Errors errors errors - A blog about errors
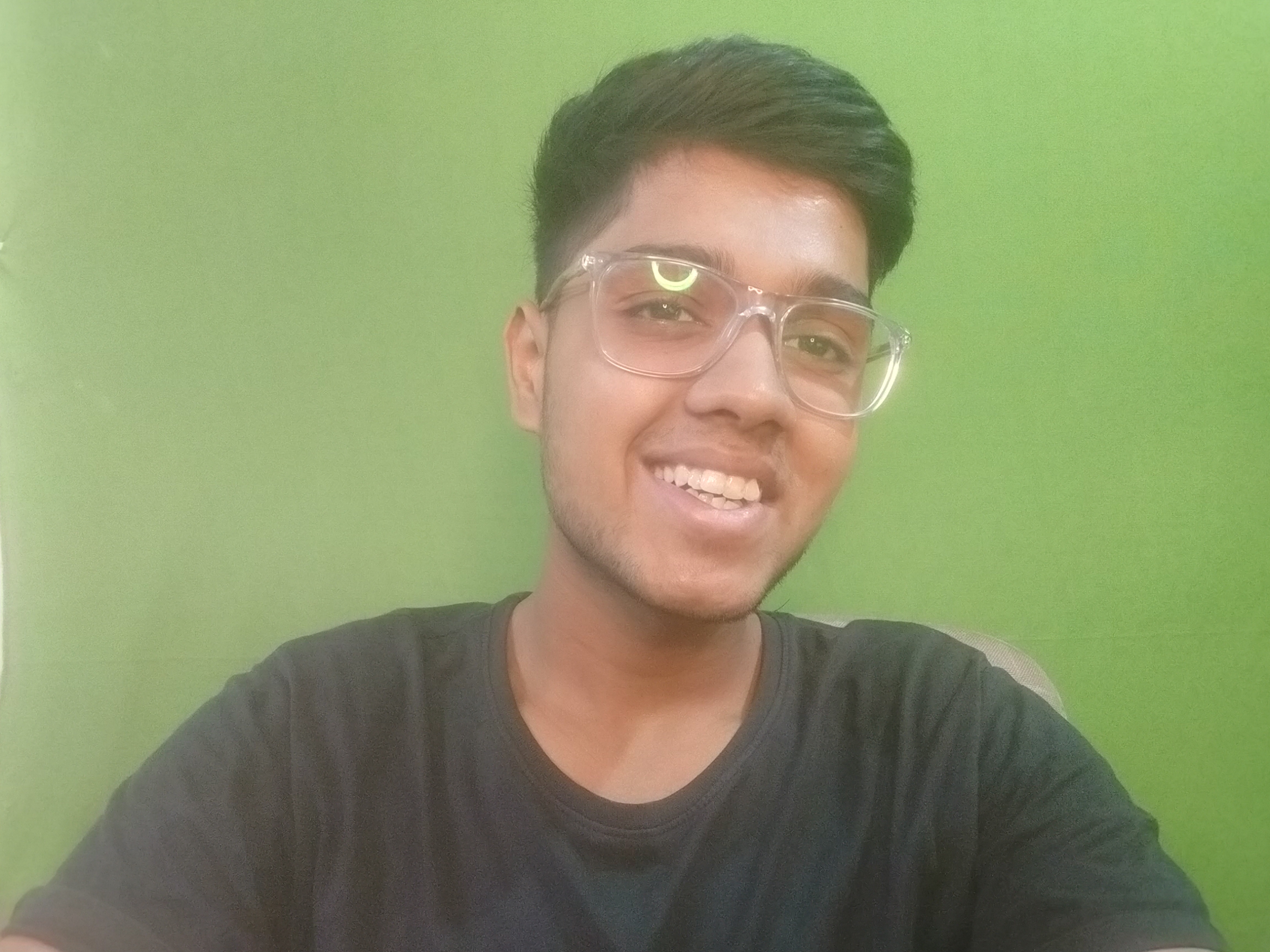
Errors... errors... errors! I don't like it. I avoid, But, error likes me! I can't avoid.
As web devs, We all have had this emotion. Solving one error leads to another and solving that leads to another.
Searching on google, Stackoverflow, Reddit even Yahoo!! just kidding no one uses yahoo.
So, What are the common errors we face -
ReferenceError
TypeError
SyntaxError
Let's start with ReferenceError -
A ReferenceError occurs when you try to use a variable that doesn't exist at all.
const x = 10
console.log(y)
// ReferenceError: y is not defined
What if we assign the variable after calling it -
const x = 10
console.log(y)
const y = 20
//ReferenceError: Cannot access 'y' before initialization
We can't call a variable before declaring it because there is no reference to that variable.
How to fix ReferenceErrors -
You declare the variable before accessing it -
const x = 10
const y = 20
console.log(y)
//20
What is TypeError -
Before I give you the definition look at an example -
const x = 10
console.log(x)
x = 11
console.log(x)
//10
//TypeError: Assignment to constant variable
You get a type error here because you are trying to reassign the value of a constant variable.
You get a TypeError when you try to modify a value that cannot be changed or use a value in an inappropriate way.
How to fix TypeError-
You fix type errors by casting your values to the correct type -
//When you know you will have to reassign a variable then assign it with "let"
let x = 10
console.log(x) //10
x=11
console.log(x)//11
Syntax Errors-
Syntax errors are caused by incorrect use of predefined syntax.
let me show you with an example -
function lululemon(huhu){
if ("lulul" === huhu){
console.log(huhu)
}
lululemon(lulul)
//SyntaxError: Unexpected end of input
Why do you think we had a syntax error? Look closely there is a missing closing bracket for the if statement.
How to fix SyntaxErrors-
function lululemon(huhu){
if ("lulul"===huhu){
console.log(huhu)
}
}
lululemon("lulul")
//lulul
You put the closing bracket in its place and voila no errors!!!
Thanks for reading.
bye
Subscribe to my newsletter
Read articles from anand directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
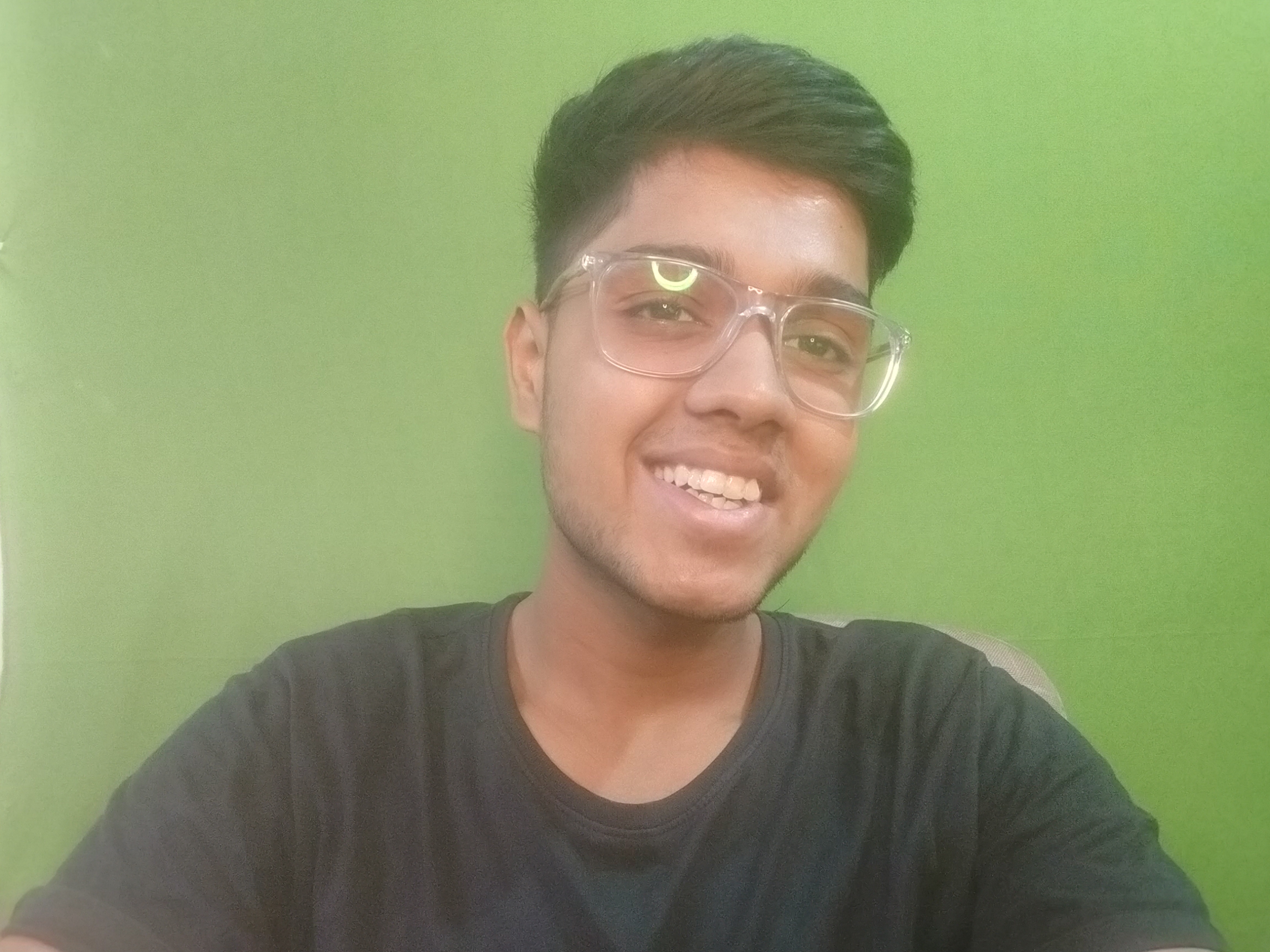