Python Program to Multiply all the Values in a Dictionary

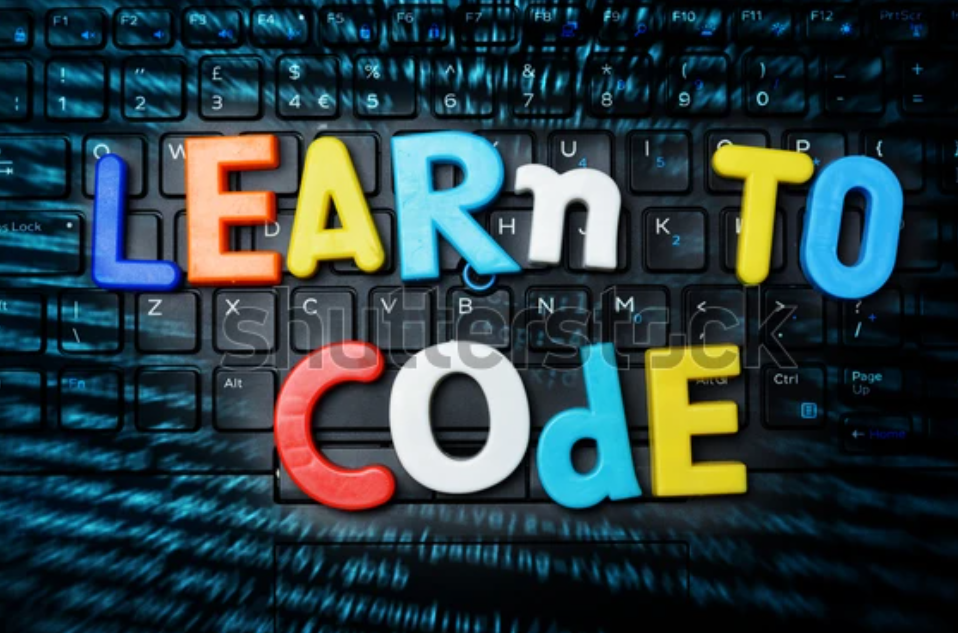
We are going to learn about how can we multiply the values of the dictionary by accessing the values from it in Python.
Example
Input 1: {'maths': 90, 'English': 70, 'Hindi': 90, 'science': 90}
Output: 51030000
Explanation: We use the .values() method to access the values from the dictionary and its return type is 'dict_values' which is similar to a list.
A dictionary is the key value-pair Data Set in which for every key there is a value. The Key is always unique in the dictionary while the value can be duplicated. Syntax of the dictionary is given below:
{keyName1: Value1, KeyName2: Value2, .............}
Access the value list from the Python dictionary with the help of the values function:
# Here we have used the .values() method to access
# the values that will give you the list data type
data = {'Platform': 'GeeksforGeeks',
'Shortname': 'GFG', 'Quality': 'Excellent'}
result = data.values()
print(result)
Output:
dict_values(['GeeksforGeeks', 'GFG', 'Excellent'])
Product of Values in a Python Dictionary
Example 1: Here, we will store the values of the dictionary in a values list and will iterate by using the conditional for loop similar to the list to access the values that we have stored in the values list to multiply all the values one by one.
data = {'maths': 90, 'english': 70,
'hindi': 90, 'science': 90}
# Here we have stored all the key values in valueList
valuesList = data.values()
result = 1
# Here we have just iterated the items of
# list that is valueList
for val in valuesList:
result = result * val
print(result)
Output:
5130000
Example 2: Here, we have taken values as a list for every key and have stored it in a value list and accessed all the items from the value List by a nested loop because every value in the value list is itself a list so the first loop will be used for accessing the list from value-list and second loop for accessing the value from that list and after that, we will multiply all that elements to get the result as a product of values.
data = {'student1': [5, 6, 8],
'student2': [1, 3, 4], 'student3': [8, 9, 10]}
# list of list in valueList
valueList = data.values()
result = 1
# In this loop we are accessing the item
# from the valueList whose type is list
for dataList in valueList:
# In this loop we are accessing the
# value from the dataList
for value in dataList:
result = result * value
print(result)
Output:
2073600
Example 3: This is similar to the above problem but the only difference is that before running the second we will first check the type of value if the value type is 'int' then we can directly multiply and if it is a Python list then we will run the second loop for accessing all the elements and will get the product of the values.
# In valuesList there are types of values
# one is int and other one is list
data = {'student1': [1, 2, 3], 'english': 5,
'maths': 5, 'student2': [5, 6, 7]}
valuesList = data.values()
result = 1
for data in valuesList:
# Here type of data is int So we can directly multiply
if type(data) == int:
result = result * data
# Here type of data is list So we will iterate the
# list to multiply all the items
elif type(data) == list:
for value in data:
result = result * value
else:
continue
print(result)
Output:
31500
Subscribe to my newsletter
Read articles from Prince Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
