Type Annotations in TypeScript - Simple & Code Examples

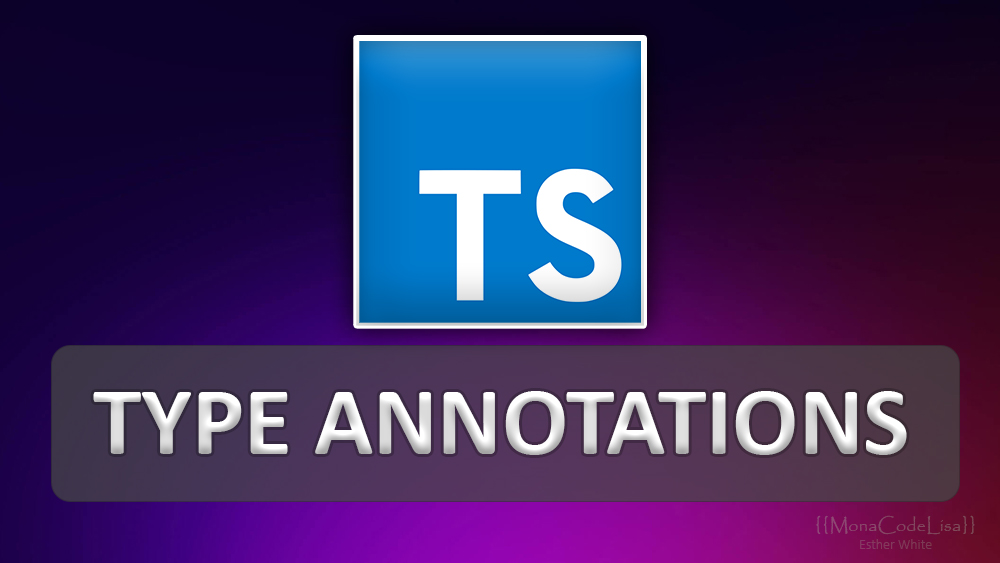
In TypeScript, type annotations are used to specify the data type of a variable, function return type, or function parameter type. They provide the TypeScript compiler with information about the type of data a variable can store, which can help catch type-related errors during development.
Type annotations are added after the variable name and are preceded by a colon (:). For example, the following code demonstrates how to use a type annotation to declare a variable in TypeScript:
let name: string = "John Doe";
let age: number = 30;
let isMarried: boolean = false;
In the above code, the string
type annotation is added to the name
variable, the number
type annotation is added to the age
variable, and the boolean
type annotation is added to the isMarried
variable.
In addition to primitive data types, TypeScript also supports more advanced types such as arrays and objects, and you can use type annotations to specify the type of data stored in arrays and objects.
For example, the following code demonstrates how to use a type annotation to declare an array in TypeScript:
let fruits: string[] = ["apple", "banana", "orange"];
In the above code, the string[]
type annotation is added to the fruits
array, indicating that it can only store strings.
Similarly, you can use type annotations to declare objects in TypeScript:
let person: {
firstName: string,
lastName: string,
age: number,
isMarried: boolean,
job: string
} = {
firstName: "John",
lastName: "Doe",
age: 30,
isMarried: false,
job: "Developer"
};
In the above code, the {firstName: string, lastName: string, age: number, isMarried: boolean, job: string}
type annotation is added to the person
object, indicating that it can only store properties with the specified types.
Type annotations are an important aspect of writing TypeScript code and provide several benefits, including improved code readability, enhanced type checking, and reduced errors. By annotating the data types of variables, functions, and objects, you can make your code more maintainable and prevent type-related issues from arising.
It's important to note that TypeScript is a statically typed language, which means that the data types of variables and other entities must be known at compile-time, rather than at runtime. The use of type annotations allows the TypeScript compiler to perform type-checking during development and can help catch type-related issues before they become problems in your code.
Type annotations are a practical and valuable tool for writing robust and maintainable code in TypeScript. If you are just starting with TypeScript or are looking to improve your TypeScript skills, it's well worth your time to familiarize yourself with type annotations and how to use them effectively.
Subscribe to my newsletter
Read articles from Esther White | EstherSoftwareDev directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Esther White | EstherSoftwareDev
Esther White | EstherSoftwareDev
Hello, I'm Esther White, I am an experienced FullStack Web Developer with a focus on Angular & NodeJS.