Crack the Angular Code: 50 Questions to Test Your Knowledge and Skills
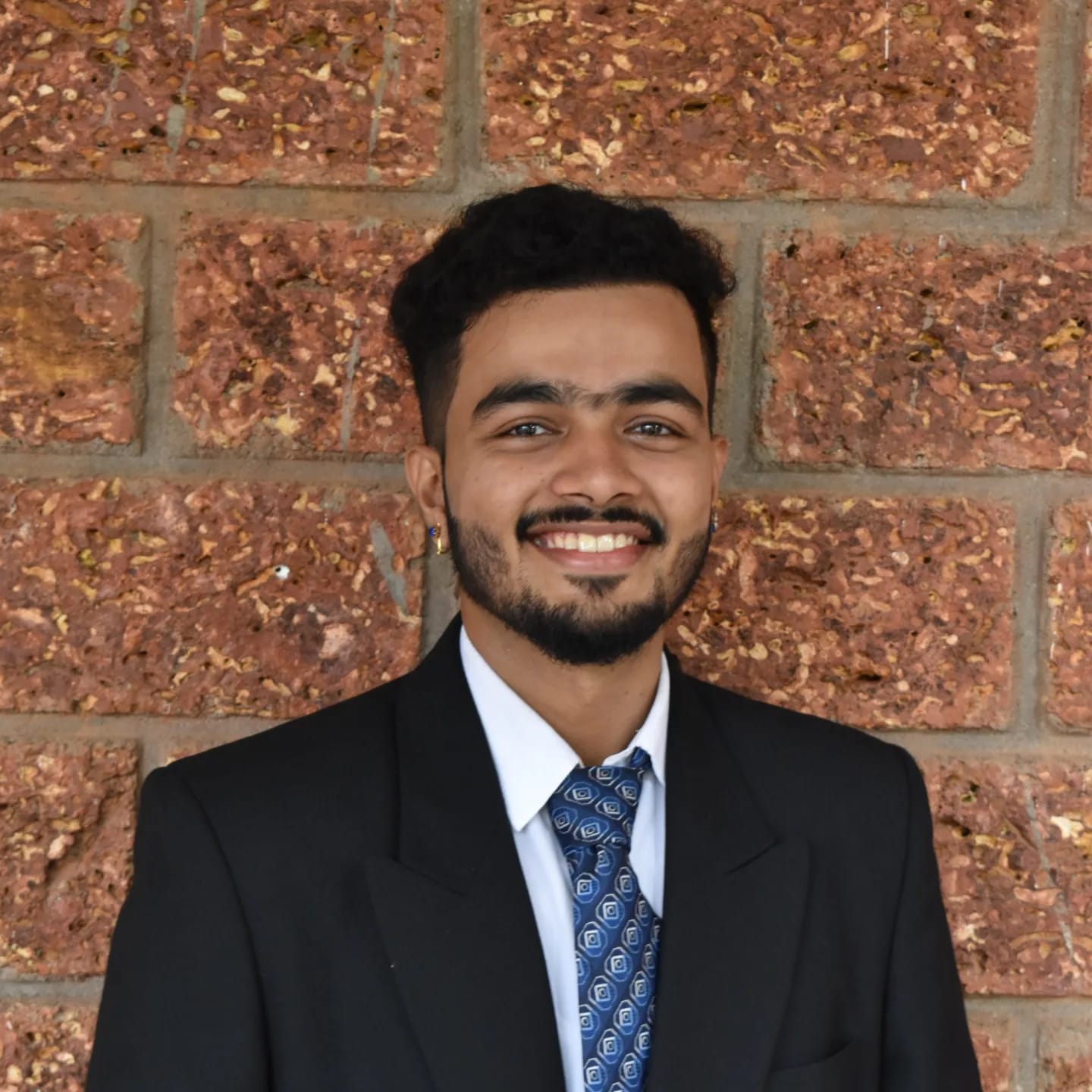
Table of contents
Angular is one of the most popular web application frameworks in the world, used by developers to build everything from simple websites to complex enterprise applications. Whether you're a beginner just learning the basics or an experienced developer looking to refine your skills, having a solid understanding of Angular is essential. To help you get there, we've compiled a list of 50 essential questions and coding challenges to test your Angular knowledge and skills.
HTML/CSS/JS Questions:
What is the difference between HTML and XHTML?
What are the different types of lists in HTML?
What is the purpose of the alt attribute in an image tag?
How do you link an external style sheet in HTML?
How do you create a hyperlink in HTML?
What is the CSS box model?
How do you center an element horizontally and vertically in CSS?
What is the difference between margin and padding in CSS?
How do you create a responsive website using CSS?
What is the difference between class and ID in CSS?
How do you create a hover effect using CSS?
What is a media query in CSS?
What is a pseudo-class in CSS?
How do you use CSS to create a drop-down menu?
What is a CSS reset?
What is the difference between display:none and visibility:hidden in CSS?
What is the difference between inline, block and inline-block elements in CSS?
What is the difference between absolute and relative positioning in CSS?
What is the purpose of a clearfix in CSS?
How do you create a gradient background in CSS?
JavaScript Questions:
What is JavaScript and what are its advantages?
What is the difference between let, const and var in JavaScript?
What is hoisting in JavaScript?
What is the purpose of the keyword this in JavaScript?
What is a closure in JavaScript?
What are the different data types in JavaScript?
What is the difference between null and undefined in JavaScript?
What is a callback function in JavaScript?
How do you declare and use an object in JavaScript?
What is the difference between == and === in JavaScript?
How do you loop through an array in JavaScript?
How do you create and use a function in JavaScript?
What is the difference between a for loop and a forEach loop in JavaScript?
What is the difference between synchronous and asynchronous code in JavaScript?
What is the difference between a promise and a callback in JavaScript?
What is the purpose of the spread operator in JavaScript?
What is a generator function in JavaScript?
What is destructuring in JavaScript?
What is the difference between a reference type and a value type in JavaScript?
How do you handle errors in JavaScript?
TypeScript Questions:
What is TypeScript and what are its advantages?
How does TypeScript differ from JavaScript?
What are the different data types in TypeScript?
What is the purpose of an interface in TypeScript?
What is the difference between a class and an interface in TypeScript?
How do you use generics in TypeScript?
What is a union type in TypeScript?
What is an intersection type in TypeScript?
What is a type alias in TypeScript?
How do you declare and use an enum in TypeScript?
How do you declare and use a namespace in TypeScript?
How do you use decorators in TypeScript?
How do you use async and await in TypeScript?
How do you declare and use a module in TypeScript?
What is the difference between declare and export in TypeScript?
How do you use type assertions in TypeScript?
How do you use type guards in TypeScript?
What is the difference between any and unknown in TypeScript?
What is a tuple type in TypeScript?
How do you handle errors in TypeScript?
Angular Questions:
What is Angular and what are its advantages?
What is the difference between AngularJS and Angular?
What is the Angular CLI and how do you use it to create and manage an Angular project?
How do you create a new Angular project using the CLI?
What is an Angular module and what is its purpose?
What is the difference between a component and a directive in Angular?
How do you create a new component in Angular?
What is data binding in Angular?
What is the difference between one-way binding and two-way binding in Angular?
How do you handle user input in Angular?
What is dependency injection in Angular?
How do you create a service in Angular?
What is a provider in Angular?
How do you use HTTP services in Angular to retrieve data from a server?
What is a router in Angular and how do you use it?
How do you handle errors in Angular applications?
What is lazy loading in Angular and why is it useful?
How do you use forms in Angular?
What is an Angular pipe and how do you create one?
What is Angular Material and how do you use it in an Angular project?
Coding Test:
Write a function in JavaScript that takes an array of numbers and returns the sum of all the even numbers in the array.
Write a function in TypeScript that takes a string and returns the reverse of the string.
Create an HTML form with a text input and a button. When the button is clicked, display an alert with the value of the text input.
Create a CSS class that will center an element both horizontally and vertically.
Create an Angular component that displays a list of items retrieved from an HTTP service.
Write a function in JavaScript that takes an array of strings and returns the longest string in the array.
Create an Angular component that displays a form with input fields for name, email, and password. When the form is submitted, use an HTTP service to send the data to a server.
Write a function in TypeScript that takes an object with properties name and age and returns a string in the format "My name is <name> and I am <age> years old."
Create a CSS class that will create a border around an element with a specified color and thickness.
Create an Angular pipe that takes a string and returns the string in uppercase.
Write a function in JavaScript that takes an array of numbers and returns a new array with only the positive numbers.
Create an Angular component that displays a list of items with a checkbox next to each item. When the user clicks a checkbox, add or remove the item from a selected items array.
Write a function in TypeScript that takes an array of numbers and returns the sum of all the numbers in the array.
Create a CSS class that will make an element's text color white and its background color black.
Create an Angular directive that will highlight an element when the user hovers over it.
Write a function in JavaScript that takes a string and returns the number of vowels in the string.
Create an Angular component that displays a list of items and allows the user to sort the list by different criteria.
Write a function in TypeScript that takes an array of strings and returns a new array with only the strings that contain the letter "a".
Create a CSS class that will rotate an element by 45 degrees.
Create an Angular pipe that takes a number and returns the number with a dollar sign and two decimal places.
Whether you're preparing for an Angular job interview, studying for a certification exam, or just looking to improve your skills, these 50 questions and coding challenges provide a comprehensive overview of the framework's most important concepts and features.
By mastering these topics, you'll be well on your way to becoming an expert in Angular development and creating high-quality web applications that are responsive, scalable, and easy to maintain. So take some time to go through the questions and challenges in this list, and see how much you know about Angular - you might just surprise yourself with what you can accomplish!
Subscribe to my newsletter
Read articles from Shivathmaj Shenoy M directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
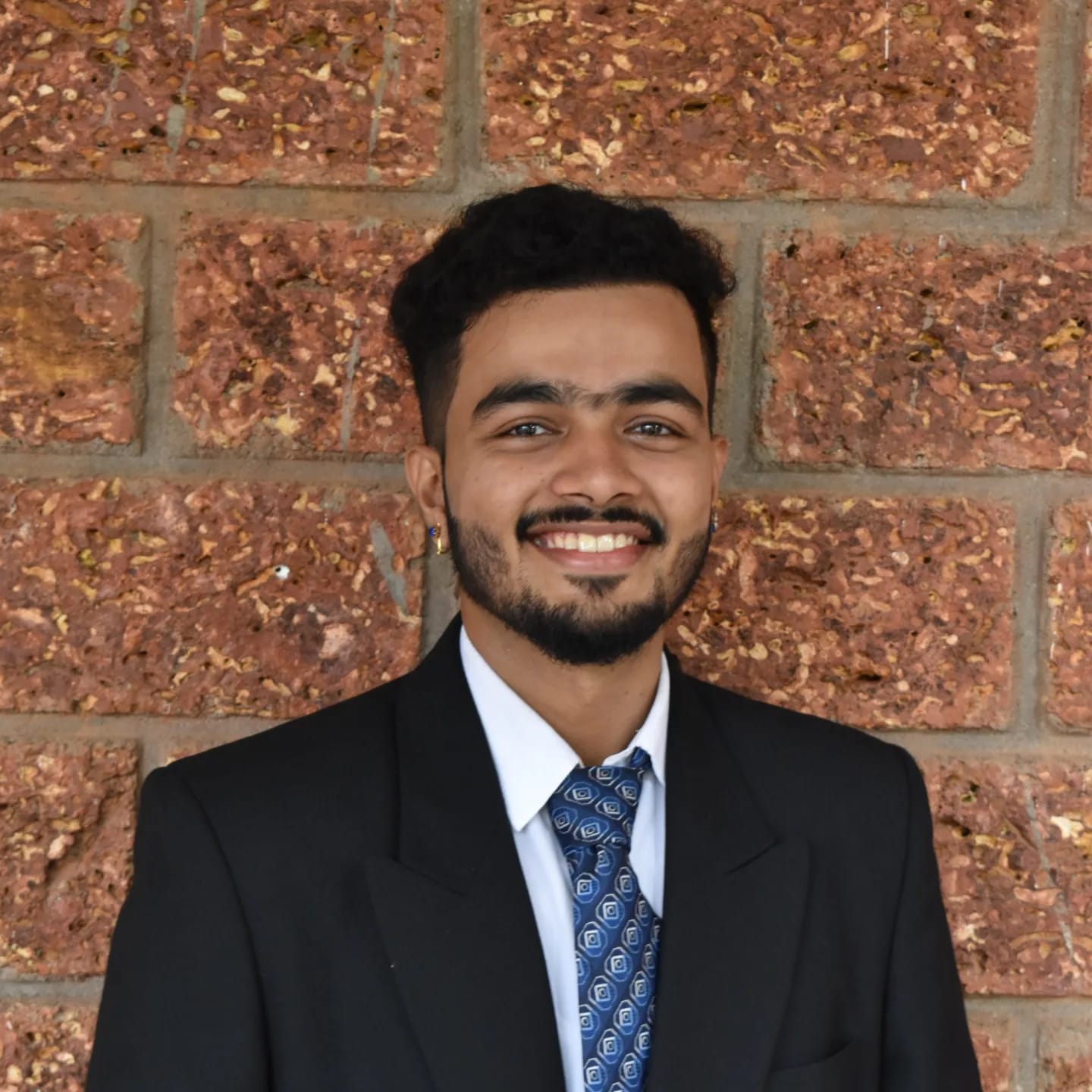
Shivathmaj Shenoy M
Shivathmaj Shenoy M
A skilled and experienced Front-End Web Developer with a passion for creating visually appealing and user-friendly websites. Proficient in a variety of technologies, including Angular, TypeScript, HTML, CSS, and JavaScript. Strong understanding of web development best practices and a keen eye for design. Committed to delivering high-quality and innovative web solutions that exceed client expectations. Dedicated to staying up-to-date with the latest technologies and trends in the industry.