Understanding the "window is undefined" error in Next.js and how to resolve it
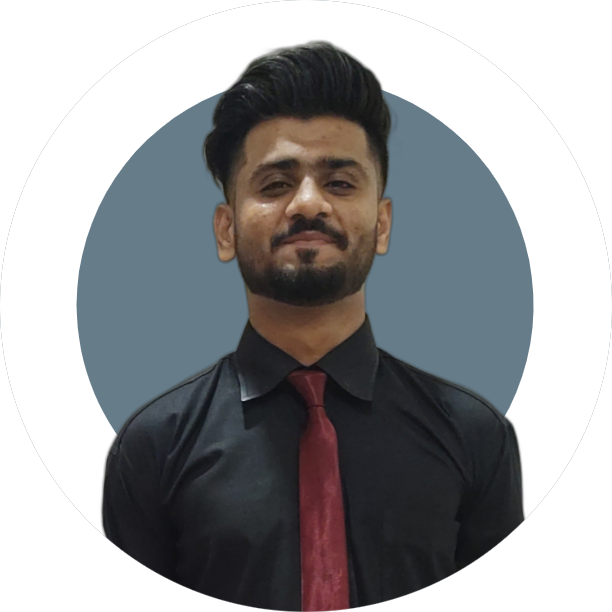
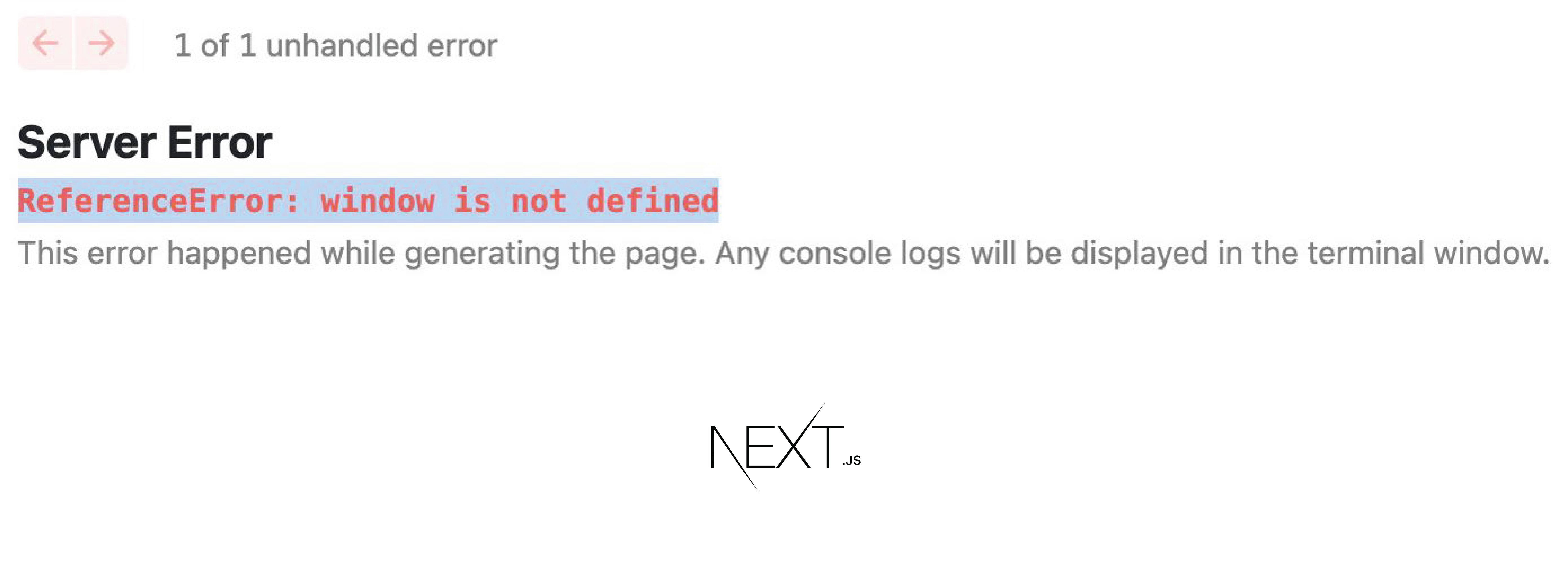
Next.js is a powerful React-based framework for building server-rendered or statically-generated web applications. However, sometimes, developers might face errors when using certain JavaScript methods that are specific to the client side, such as window
, sessionStorage
, and others. In this article, we'll explore the "window is undefined" error and how to resolve it using a simple check.
The "window is undefined" error
This error occurs when trying to access client-side methods on the server side during the initial render. As Next.js initially renders the application on the server side, these client-side methods are not available at that time, causing the application to break. To resolve this issue, the common solution is to make a check and only return the logic when the method is available.
Here's a solution through which we can get rid of this problem, we are using a conditional statement to check if window
is defined. If it is, then we know that we are on the client side and can return the logic for the client side. If not, we return the logic for the server side.
import React from 'react';
const Home = () => {
if (typeof window !== 'undefined') {
// The code inside this block will only run on the client-side
return (
<div>
<h1>Welcome to the client-side</h1>
<p>Here, you have access to window and other client-side methods</p>
</div>
);
}
return (
<div>
<h1>Welcome to the server-side</h1>
<p>Here, you don't have access to window and other client-side methods</p>
</div>
);
};
export default Home;
Subscribe to my newsletter
Read articles from Usama Usman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
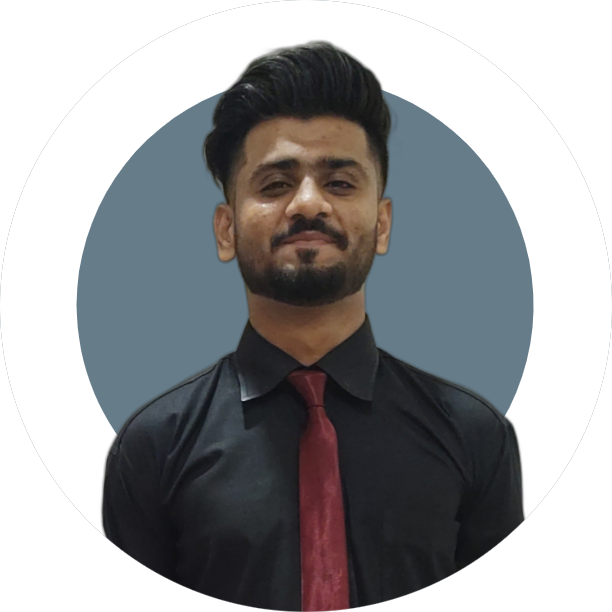
Usama Usman
Usama Usman
An All-Rounder in the field of Computer Science having a skill set that of Development and Design