Classes and Constructor Functions in JavaScript

Table of contents
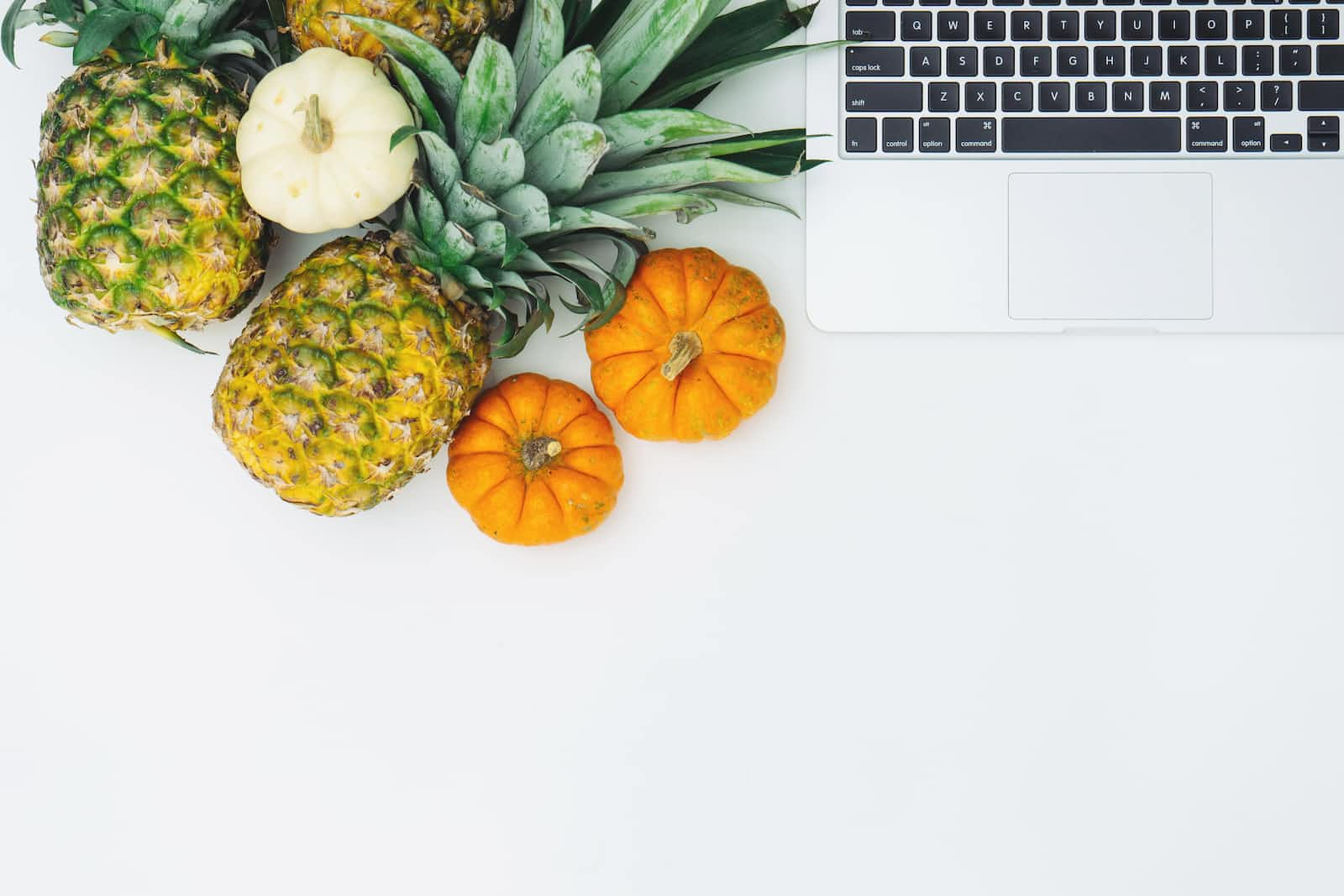
A class is a function that helps to create multiple instances of similar objects. This is similar to constructor functions. Classes and Constructor functions are ways of writing reusable code useful when dealing with objects with similar variables. You can see it as a template of what the programmer wants to happen when they are dealing with similar objects. They are based on OOP; Object Oriented Programming. Let us look at constructor functions before we dive into classes using an object containing fruits. Note, this will aid our understanding of classes and their syntax.
const ObjectOfFruit={
Apple:{
name:'Apple',
isRipe:false,
emoji:'π',
monthToRipen:4
},
Tomato:{
name:'Tomato',
isRipe: false,
emoji:'π₯',
monthToRipen:2
},
Watermelon:{
name:'Watermelon',
isRipe: true,
emoji:'π',
monthToRipen:0
},
Carrot:{
name:'carrot',
isRipe: true,
emoji:'π₯',
monthToRipen:0
}
}
Constructor Functions
Constructor functions are created like this:
function Fruit(data){
}
The name starts with a capital letter to differentiate it from a normal function. it takes a parameter of data (it can be any name of your choice). Inside the constructor function, a major ingredient of its work is this
keyword. The keyword is used to take what we get from the parameter into the constructor and the instance that will later be created. It can also be used to create a variable in the constructor.
function Fruit(data){
this.name = data.name
this.isRipe = data.isRipe
this.emoji = data.emoji
this.monthToRipen = data.monthToRipen
}
This means the name, emoji, isRipe, monthToRipen
that we want to get should be what we get from the data
. Now the major advantage of constructors is their ability to have their functions
this.getFruitDetails = ()=>{ const message =`This is a/an ${this.name} ${this.emoji}. It is ${this.isRipe?'ripe for you to eat':'not ripe for you to eat'}. ${this.monthToRipen>0?`It will take ${this.monthToRipen} to be ready. Be Patient `:'Enjoy!!'}`
console.log(message)
}
The function inside the constructor function is peculiar to it. This is what our constructor function looks like.
function Fruit(data){
this.name = data.name
this.isRipe= data.isRipe
this.emoji = data.emoji
this.monthToRipen = data.monthToRipen
this.getFruitDetails = ()=>{
const message =`This is a/an ${this.name} ${this.emoji}. It is ${this.isRipe?'ripe for you to eat':'not ripe for you to eat'}. ${this.monthToRipen>0?`It will take ${this.monthToRipen} to be ready. Be Patient `:'Enjoy!!'}`
console.log(message)
}
}
Now to create an instance will have.
const apple = new Fruit(ObjectOfFruit.Apple)
To call the function in it:
const apple = new Fruit(ObjectOfFruit.Apple)
apple.getFruitDetails()
result: This is a/an Apple π. It is not ripe for you to eat.
It will take 4 to be ready. Be Patient
Another example:
const carrot = new Fruit(ObjectOfFruit.Carrot)
carrot.getFruitDetails()
result: This is a/an carrotπ₯. It is ripe for you to eat. Enjoy!!
Classes
The work of constructor function and classes are similar. In terms of syntax, There are some changes. We create classes with class,
not function
.
class Fruit {
}
You will notice that the class did not take a parameter, this is because classes have a constructor method in them which is compulsory. So it is inside the constructor that we will define everything that will make use of this
keyword. The functions created inside classes do not take this
and they are created normally without the function keyword in front of them.
class Fruit {
constructor(data) {
this.name = data.name
this.isRipe = data.isRipe
this.emoji = data.emoji
this.monthToRipen = data.monthToRipen
}
getFruitDetails(){
const message = `This is a/an ${this.name} ${this.emoji}. It is ${this.isRipe ? 'ripe for you to eat' : 'not ripe for you to eat'}. ${this.monthToRipen > 0 ? `It will take ${this.monthToRipen} to be ready. Be Patient ` : 'Enjoy!!'}`
console.log(message)
}
}
This is basically how a class is created. You can do a lot of things in them. The possibilities are endless.
Object.assign
Imagine if our class was not taking just 4 items from data. what if we were taking 10 or 20 or more? It will be cumbersome to write this.name = data.name
and other things. This is where Object.assign comes in. Object.assign is an object method that takes the values of one object and gives them to another. For example;
const student= {
name: "Jude",
age:26,
grades: "Excellent",
NoOfCourses: 9,
year: 5
}
let studentCopy ={}
Object.assign(studentCopy, student)
console.log(studentCopy)
result: Object { name: "Jude", age: 26, grades: "Excellent", NoOfCourses: 9, year: 5 }
(You can try it out and check your console). So studentCopy to attributes of the student through Object.assign.
In English, I think of it as "Assign to studentcopy, student ". Hence, what we need to do to copy what we have inside of data is to say "assign to this, data". Imagine telling a friend to take a task and assign it to someone. So we are assigning data to this
, for this
to use. Like that data.name
will be equal this.name
without writing it out. So why don't you try to replace all this.name, this.emoji
etc with Object.assign(this,data)
. Easy right!
While we have constructor functions and classes. Classes are more widely used as you will meet them in other frameworks and programming languages.
Reference
Subscribe to my newsletter
Read articles from Oladayo Ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
