Exploring the Most Common Searching Algorithms: Linear, Binary, Interpolation, and Hash Tables
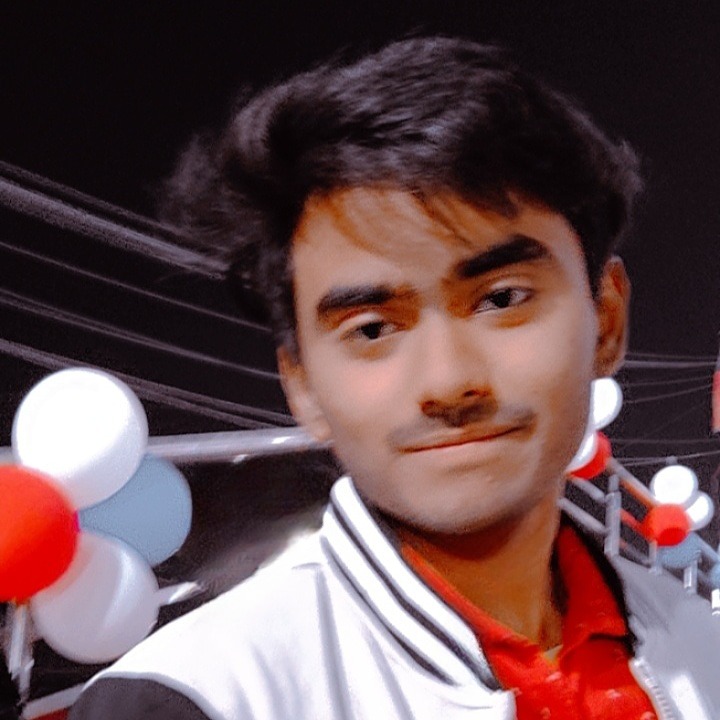
Searching algorithms are a crucial part of computer science and are used in a wide variety of applications, from web search engines to computer games. The goal of a searching algorithm is to efficiently find a specific item in a large collection of data. There are many different searching algorithms, each with its own strengths and weaknesses. In this blog post, we will discuss some of the most commonly used searching algorithms.
Linear Search:
Linear search, also known as sequential search, is the simplest and most basic searching algorithm. It works by checking each item in the collection until the target item is found. If the target item is not in the collection, then the algorithm will continue to search until the end of the collection is reached.
The time complexity of a linear search algorithm is O(n), where n is the number of items in the collection. This means that the time taken to search through the collection increases linearly with the size of the collection.
def linear_search(collection, target):
for item in collection:
if item == target:
return True
return False
Binary Search:
Binary search is a more efficient searching algorithm than linear search. It works by dividing the collection in half repeatedly until the target item is found. The algorithm compares the target item with the middle item in the collection. If the target item is greater than the middle item, then the algorithm searches the right half of the collection. If the target item is less than the middle item, then the algorithm searches the left half of the collection. The process is repeated until the target item is found or until there are no more items to search.
The time complexity of a binary search algorithm is O(log n), where n is the number of items in the collection. This means that the time taken to search through the collection increases logarithmically with the size of the collection. Binary search is faster than linear search for large collections, but it requires the collection to be sorted.
def binary_search(collection, target):
low = 0
high = len(collection) - 1
while low <= high:
mid = (low + high) // 2
if collection[mid] == target:
return True
elif collection[mid] < target:
low = mid + 1
else:
high = mid - 1
return False
Interpolation Search:
Interpolation search is a variation of binary search that is used when the data in the collection is uniformly distributed. It works by calculating an estimate of the position of the target item based on its value and the values of the first and last items in the collection. The algorithm then checks the estimated position to see if it contains the target item. If the estimated position is too high or too low, then the algorithm adjusts the estimate and repeats the process.
The time complexity of an interpolation search algorithm is O(log log n) in the best case and O(n) in the worst case. This means that interpolation search is faster than binary search for uniformly distributed data, but it can be slower in other cases.
def interpolation_search(collection, target):
low = 0
high = len(collection) - 1
while low <= high and target >= collection[low] and target <= collection[high]:
pos = low + ((target - collection[low]) * (high - low)) // (collection[high] - collection[low])
if collection[pos] == target:
return True
elif collection[pos] < target:
low = pos + 1
else:
high = pos - 1
return False
Hash Tables:
Hash tables are a data structure that is used to implement fast searching algorithms. They work by using a hash function to map the key of each item to a unique index in an array. The key is then used to access the item at the corresponding index in the array. Hash tables have an average time complexity of O(1) for search operations, but the worst case time complexity is O(n), where n is the number of items in the table.
Hash tables are often used to implement search engines and databases, where fast searching is critical.
def hash_table_search(table, key):
index = hash_function(key) % len(table)
while table[index] is not None:
if table[index].key == key:
return table[index].value
index = (index + 1) % len(table)
return None
Conclusion:
In conclusion, searching algorithms are an essential part of computer science and are used in many applications. The choice of algorithm depends on the size and nature of the data, as well as the requirements of the application. Linear search is the most basic searching algorithm, but it is not very efficient for large collections. Binary search is faster than linear search for large collections, but it requires the data to be sorted. Interpolation search is a variation of binary search that is faster for uniformly distributed data, but it can be slower in other cases. Hash tables are a data structure that provides fast searching for a wide variety of applications.
Subscribe to my newsletter
Read articles from Mohammad Ruman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
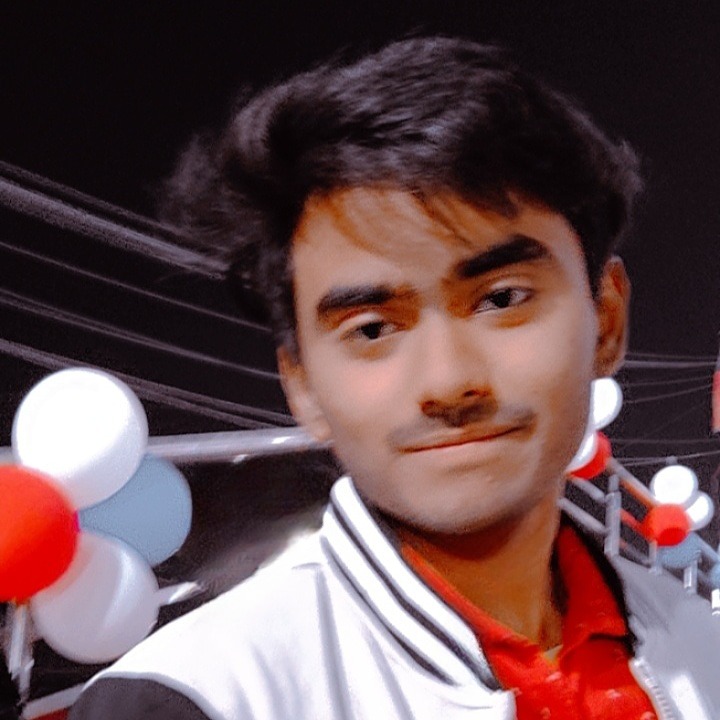
Mohammad Ruman
Mohammad Ruman
I am Mohammad Ruman, currently pursuing my BTech majoring in computer science from Kalinga Institute Of Industrial Technology. I am a tech enthusiast and always open to collaborating on projects and innovative ideas. Find out more about me & feel free to connect with me here: