How to convert an image to WebP in NodeJS Express Backend
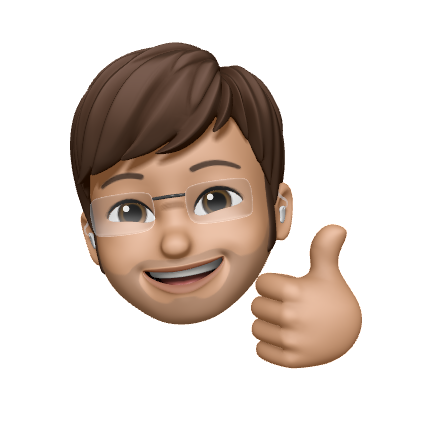
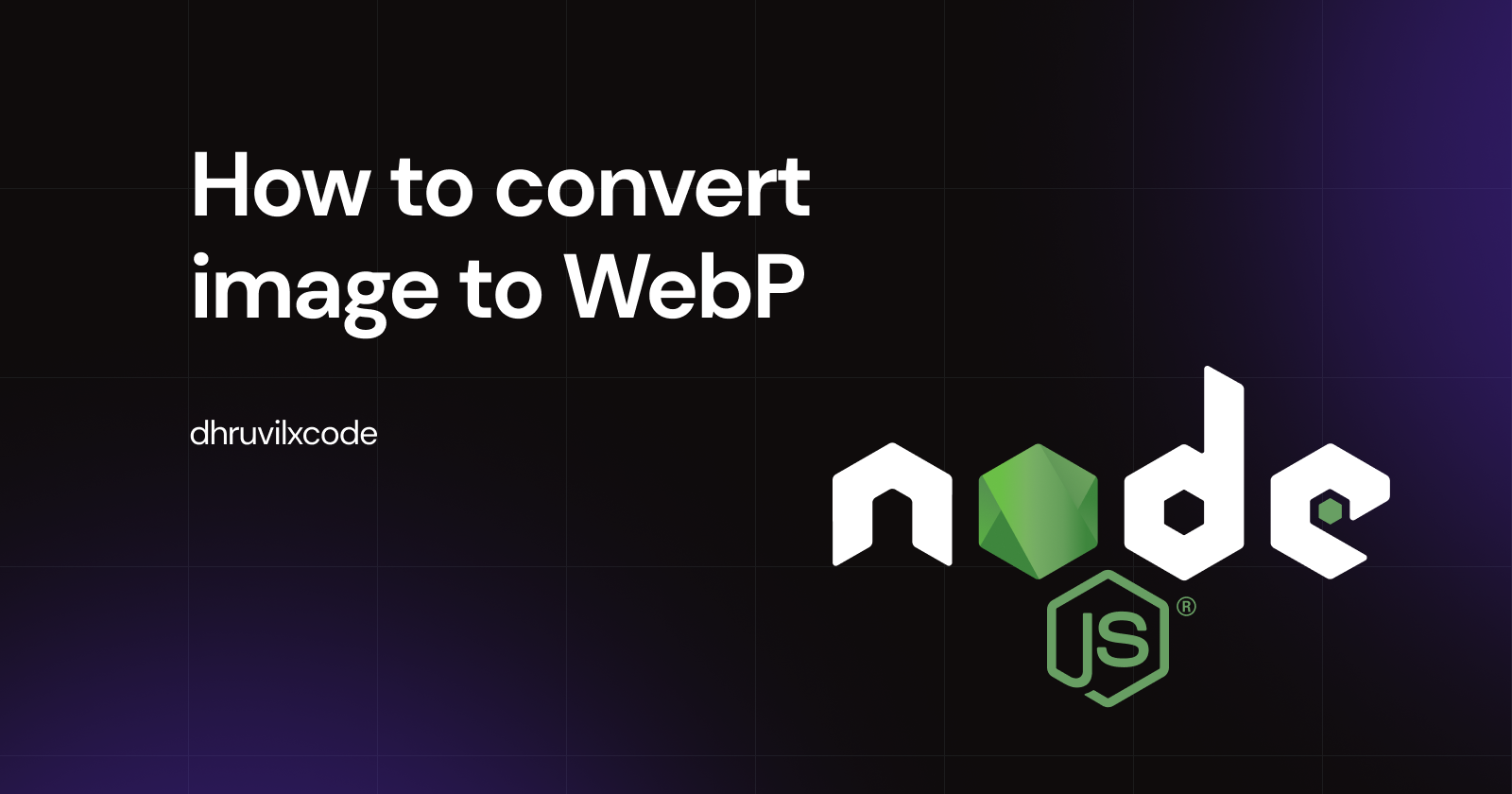
In this article, we will learn how we can convert the uploaded image to NodeJS Express Server to WebP image. Before we dive into code first let's understand Why it's a good idea to convert the image to WebP, and what are the Advantages of it? Let's start.
Why WebP?
WebP is an image extension by Google's Chromium Team, which provide both Lossless and Lossy Image compression with Transparency like PNG
Supports Transparency
25% to 34% smaller file size
WebP is natively supported in Google Chrome, Safari, Firefox, Edge, the Opera browser, Mobile Apps, and many other tools
Reducing image size leads to Improved Performance and faster site loads.
Let's Start by creating Node-Express Project
here is a Full code example if you want to dive into that first
Step 1: Create a Backend project & Install the following dependency
to create a backend project first create a directory,
mkdir image-to-webp-example-nodejs && cd image-to-webp-example-nodejs
npm init
now it's time to install some dependency
npm i express formidable
npm i image-to-webp
This NPM package provides the method to convert the image to a WebP image.
now edit the `package.json` file according to your project needs. make sure you create the empty index.js file
now paste the following code
const fs = require("fs");
const express = require("express");
const formidable = require("formidable");
const { imageToWebp } = require("image-to-webp");
const app = express();
app.post("/upload", async (req, res) => {
const form = formidable({ multiples: false });
form.parse(req, async (err, fields, files) => {
if (err) {
next(err);
return;
}
const image = files.image.filepath;
console.log(image);
const webpImage = await imageToWebp(image, 30);
console.log(webpImage);
// now you can copy this file to public directory or simply upload to your cloud storage provider like AWS S3 / Cloudinary / Google Cloud Storage
fs.copyFileSync(webpImage, "./public/images/myimg.webp");
res.json({ image, webpImage });
});
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on the ${PORT}`);
});
see here I called the imageToWebp
method which takes one required parameter which is the input image path. formidable
returns the Submitted image path from FormData.
Optionally you can pass the Quality of the output image in the second argument of imageToWebp
it accepts the number ranging between 0 to 100.
0 is for High compression and lossy quality. the lesser value means more compression. if you don't pass it, then it will consider 80 as the default.
The imageToWebp
return the output WebP image path which is stored in the tmp
directory. you should move it to your Public
directory to make it accessible to the internet. Or upload the image to any Cloud Provider like S3 / Cloudinary / Google Cloud Storage etc.
I hope you will find it very helpful. I am attaching links here for reference, Thanks for reading.
You can follow me on Social media ๐ง๐ปโ๐ป
Subscribe to my newsletter
Read articles from Dhruvil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
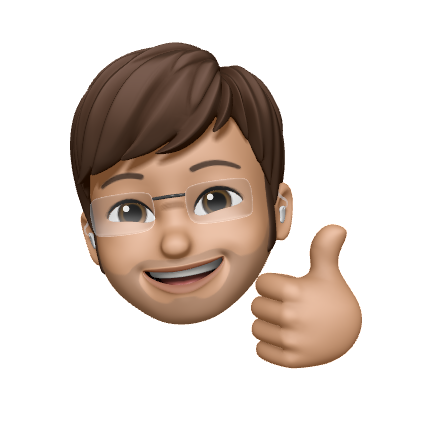
Dhruvil
Dhruvil
I am Dhruvil, doing freelancing for App Development & UI/UX Design. I casually write here and share my knowledge and experience.