What are Pure functions?

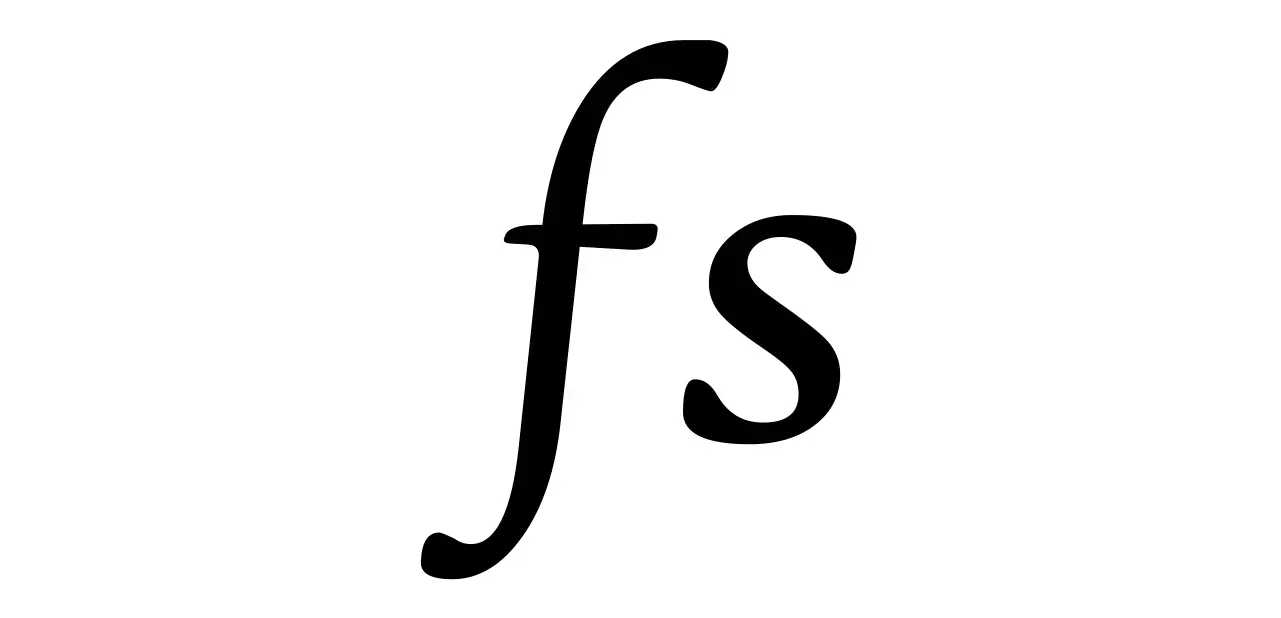
While working on the projects we always get to hear the term Pure functions, but what are Pure functions and why should we use Pure Functions? Let's find out.
Pure Functions are functions that have the following characteristics-
Pure functions always return the same output if the input is the same.
Pure functions do not have any side effects.
Let's suppose we have a function add
, it takes two arguments number1
and number2
and returns the sum of both numbers.
const add = (number1, number2)=>{
let sum = nummber1+number2;
return sum;
}
This function will always return the same output for the same given input and also it does not have any side effects so we can call this function Pure.
What are Side Effects?
In simple terms whenever a function tries to change an external state (outside the scope of that function) we call it a Side Effect and the functions which have side effects are not pure.
Let's suppose a function addUser
that adds the name of the user to the array.
let users=[];
const addUser=(name)=>{
users.push(name)
return users;
}
The above function is not pure because it is mutating the external state which is outside the function scope. We can make this function pure by declaring a new array inside the function scope and modifying that array instead of the original array.
let users=[];
const addUser=(name)=>{
let newUsers=[...users,name];
return newUsers;
}
Why we should use Pure Functions?
Now we know what are the characteristics of pure functions we can explore the benefits of using pure functions.
Pure Functions always return the same value for the same input, so they are very much predictable and easier to test.
Pure does not have side effects and is immutable so they have better code readability.
Since the output of pure functions is predictable, so these functions can easily handle concurrency.
Pure functions are reusable because they don't depend on any external state.
Since the output of pure functions is predictable we can cache/memoize the output and reduce the cost of complex computations.
Subscribe to my newsletter
Read articles from Vinay Kushwaha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
