Managing Query Parameters in Angular: A Guide
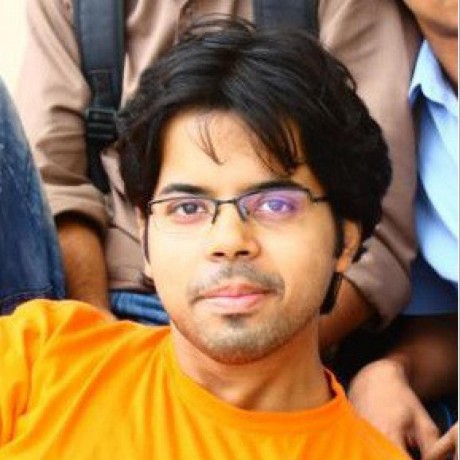
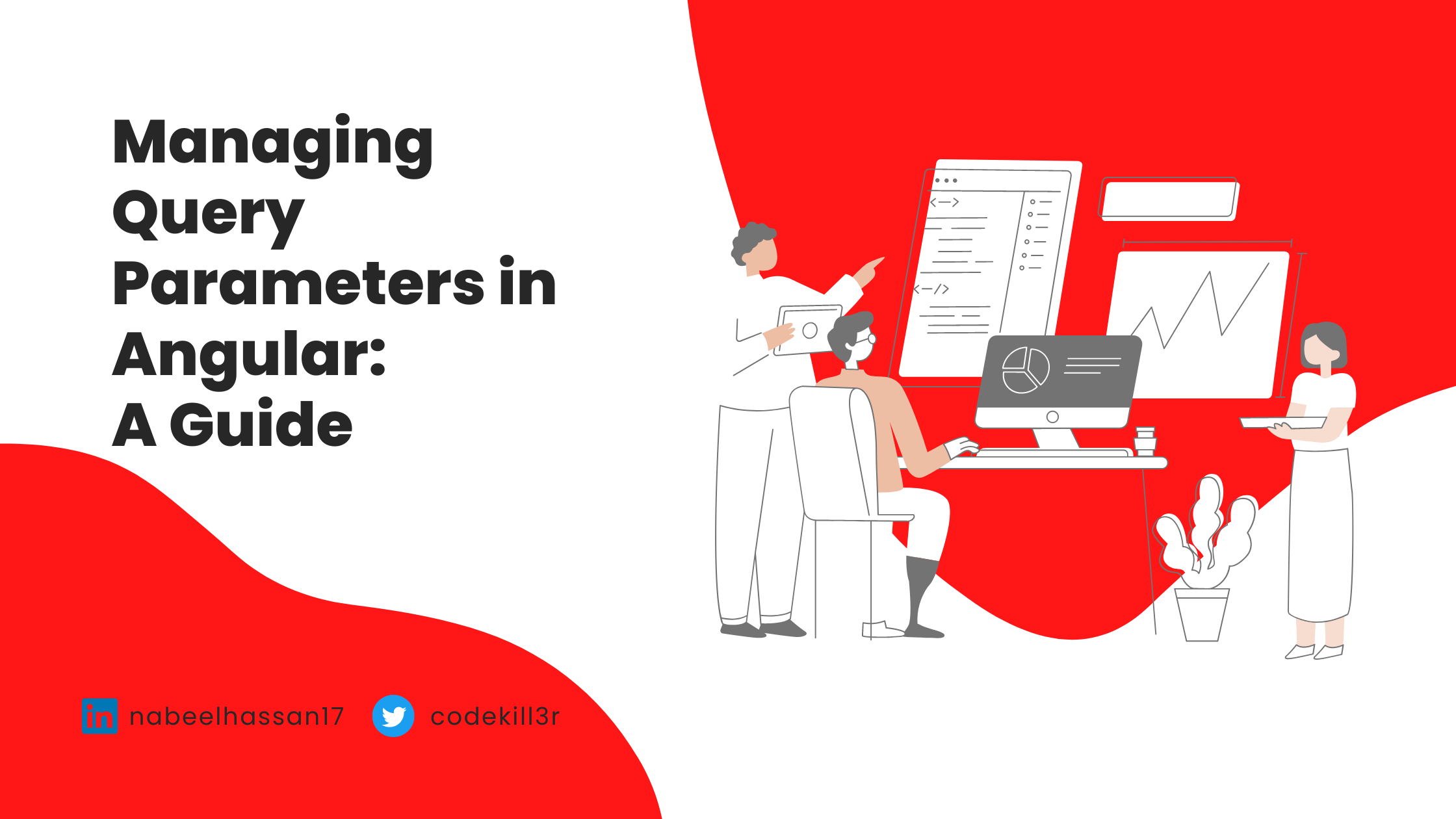
Introduction
When it comes to routing in Angular, query parameters play a crucial role in providing additional information to the router for navigating to a specific route. However, handling query parameters can sometimes become complex, especially when dealing with multiple values for the same key.
In this article, we will cover the basics of query parameters in Angular, and then dive into some real-world scenarios where you might need to pass multiple values for the same key. We will provide practical examples and solutions for handling such cases.
Basics
First, let's start with a basic understanding of what query parameters are and how they work in Angular. In simple terms, query parameters are additional information that you can pass to a route, in the form of key-value pairs, after a question mark (?) in the URL. The official router guide from angular is available here.
Using Router.navigate
In Angular, you can pass query parameters using the queryParams
property of the Router
object.
this.router.navigate(
['/search'],
{ queryParams: { country: 'USA' } }
);
Using the above code, we get the following URL.
https://localhost:4200/search?country=USA
Multiple Key-Values Pairs
By default, Angular only allows one value per key in the query parameters. However, in some cases, you might need to pass multiple values for the same key.
You can pass multiple values for the same key in an object and pass the object as a query parameter to the navigate
method in Angular.
const values = { country1: 'USA', country2: 'UK' };
this.router.navigate(['/search'], { queryParams: values });
In this example, the query parameters are passed as an object with the key queryParams
and the values values
which contains multiple key-value pairs.
Same Key Multiple Values
If you have multiple key-value pairs with the same key in an object, only the last one will be considered in the resulting query parameters.
Consider the following example
const values = { country: 'USA', country: 'UK' };
this.router.navigate(['/search'], { queryParams: values });
In this case, only the recent value of 'country' will be passed.
https://localhost:4200/search?country=UK
If you want to pass multiple values for the same key, you should use an array of values. Here's an example:
const values = { country: ['USA', 'UK'] };
this.router.navigate(['/search'], { queryParams: values });
This will result in a query parameter like country=USA&country=UK
.
https://localhost:4200/search?country=UK&country=UK
Conclusion
In conclusion, query parameters play an important role in Angular routing, and it's important to understand how to handle them properly. Whether you need to pass multiple values for the same key or encode complex data structures, there are solutions available to help you achieve your goals.
Disclaimer
This article was written with the help of ChatGPT (openai.com). No suitable online resource highlighted the usage of passing multiple values to a single key on the internet so I decided to try out ChatGPT. It gave me the solution, so I went on ahead and wrote my first blog.
The opinions and recommendations expressed by ChatGPT do not represent the official views of Angular or OpenAI. Use of the information provided in this article is at the reader's own risk. Although, it works perfectly ;).
Subscribe to my newsletter
Read articles from Nabeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
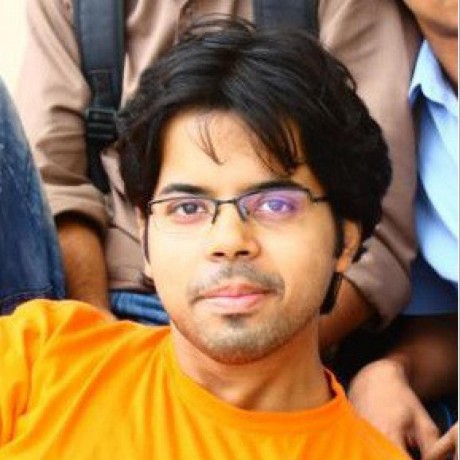