Creating a Dropdown Menu Using Javascript's classList and toggle Function
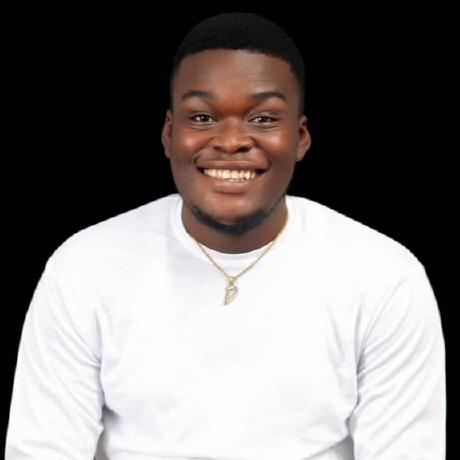
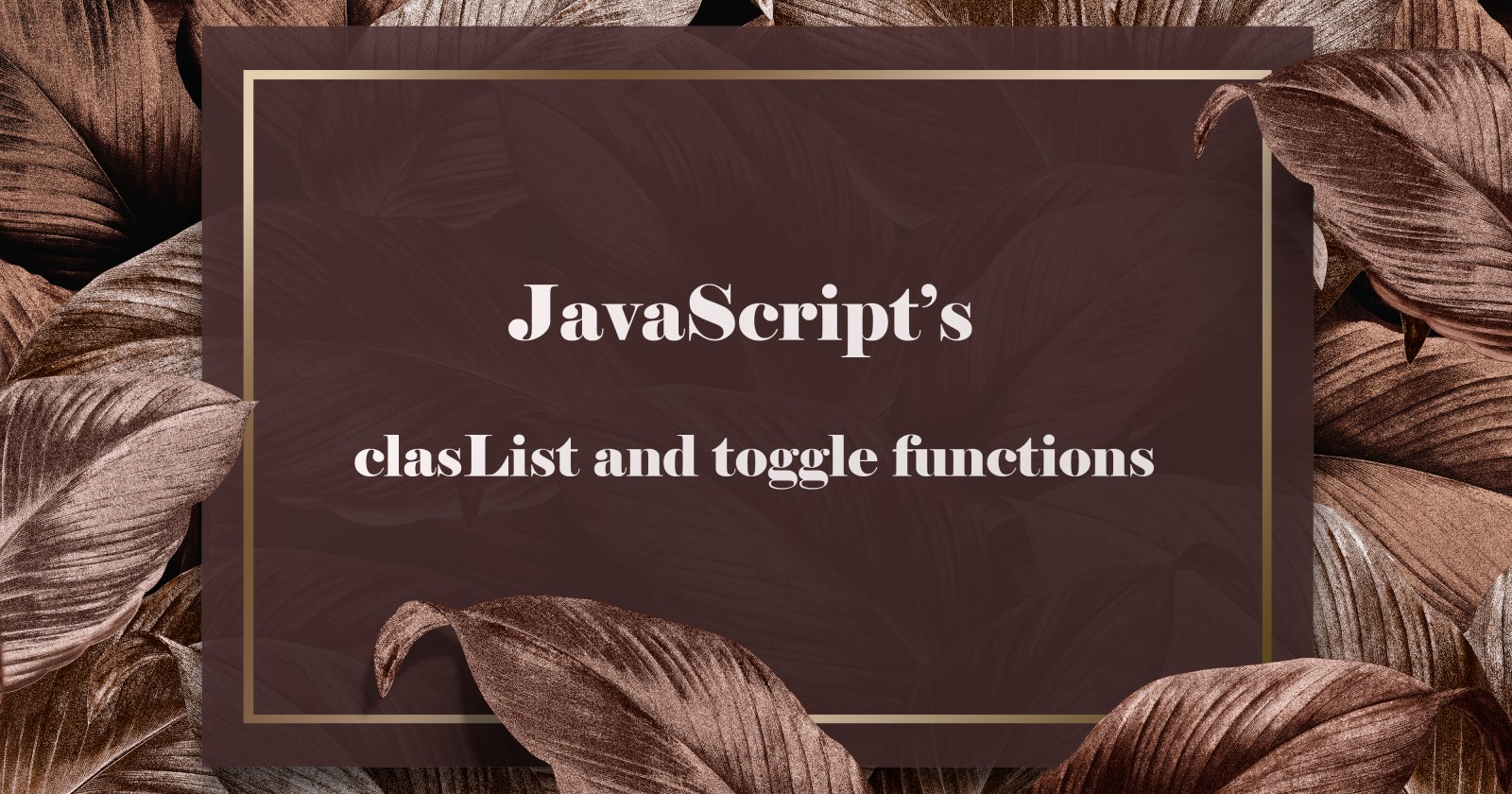
Have you ever been to a website where you click on a dropdown menu and the items appear out of nowhere? It almost seems like magic, doesn't it? Javascript, however, contains two functions that enable you to accomplish this and much more. In this article, we'll go over how these two functions operate and how we can utilize them to develop a simple button that simulates the dropdown effect.
Prerequisites
Basic knowledge of HTML and CSS
Basic knowledge of javascript
A code editor (I will recommend you use VS Code)
A browser (Chrome recommended)
What is the classList function?
Javascript classList is a DOM property that enables customizing of an element's CSS class. The property returns the names of the CSS classes and is read-only (this is a file attribute that only allows a user to view a file, restricting writing to the file). Let's break down how this function can customize our class elements.
DOM(Document Object Model)
The DOM is an application programming interface for HTML and XML documents. This suggests that the DOM is a software bridge that enables communication between two applications.
Let's imagine, for illustration, that we produce an HTML document and render it in our browser. The DOM of our document is then automatically created by our browser. Programs and scripts (in our case, our classList property) can dynamically access and edit the content, structure, and style of our HTML document using the interface that was made for us.
So with that, we've demonstrated that our classList functions will be able to alter the class elements' styles in our HTML document via the DOM.
Below is an example of a classList function in javascript that we can use to determine the class value of our button element.
<!DOCTYPE html>
<html lang="en">
<body>
<main>
<h3>To obtain the class value, click the button below.</h3>
</br>
<button id="btn1" class="classValue" onClick="getClass()">
Click me!
</button>
</main>
<script>
const getClass = () => {
const btn = document.getElementById("btn1");
alert(btn.classList);
}
</script>
</body>
</html>
The output of the above code is shown below:
The class element's value, which is a DOMTokenList, is represented by the classList function. Keep in mind that the read-only feature of our classList function prevents any kind of writing to this file. So if we can't write to our HTML content, how can we edit and modify it?
We can alter the value by tinkering with the classes that the program uses rather than writing directly to it. We'll concentrate on two of them in this article: item() and toggle ().
item()
The item method is used for displaying the name of the classes at the given index. So how do we go about getting the index of our class names within the class value of the element?
Recall that our class elements value is a DOMTokenList. A DOMTokenList is a set of space-separated tokens and it can be assessed by index (starts at 0). This suggests that each class name contained in a class element's value is a token. Because the classList function saves the class names of our element in an array and the item() method accepts an index as a parameter, we can write multiple class names separated by spaces.
Below is an example of the item() method through which we will get the class names of our button element by using its index.
<!DOCTYPE html>
<html lang="en">
<body>
<main>
<h3>To obtain the class value, click the button below.</h3>
</br>
<button id="btn1" class="classValue0 classValue1 classValue3" onClick="getClass()">
Click me!
</button>
</main>
<script>
const getClass = () => {
const btn = document.getElementById("btn1");
alert(`Position 0: ${btn.classList.item(0)}; Position1: ${btn.classList.item(1)}; Position2: ${btn.classList.item(2)}`);
}
</script>
</body>
</html>
The output of the above code is shown below
From the output above, it is evident that our class names are tokens that can be assessed by using the item() method with the classList function.
toggle()
An element's specified class names can be toggled using the toggle method. The specified class is added with one click, and the class is removed with another click.
Below is an example of the toggle method through which we will add a class name to our class elements value that will change the background color of our button to red.
Using the example we created for the item() method, we are going to edit just the getClass() function and change it to the toggleClass function.
<script>
const toggleClass = () => {
const btn = document.getElementById("btn1");
btn.classList.toggle("classValue3");
}
</script>
.classValue3 {
background-color: "red";
color: "white"
}
The output of the above code is shown below
Creating our HTML/CSS Page
We can move on to constructing our drop-down menu now that we understand how to alter and adjust the style of our class elements using these two functions. We'll begin by building a straightforward HTML page containing a button element, after which we'll style this element with CSS.
<body>
<div class="main-Button">
<button onClick="displayButtonClicked()">Categories</button>
<button
onClick="toggleButton()"
>
<span
class="material-symbols-outlined id="display"
>
expand_more
</span>
</button>
<div class="menu" id="menu">
<button onClick="menuButtonClicked()">
<span class="material-symbols-outlined">hotel</span>
Hotels
</button>
<button onClick="menuButtonClicked()">
<span class="material-symbols-outlined">
warehouse
</span>
Warehouses
</button>
<button onClick="menuButtonClicked()">
<span class="material-symbols-outlined">pool</span>
Pools
</button>
</div>
</div>
<script src="./index.js" type="text/javascript"></script>
</body>
*{
box-sizing: border-box;
}
body, html{
height: 100%;
}
body{
display: flex;
align-items: center;
justify-content: center;
background: rgba(0,0,0,0.8);
}
.main-Button{
position: relative;
display: flex;
align-items: center;
border-radius: 8px;
height: 60px;
background: rgba(255,255,255,0.45);
}
button{
display: flex;
align-items: center;
cursor: pointer;
color: black;
height: 100%;
border: 0;
background: transparent;
font-size: 16px;
}
.main-Button > button:nth-child(1) {
padding: 0 24px;
}
.main-Button > button:nth-child(2) {
width: 60px;
justify-content: center;
border-top-right-radius: 8px;
border-bottom-right-radius: 8px;
background: rgba(255,255,255,0.3);
}
.menu{
overflow: hidden;
position: absolute;
top: 68px;
left: 0;
background: rgba(255,255,255,0.45);
width: 100%;
transform: translate(-30px,0);
opacity: 0;
visibility: hidden;
transition: 0.4s;
z-index: 1;
border-radius: 8px;
}
.menu.open{
transform: translate(0,0);
opacity: 1;
visibility: visible;
}
.menu > button {
gap: 10px;
padding: 0 18px;
width: 100%;
padding: 5px;
}
.menu > button:hover{
background: rgba(255,255,255,0.3);
}
The resulting layout is shown below:
Great, now that the foundation of our website has been established, let's dig into JavaScript and discuss the classList and toggle functions.
Implementing the classList and toggle functions.
Using the script tag, we have already linked the "index.js" file to our HTML document. It's now time to add content to our javascript file so that our rendered page will be dynamic.
const menu_v = document.getElementById("menu");
const display_v = document.getElementById("display");
const toggleButton = () => {
menu_v.classList.toggle("open");
display_v.innerHTML = !menu_v.classList.contains("open") ? "expand_more" : "close";
}
Let's break down and discuss the code above so we can better understand how we add the drop-down effect to our button element.
Creating the "menu" and "display" variables
const menu_v = document.getElementById("menu");
const display_v = document.getElementById("display");
One of the most often used methods in the HTML DOM is getElementById(). It is utilized almost always when reading or editing an HTML element. In order to assign the element to our "menu_v" and "display_v" variables, our document method first searches our HTML document for elements with the "menu" and "display" ids.
Creating the "toggleButton" function
const toggleButton = () => {
menu_v.classList.toggle("open");
display_v.innerHTML = !menu_v.classList.contains("open") ? "expand_more" : "close";
}
It is obvious from our prior discussion that we are changing the element's CSS by appending the "open" class name to the "menu v" class value. The "innerHTML" property will then be used to change the element's HTML content.
The output of the above code is shown below:
From the image above, it is evident that our page has been updated. Can you figure out the things that changed? Let's quickly discuss these changes
Because of a conditional statement telling it to alter its content based on whether the class name "open" is part of the class value of the element, the content of the button with the id of "display" has changed from a downward pointing arrow to a close icon.
Our div with the class "menu" is now visible. The "open" class name merely modifies the div's class value, which in turn modifies the element's style.
Conclusion
Congrats!!! You've used the classList and toggle function to create a simple drop-down menu, but there are a few things I didn't get to talk about because the post got too long. The other methods that can be utilized to change our class value were not covered. You can find these methods here.
If you were wondering what else you could accomplish with these functions, your imagination is the limit, you could implement them in so many ways. So stay tuned since I'll probably develop future tutorials covering these features.
Subscribe to my newsletter
Read articles from Ayodeji Adebiyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
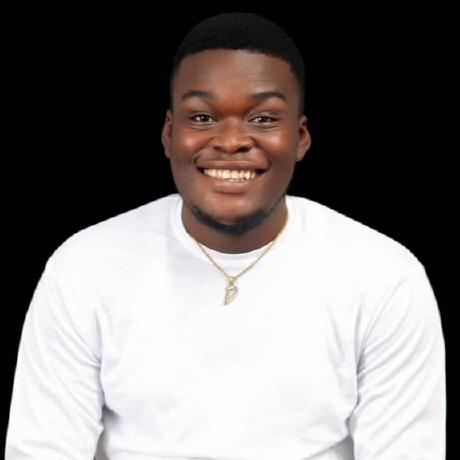