Call(), Bind() and Apply() in JavaScript
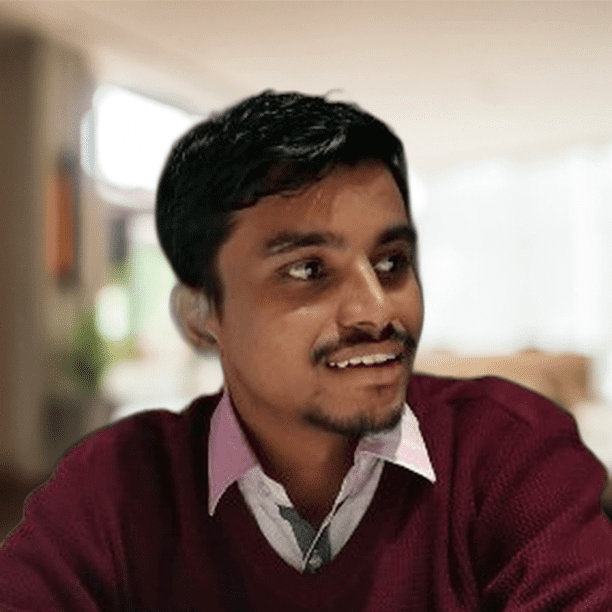
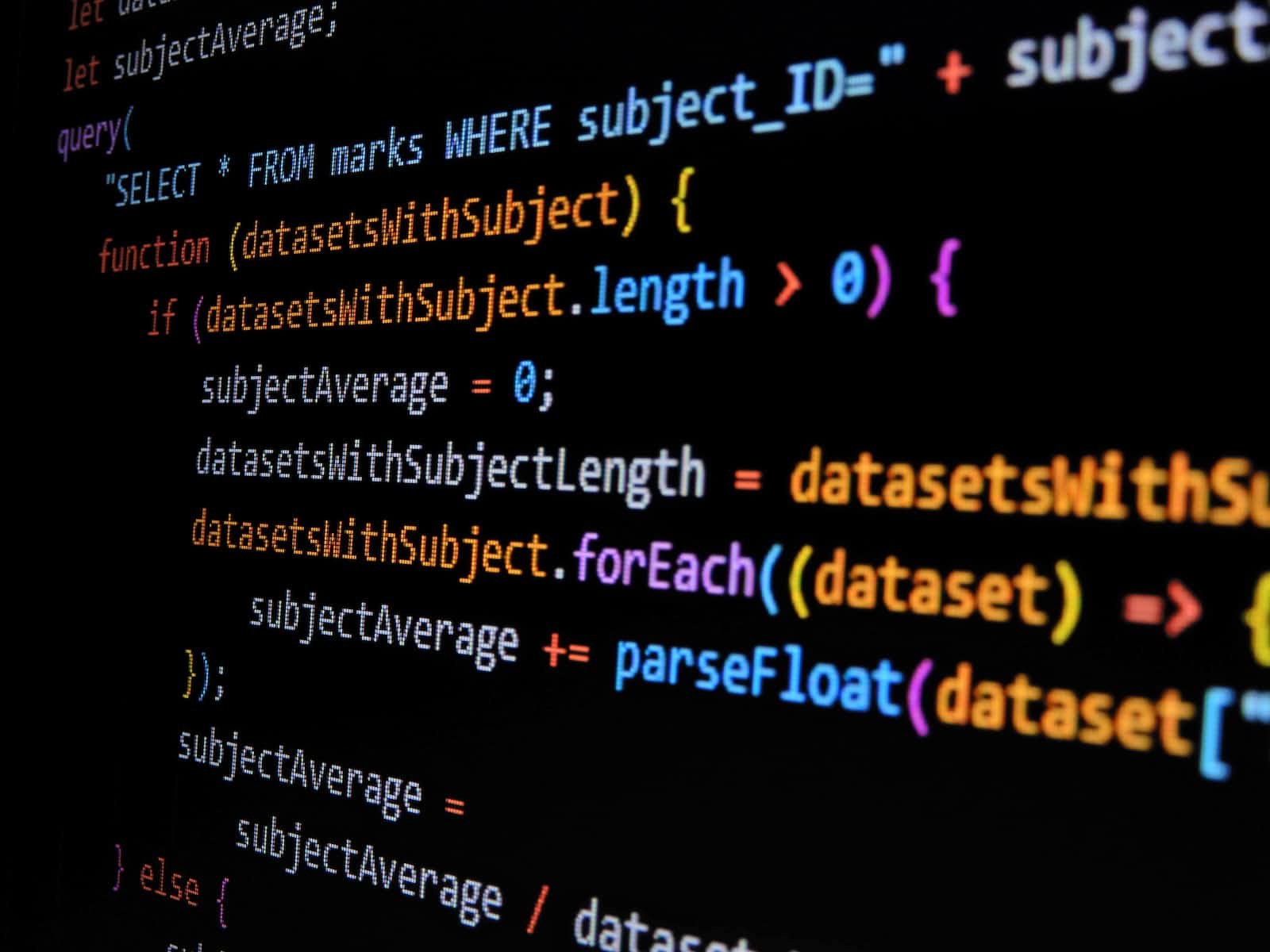
In this article, I am going to explain why we need call apply and bind
how to use call, apply,
and bind
in JavaScript with simple examples.
Before you are going to read this article you should have a basic understanding of how to use this
keyword in Javascript.
call
bind
and apply
methods are used to invoke a function with a specified value and arguments are passed individually to the function.
invoke a function is nothing but calling a function
eg:
const printName = function myName(){
console.log(”My name”)
}
printName();
here printName()
is function invocation
object = {
firstName:'Rohit',
lastName:'Sharma',
age:34
}
function printName(){
console.log("My Name is ",this.firstName, "" ,this.lastName, "age is ", this.age)
}
printName.call(object);
//output
//My Name is Rohit Sharma age is 34
In the above code, there is no use for a call
But below code, I am showing it to you in which case we can use
object ={
firstName:'Rohit',
lastName:'Sharma',
age:34
}
function printName(city,state){
console.log("My Name is ",this.firstName, "" ,this.lastName, "age is ", this.age,"and my city is",city,"from",state )
}
printName.call(object,"Hyderabad","Telangana");
//output
// My Name is Rohit Sharma age is 34 and my city is Hyderabad from Telangana
In the above code, we are passing city and state as arguments to the function printName()
when it comes to apply
it works the same as a call
but instead of passing arguments as separate values, we need to pass them as an array.
object ={
firstName:'Rohit',
lastName:'Sharma',
age:34
}
function printName(city,state){
console.log("My Name is ",this.firstName, "" ,this.lastName, "age is ", this.age,"and my city is",city,"from",state )
}
printName.apply(object,["Hyderabad","Telangana"]);
// output
// My Name is Rohit Sharma age is 34 and my city is Hyderabad from Telangana
when it comes to bind
it returns a new function we need to invoke that function and pass the arguments to that function
in case you didn't understand the above sentence have a look at the below code you get to know how bind works
object ={
firstName:'Rohit',
lastName:'Sharma',
age:34
}
function printName(city,state){
console.log("My Name is ",this.firstName, "" ,this.lastName, "age is ", this.age,"and my city is",city,"from",state )
}
//we can pass arguements like this
const printMyName=printName.bind(object);
printMyName("Hyderabad","Telangana")
//we can pass arguements like this
// const printMyName=printName.bind(object,"Hyderabad","Telangana");
//output
//My Name is Rohit Sharma age is 34 and my city is Hyderabad from Telangana
So, to summarize, you would use call
if you want to invoke a function immediately apply
if you want to pass the arguments as an array, and bind
if you want to create a new function set to a specific value.
Thank you for Reading!
If you like this article please do follow me on Hashnode
Subscribe to my newsletter
Read articles from Chimata Manohar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
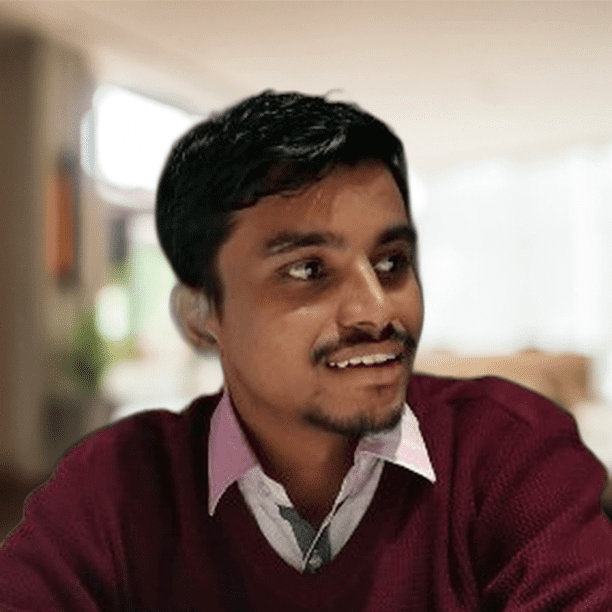
Chimata Manohar
Chimata Manohar
I am a developer from Arshaa Technologies Pvt.Ltd