Sorting Algorithms: Bird's eye view
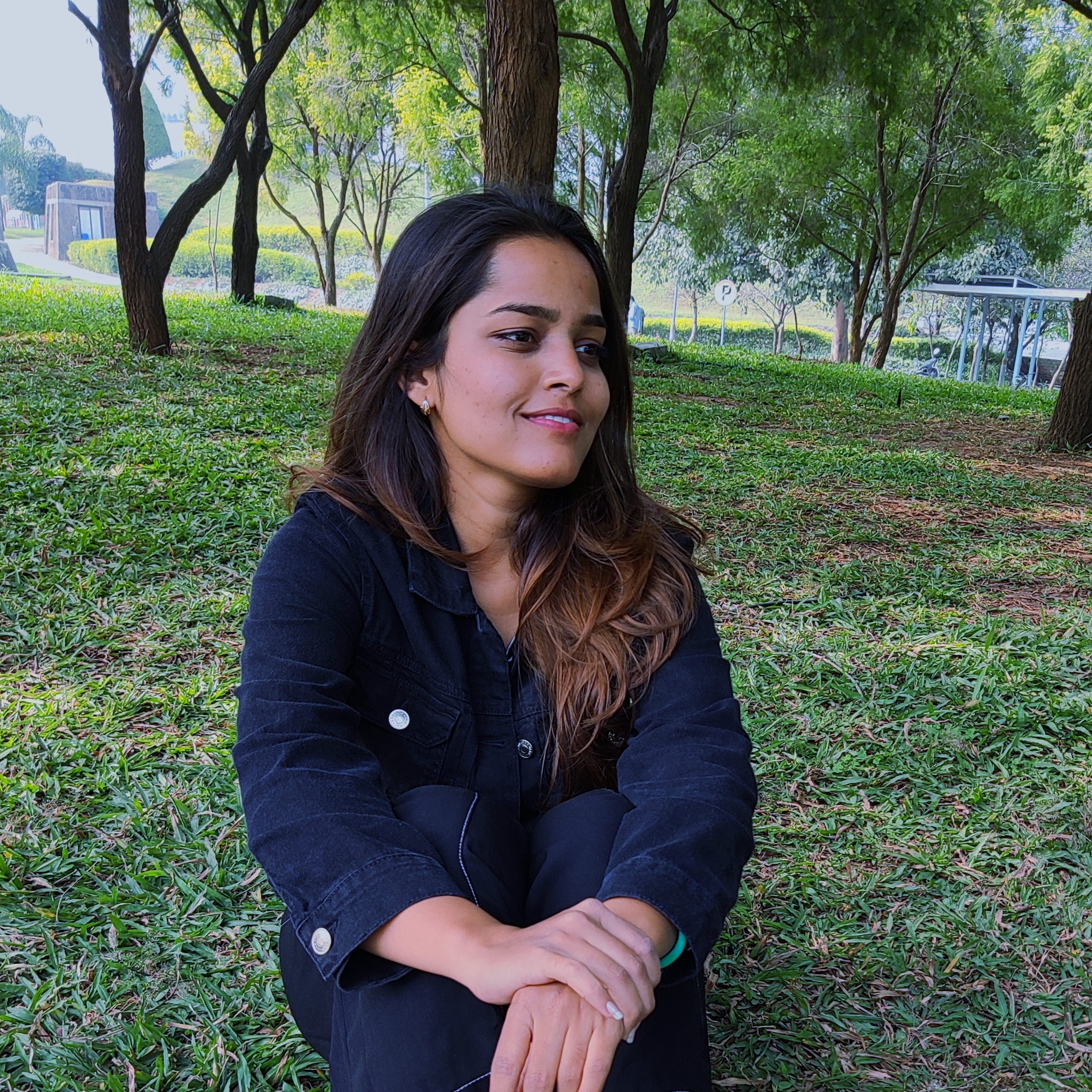
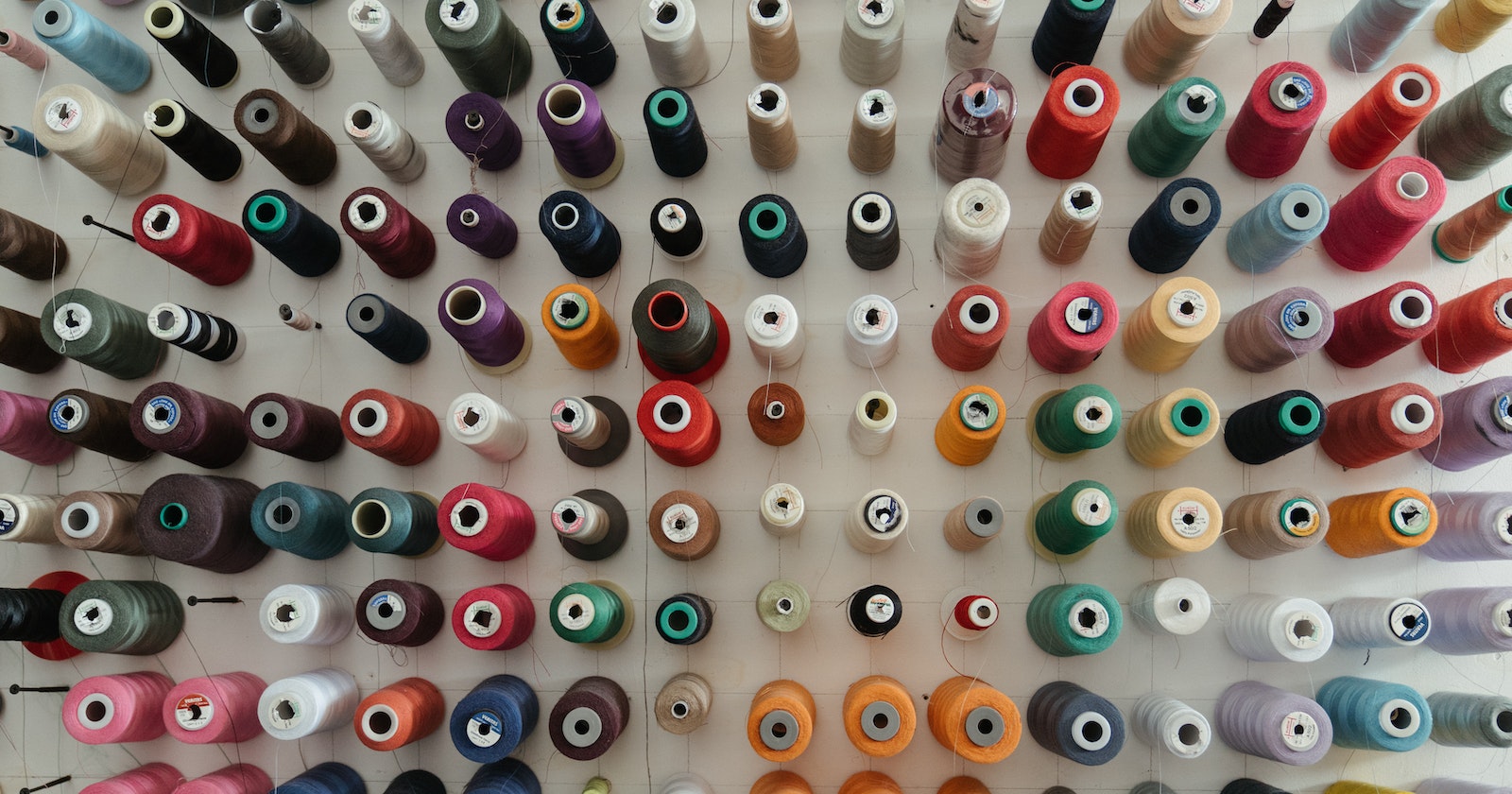
Sorting algorithms are a set of procedures that are used to organize a collection of items into a specific order. This process is important in computer science, as sorting is a fundamental operation that is used in many different applications.
Sorting algorithms work by comparing pairs of items in the collection and swapping their positions according to a specific rule. For example, if we want to sort a list of numbers from smallest to largest, we might use an algorithm that compares pairs of adjacent numbers and swaps them if they are in the wrong order. We repeat this process until the entire list is sorted.
There are many different types of sorting algorithms, each with its own set of trade-offs in terms of speed, memory usage, and complexity. Here is the list of sorting algorithms :
Bubble Sort
Selection Sort
Insertion Sort
Merge Sort
Quick Sort
Heap Sort
Radix Sort
Bucket Sort
Counting Sort
Shell Sort
Pigeonhole Sort
Tree Sort.. and many more
In general, the choice of sorting algorithm will depend on the specific application and the size and nature of the data being sorted. We will study these algorithms and their properties to find the best algorithm for a given task.
Let's take a brief glance at some of the following sorting algorithms and their brief idea :
Bubble Sort - Bubble sort is one of the simplest sorting algorithms. It works by repeatedly swapping adjacent elements if they are in the wrong order. The algorithm passes through the list multiple times until the list is sorted.
Insertion Sort - Insertion sort works by taking one element from the unsorted list and inserting it into its correct position in the sorted list. It repeats this process until the entire list is sorted.
Selection Sort - Selection sort works by finding the smallest element in the unsorted list and swapping it with the first element in the list. The algorithm then repeats this process, finding the next smallest element and swapping it with the second element in the list.
Merge Sort - Merge sort is a divide-and-conquer algorithm that works by dividing the unsorted list into n sublists, each containing one element. It then repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining, which is the sorted list.
Quicksort - Quicksort is another divide-and-conquer algorithm that works by selecting a pivot element and partitioning the other elements into two sub-lists, according to whether they are less than or greater than the pivot. It then recursively sorts the sub-lists.
Each of these sorting algorithms has its own advantages and disadvantages. For example, bubble sort is easy to understand and implement but is not very efficient for large datasets. Merge sort is efficient but requires additional memory to store the sublists. Quick sort is fast but can have poor worst-case performance.
To see the working of each algorithm, there are several great sorting algorithm visualizers available online. Here are some of the best ones:
When choosing a sorting algorithm, it is important to consider the size of the data set, the type of data being sorted, and the required performance characteristics. Some sorting algorithms may be more suited to specific tasks than others. Well, we have a good deal of algorithms suitable for almost every need.
However, there are a few sorting algorithms that are widely used :
Quicksort: Quicksort is often the first choice for sorting algorithms in computer science. It is generally considered to be very efficient and has an average-case time complexity of O(n log n).
Merge sort: Merge sort is another commonly used sorting algorithm, especially when stable sorting is required. It has a time complexity of O(n log n) in all cases.
Heap sort: Heap sort is a comparison-based sorting algorithm that has a time complexity of O(n log n) in all cases. It is often used in computer science applications where sorting speed is a priority.
Insertion sort: Insertion sort is a simple and easy-to-understand sorting algorithm that is often used for small data sets. It has a time complexity of O(n^2), but its advantage is that it requires very little additional memory.
Radix sort: Radix sort is a non-comparison based sorting algorithm that is often used for sorting large data sets with specific properties. It has a time complexity of O(nk), where k is the number of digits in the longest number in the data set.
To sum up, sorting algorithms are a fundamental part of computer science and are used in many different applications. There are many different types of sorting algorithms, each with its own strengths and weaknesses.
Implementation of all the above algorithms can be found here.
Subscribe to my newsletter
Read articles from Mrunalini Dudhagawali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
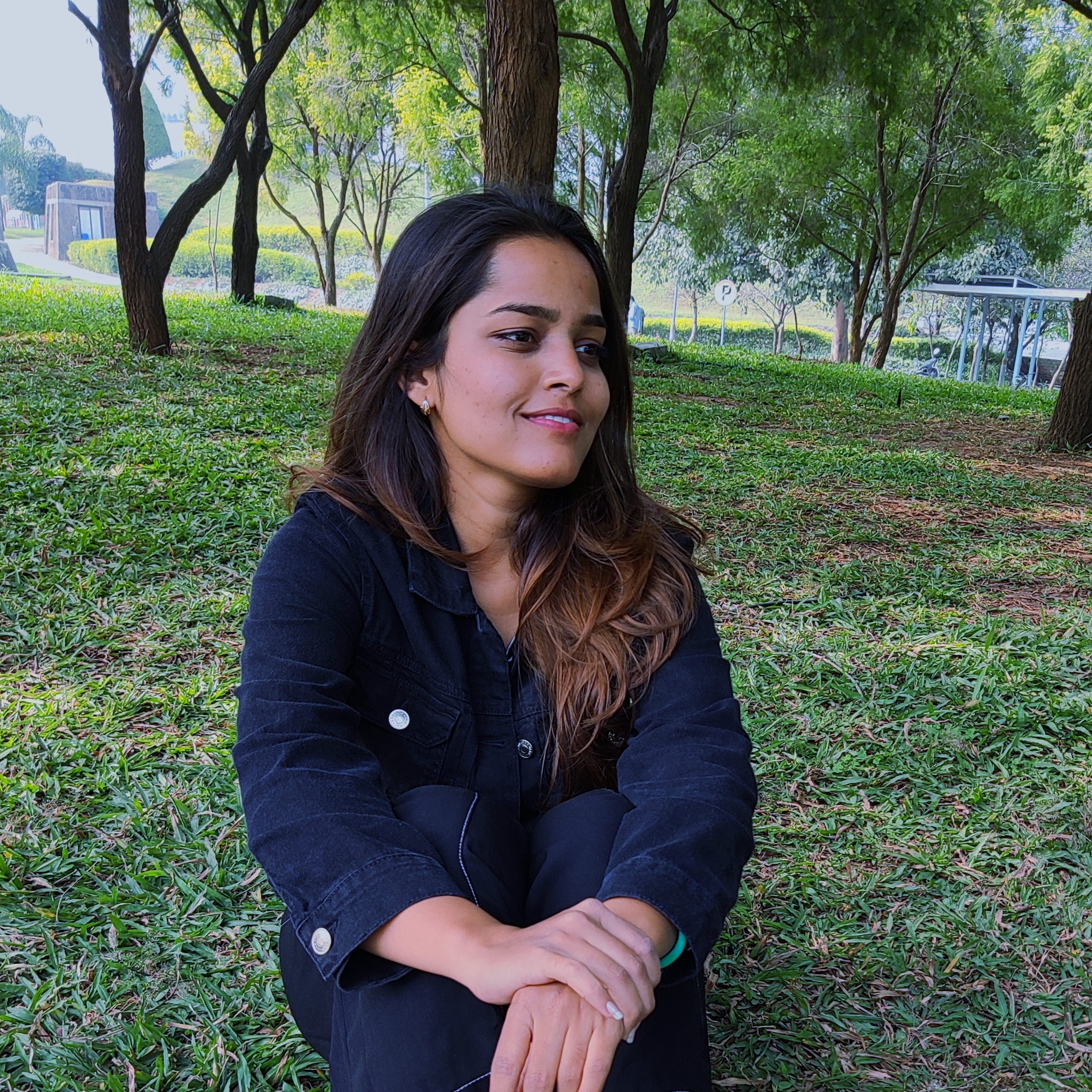
Mrunalini Dudhagawali
Mrunalini Dudhagawali
I am a developer with an eye for design, I approach every project as a chance to create something beautiful and functional. In my free time, I indulge my passions for art, travel, food, and music. Whether I'm making a sketch, local cuisine or just being a wanderlust, I'm always seeking out new experiences and inspiration. I bring this same sense of curiosity and creativity to my coding, always striving to find elegant solutions to complex problems.