Mastering JavaScript String Properties and Methods.
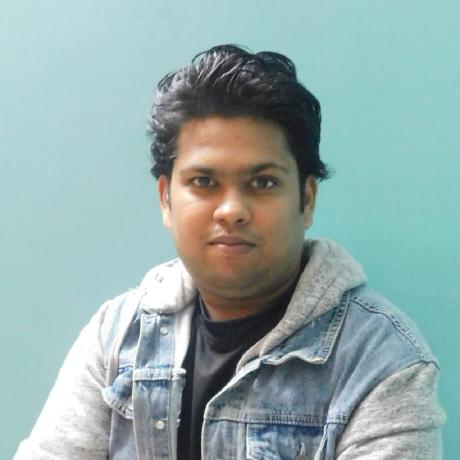
Table of contents
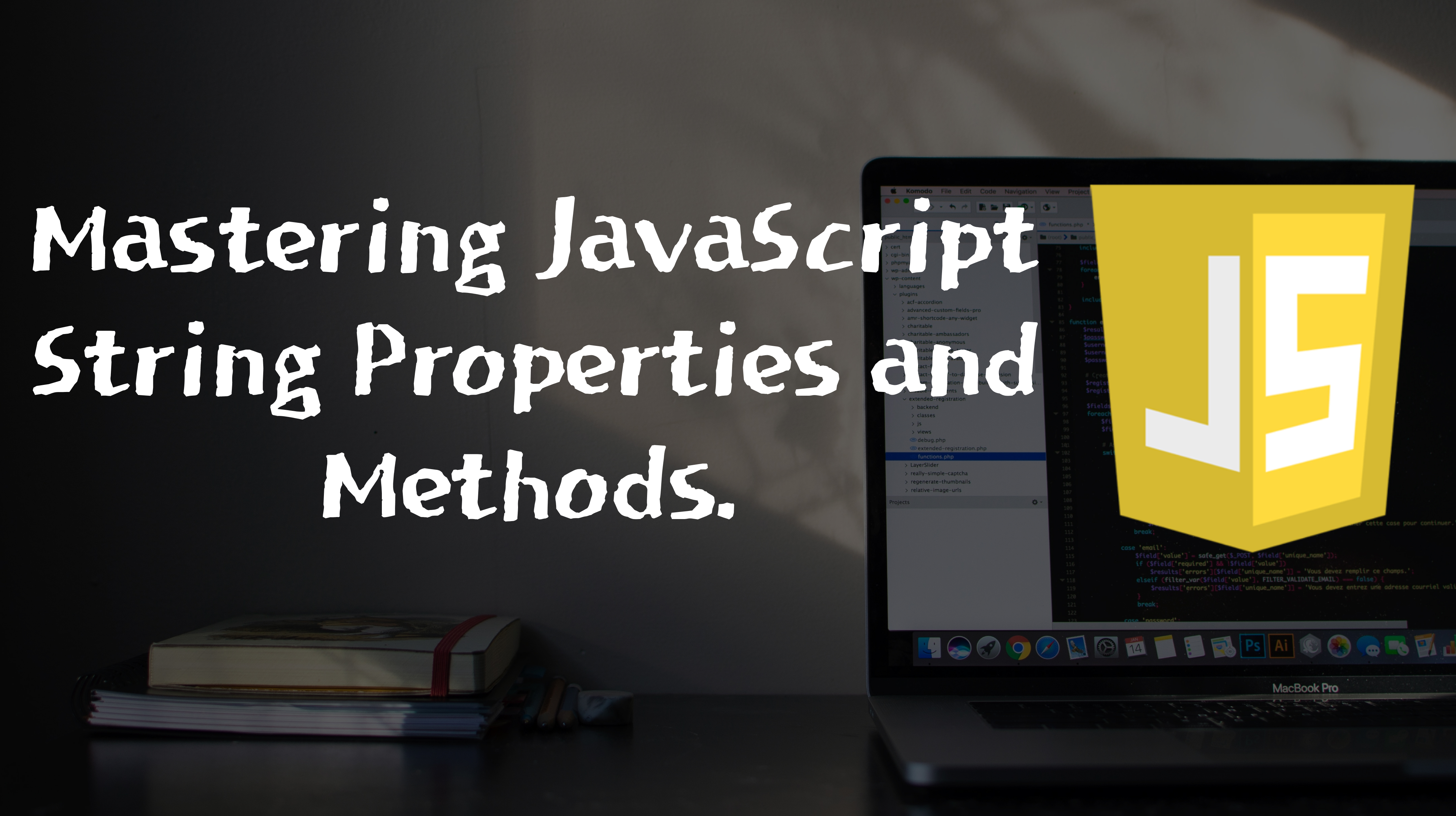
In JavaScript, a string is a sequence of characters used to represent text. Strings are considered primitive data types in JavaScript and are often used to store and manipulate text-based data. Strings can be enclosed in single quotes ('), double quotes ("), or Tempral literal backticks (`). They can be created using string literals or the String object. JavaScript provides a variety of properties and methods for working with strings, such as manipulating the cast of characters, searching for substrings, and concatenating strings. This article will cover the most common and important used string properties and methods in JavaScript.
String Properties:
length: returns the number of characters in the string. Example:
let str = "Hello"; console.log(str.length); // 5
constructor: returns the function that created the string object. Example:
let str = "hello"; console.log(str.constructor); // [Function: String]
prototype: allows you to add new properties and methods to the String object. Example:
String.prototype.greet = function() { return "Hello, " + this; }; let str = "world"; console.log(str.greet()); // Hello, world
String Methods:
charAt(): returns the character at the specified index. Example:
let str = "hello"; console.log(str.charAt(2)); // 'l'
concat(): combines two or more strings into a single string. Example:
let str1 = "hello"; let str2 = "world"; console.log(str1.concat(" ", str2)); // hello world
indexOf(): returns the index of the first occurrence of the search value, starting from the specified index. Example:
let str = "hello world"; console.log(str.indexOf("l")); // 2 console.log(str.indexOf("l", 3)); // 3
lastIndexOf() method returns the index of the last occurrence of a specified value in a string. If the value is not found, the method returns -1. For example:
let str = "Hello World"; console.log(str.lastIndexOf("l")); // 9
trim() This method removes whitespace from both ends of a string. For example:
let str = " Hello World "; console.log(str.trim()); // "Hello World"
toLowerCase() and toUpperCase() These methods convert a string to upper or lower case, respectively. For example:
let str = "Hello World"; console.log(str.toLowerCase()); // "hello world" let str = "Hello World"; console.log(str.toUpperCase()); // "HELLO WORLD"
slice() This method extracts a part of a string and returns a new string. For example:
let str = "Hello World"; console.log(str.slice(0, 5)); // "Hello"
split() method splits a string into an array of substrings based on a specified separator. For example:
let str = "Hello World"; let words = str.split(" "); console.log(words); // ["Hello", "World"]
replace() method replaces a specified value with another value in a string. For example:
let str = "Hello World"; console.log(str.replace("World", "JavaScript")); // "Hello JavaScript"
padStart() and padEnd() methods in JavaScript are string methods that allow you to add padding to the start or end of a string, respectively. They were introduced in ECMAScript 2017 (ES8) and are supported in most modern browsers and Node.js environments.
let originalString = 'Hello'; let paddedString = originalString.padStart(10, '-'); console.log(paddedString); // Output: -------Hello let paddedString = originalString.padEnd(10, '-'); console.log(paddedString); //Output: Hello-------
Conclusion
In conclusion, JavaScript provides a rich set of string methods and properties that allow developers to manipulate and interact with strings in various ways. Whether it's for string concatenation, searching for specific characters, converting to uppercase or lowercase, or formatting strings with padding, JavaScript has a method or property for every need.
Knowing and understanding these methods and properties can greatly improve the efficiency and readability of your code, making it easier to manipulate strings and achieve desired outcomes.
It's also important to note that many of these methods and properties are supported in modern browsers and Node.js environments, but some may not be available in older browsers, so it's always a good idea to check compatibility before using them in production.
Subscribe to my newsletter
Read articles from Bhaskar Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
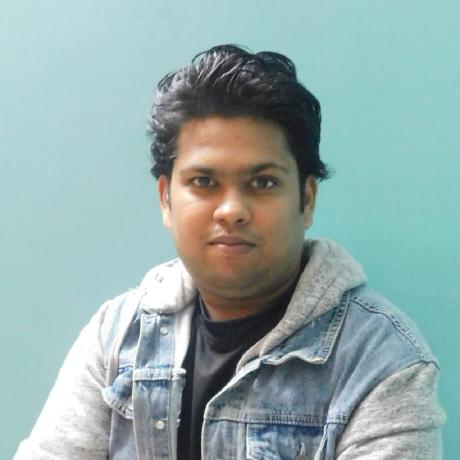