Symmetric File encryption in node js (AES-192)
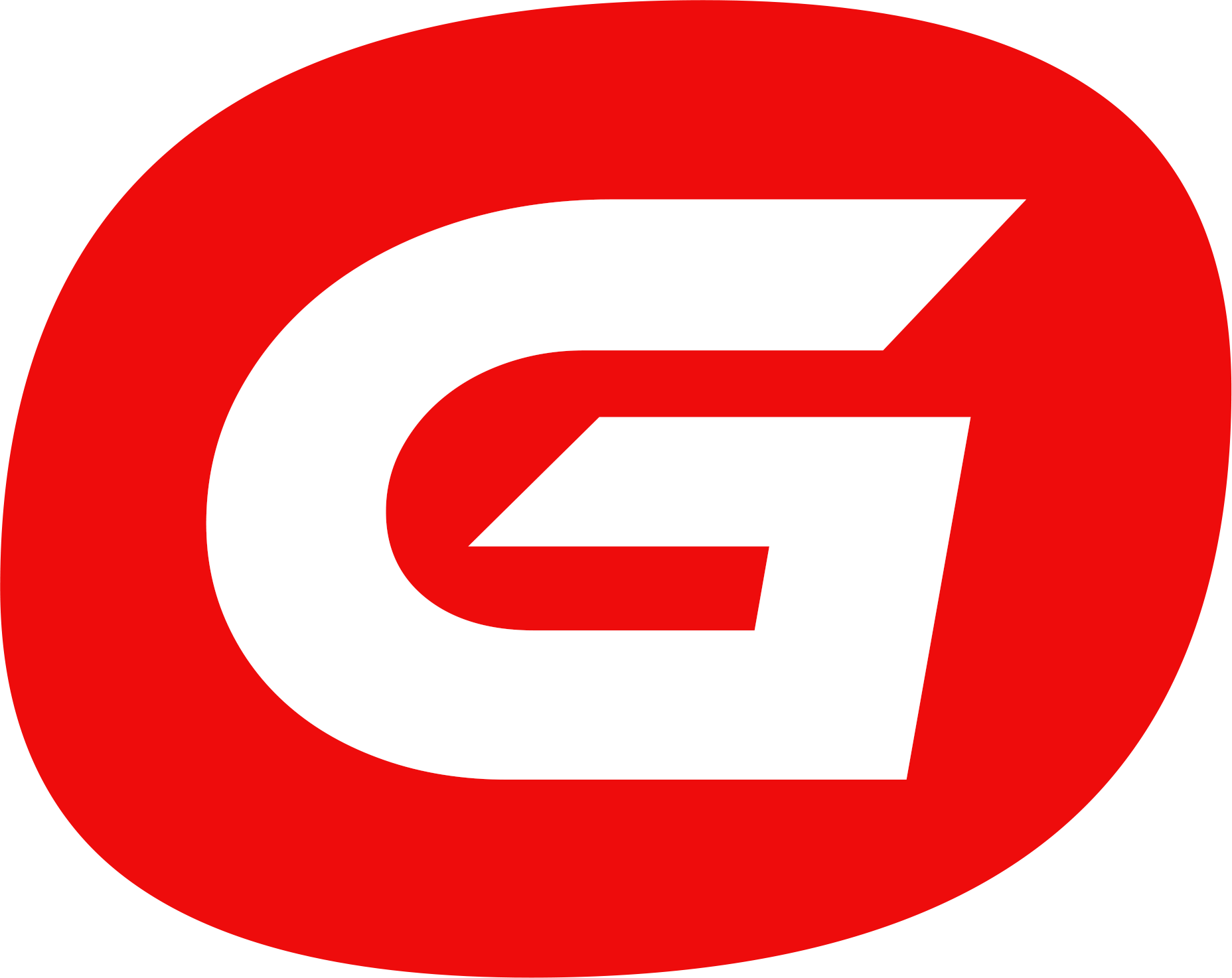
2 min read
how everybody. today I'm gonna show you how to encrypt a file using a symmetric encryption algorithm such as AES in node js.
first of all, we need to import the fs module to make us able to work with files:
const fs = require('fs')
and also some methods from the crypto module:
const {scryptSync, createDecipheriv, createCipheriv} = require('crypto')
now it's the time to create encrypt function that take required arguments and encrypt
const encrypt = (filePath, output, algorithm, key, iv)=> {
const fileStream = fs.ReadStream(filePath)
const outputFileStream = fs.createWriteStream(output)
const cipher = createCipheriv(algorithm, key, iv)
let encrypted
fileStream.on('data', (data)=>{
encrypted = cipher.update(data)
outputFileStream.write(encrypted)
})
fileStream.on('end', ()=>{
outputFileStream.end()
})
}
and also the decrypt function to decrypt the encrypted file:
const decrypt = (inputFilePath, outputFilePath, algorithm,key, iv)=> {
const outputWriteSteam = fs.createWriteStream(outputFilePath)
const inputReadStream = fs.ReadStream(inputFilePath)
const decipher = createDecipheriv(algorithm,key, iv)
let decrypted
inputReadStream.on('data', (data)=>{
decrypted = decipher.update(data)
outputWriteSteam.write(decrypted)
})
inputReadStream.on('end', ()=>{
outputWriteSteam.end()
})
}
new before calling encrypt or decrypt function we need to determine password, algorithm, key and iv:
const password= '0000'
const algorithm = 'aes-192-cbc' // can be replaced with other algorithms
let key = scryptSync(password, 'salt',24)
let iv = Buffer.alloc(16,0)
now we just need to call encrypt or decrypt function
encrypt('./f.mp3', 'enc.enc', algorithm, key, iv) // to ecnrypt
decrypt('enc.enc', './f.mp3', algorithm, key, iv) // to decrypt
0
Subscribe to my newsletter
Read articles from gorgin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
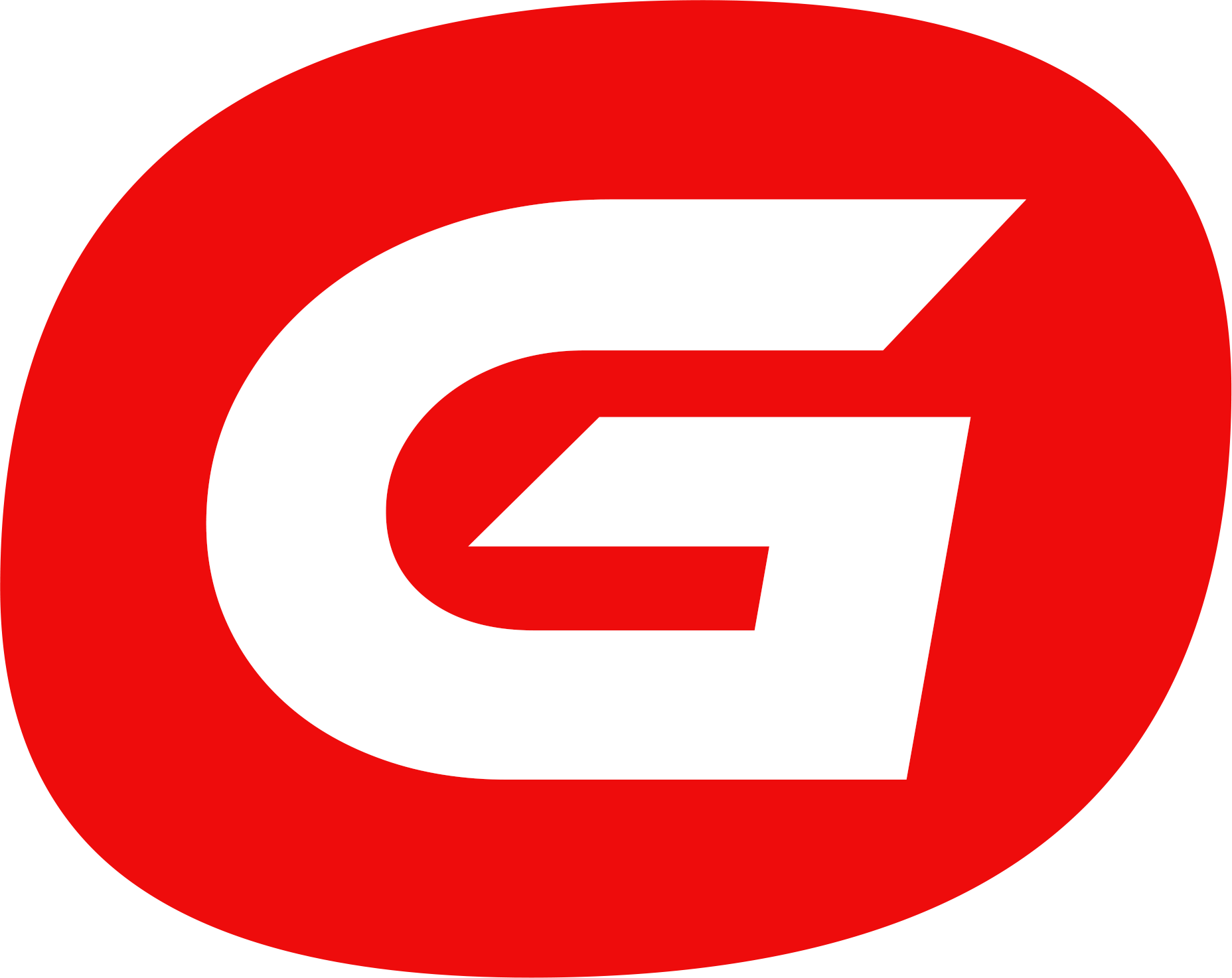