Understanding CORS (Cross-Origin Resource Sharing) in JavaScript
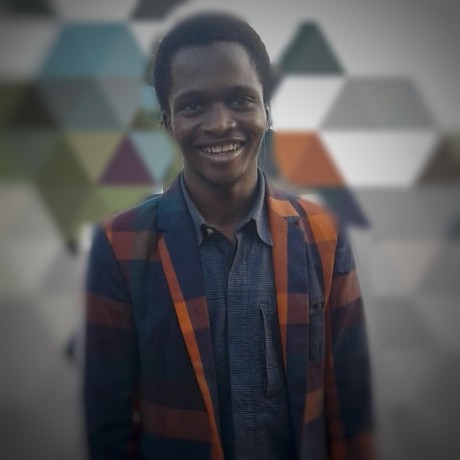
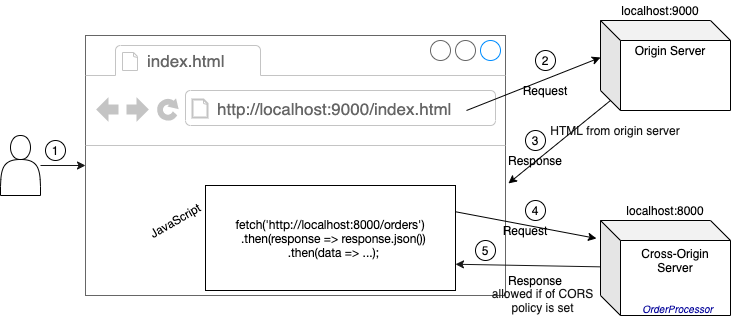
What is Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS) is a security mechanism that restricts a web page from making requests to a different domain than the one that served the web page. Browsers enforce this security restriction through the same-origin policy, which prohibits a web page from making requests to a different domain than the one the web page came from unless the server explicitly allows it.
CORS works by adding HTTP headers to the response of the server, indicating that the server allows requests from a specific origin (domain). When the browser makes a request to a different domain, it first checks the response headers for these CORS headers, and if they are present, the browser allows the request to proceed.
Here's an overview of how CORS works:
The browser sends an HTTP request to the server.
The server checks the request headers to see if the origin of the request is allowed to make the request. This is usually done by checking the
Origin
header in the request.If the origin is not allowed, the server returns an HTTP response with a
403 Forbidden
status code.If the origin is allowed, the server returns an HTTP response with an
Access-Control-Allow-Origin
header, indicating which origins are allowed to make the request. The header can contain a wildcard*
, indicating that any origin is allowed to make the request, or it can contain a specific origin.The browser then checks the
Access-Control-Allow-Origin
header in the response. If the origin of the web page is not listed in the header, the browser blocks the response and the request fails.If the origin of the web page is listed in the header, the browser allows the response to be processed and the request succeeds.
Note that CORS only applies to certain types of requests, such as XMLHttpRequests, Fetch API requests, and WebSockets. Some requests, such as image and script tags, are not subject to CORS restrictions.
CORS headers can be added to the response of the server using server-side code, such as in PHP, Ruby on Rails, or ASP.NET. Here's an example of adding CORS headers in PHP:
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: X-Requested-With");
The example above, Access-Control-Allow-Origin: *
allows requests from any origin, while Access-Control-Allow-Headers: X-Requested-With
allows the X-Requested-With header to be included in the request.
If you're making a CORS request from JavaScript, you can check if the request was successful by checking the status
of the response. If the status
is in the 200-299 range, the request was successful. If the status
is 0, the request was blocked by the browser due to CORS restrictions.
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/data');
xhr.onload = function() {
if (xhr.status >= 200 && xhr.status < 300) {
console.log('Success!', xhr.response);
} else {
console.error('Error', xhr.statusText);
}
};
xhr.send();
In the example above, XMLHttpRequest
is used to make the CORS request. The open
method is used to set the HTTP method (GET in this case) and the URL of the request. The onload
event is used to check the status
of the response and log the response if the request was successful.
Implementing CORS (Cross-Origin Resource Sharing) in JavaScript
There are a few ways to implement CORS in JavaScript, depending on your use case. Here are a few common scenarios:
If you're the owner of the server that will receive the requests, you can add CORS headers to the response. This is the most straightforward way to implement CORS, as it only requires changes on the server side. Here's an example of adding CORS headers in a Node.js Express application:
app.use(function(req, res, next) { res.header("Access-Control-Allow-Origin", "*"); res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept"); next(); });
If you're making a request from a website to an API that you don't control, you can use a CORS proxy. A CORS proxy is a server that acts as an intermediary between your website and the API, adding the necessary CORS headers to the response before sending it back to the browser. This is a useful solution if the API you're using doesn't support CORS.
If you're making a request from a website to an API that you don't control, and you can't or don't want to use a CORS proxy, you can use JSONP (JSON with Padding). JSONP is a technique for sending JSON data in a script tag, rather than as an AJAX request. Because JSONP requests are sent as script tags, they are not subject to the same-origin policy and can be used to make cross-origin requests.
var script = document.createElement('script');
script.src = 'https://example.com/api/data?callback=handleData';
document.body.appendChild(script);
function handleData(data) {
console.log(data);
}
In the example above, a script tag is created and its src
attribute is set to the URL of the API. The callback
parameter is set to the name of a function that will be called when the data is received. The script tag is then added to the page, and the API will respond with a script that calls the handleData
function with the JSON data.
Relevance of CORS to Web Development
CORS (Cross-Origin Resource Sharing) is an important concept in web development because it helps to prevent security vulnerabilities by controlling which resources can be accessed from a web page that came from a different origin.
Here are some reasons why CORS is relevant to web development:
Security: CORS helps to prevent cross-site scripting (XSS) and cross-site request forgery (CSRF) attacks by controlling which resources can be accessed from a web page. This helps to ensure that sensitive information is not leaked to unauthorized parties.
Interoperability: CORS enables different web applications to share resources with each other, even if they are hosted on different domains. This allows for greater interoperability and flexibility in web development.
Data sharing: CORS makes it possible to access data from remote servers, which is useful for a variety of web development tasks, such as retrieving data for a single-page application, or accessing data from third-party APIs.
Cross-domain communication: CORS enables web applications to communicate with each other even if they are hosted on different domains. This is important for creating web applications that can work together to provide a seamless user experience.
In conclusion, CORS is a critical aspect of web development that enables different web applications to interact and share resources, while also helping to ensure that sensitive information is protected from malicious actors.
Subscribe to my newsletter
Read articles from Agbeniga Agboola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
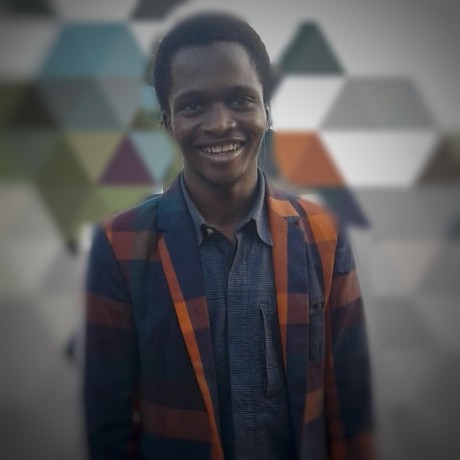