JS Debugging 101: Differentiating TypeError and ReferenceError

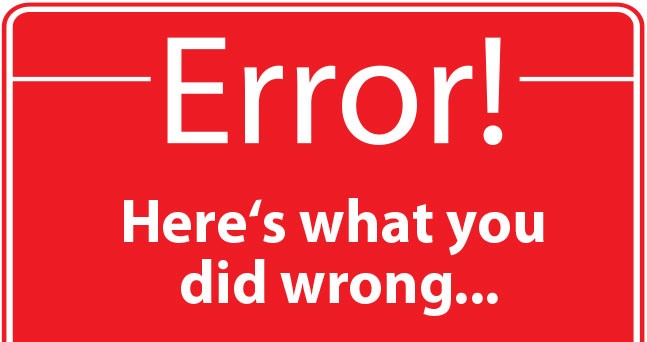
"Errors come in all shapes and sizes, but at the end of the day, they all mean the same thing - time for some debugging!"
A ReferenceError in JavaScript is when you try to use a variable or a function that hasn't been declared or defined yet.
Think of it like in a big library when you want to find a book, you look up its title in the library's catalogue to find out where it's located on the shelves. If the book isn't in the catalogue, you can't find it in the vast library, even if it's there.
In JavaScript, the memory is the catalogue. If you try to use a variable or a function that isn't in the memory, JavaScript won't be able to find it and it will give you a ReferenceError.
Instances that result in ReferenceError are:
Using an undeclared variable
console.log(x); // ReferenceError: x is not defined
Typo in a variable name
let name = "Yash"; console.log(nam); // ReferenceError: nam is not defined
Accessing a variable in a different scope
function whatIsX() { {let x=5;} console.log(x); } whatIsX(); //ReferenceError: x is not defined
Reference a variable before it's declared (when declared with
let
orconst
keyword)function myNum(){ console.log(num); let num = 5; } myNum(); //ReferenceError: Cannot access 'num' before initialization
A TypeError occurs when you try to perform an operation or call a method on a value that is not of the expected type. In other words, you are trying to use a value in a way that it doesn't support.
It's like trying to fit a square block into a round hole. The block is a good thing, but it just doesn't fit in that hole.
Instances that result in TypeError are:
Reassignment to Const variable
const val = 2; val = 3 //TypeError: Assignment to constant variable.
Calling a method on a value that is not of the expected type
let num=5; num.toUpperCase(); //TypeError: num.toUpperCase is not a function
Calling a non-function value
let obj = {}; obj(); //TypeError: obj is not a function
Accessing a property of
null
orundefined
let a = null; console.log(a.property); //TypeError: Cannot read properties of null
Trying to use an object that does not have the expected method
let obj = {}; obj.method(); //TypeError: obj.method is not a function
So, What did this teach us?
To avoid ReferenceError, make sure you have declared and defined all the variables and functions you need at the top before you try to use them.
To avoid TypeError, make sure you are using values in a way that they can be used
Subscribe to my newsletter
Read articles from Shushma Muniraj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
