Can we use jsx without react?
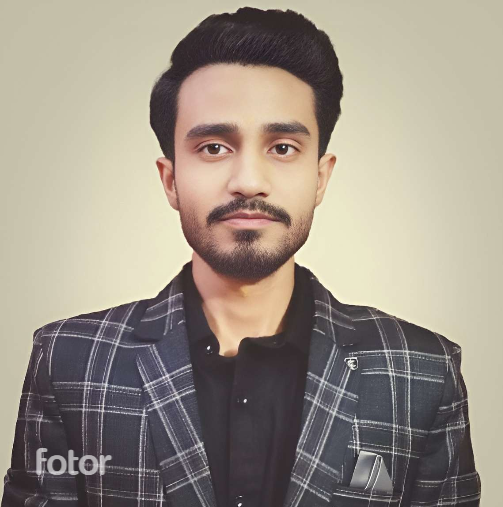
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. It is commonly used with React, but it is not limited to React and can be used with other libraries or frameworks.
Here's an example of how you can use JSX without React:
const element = <h1>Hello, world!</h1>;
document.body.appendChild(element);
In this example, we're creating a new JSX element using the <h1>
tag and the Hello, world!
text. We're then appending the element to the body
of the HTML document.
However, in order to use JSX without React, you need to compile the JSX code into regular JavaScript code. You can use tools like Babel or TypeScript to do this.
Here's an example of how to set up Babel to compile JSX code:
Install Babel and the necessary plugins:
npm install --save-dev @babel/core @babel/cli @babel/preset-env @babel/preset-react
Create a
.babelrc
file in your project's root directory with the following content:{ "presets": [ "@babel/preset-env", "@babel/preset-react" ] }
Add a build script to your
package.json
file:"scripts": { "build": "babel src -d dist" }
Create a
src
directory in your project's root directory, and create aindex.jsx
file with the following content:const element = <h1>Hello, world!</h1>; document.body.appendChild(element);
Run the build script:
npm run build
This will compile your
index.jsx
file into a regular JavaScript file, which you can then include in your HTML document:<!DOCTYPE html> <html> <head> <meta charset="UTF-8" /> <title>JSX without React</title> </head> <body> <script src="dist/index.js"></script> </body> </html>
Note:- This is a simple example, and using JSX without React may not be practical for more complex applications.
Hope I was able to clear my point and if you have any doubt(s) or suggestion(s) then you may comment me, I will try to reply as soon as possible. Thanks for reading...
Subscribe to my newsletter
Read articles from Jayesh Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
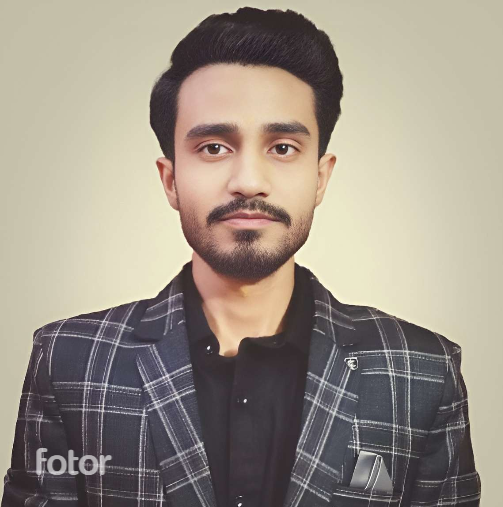
Jayesh Chauhan
Jayesh Chauhan
Hey I am Full Stack Developer.