Java 8 : Functional Interface
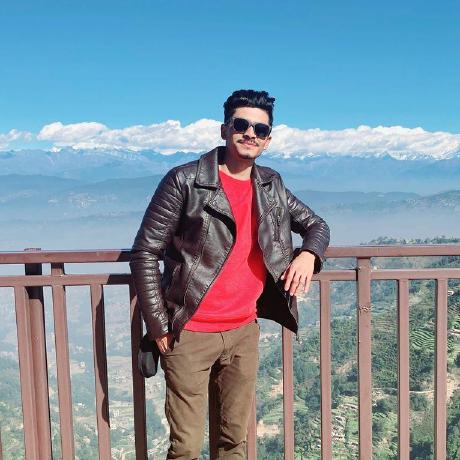
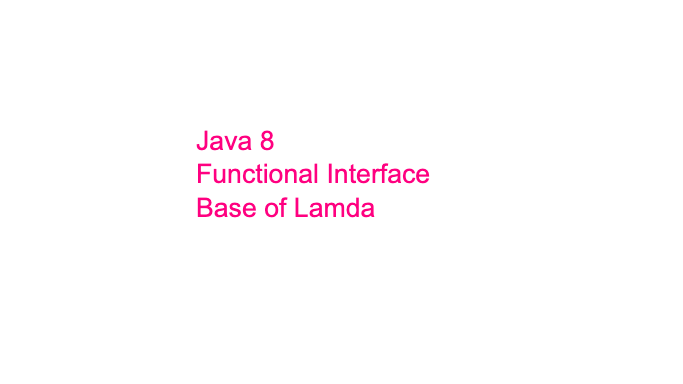
Comparable
public class Employee implements Comparable<Employee> {
private String name;
private float salary;
public Employee(String name, float salary) {
this.name = name;
this.salary = salary;
}
@Override
public int compareTo(Employee e) {
if (this.getSalary() < e.getSalary()) {
return -1;
}
if (this.getSalary() == e.getSalary()) {
return 0;
}
return 1;
}
}
//main method
public class Main {
public static void main(String[] args) {
List<Employee> employeeList = Arrays.asList(new Employee("Bikash", 120), new Employee("Prakash", 110),
new Employee("Bipin", 200));
System.out.println("before sorting by name " + employeeList);
Collections.sort(employeeList);
System.out.println("after sorting by name " + employeeList);
}
}
ComparatorWithClosure
public class Employee {
private String name;
private float salary;
public Employee(String name, float salary) {
this.name = name;
this.salary = salary;
}
}
public class EmployeeComparator {
public enum SortMethod {
BYSALARY, BYNAME
}
public static void sortWithClosure(List<Employee> list, SortMethod sortMethod) {
Comparator<Employee> comparator = new Comparator<Employee>() {
@Override
public int compare(Employee e1, Employee e2) {
if (sortMethod == SortMethod.BYNAME) {
return e1.getName().compareTo(e2.getName());
} else {
if (e1.getSalary() > e2.getSalary()) {
return 1;
} else if (e1.getSalary() < e2.getSalary()) {
return -1;
} else {
return 0;
}
}
}
};
Collections.sort(list, comparator);
}
}
//MAIN METHOD
public class Main {
public static void main(String[] args) {
List<Employee> employeeList = Arrays.asList(new Employee("Bikash", 120), new Employee("Prakash", 110),
new Employee("Bipin", 200));
System.out.println("before sorting by name " + employeeList);
EmployeeComparator.sortWithClosure(employeeList, EmployeeComparator.SortMethod.BYSALARY);
System.out.println("after sorting by name " + employeeList);
}
}
ComparatorWithoutClosure
public class Employee {
private String name;
private float salary;
public Employee(String name, float salary) {
this.name = name;
this.salary = salary;
}
}
public class EmployeeComparator {
public enum SortMethod {
BYSALARY, BYNAME
}
public static void sortWithClosure(List<Employee> list, SortMethod sortMethod) {
Comparator<Employee> comparator = (e1, e2) -> {
if (sortMethod == SortMethod.BYNAME) {
return e1.getName().compareTo(e2.getName());
} else {
if (e1.getSalary() > e2.getSalary()) {
return 1;
} else if (e1.getSalary() < e2.getSalary()) {
return -1;
} else {
return 0;
}
}
};
Collections.sort(list, comparator);
}
}
//main method
public class Main {
public static void main(String[] args) {
List<Employee> employeeList = Arrays.asList(new Employee("Bikash", 120), new Employee("Prakash", 110),
new Employee("Bipin", 200));
System.out.println("before sorting by name " + employeeList);
EmployeeComparator.sortWithClosure(employeeList, EmployeeComparator.SortMethod.BYSALARY);
System.out.println("after sorting by name " + employeeList);
}
}
Bi-consumer
public class BiConsumerDemo {
public static void main(String[] args) {
BiConsumer<String, Double> biConsumer = (param1, param2) -> System.out.println(Double.valueOf(param1) + param2);
biConsumer.accept("12.00", 34.00);
}
}
Consumer: Example 1
public class ConsumerImpl implements Consumer<String> {
@Override
public void accept(String s) {
System.out.println(s);
}
}
public class Main {
public static void main(String[] args) {
ConsumerImpl consumerImpl = new ConsumerImpl();
consumerImpl.accept("consumer functional interface");
}
}
Consumer: Example 2
public class Main {
public static void main(String[] args) {
Consumer consumer = new Consumer<String>() {
@Override
public void accept(String s) {
System.out.println(s);
}
};
consumer.accept("consumer functional interface demo 2");
}
}
Consumer: Example 3
public class Main {
public static void main(String[] args) {
Consumer consumer = (s) -> System.out.println(s);
consumer.accept("consumer functional interface demo 3");
}
}
Consumer: Example 4
public class Main {
public static void main(String[] args) {
Collection<String> list = new ArrayList<>(){{
add("Bikash");
add("BB");
add("CC");
add("DD");
}};
list.forEach((x) -> System.out.println(x));
}
}
Function
public class FunctionDemo {
public static void main(String[] args) {
Function<String, String> function = (s) -> s;
System.out.println(function.apply("Name"));
BiFunction<Double, Double, Double> biFunction = (param1, param2) -> param1 + param2;
System.out.println(biFunction.apply(12.00, 120.55));
}
}
Custom Functional Interface
@FunctionalInterface
public interface CustomFunction<S, T, U, R> {
R apply(S s, T t, U u);
}
public class Main {
public static void main(String[] args) {
CustomFunction<Integer, Integer, Integer, Integer> triFunction = new CustomFunction<Integer, Integer, Integer, Integer>() {
@Override
public Integer apply(Integer s, Integer t, Integer u) {
return s + t + u;
}
};
System.out.println(triFunction.apply(12, 11, 14));
}
}
Predicate
public class PredicateDemo {
public static void main(String[] args) {
Predicate<Integer> predicate = (value) -> value < 100 ? true false;
System.out.println(predicate.test(12));
BiPredicate<String, Integer> biPredicate = (param1, param2) -> param1.equals("Biki") && param2 == 10 ? true : false;
System.out.println(biPredicate.test("BB", 10));
}
}
Supplier
public class SupplierDemo {
public static void main(String[] args) {
Supplier<String> supplier = () -> "Bikash Mainali";
System.out.println(supplier.get());
}
}
Subscribe to my newsletter
Read articles from Bikash Mainali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
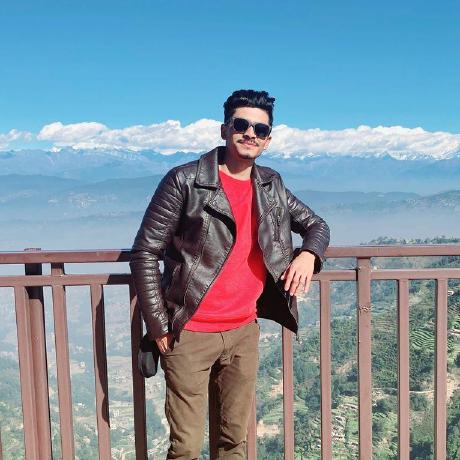
Bikash Mainali
Bikash Mainali
Experienced Java/JavaScript full-stack developer over 6 years of extensive expertise serving key role on elite technical teams developing enterprise software for healthcare, apple ad-platform, banking, and e-commerce. Adaptable problem-solver with high levels of skill in Groovy, Java, Spring, Spring Boot, Hibernate, JavaScript, TypeScript, Angular, Node, Express, React, MongoDB, IBM DB2, Oracle, PL/SQL, Docker, Kubernetes, CI/CD pipelines, AWS, Micro-service and Agile/Scrum. Strong technical skills paired with business-savvy UI design expertise. Personable team player with experience collaborating with diverse cross-functional teams.