Event Propagation In JavaScript
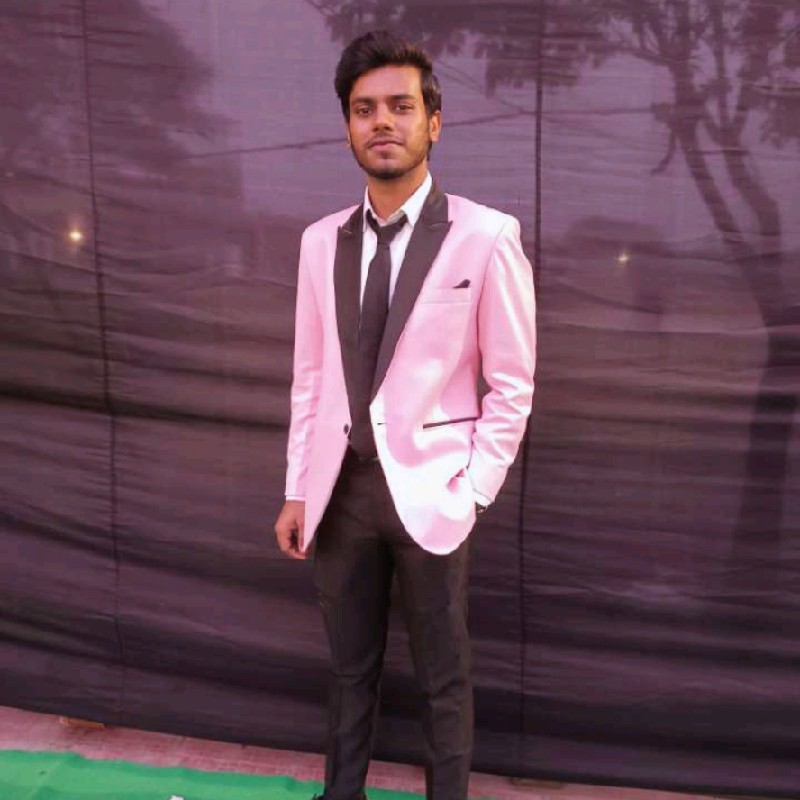
Table of contents
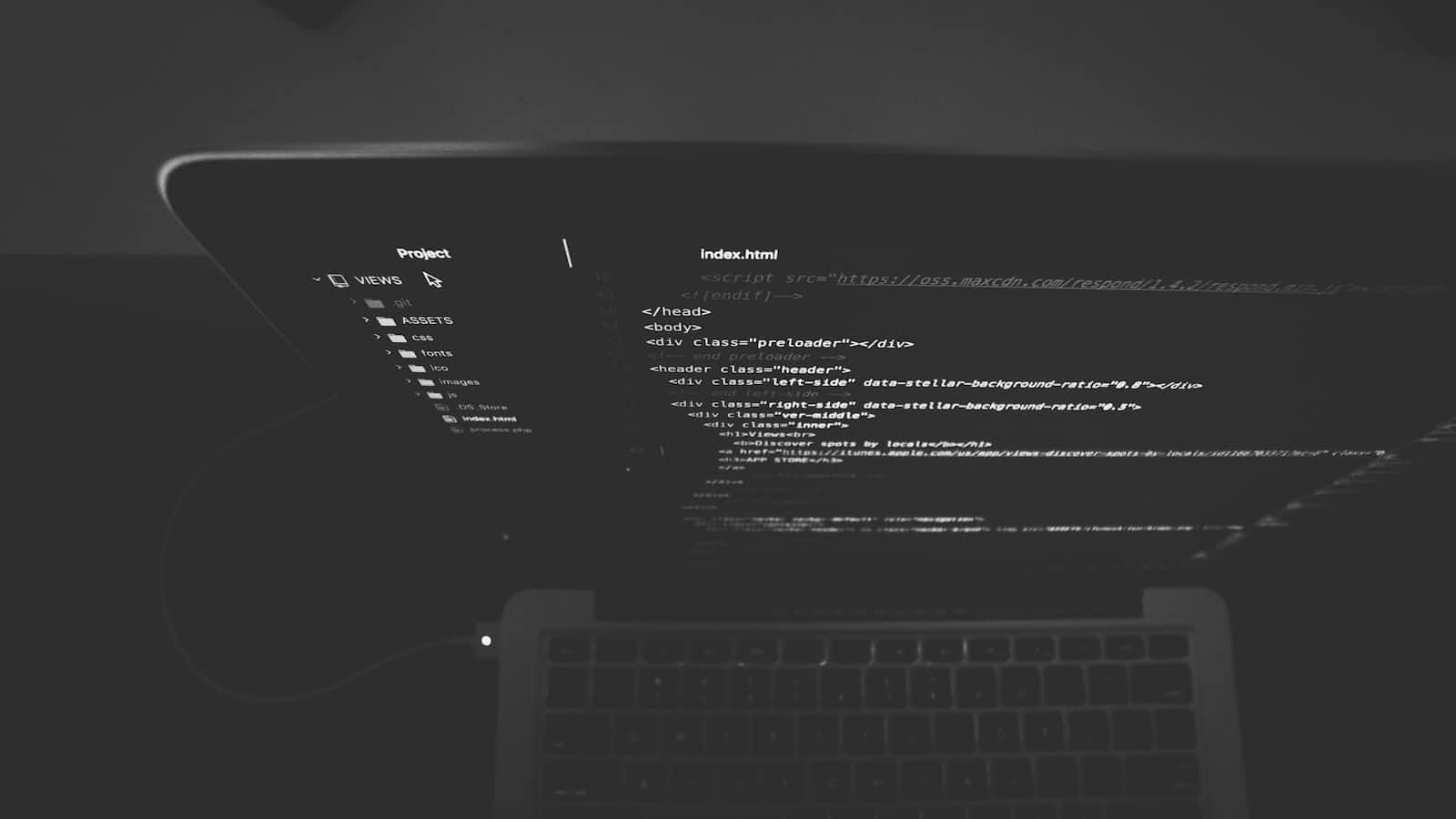
Event Propagation is a mechanism that explains how an event propagates or travels through the dom tree.
Before understanding Event propagation we have to remember one point.
Events are always created whether we listen to them or not.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Event Propagation</title> </head> <body> <section> <p>A paragraph with a <a>Link</a></p> </section> <script> //No script - we are not listening to any event. </script> </body> </html>
In the above example, we have an anchor tag, now if we click on it a click event will be created in the dom tree even if we are not listening to that event.
Let's come back to the Event Propagation,
There are three phases in the process of event propagation, which are as followed,
Capturing Phase: Events are always created at the root element of the dom tree then it propagates down to the target element where that event occurred.
Target Phase: The target phase is when an event is reached by the target after the capturing phase. Now event can be handled on the target element(i.e. where that event has occurred).
Bubbling Phase: After the target phase, the bubbling phase starts, it is the opposite of capturing phase. In this phase, the event propagates upwards to the parent element and all the ancestor elements.
NOTE:
👉 By default events can be handled only in the Target phase and Bubbling phase.
👉 However we can write the event handlers in a way to handle the events in capturing phase, not in the bubbling phase.
🔥Handling Events In The Bubbling Phase
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Event Propagation</title>
</head>
<body>
<section class="section">
<p class="para">A paragraph with a
<a class="link">Link</a>
</p>
</section>
<!-- JavaScript Code To Handle Events-->
<script>
const section = document.querySelector(".section");
const para = document.querySelector(".para");
const link = document.querySelector(".link");
section.addEventListener("click", function(e){
console.log("Click event arrived at section");
console.log("Target is: ", e.target);
console.log("Current Target is: ", e.currentTarget);
})
para.addEventListener("click", function(e){
console.log("Click event arrived at para");
console.log("Target is: ", e.target);
console.log("Current Target is: ", e.currentTarget);
})
link.addEventListener("click", function(e){
console.log("Click event arrived at link");
console.log("Target is: ", e.target);
console.log("Current Target is: ", e.currentTarget);
})
</script>
</body>
</html>
Output: (Click On Link)
Click event arrived at link
Target is: <a></a>
Current Target is: <a></a>
Click event arrived at para
Target is: <a></a>
Current Target is: <p></p>
Click event arrived at section
Target is: <a></a>
Current Target is: <section></section>
🔥Handling Events In The Capturing Phase
By passing true as a third argument to the .addEventHandler(), we can handle events in the capturing phase. Now we will not be able to handle it in the bubbling phase.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Event Propagation</title>
</head>
<body>
<section class="section">
<p class="para">A paragraph with a
<a class="link">Link</a>
</p>
</section>
<!-- JavaScript Code To Handle Events-->
<script>
const section = document.querySelector(".section");
const para = document.querySelector(".para");
const link = document.querySelector(".link");
section.addEventListener("click", function(e){
console.log("Click event arrived at section");
console.log("Target is: ", e.target);
console.log("Current Target is: ", e.currentTarget);
}, true)
para.addEventListener("click", function(e){
console.log("Click event arrived at para");
console.log("Target is: ", e.target);
console.log("Current Target is: ", e.currentTarget);
}, true)
link.addEventListener("click", function(e){
console.log("Click event arrived at link");
console.log("Target is: ", e.target);
console.log("Current Target is: ", e.currentTarget);
}, true)
</script>
</body>
</html>
Output: (Click on link)
Click event arrived at section
Target is: <a></a>
Current Target is: <section></section>
Click event arrived at para
Target is: <a></a>
Current Target is: <p></p>
Click event arrived at link
Target is: <a></a>
Current Target is: <a></a>
Thank You For Reading!♥
If you have any doubts or queries please feel free to ask my in comment section.
Subscribe to my newsletter
Read articles from Akash Deep Chitransh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
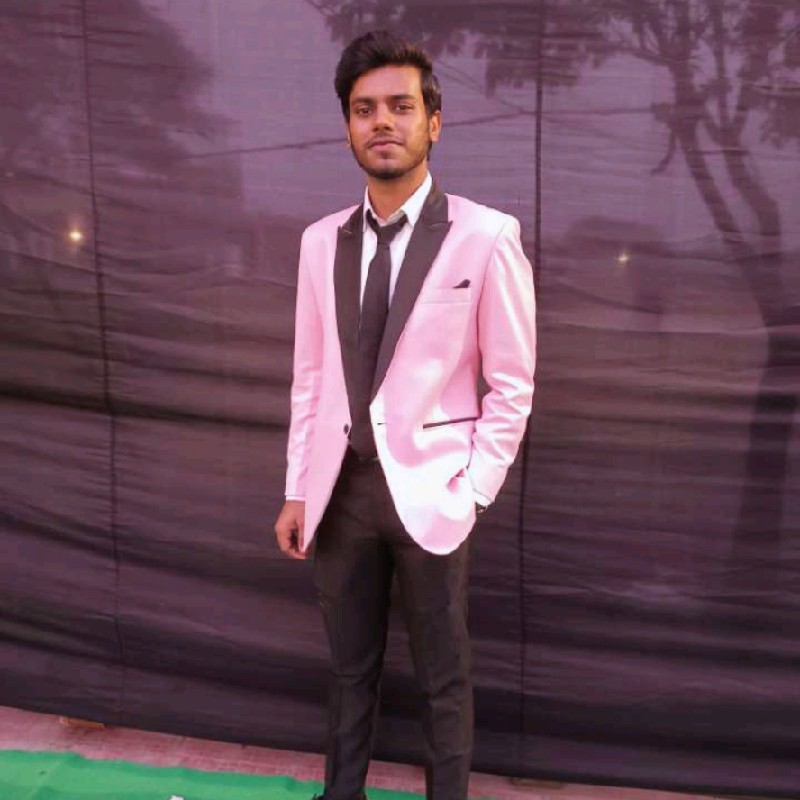
Akash Deep Chitransh
Akash Deep Chitransh
I am a front-end developer, currently working at TCS.