Loops in JavaScript and TypeScript - Simple & Code Examples

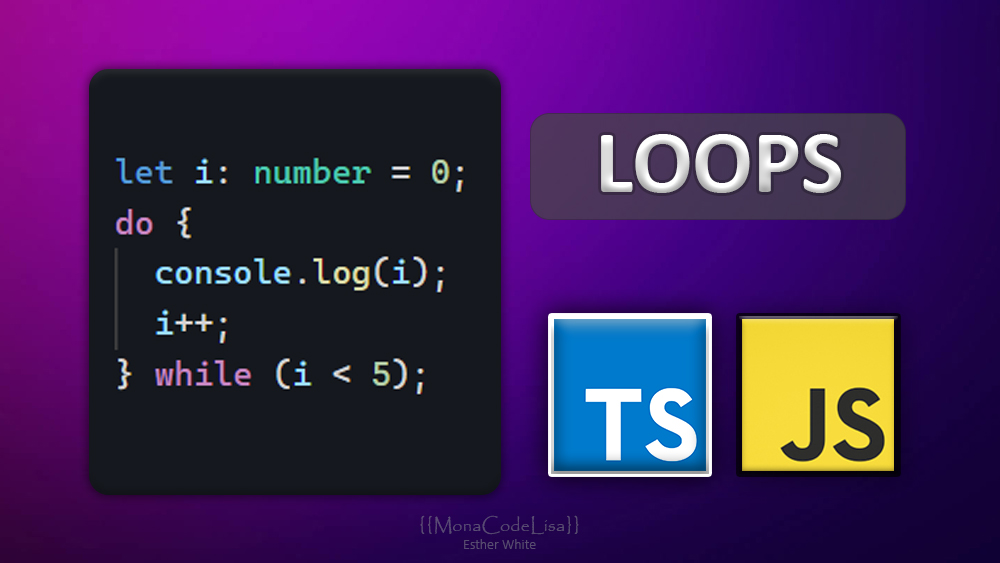
Loops are a fundamental concept in programming that allow developers to execute a block of code repeatedly. JavaScript and TypeScript provide several types of loops that can be used to achieve this functionality. In this article, we will explore the available loop types in both languages, their differences, and provide code examples of their usage.
What are Loops and What are They Commonly Used for?
A loop is a control structure that repeats a specific set of instructions until a certain condition is met. They are commonly used to iterate over collections of data, such as arrays or objects, and execute a block of code for each item in the collection. Loops are also used to perform repetitive tasks, such as printing numbers or generating patterns.
The Available Loop Types in JavaScript and TypeScript
JavaScript and TypeScript provide several types of loops that developers can use to achieve their desired functionality. These include:
For Loop
The for loop is a commonly used loop in both JavaScript and TypeScript. It allows developers to iterate over a range of values and execute a block of code for each iteration. The syntax for a for loop is as follows:
for (initialization; condition; increment) {
// code to be executed
}
Here's an example of using a for loop in JavaScript to print the numbers from 0 to 4:
for (let i = 0; i < 5; i++) {
console.log(i);
}
And here's an example of using a for loop in TypeScript to print the numbers from 0 to 4:
for (let i: number = 0; i < 5; i++) {
console.log(i);
}
While Loop
The while loop is another commonly used loop in both JavaScript and TypeScript. It allows developers to execute a block of code as long as a certain condition is true. The syntax for a while loop is as follows:
while (condition) {
// code to be executed
}
Here's an example of using a while loop in JavaScript to print the numbers from 0 to 4:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
And here's an example of using a while loop in TypeScript to print the numbers from 0 to 4:
let i: number = 0;
while (i < 5) {
console.log(i);
i++;
}
Do-while Loop
The do-while loop is similar to the while loop, but it executes the block of code at least once before checking the condition. The syntax for a do-while loop is as follows:
do {
// code to be executed
} while (condition);
Here's an example of using a do-while loop in JavaScript to print the numbers from 0 to 4:
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
And here's an example of using a do-while loop in TypeScript to print the numbers from 0 to 4:
let i: number = 0;
do {
console.log(i);
i++;
} while (i < 5);
For... in Loop
The for...in loop is used to iterate over the properties of an object. It is not recommended to use this loop with arrays, as it can cause unexpected behavior. The syntax for a for...in loop is as follows:
const person = {
name: 'Esther',
age: 30,
occupation: 'Developer'
};
for (let property in person) {
console.log(`${property}: ${person[property]}`);
}
And here's an example of using a for...in loop in TypeScript to iterate over the properties of an object:
interface Person {
name: string;
age: number;
occupation: string;
}
const person: Person = {
name: 'Esther',
age: 30,
occupation: 'Developer'
};
for (let property in person) {
console.log(`${property}: ${person[property]}`);
}
For... of Loop
The for...of loop is used to iterate over the values of an iterable object, such as an array or a string. It is not recommended to use this loop with objects, as it can cause unexpected behavior. The syntax for a for...of loop is as follows:
for (let value of iterable) {
// code to be executed
}
Here's an example of using a for...of loop in JavaScript to iterate over the values of an array:
const numbers = [0, 1, 2, 3, 4];
for (let number of numbers) {
console.log(number);
}
And here's an example of using a for...of loop in TypeScript to iterate over the values of an array:
const numbers: number[] = [0, 1, 2, 3, 4];
for (let number of numbers) {
console.log(number);
}
Conclusion
Loops are an essential concept in programming that allow developers to execute a block of code repeatedly. JavaScript and TypeScript provide several types of loops that can be used to achieve this functionality, including the for loop, while loop, do-while loop, for...in loop, and for...of loop. Each loop type has its own syntax and use cases, and developers should choose the appropriate loop type based on their specific needs. We hope this guide has helped you better understand the available loop types in JavaScript and TypeScript and how to use them in your own code.
Subscribe to my newsletter
Read articles from Esther White | EstherSoftwareDev directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Esther White | EstherSoftwareDev
Esther White | EstherSoftwareDev
Hello, I'm Esther White, I am an experienced FullStack Web Developer with a focus on Angular & NodeJS.