EcmaScript 6 - Map method in JavaScript
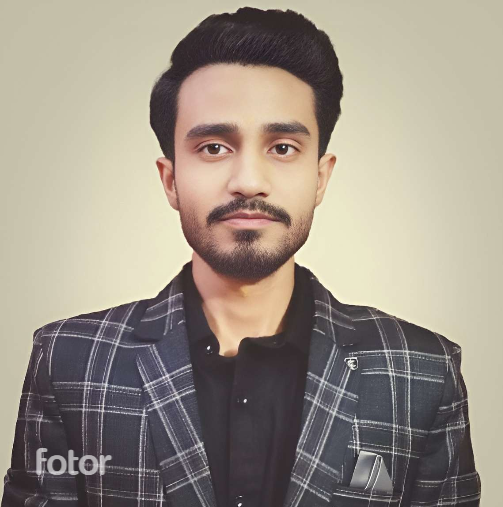
A Map object can be used to hold key/value pairs. A key or value in a map can be anything (objects and primitive values).
The syntax new Map([iterable]) creates a Map object where iterable is an array or any other iterable object whose elements are arrays (with a key/value pair each).
An Object is similar to Map but there are important differences that make using a Map preferable in certain cases:
1) The keys can be any type including functions, objects, and any primitive.
2) You can get the size of a Map.
3) You can directly iterate over Map.
4) Performance of the Map is better in scenarios involving frequent addition and removal of key/value pairs.
The size property returns the number of key/value pairs in a map.
For example:
let map = new Map([['k1', 'v1'], ['k2', 'v2']]);
console.log(map.size); // 2
Methods of Map in JavaScript
set(key, value) Adds a specified key/value pair to the map. If the specified key already exists, a value corresponding to it is replaced with the specified value.
get(key) Gets the value corresponding to a specified key in the map. If the specified key doesn't exist, undefined is returned.
has(key) Returns true if a specified key exists in the map and false otherwise.
delete(key) Deletes the key/value pair with a specified key from the map and returns true. Returns false if the element does not exist.
clear()
Removes all key/value pairs from the map.
keys()
Returns an Iterator of keys in the map for each element.
values()
Returns an Iterator of values in the map for each element.
entries()
Returns an Iterator of array[key, value] in the map for each element.
let map = new Map();
map.set('k1', 'v1').set('k2', 'v2');
console.log(map.get('k1')); // v1
console.log(map.has('k2')); // true
for (let kv of map.entries())
console.log(kv[0] + " : " + kv[1]);
The above example demonstrates some of the ES6 Map methods, rest you have to explore with your 100% pure practice...
Note:-
Map supports different data types i.e. 1 and "1" are two different keys/values.
Hey guys there is a practice question for you guys as well, for Map... i.e.,
Question:
Five employees at a company are stored in Map in the program you are given. Their names are set as keys and their positions as values. The company is hiring one more employee. The program should take the name and the position as inputs and store them in the existing map.
Complete the program to perform that operation and output to the console the list of employees in the format shown in the sample output.
Sample Input
Bob
Developer
Sample Output
Richard : Developer
Maria : SEO Specialist
Tom : Product Manager
David : Accountant
Sophia : HR Manager
Bob : Developer
HINT:- The entries() method returns an Iterator of array[key, value] in the map for each element. Don't forget to put spaces before and after the colon (:) in the output.
Guys, don't worry if you are not able to figure it out, you may go through its solution as well... But first, try to your end.
I am putting the answer at the extreme end of this article...
.
.
.
.
.
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
..
Solution of the problem.
function main() {
var name = readLine();
var position = readLine();
let employees = new Map([
["Richard", "Developer"],
["Maria", "SEO Specialist"],
["Tom", "Product Manager"],
["David", "Accountant"],
["Sophia", "HR Manager"]
]);
//add the new pair to the map
employees.set(name,position);
for (var i of employees.entries()){
//interating every entry of array
//and printing the values as expected.
console.log(i[0] + " : " + i[1]);
}
}
Hope I was able to clear my point, if you have any doubt(s) or suggestion(s) then comment to me I will try to reply as soon as possible. Thanks for reading this article.
Subscribe to my newsletter
Read articles from Jayesh Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
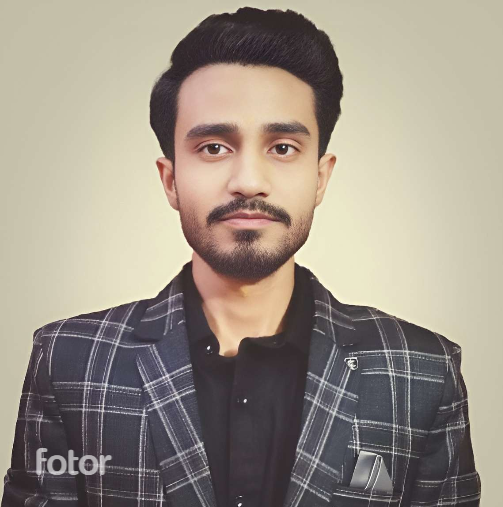
Jayesh Chauhan
Jayesh Chauhan
Hey I am MERN Developer.