APIs for Dummies: How to Speak Geek Like a Pro (Without Actually Being One)
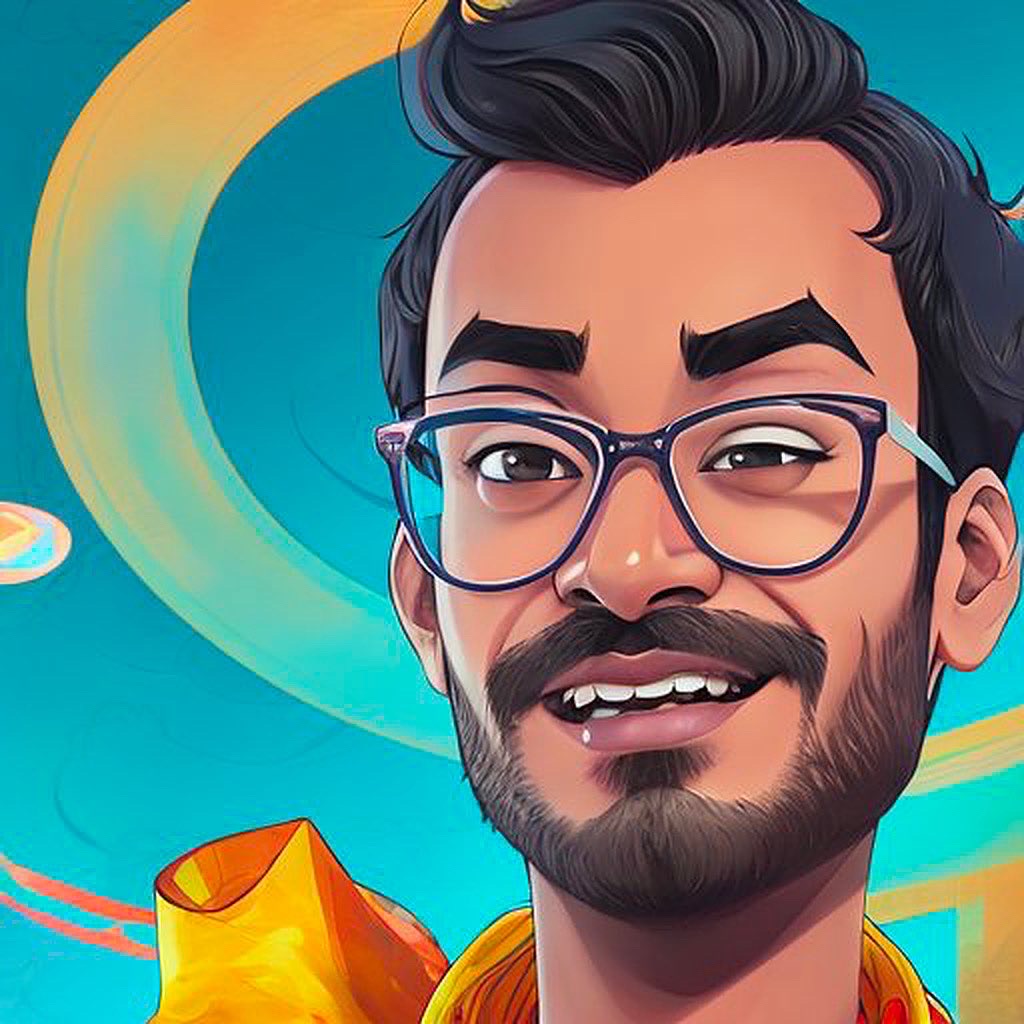
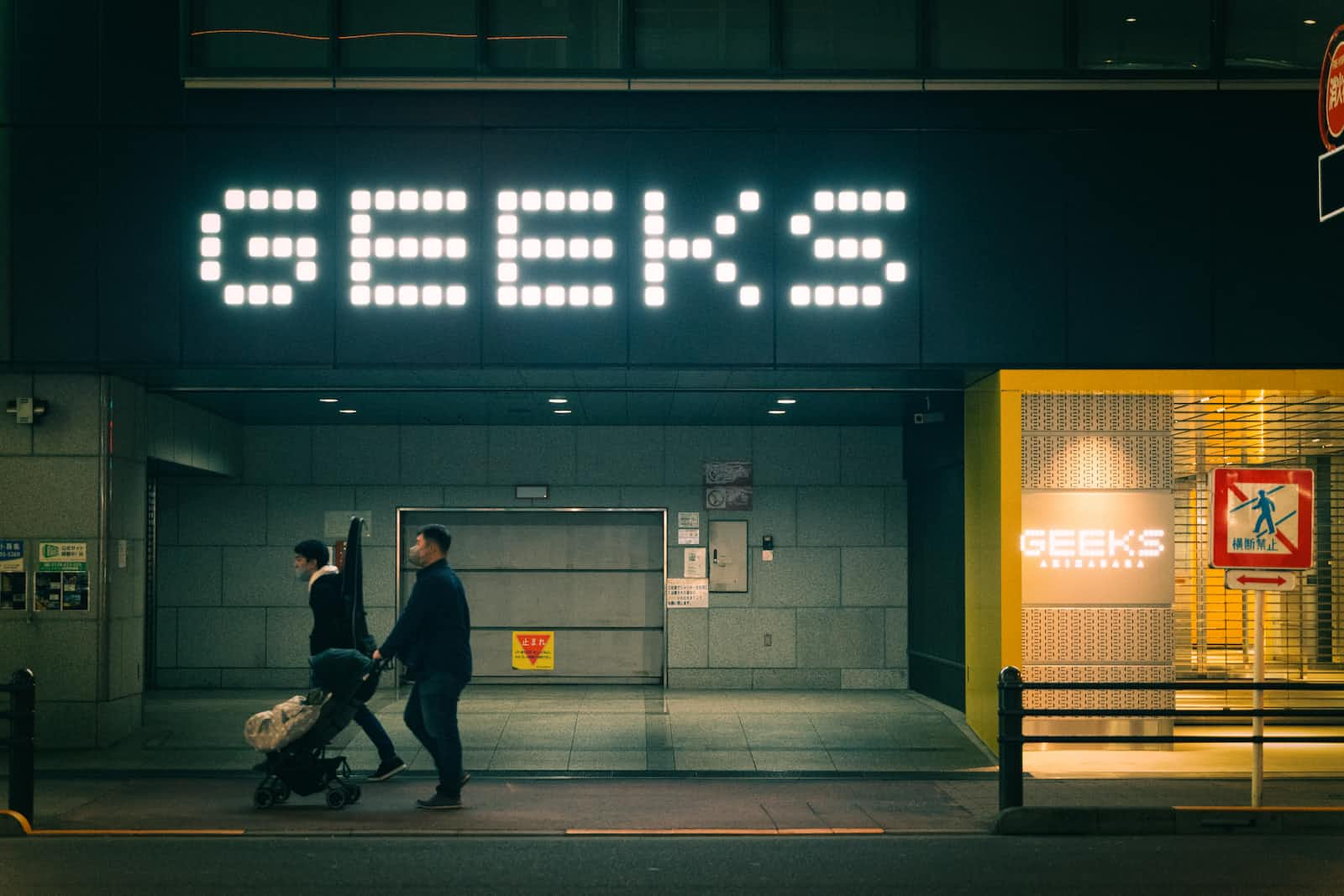
Let me tell you a story about APIs. Once upon a time, there was a group of developers who wanted to build an app that would allow users to track their fitness goals. They had a great idea for the app, but they needed to find a way to get the fitness data from different sources, such as fitness trackers and health apps, into their app. They didn't want to waste time building custom integrations for each data source, so they decided to use APIs.
An API, they learned, was like a secret door that would allow their app to communicate with other apps and services. By using APIs, they could easily access fitness data from various sources and integrate it into their app. They didn't need to worry about the technical details of how the data was being retrieved, because the API took care of all that. All they needed to do was to send a request to the API, and the data would be delivered to their app.
Using APIs not only made their app development faster and more efficient, but it also enabled them to provide a better user experience. Users could connect their preferred fitness tracker or health app to the fitness tracking app, and the data would automatically sync. They didn't need to manually enter their data, and the app could provide more accurate insights and recommendations.
In the end, the fitness tracking app became a huge success, and the developers realized that APIs were the key to building scalable and robust software. They continued to use APIs in all their projects, and they shared their knowledge with other developers, who also saw the value of APIs. And that's how APIs became a superhero for developers and product managers, enabling them to build amazing apps that make people's lives better.
An API, or Application Programming Interface, is a way for different software systems to communicate with each other. Imagine you are in a restaurant and want to order food. The menu is like an API, which tells you what dishes are available and how you can order them. You can choose a dish from the menu, and the kitchen staff will prepare it according to your order.
The Client Server Model
The client-server model using APIs is a common way for different software systems to communicate with each other. Imagine you are at a restaurant, and you want to order food. The restaurant's kitchen is like a server that provides a service (preparing and delivering food), and you are like a client that requests that service (ordering food).
Now, imagine that the restaurant has an API that allows you to place your order through a mobile app instead of speaking with a waiter. You use the app to select the items you want, and the app sends a request to the restaurant's server through the API. The server receives the request, processes it, and prepares your food. When your order is ready, the server sends a response through the API, letting the app know that your food is on the way.
The API acts as an intermediary between the client (the app) and the server (the kitchen), providing a standard way for them to communicate with each other. The client can send requests to the server using the API, specifying what data it wants or what actions it wants the server to perform. The server receives the requests, processes them, and sends responses back to the client through the API.
The client-server model is a technical architecture used in computing that consists of two main components: the client and the server. The client is typically a software application or a device that requests a service, while the server is a computer system that provides the service.
When a client wants to request a service from a server, it sends a request message to the server. This message typically contains information such as the type of service requested, any required parameters or data, and the destination server address.
Upon receiving the request message, the server processes the request and generates a response message, which contains the requested information or service. The response message is then sent back to the client, which can then process the received information or service.
To ensure that the client and server can communicate effectively and efficiently, the client-server model also includes a mechanism for acknowledging messages. After the client sends a request message, it waits for an acknowledgement from the server that the request was received and is being processed. Once the server has processed the request and generated a response message, it sends an acknowledgement back to the client to indicate that the response is on the way.
This mechanism of request and acknowledgement ensures that the client and server can communicate reliably and securely. It also allows for error handling and recovery, as the client can detect if a request is lost or the server is not responding and take appropriate action.
What the heck is JSON?
JSON stands for JavaScript Object Notation, and it is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate.
JSON is often used to transmit data between a server and a client, as well as between different software applications. It is based on a collection of name/value pairs, where the name is a string and the value can be a string, number, boolean, null, array, or another JSON object.
{
"name": "John",
"age": 30,
"city": "New York",
"isMarried": true,
"hobbies": ["reading", "music", "travel"],
"address": {
"street": "123 Main St",
"zip": "10001"
}
}
JSON is a lightweight data interchange format that consists of a collection of name/value pairs, where the name is a string and the value can be a string, number, boolean, null, array, or another JSON object. Here are some properties of JSON:
Easy to read and write: JSON is easy to read and write for humans, which makes it a popular choice for data interchange between different systems.
Lightweight: JSON is a lightweight data format, which makes it easy to transmit over the internet or between different software systems.
Supports arrays: JSON supports arrays, which are ordered collections of values.
Supports objects: JSON also supports objects, which are collections of name/value pairs.
Supports nested objects: JSON allows for nested objects, which means that an object can contain another object as a value.
Supports strings: JSON supports string values, which are enclosed in double quotes.
Supports numbers: JSON supports numeric values, which can be integers or floating-point numbers.
Supports boolean values: JSON supports boolean values, which can be either true or false.
Supports null values: JSON supports null values, which represent a lack of a value.
Language-independent: JSON is a language-independent data format, which means that it can be used with a variety of programming languages.
HTTP Methods
HTTP defines several methods, also known as verbs, that can be used to interact with web resources like APIs. These methods include:
GET: The GET method is used to retrieve information from a web resource, such as an API endpoint. It is a safe and idempotent method, meaning it can be called multiple times without changing the state of the resource.
POST: The POST method is used to submit data to a web resource, such as creating a new record in a database through an API. It is not idempotent, meaning calling it multiple times will create multiple new records.
PUT: The PUT method is used to update an existing resource on a web server, such as updating a user's profile through an API. It is idempotent, meaning calling it multiple times with the same data will have the same effect as calling it once.
DELETE: The DELETE method is used to delete a resource on a web server, such as deleting a user account through an API. It is idempotent, meaning calling it multiple times with the same data will have the same effect as calling it once.
PATCH: The PATCH method is used to update a portion of an existing resource on a web server, such as changing a user's email address through an API. It is not idempotent, meaning calling it multiple times with the same data may result in different outcomes.
Each of these HTTP methods has its own specific use case and should be used appropriately based on the requirements of the API and the specific operation being performed. By leveraging these methods effectively, developers can create powerful and flexible APIs that can handle a wide range of use cases and user interactions.
HTTP status codes are your best friend
How do you figure out if something is wrong with your APIs?
HTTP status codes are three-digit numbers that are returned by web servers to indicate the status of a client's request. They are grouped into 5 classes, each starting with a number from 1 to 5, and each indicating a different type of response:
1xx - Informational: The server has received the request, and the client should continue with its request.
2xx - Success: The request was successfully received, understood, and accepted.
3xx - Redirection: The client must take additional action to complete the request.
4xx - Client Error: The request contains bad syntax or cannot be fulfilled by the server.
5xx - Server Error: The server failed to fulfil a valid request.
Here are some common HTTP status codes and their meanings:
200 OK: The request was successful and the server has returned the requested data.
201 Created: The request was successful, and a new resource was created on the server.
204 No Content: The request was successful, but there is no content to return.
301 Moved Permanently: The requested resource has been permanently moved to a new location.
400 Bad Request: The server cannot understand the request due to bad syntax or missing parameters.
401 Unauthorized: The client must authenticate itself before accessing the resource.
403 Forbidden: The client does not have permission to access the requested resource.
404 Not Found: The requested resource could not be found on the server.
500 Internal Server Error: The server encountered an unexpected error and could not fulfil the request.
503 Service Unavailable: The server is currently unavailable or undergoing maintenance and cannot fulfil the request.
HTTP status codes are important because they provide information about the success or failure of a client's request, which can help diagnose and fix problems with web applications or services.
How to test APIs ?
There are several tools available for API testing that help developers and testers to automate, monitor, and analyze the behavior of APIs. Here are some of the most popular tools for API testing:
Postman: Postman is a popular API development tool that also includes powerful testing capabilities. It provides a user-friendly interface to create and send HTTP requests, and allows users to easily set up automated tests.
SoapUI: SoapUI is an open-source tool for functional testing, automated testing, and load testing of web services. It supports REST and SOAP APIs and provides a graphical user interface for test case creation and execution.
Swagger UI: Swagger UI is an open-source tool that provides a visual interface for API testing and documentation. It allows developers to interact with APIs and test them directly from the browser.
JMeter: JMeter is a popular open-source tool for load testing and functional testing of web applications, including APIs. It can simulate multiple concurrent users and can be used to test the performance and scalability of APIs.
Assertible: Assertible is a cloud-based API testing tool that allows developers and testers to create and run automated API tests. It supports a wide range of HTTP methods and provides detailed test reports.
Rest-Assured: Rest-Assured is a Java-based library that provides a domain-specific language for testing RESTful APIs. It allows developers to write concise and readable tests and provides powerful assertion capabilities.
Newman: Newman is a command-line tool that allows developers to run Postman collections in a CI/CD pipeline or from the command line. It can be used to automate API testing and integrate with other testing tools.
These are just a few of the many tools available for API testing, and the choice of tool will depend on the specific requirements and preferences of the developer or tester.
In conclusion, APIs have become an essential part of modern software development, allowing developers to create powerful and flexible applications that can integrate with a wide range of systems and services. APIs provide a standard way for different software components to communicate and exchange data, making it easier to build complex applications with diverse functionality. Understanding the basics of APIs, including how they work and how to interact with them, is critical for developers and other technical professionals working in the software industry. By leveraging APIs effectively, developers can accelerate the development process, improve the functionality of their applications, and provide a better user experience to customers. Additionally, APIs are driving innovation across many industries, from finance and healthcare to retail and transportation, and are likely to continue playing a vital role in shaping the future of technology.
Subscribe to my newsletter
Read articles from Rohit Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
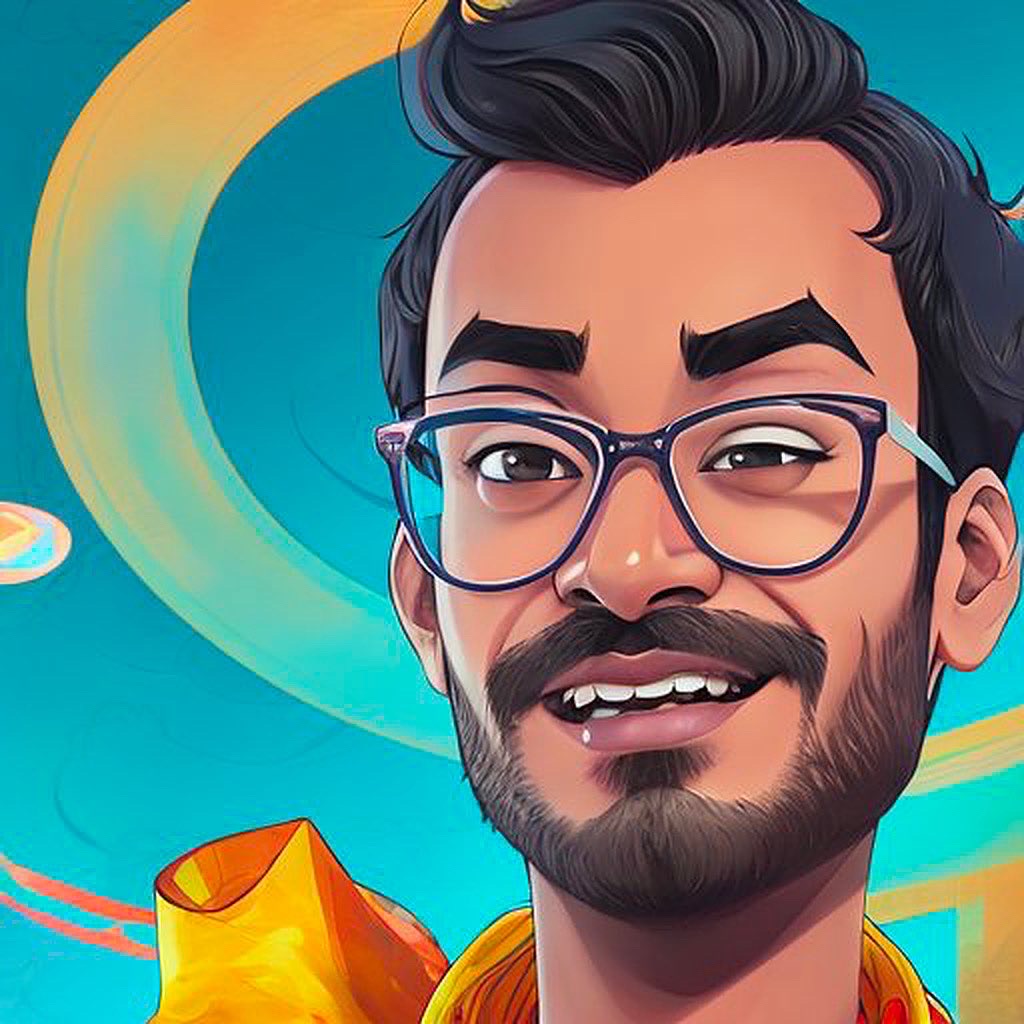
Rohit Jain
Rohit Jain
Product Technology Lead with 5+ years of experience, I specialize in driving product innovation and development through my skills in Java, NodeJS, AWS, Docker, Product Management and API platform development. My experience in product strategy and Cloud Computing expertise has enabled me to deliver scalable and robust solutions that drive business growth. I have a track record of successfully launching and scaling products and have the ability to lead cross-functional teams to deliver exceptional results. I am well-versed in cloud computing technologies, and have experience in designing and implementing cloud-based solutions. My passion for staying up-to-date with the latest technologies and trends in the industry makes me a valuable asset to any organization looking to implement and optimize their use of cloud technology.