How To Optimize Your Code (Reactjs)

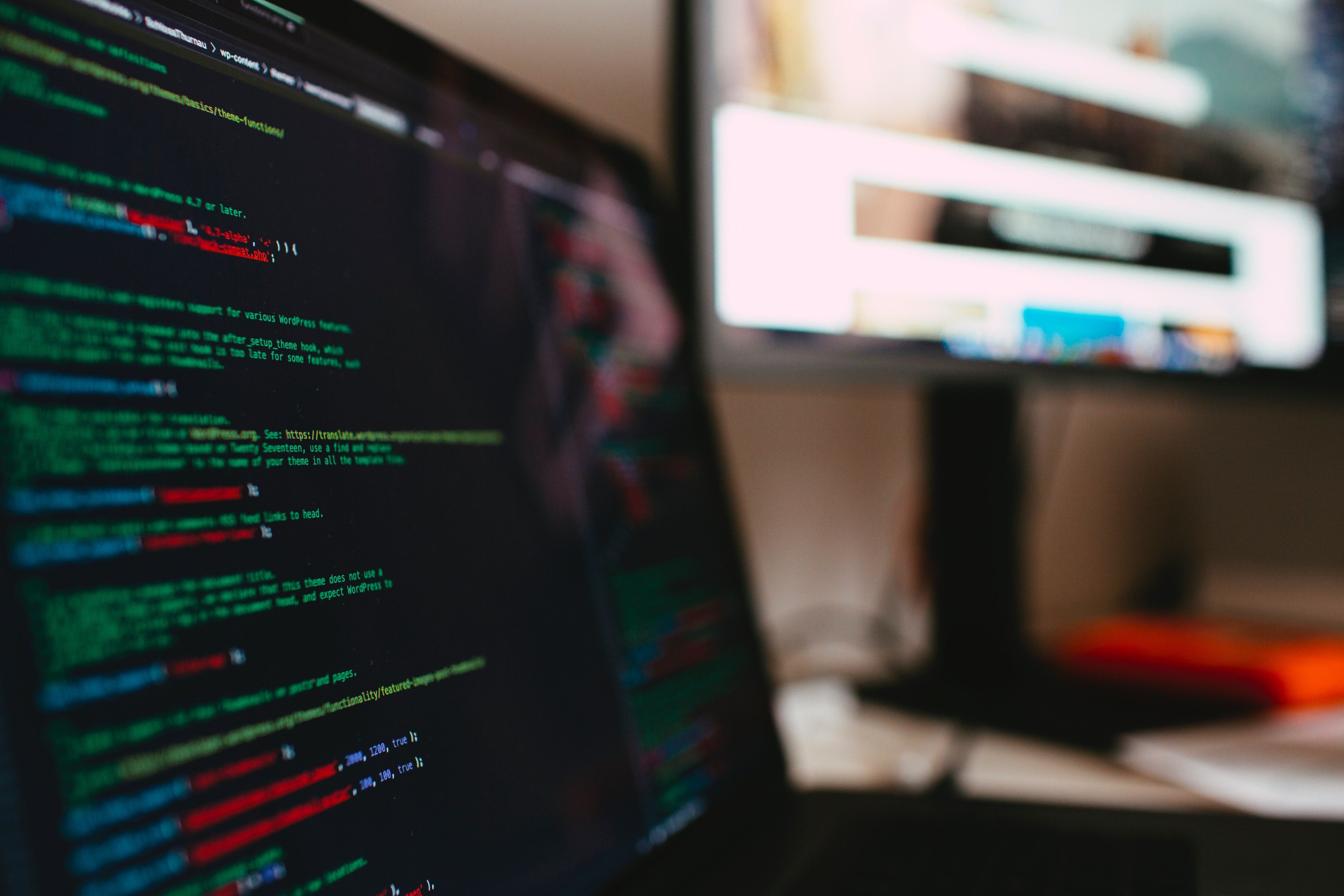
Optimizing code in ReactJS functional components can help improve the performance and user experience of your application. Here are a few tips and examples on how to optimize your functional React components:
Use the React.memo() Higher Order Component: React.memo() is a higher-order component that allows you to wrap your functional component and memoize it. Memoization is a technique that helps to avoid re-rendering components that haven't changed, which can improve the performance of your application.
Consider the below Code :
import React from 'react';
const MyComponent = React.memo(({ props }) => { return
{props.text}
Use the useCallback Hook: The useCallback hook allows you to memoize callback functions. This means that the callback function will only change if one of its dependencies changes. This can help to avoid unnecessary re-renders of your component.
Example:import { useCallback } from 'react'; const MyComponent = ({ props }) => { const memoizedCallback = useCallback((a, b) => { return a + b; }, [props.a, props.b]); return <div onClick={memoizedCallback}>{props.text}</div>; };
Use the useMemo Hook: The useMemo hook allows you to memoize a value that is computed from props or state. This can help to avoid unnecessary computation and improve the performance of your component.
import { useMemo } from 'react';
const MyComponent = ({ props }) => { const memoizedValue = useMemo(() => { return props.a + props.b; }, [props.a, props.b]);
return
(<div>memoizedValue}</div>)
By using these techniques, you can help to improve the performance of your React functional components and provide a better user experience for your users.
Subscribe to my newsletter
Read articles from Naba Zehra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Naba Zehra
Naba Zehra
I am Naba Zehra a frontend developer with a passion for coding and a Bachelor's degree in Computer Science. I have extensive experience in developing web applications and user interfaces using modern frameworks and technologies. I am proficient in the MERN stack, including MongoDB, Express, React, and Node.js, and I am skilled in HTML, CSS, JavaScript, and React, among others. As a front-end developer, I am dedicated to creating visually appealing and user-friendly interfaces that meet client requirements. I have a keen eye for design and always strive to deliver high-quality work that exceeds expectations. In my free time, I enjoy exploring new technologies, attending tech conferences, and contributing to open-source projects. I am also passionate about mentoring and sharing my knowledge with other developers. I am committed to continually improving my skills and knowledge in web development and delivering the best possible solutions for my clients.