SHORTEST JS Program 🔥window & this keyword
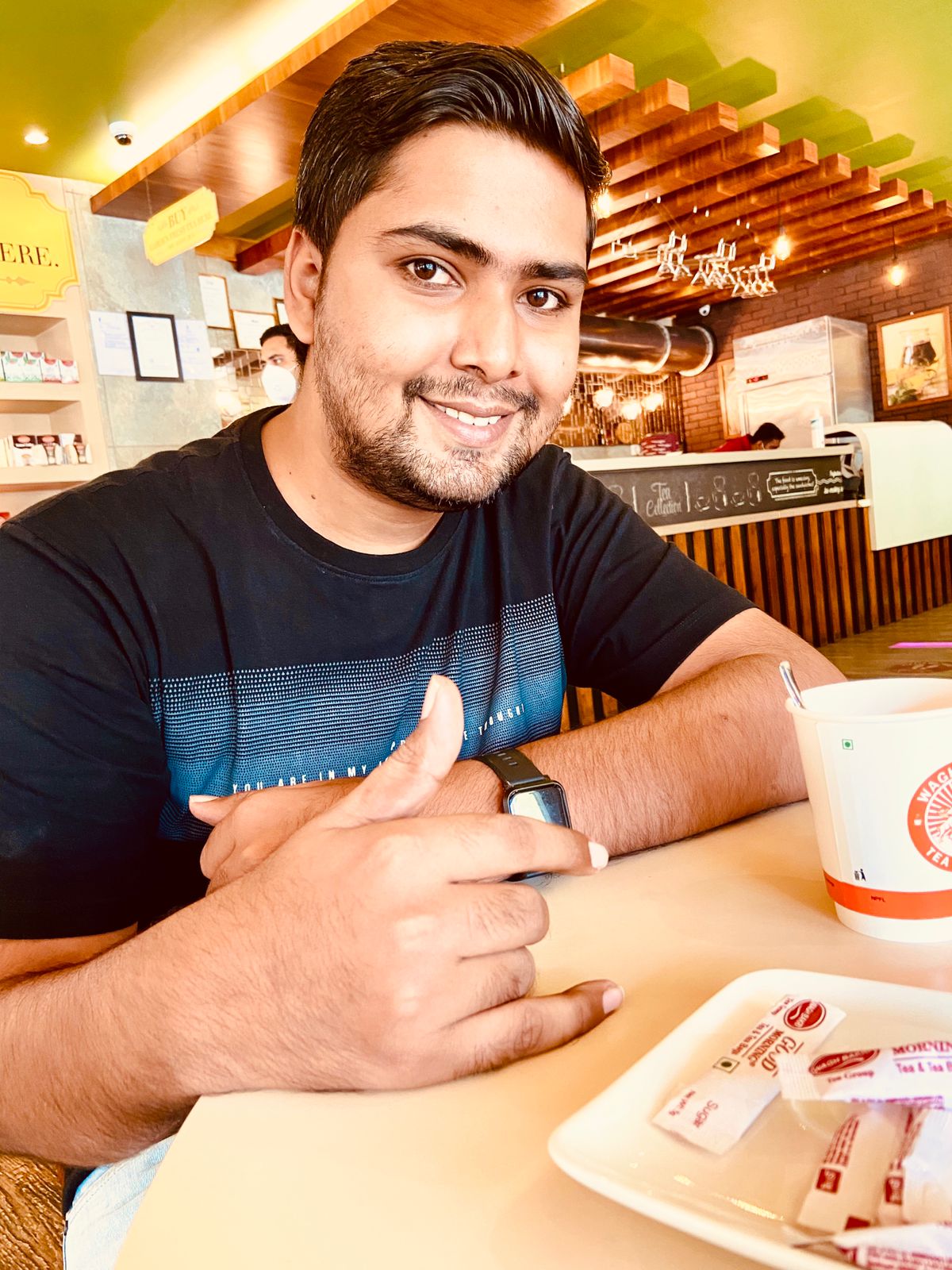
Table of contents
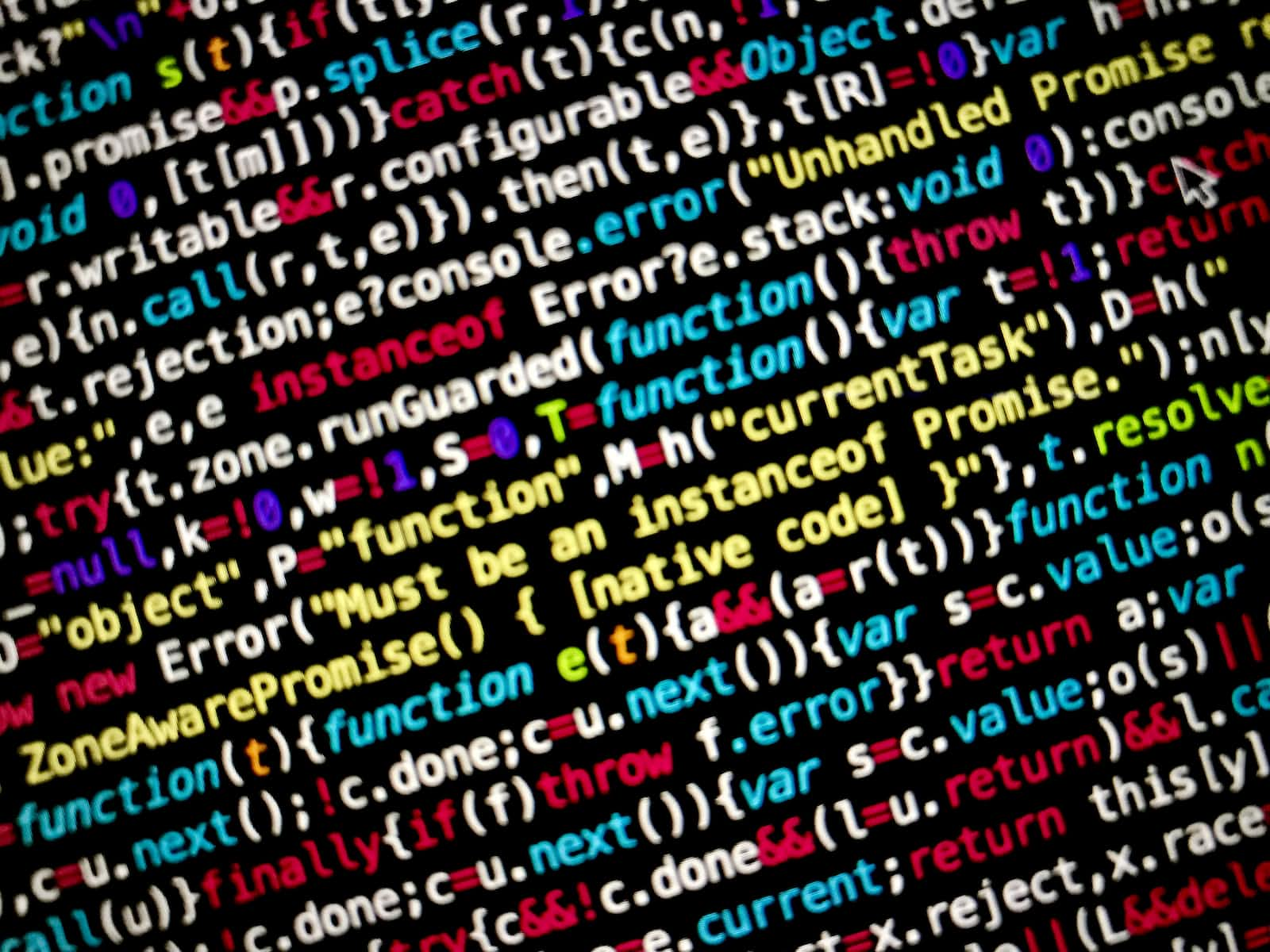
In this blog, we will explore the window object in JavaScript and its connection to the "this" keyword.
To understand this connection, we will write the shortest possible program in JavaScript and see how it behaves.
Here is the shortest program we can write
// index.js
// No code in this file
As soon as we run any JavaScript program, a global execution context is created, which we have discussed in our previous blog post. Along with the global execution context, JavaScript also creates a window object, which we can use anywhere in our program.
The window object contains many functions and variables that we can use, although some are used internally by JavaScript and we don't need to worry about them.
Now let's look at an example of the window object:
// index.js
var x = "DO BEST";
var y = "DO BETTER";
function doSomething() {
var z = "Do something";
}
In this example, we have defined two variables, x
and y
, and declared one function, doSomething()
. These are in the global environment. Variables and functions defined outside a function are in the global environment, whereas those declared inside a function are in the local environment. So, in this example, we can say that x
, y
, and doSomething()
are in the global environment, whereas z
is in the local environment, as it is declared inside the doSomething()
function.
As soon as we run this JavaScript program, the window object will store all the variables and functions that are in the global environment. Here is what the window object will look like at this moment:
// WINDOW OBJECT
{
x: "DO BEST",
y: "DO BETTER",
doSomething: function doSomething() {
// Entire code of this function
},
// Default properties of the window object
}
Now we can access the variables in three different ways:
The first way is to access variables and functions directly with their names. In this case, JavaScript assumes that you are trying to access something at the global level and attach the window object to it. Internally, it is like console.log(window.x)
.
console.log(x); // Internally for JavaScript, this code will be window.x
console.log(y); // Internally for JavaScript, this code will be window.y
doSomething(); // Internally for JavaScript, this code will be window.doSomething()
The second way is to manually add the window object. We usually don't need to do this, but we can write the code like this:
console.log(window.x); // You are just saving JavaScript time :)
console.log(window.y);
window.doSomething();
The third way is to use the "this" keyword to access any variables and functions in the global space. This is because, at the global level, the window object is equal to "this". The "this" keyword itself is a big topic of discussion, and we will have another blog post specifically on this keyword. But for now, we need to understand that in the global space, the window object and the "this" keyword are the same, and that is why console.log(window === this)
will return true
.
console.log(this.x);
console.log(this.y);
console.log(this.doSomething());
In summary, we have learned how JavaScript creates the window object, even with a single line of code, how global variables and functions are attached to the window object, and how, in the global space, the window.
In addition to global variables and functions, the window object also contains several properties and methods that can be useful when working with the browser. For example, you can use the window object to access the current URL, open a new browser window, or display a pop-up alert message. Some common properties and methods of the window object include:
location: Contains information about the current URL, such as the domain name, path, and query parameters.
document: Represents the current web page as an object, which you can use to manipulate the HTML and CSS.
alert(): Displays a pop-up window with a message, which is often used to alert the user of an error or other important information.
It's worth noting that the window object is only available in the browser environment, and not in other JavaScript environments such as Node.js. In these environments, the global object is different and does not have access to the browser-specific properties and methods of the window object.
That's all above the SHORTEST JS Program window and this keyword. And thanks to Akshay Saini for creating an incredible Namaste Javascript playlist this blog is just whatever learning I had from there.
Subscribe to my newsletter
Read articles from haresh lakhwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
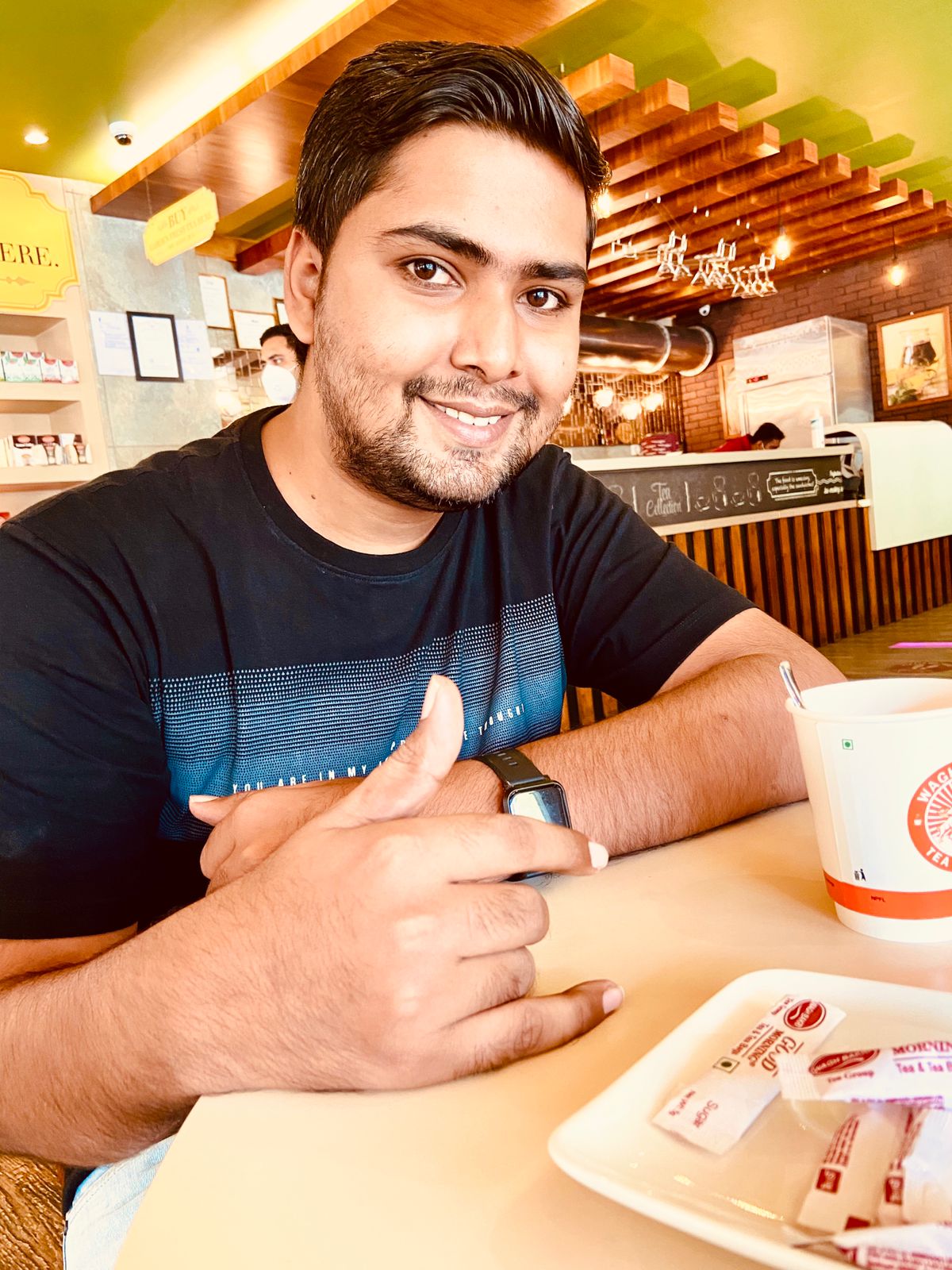
haresh lakhwani
haresh lakhwani
Experienced developer with a background in native Android development and cross-platform development with React Native. Strong collaborator with a focus on attention to detail and staying up-to-date with the latest technologies. Currently expanding knowledge in data structures and system design. Capable of delivering high-quality applications utilizing React and React Native.