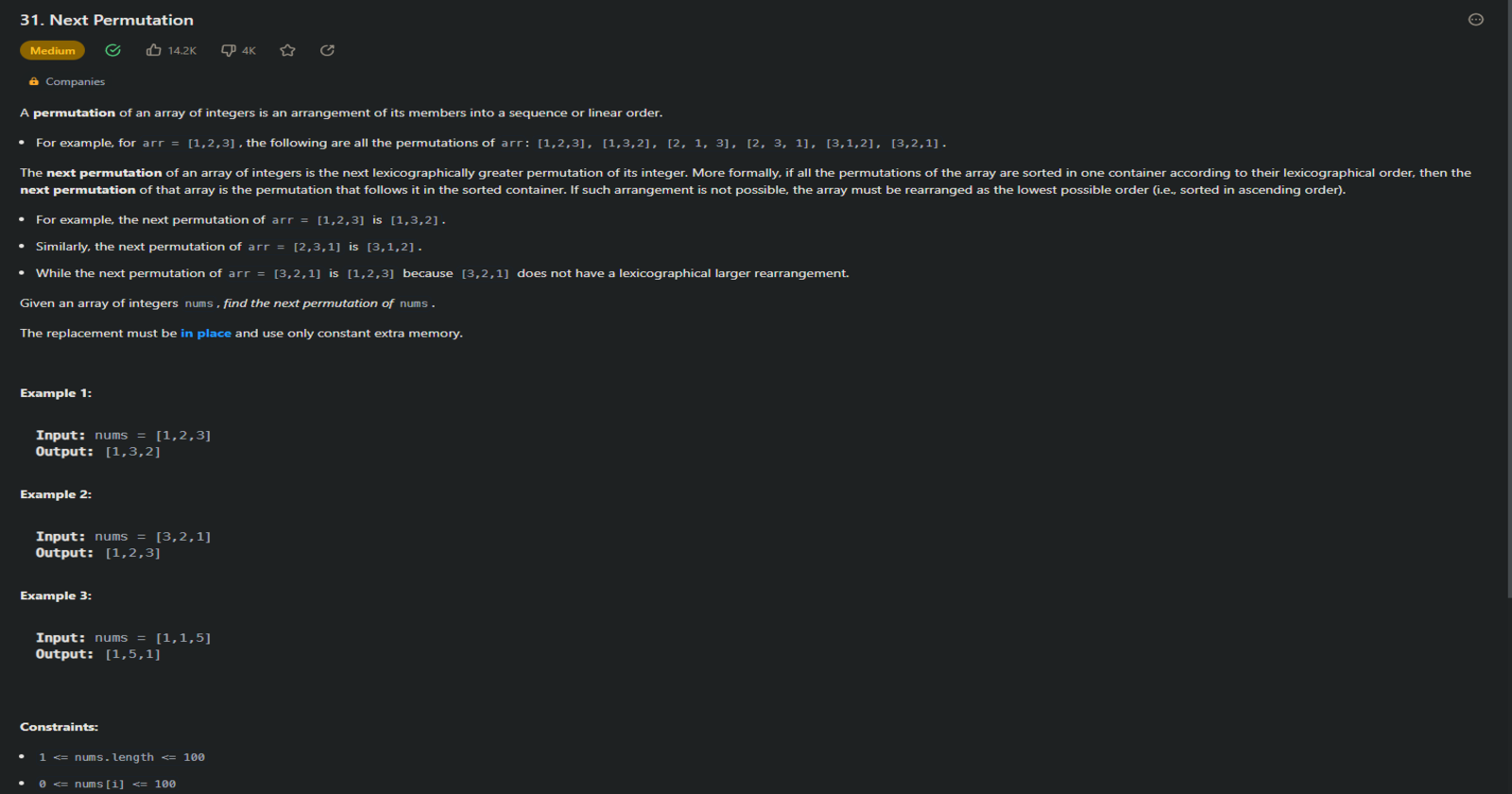
Question link: https://leetcode.com/problems/next-permutation/
Approach :
Step 1: Linearly traverse array from backward such that ith index value of the array is less than (i+1)th index value. Store that index in a variable.
Step 2: If the index value received from step 1 is less than 0. This means the given input array is the largest lexicographical permutation. Hence, we will reverse the input array to get the minimum or starting permutation. Linearly traverse array from backward. Find an index that has a value greater than the previously found index. Store index is another variable.
Step 3: Swap values present in indices found in the above two steps.
Step 4: Reverse array from index+1 where the index is found at step 1 till the end of the array.
class Solution
{
public:
void nextPermutation(vector<int> &nums)
{
next_permutation(nums.begin(), nums.end());
}
};
class Solution
{
public:
void nextPermutation(vector<int> &nums)
{
int n = nums.size(), k, l;
for (k = n - 1; k > 0; k--)
{
if (nums[k - 1] < nums[k])
{
break;
}
}
if (k < 1)
{
reverse(nums.begin(), nums.end());
}
else
{
for (l = n - 1; l > k - 1; l--)
{
if (nums[l] > nums[k - 1])
{
break;
}
}
swap(nums[k - 1], nums[l]);
reverse(nums.begin() + k, nums.end());
}
}
};
Subscribe to my newsletter
Read articles from Atharva directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
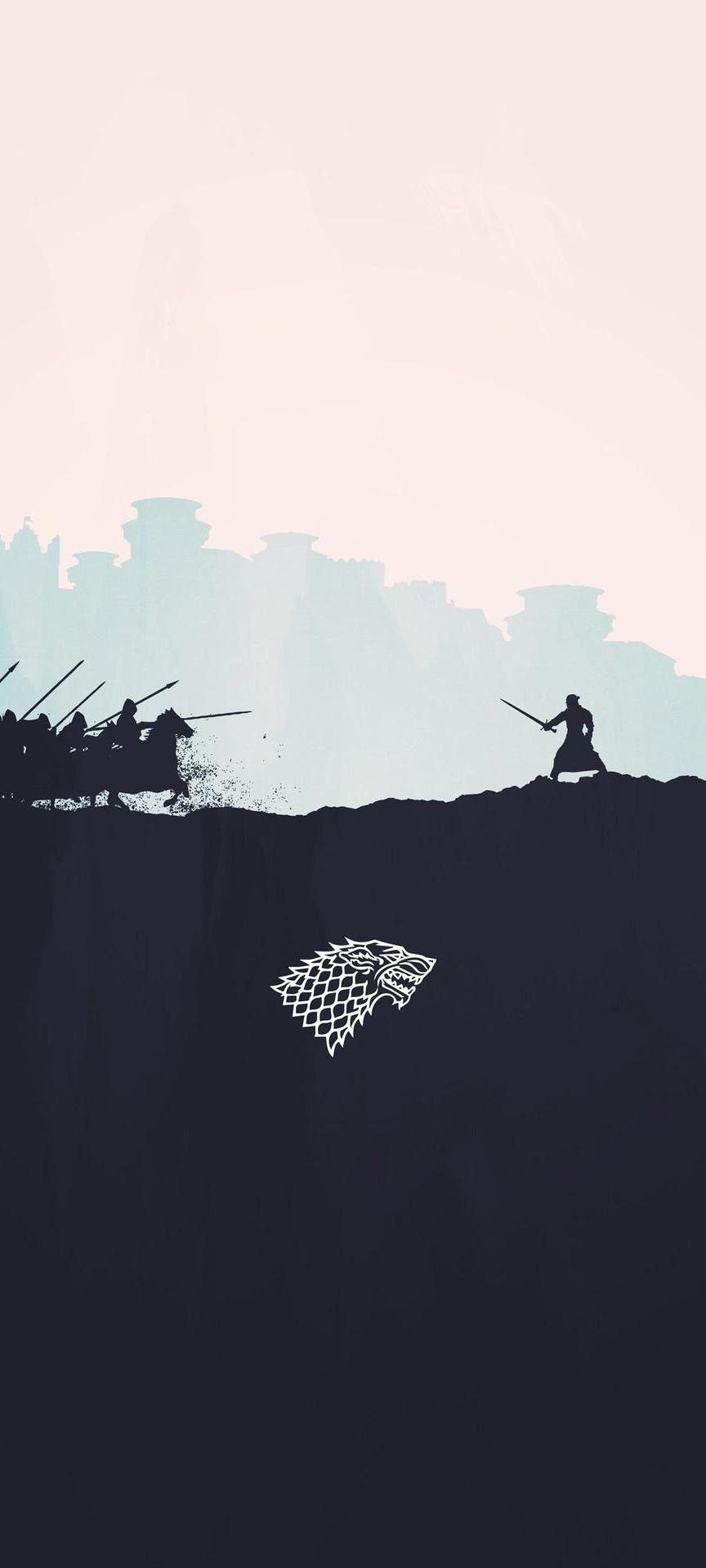
Atharva
Atharva
Hi, I'm Atharva Martiwar, a pre-final year student at National Institute of Technology Rourkela doing my bachelor's degree in Electronics and Electrical Engineering. As a Computer Science & CP Enthusiast, I am keen on problem-solving and logical reasoning through coding. Love to learn DSA and solve coding questions. Good proficiency in C++, Dart, and Python. Currently exploring flutter for Android app development and python for Automation, building web, and Desktop Applications. Apart from my technical skills, I have a great experience in event management and planning. Through experiences and voluntary efforts, I have managed to develop leadership qualities and proficiency in speaking skills. PS: Love to play Badminton & Table Tennis :D