Nullish coalescing (??) & Optional chaining (?.) in JS
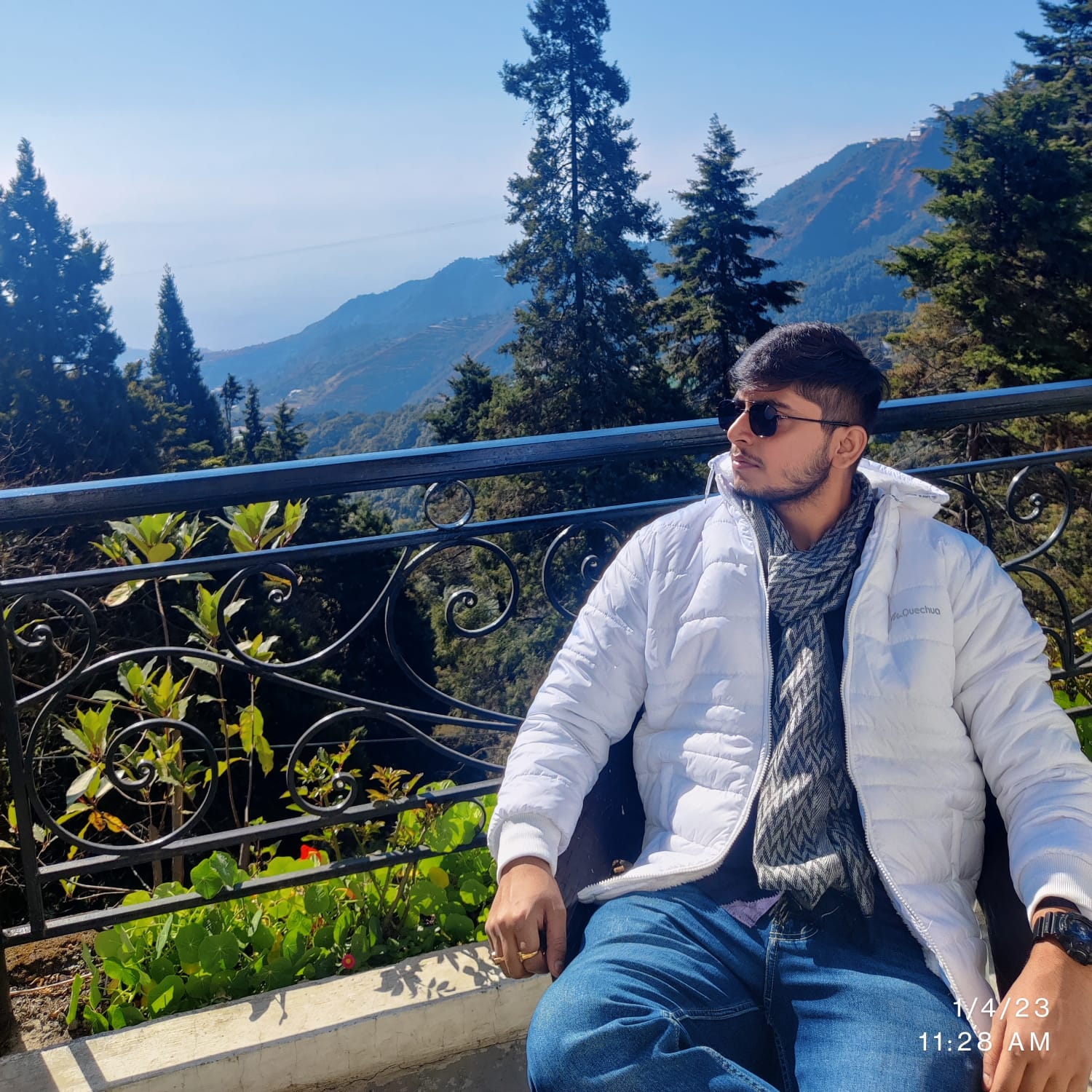
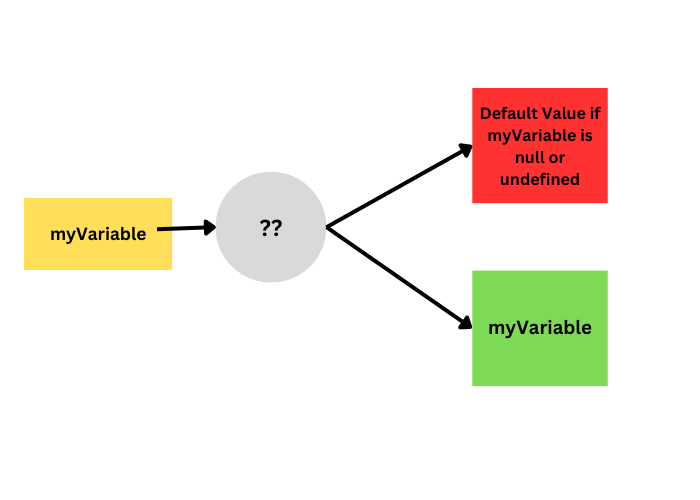
Introduction
While writing JavaScript code we come across multiple errors like
TypeError: Cannot read property
TypeError: ‘undefined’ is not an object
TypeError: null is not an object
and these errors were caused when we try to reference a variable or object which is undefined or null.
To avoid these errors we need to explicitly check every reference if it is defined or not.
Lets us understand this with an example
const person1 ={
name:"Aman",
address:{
city:"Ranchi",
pincode:232421
}
}
const person2 = {
name:"Amar",
address:undefined,
}
//Suppose we want to get the value of city of the person object
const getCity = data =>{
if(data !== undefined && data.address !== undefined && data.address.city !== undefined){
return data.address.city;
}
}
In the above example, you can see that we have put multiple conditions in the getCity
function.
If we don't use the above conditions we will get Uncaught TypeError: Cannot read properties of undefined (reading 'city')
error when we pass a person2
.
The condition keeps on increasing with highly nested objects, keeping this problem in mind and to make the code more readable and clean two operators were introduced
Nullish coalescing operator (??)
Optional chaining (?.)
Let's understand these two operators in detail
Nullish coalescing operator (??)
Definition MDN
The nullish coalescing (??) operator is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand.
Syntax
leftExpr ?? rightExpr
Lets understand this with code
let myVariable = undefined;
let defaultValue = "default";
console.log(myVariable??defaultValue); //default
myVariable = "Aman";
console.log(myVariable??defaultValue); //Aman
In the above code as myVariable
is undefined
so when we use the ??
operator gives us the default value instead of logging undefined.
Note:
Combine both the AND (
&&
) and OR operators (||
) directly with??
will give a syntax error.For example :
null || undefined ?? "aman"; // raises a SyntaxError true && undefined ?? "aman"; // raises a SyntaxError
Optional Chaining (?.)
Definition MDN
The optional chaining (
?.
) operator accesses an object's property or calls a function. If the object accessed or function called using this operator is[undefined]
or[null]
, the expression short circuits and evaluates to[undefined]
instead of throwing an error.
Syntax
obj.val?.prop
obj.val?.[expr]
obj.func?.(args)
So let's take the first example of this blog to understand optional chaining.
Approach before optional chaining
const person1 ={
name:"Aman",
address:{
city:"Ranchi",
pincode:232421
}
}
const person2 = {
name:"Amar",
address:undefined,
}
//Approch before Optional chaining.
//Suppose we want to get the value of city of the person object
**const getCity = data =>{
if(data !== undefined && data.address !== undefined && data.address.city !== undefined){
return data.address.city;
}
}**
console.log(getCity(person1));//Ranchi
console.log(getCity(person2));//Undefined
Approach after optional chaining
const person1 ={
name:"Aman",
address:{
city:"Ranchi",
pincode:232421
}
}
const person2 = {
name:"Amar",
address:undefined,
}
//Approch after Optional chaining.
//Suppose we want to get the value of city of the person object
**const getCity = data => data?.address?.city;**
console.log(getCity(person1));//Ranchi
console.log(getCity(person2));//Undefined
The optional chaining approach instead of explicitly checking for each reference in the provided object we can simply use ?.
the operator implicitly checks it for us and gives undefined
instead of throwing a type error. This makes the code clean and increases the code readability.
Note
Optional chaining cannot be used on undeclared object or variable.
undeclaredVar?.prop; // ReferenceError: undeclaredVar is not defined
Conclusion
Using Nullish coalescing & optional chaining helps to make the JavaScript code base clean and readable.
Thanks for reading❤
Subscribe to my newsletter
Read articles from Aman kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
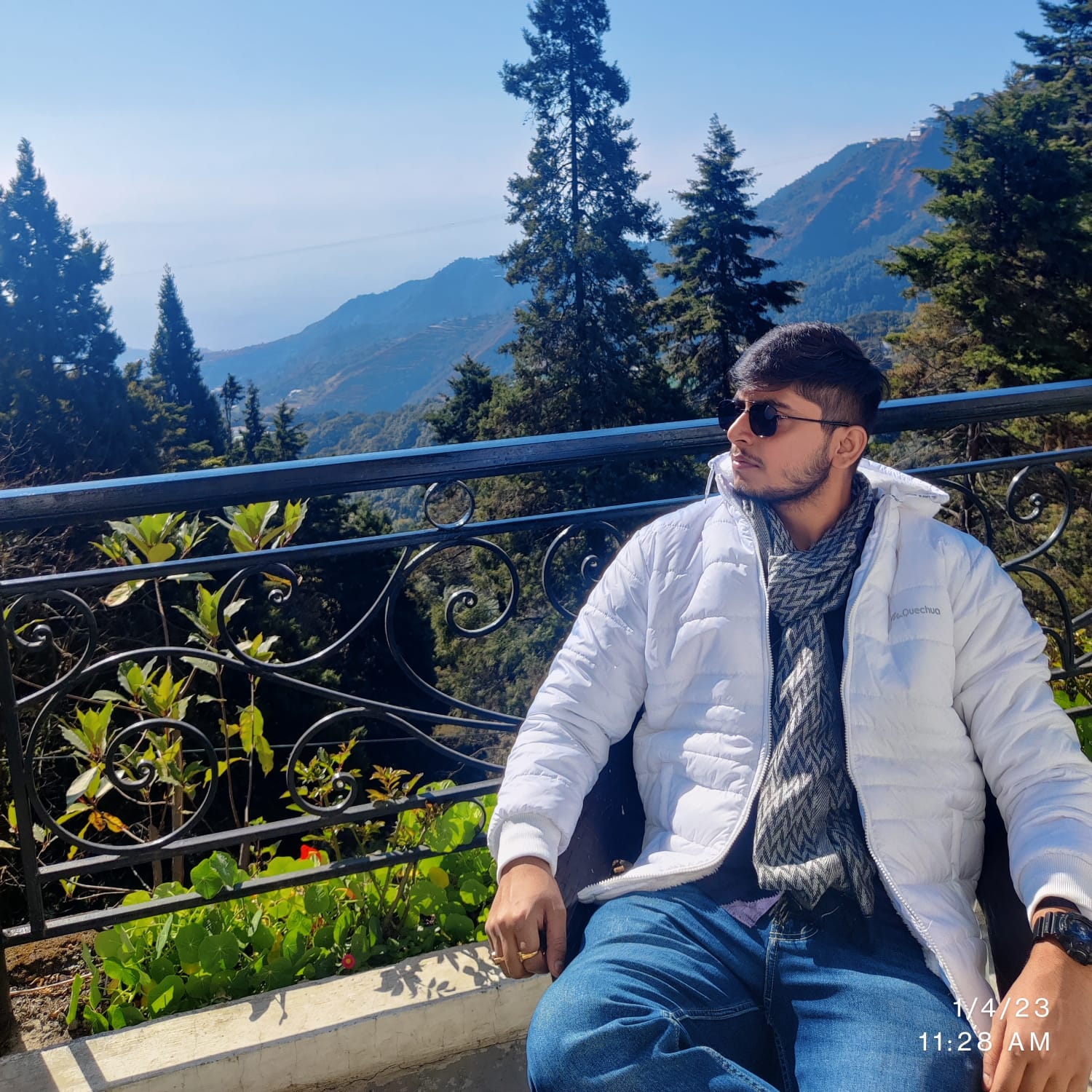
Aman kumar
Aman kumar
Software Developer @Accenture | NeoG Undergrad | Frontend developer