Resolve Conflicts with Git Rebase: A Step-By-Step Guide

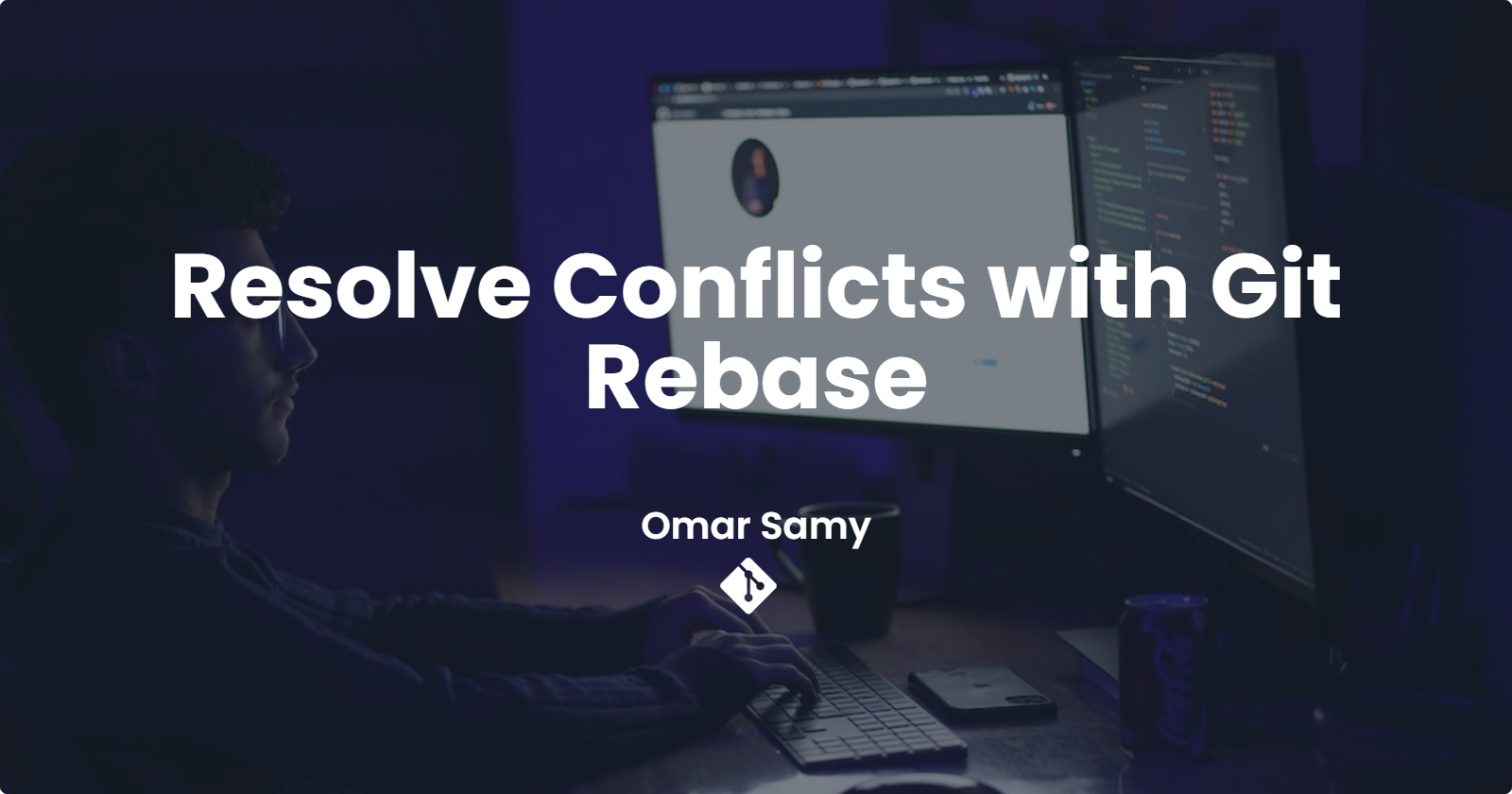
One of the most useful features of Git is Git rebase, which allows you to combine multiple branches into a single, linear history. In this post, we'll take a closer look at Git rebase, including how it works and how to use it in your projects.
What is Git Rebase?
Git rebase is a feature that allows you to take a set of commits from one branch and apply them to another branch. When you use Git rebase, you essentially "replay" the changes made in one branch onto another branch. This is useful when you want to merge changes from one branch into another without creating a merge commit.
When you use Git rebase, Git applies changes made in one branch to another branch as if they were made directly in the target branch. This creates a linear history and makes it easier to keep track of changes and resolve conflicts.
How to Use Git Rebase:
Create a new branch: The first step in using Git rebase is to create a new branch for the changes you want to make. This branch should be based on the branch you want to make changes to. In this example, we'll create a new branch called "feature-branch" based on the "master" branch.
$ git checkout master $ git checkout -b feature-branch
Make changes: Once you've created your new branch, make the changes you want to make. In this example, we'll create a simple "hello world" application and add it to our new branch.
$ touch hello.txt $ echo "Hello, World!" > hello.txt $ git add hello.txt $ git commit -m "Added hello.txt"
Switch to target branch: Once you've made your changes, switch back to the target branch you want to apply your changes to. In this example, we'll switch back to the "master" branch.
$ git checkout master
Use git rebase: Use the git rebase command to apply the changes made in your feature branch to the target branch.
$ git rebase feature-branch
Resolve conflicts: When you use git rebase, conflicts may arise if changes made in one branch conflict with changes made in another branch. Git will prompt you to resolve these conflicts manually. You can use "git status" to see which files have conflicts and resolve them using a text editor.
Push changes: Once you've resolved any conflicts, push your changes to the target branch.
$ git push origin master
Demonstration with a Simple "Hello World" App:
Create a new repository on GitHub and clone it to your local machine.
$ git clone https://github.com/<your-username>/hello-world.git
Create a new branch based on the master branch.
$ git checkout master $ git checkout -b feature-branch
Create a new file called "hello.txt" and add some text to it.
$ touch hello.txt $ echo "Hello, World!" > hello.txt $ git add hello.txt $ git commit -m "Added hello.txt"
Switch back to the master branch and make a change.
$ git checkout master $ echo "This is a new line." >> hello
Commit the change to the master branch.
$ git add hello.txt $ git commit -m "Added a new line to hello.txt"
Switch back to the feature branch and use git rebase to apply the changes made in the master branch.
$ git checkout feature-branch $ git rebase master
Resolve any conflicts that arise.
CONFLICT (content): Merge conflict in hello.txt Automatic merge failed; fix conflicts and then commit the result.
In this example, we have a merge conflict in the "hello.txt" file. We can use a text editor to resolve the conflict and then commit the changes.
$ vim hello.txt $ git add hello.txt $ git rebase --continue
Push the changes to the feature branch.
$ git push origin feature-branch
Merge the feature branch into the master branch.
$ git checkout master $ git merge feature-branch
Push the changes to the master branch.
$ git push origin master
Conclusion:
Git rebase is a powerful feature that allows you to integrate changes from one branch into another branch in a more streamlined way than a traditional merge. By using Git rebase, you can keep your code history more linear and easier to understand. Keep in mind that conflicts can arise when using Git rebase, but these can be resolved with a bit of manual intervention. Hopefully, this post has given you a better understanding of how Git rebase works and how you can use it in your projects.
Subscribe to my newsletter
Read articles from Omar Samy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Omar Samy
Omar Samy
Hey! I'm Omar Samy, a DevOps Engineer who is super enthused about technology. I enjoy experimenting with different technologies and using my knowledge to help organizations run their activities more efficiently, streamline their processes, and reach their objectives effectively.