Creating a Signup page from scratch!!

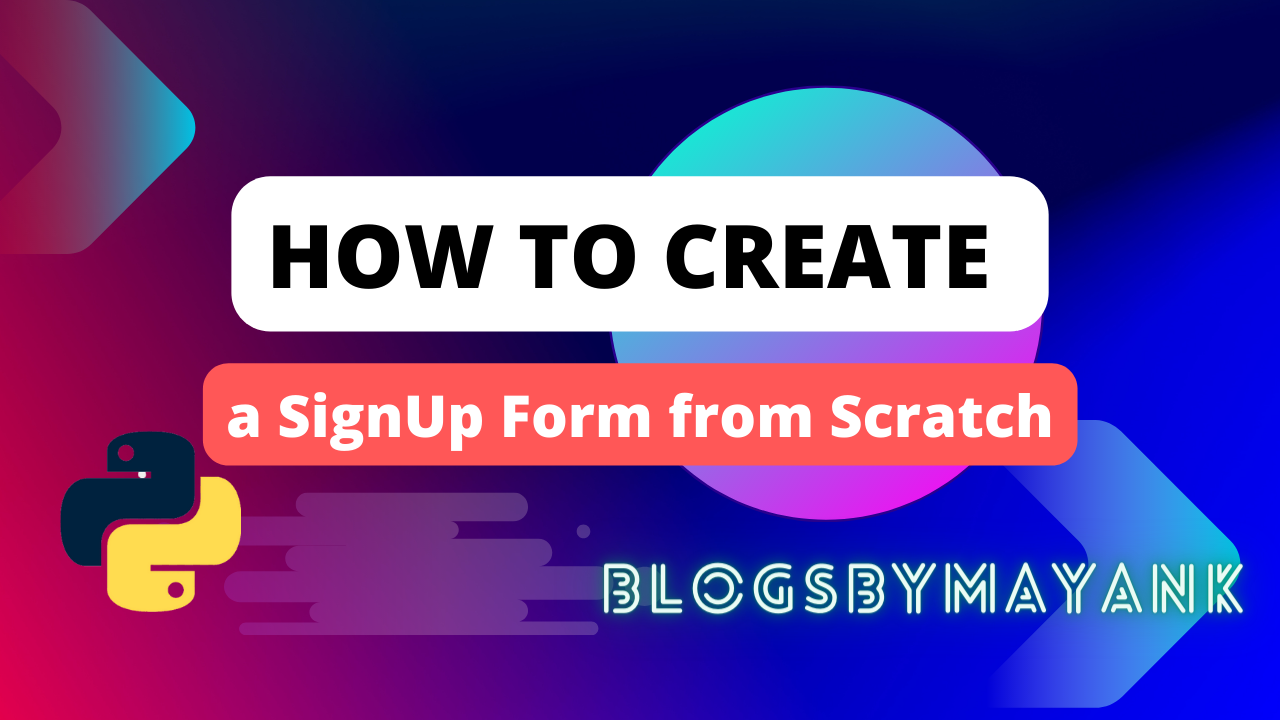
Django is a powerful and popular web framework for building web applications. In this blog, we will discuss how to create a signup page in Django from scratch, using Python code.
Step 1: Creating a Project
Setting up the project: Before we begin creating the signup page, we need to create a Django project and application. We can do this by running the following commands in the terminal:
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
Here, myproject
is the name of our project and myapp
is the name of our application. This will create the necessary files and directories for our project and application.
Step 2: Configuring Views.py
Creating the Signup view: The next step is to create the signup
view that will handle the signup request. This is done in the views.py
file in our application. Here's an example:
from django.shortcuts import render, redirect
from django.contrib.auth.forms import UserCreationForm
def signup(request):
if request.method == 'POST':
form = UserCreationForm(request.POST)
if form.is_valid():
form.save()
return redirect('home')
else:
form = UserCreationForm()
return render(request, 'signup.html', {'form': form})
Here, we import the UserCreationForm
from the django.contrib.auth.forms
module, which provides a pre-built form for user registration. We then check if the request method is POST
, which means that the user has submitted the form. If it is, we validate the form and save the user to the database, and then redirect them to the home page. If it is not, we create a new instance of the UserCreationForm
and render the signup.html
template with the form as context.
Step 3: Creating a SignUp page
Creating the Signup template: The next step is to create the signup.html
template, which will display the signup form to the user. Here's an example:
{% extends 'base.html' %}
{% block content %}
<h2>Sign up</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Sign up</button>
</form>
{% endblock %}
Here, we extend the base.html
template, which is our base template that contains the common elements of our site. We then create a form that uses the POST
method to submit the data to the server. We use the csrf_token
template tag to include the CSRF token, which is a security feature that prevents cross-site request forgery attacks. We then use the as_p
method to render the form as a series of paragraphs, and include a submit button.
Step 4: Configuring urls.py
Creating the URL pattern: Finally, we need to create the URL pattern that will point to the signup
view. This is done in the urls.py
file in our application. Here's an example:
from django.urls import path
from . import views
urlpatterns = [
path('signup/', views.signup, name='signup'),
]
Here, we define a URL pattern that will map to the signup
view. This URL will be /signup/
.
Conclusion
With these steps, we have created a signup page in Django from scratch, using Python code. This page allows users to register for our web application and adds them to our user database. This can be expanded upon with additional features, such as email verification, password strength validation, and more.
Subscribe to my newsletter
Read articles from Mayank Aggarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayank Aggarwal
Mayank Aggarwal
Hello! I'm Mayank Aggarwal a B.Tech Student. As a dedicated tech enthusiast and blogger, I'm excited to welcome you to my corner of the internet. I am A backend Developer. I am Working on My DSA Skills. Now preparing for Interviews.