RUBY (part-7)

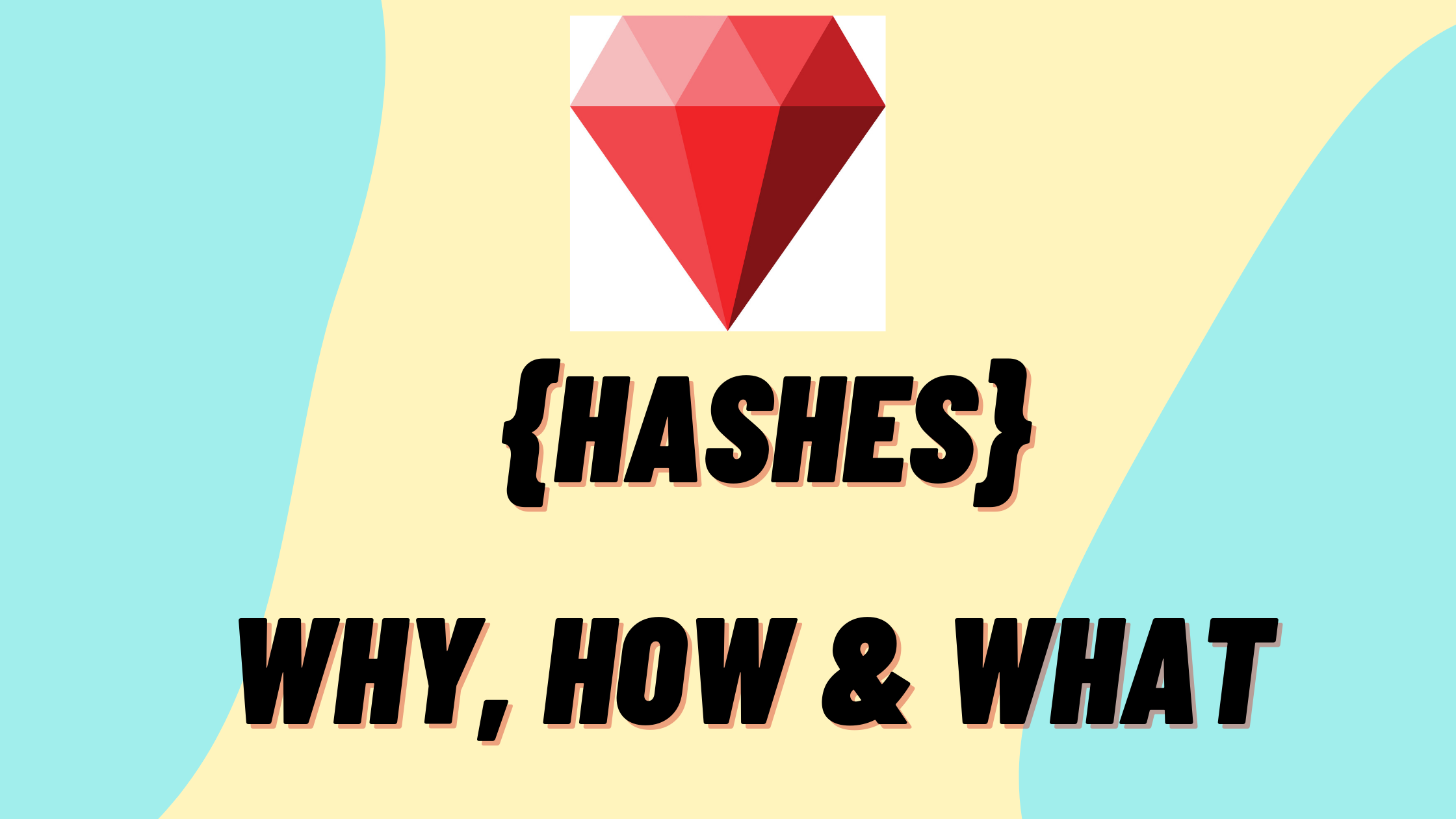
Introduction
My small please is don't take it as a new topic/concept because it is the same as arrays that we learned in the previous blog. The idea behind both concepts is similar with very few different perspectives.
Why
In the introduction, we read that both arrays and hashes have the same frankly the idea behind the implementation of both are same. But they differ when it comes to functionality, operations and even more things.
Let's look into the official definition of hash data structure
The hash data structure is used to store the data in the form of unique key-value pairs.
Let's break it
store the data -> In this sentence, the data might be of any type like integer, string, float, or even another array or hash.
in the form of unique key-value pair -> Here is the heart of the hash data structure lie in. Let's understand it in deep.
In the arrays, each object can be accessed by using the indices. Assigning the indices values is done internally. When it comes to hashes we don't need to use the indices more.
It is letting us define a name for the data that we want to store. Technically the name that we define is called a "key" and the data we have stored in the key is called "value". That's it readers as simple as it is.
In the arrays, there is no chance that 2 different objects are stored in the same index. The same thing happens in the hashes we don't name 2 different values with the same name. That's the meaning of unique key-value pairs.
The main intention behind the hashes is to get more access flexibility. But it doesn't mean that arrays won't allow us to access it DO yet hash is more specific.
That's all about why we need hashes.
How
If you are from any programming language we generally heard about dictionaries and it is somewhat similar to that only. The representation is also selfsame.
There are so many ways to represent hashes in ruby. In that, too many ways the idea is interchangeable i.e., We want to place the key-value pairs in the curly/{ } braces where the array objects are placed in the square/[ ].
Implicit form
This is one of the ways to implement arrays. The keys are assigned to the values using the "=>" (hash rocket) this symbol. Remember this is not only the one way to assign but this is the most conventional way. Let's try to code our first-ever hash.
full_name = { "first_name" => 'malavi', "secod_name" => 'pande'}
print full_name
output:
{ "first_name" => 'malavi', "secod_name" => 'pande}
Using new method
Like in the arrays, we can define an empty hash using the Hash class and new method. let's try to implement it this way. We can also place a default value in the hash it executes when we try to access values from the keys that we have not defined.
full_name = Hash.new(default value)
print full_name
output:
{}
The alternate syntax for keys
This is only for key representation. In the above, both the keys and values are placed between the single or double quotes. Placing a colon in front of keys can do the same thing as above.
full_name = {:first_name => "malavi", :second_name => "pande"}
print full_name
output:
{:first_name => "malavi", :second_name => "pande"}
Accessing
There are two mostly used accessing techniques are there in ruby.
Using Keys
Like in arrays, we can access the objects using indices by placing index values in the subscripts/[]. The same process is in the hash also but in place of the index value we have to place the key of that particular object, we want to access.
full_name = {:first_name => "malavi", :second_name => "pande"}
print full_name[:first_name] #prints the value associated with the key
output:
malavi
Using fetch method
It is the hash inbuilt method used to access the values associated with that particular key. It takes one value as an argument in that we have to define the keys only.
full_name = {:first_name => "malavi", :second_name => "pande"}
print full_name.fetch(:second_name)
output:
pande
Inbuilt Methods
Like arrays hash data structures also have a decent number of inbuilt methods to get things done fast. But we have to do a bit careful while working with them.
\== operator
Tests whether two hashes are equal or not and returns a boolean value as a result.
a = { :one => 1, :two => 2}
b = { :one => 1, :two => 2}
print a == b
output:
true
Note: It returns true only when keys and values in both the hashes should be the same.
Merge two ruby hashes
Merging nothing but we are trying to join the 2 hashes this would be super easy in the arrays but it is not a cool case in hashes. Because each value is associated with a unique key and because of these many items in the hash it is a little bit confusing while merging.
But we can easily do this by a simple hash method. "merge"
The syntax: hash_1.merge!(hash_2)
a = { :one => 1, :two => 2}
b = { :three => 3, :four => 4}
print a.merge!(b)
output:
{ :one => 1, :two => 2, :three => 3, :four => 4}
In the hash_s place, we can direct put key-value pairs no worries. There is an edge case what if we have the same key-value pairs in both hashes? The solution for this is can replace the old pair with the new one. DO try this too
a = { :one => 1, :two => 2}
b = { :one => 3, :two => 4}
print a.merge!(b)
output:
{ :one => 3, :two => 4}
Sort the Hash
In simple terms, sort can be defined as arranging the items in ascending or descending order. The question arises on which bases the sorting can be done. Super cool question and the answer is based on the key only the sorting takes place.
While exchanging the places of values remain the same but the keys change their position.
a = { :two => 1, :one => 2, :three => 3, :four => 4}
print a.sort
output:
[[:four => 4], [:one => 1], [:three => 3], [:two => 2]]
Note: After sorting it returns the result in the form of a "list" only
Keys & Values
we can access only keys and only values by these two methods. It is too returns the result in the form of a list only.
a = { :two => 1, :one => 2, :three => 3, :four => 4}
print a.keys
print a.values
output:
[:four, :one , :three ,:two ] [1,2,3,4]
Empty?
This method returns a boolean value based on whether the provided hash is empty or not.
a = { :two => 1, :one => 2, :three => 3, :four => 4}
print a.empty?
output:
false
note: Don't forget to place a "?" symbol at the end
Conclusion
There are many methods to work but it's not possible to discuss all of them. If you are interested in knowing those click the link below you can directly navigate to all hash inbuilt methods.
That's all about the hashes readers. Thanks for reading feel very free to comment below on how the blog helps me in growing in all ways.
Subscribe to my newsletter
Read articles from Malavi Pande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Malavi Pande
Malavi Pande
A young woman utterly captivated by the pursuit of knowledge.