What is the Difference Between Component DidMount and Component DidUpdate
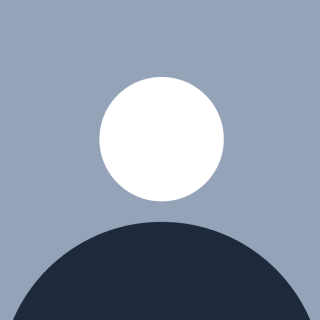
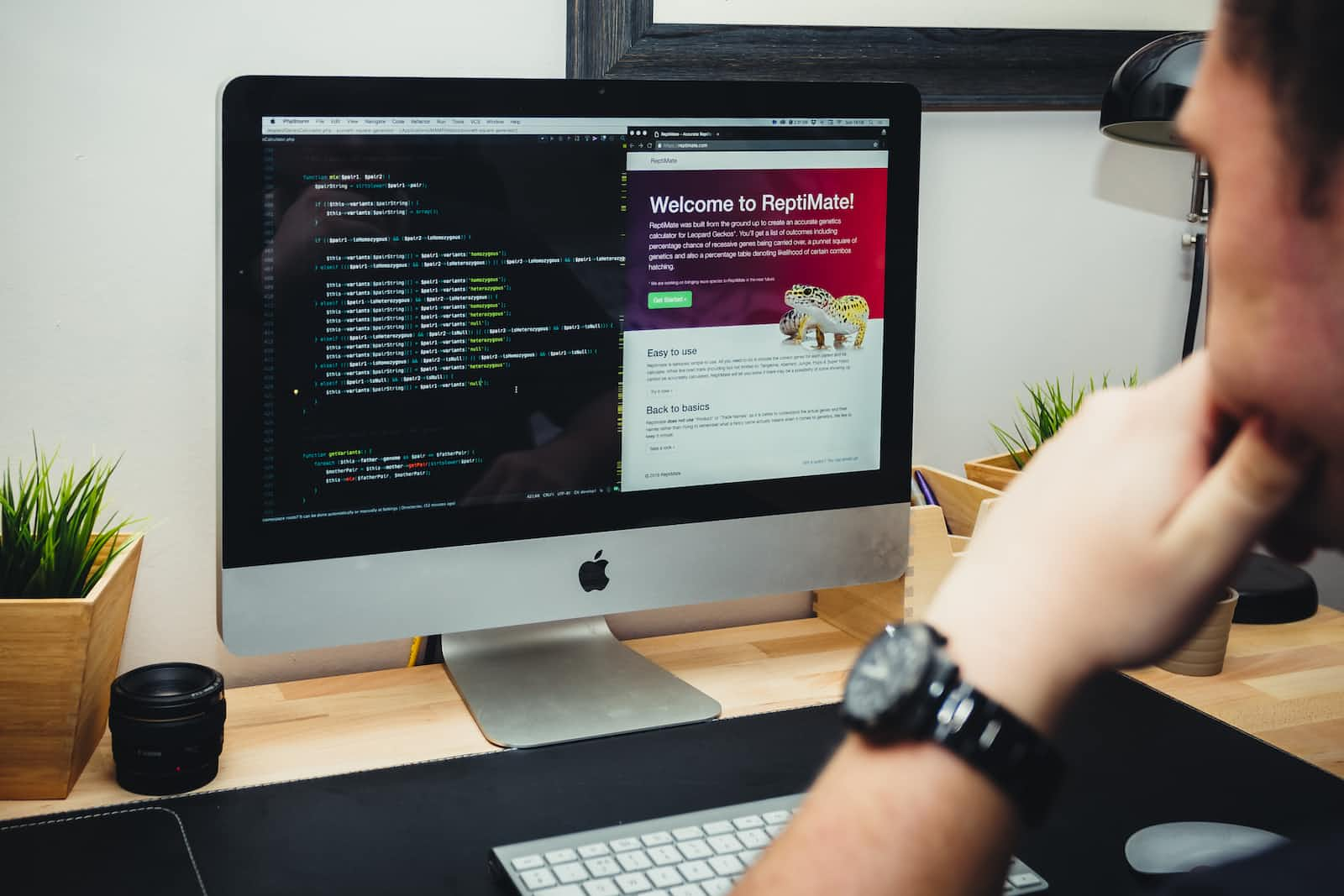
Welcome to my blog, In this blog post, we will explore the difference between DidMount and DidUpdate in React.
componentDidMount() and componentDidUpdate() are lifecycle methods in React that are called at different stages of a component's lifecycle.
componentDidMount() is called once, immediately after a component is mounted (i.e., inserted into the DOM tree). This method is typically used to perform initialization tasks, such as fetching data from an external source or setting up event listeners.
import React from "react";
import "./App.css";
// Life cycle components Component Did Mount
class App extends React.Component() {
constructor() {
super();
this.state = {
name: "preeti",
};
// console.warn("constructor");
}
componentDidMount() {
console.warn("componentDidount");
}
render() {
console.warn("render");
return (
<div className="App">
<h1>Component DidMount{this.state.name}</h1>
<button
onClick={() => {
this.setState({ name: "kamilla" });
}}
>
Update Name
</button>
</div>
);
}
}
export default App;
componentDidUpdate() is called after a component's state or props have been updated and the component has been re-rendered. This method is typically used to perform tasks that need to be updated in response to changes in the component's state or props, such as updating the DOM or fetching new data based on the new state or props.
import React from "react";
import "./App.css";
//Lifecycle componentDidUpdate
class App extends React.Component() {
constructor() {
super();
console.warn("constructor");
this.state = {
// name: "preeti",
count: 0,
};
}
//this will update the pervious and persent state
componentDidUpdate(perProps, perState, snapShot) {
//sanpashot gives us undefined when it is executed
console.warn("ComponentDidUpdate", perState.count, this.state.count);
// if (perState.count === this.state.count) {
// alert("data is already same");
// }
//infinte loop and send us the max depth achived
//this.setState({ count: this.state.count + 1 });
//instead we can make a condition for this to stop going it into infinite loop
if (this.state.count < 10) {
this.setState({ count: this.state.count + 1 });
}
}
render() {
console.warn("render");
return (
<div className="App">
<h1>Component Did Update{this.state.count}</h1>
{/* <button
onClick={() => {
this.setState({ name: "Kamilla" });
}}
></button> */}
{/* <button
onClick={() => {
this.setState({ count: this.state.count + 1 });
}}
>
Update Name
</button> */}
<button
onClick={() => {
this.setState({ count: 1 });
}}
>
Update Name
</button>
</div>
);
}
}
export default App;
//can we make conditions in render or update state in render method?
//no we should not update the state in the render method because it update automaically so when we want to update the state we should do it in the component
//can we stop the component did mount method?
// yes we can stop the component didmount method to stop shouldComponentUpdate() return false
In summary, componentDidMount() is called once when the component is first mounted, while componentDidUpdate() is called every time the component is updated. componentDidMount() is typically used for initialization tasks, while componentDidUpdate() is typically used for tasks that need to be updated in response to changes in the component's state or props.
Hope you understood the concepts follow for more such updates. I am open to suggestions and queries. Feel free to ping me. you can follow me on Twitter and Linkedin.
Subscribe to my newsletter
Read articles from Preeti samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Preeti samuel
Preeti samuel
I am Kamilla Preeti Samuel, a Fullstack Developer with a strong command of JavaScript, Node.js, MongoDB, MySQL, CSS, and HTML. Over the years, I have built and worked on a range of applications, gaining valuable hands-on experience in both backend and frontend development. My professional journey includes working as a Junior Software Engineer at Bytestrum, where I focused on software development, and at NUK9 as a UX and UI Designer, contributing to creating user-centered design solutions. I thrive on building efficient, scalable, and user-friendly applications, combining technical expertise with a keen eye for design. I enjoy collaborating with cross-functional teams to create seamless digital experiences, and I am passionate about continuously exploring new tools and frameworks to stay ahead in the fast-evolving tech landscape. I am Kamilla Preeti Samuel, a full-stack developer with a strong command of JavaScript, Node.js, MongoDB, MySQL, CSS, and HTML. Over the years, I have built and worked on various applications, gaining valuable hands-on experience in both backend and frontend development. My professional journey includes working as a Junior Software Engineer at Bytestrum, where I focused on software development, and at NUK9 as a UX and UI Designer, contributing to creating user-centered design solutions. I thrive on building efficient, scalable, and user-friendly applications, combining technical expertise with a keen eye for design. I enjoy collaborating with cross-functional teams to create seamless digital experiences, and I am passionate about continuously exploring new tools and frameworks to stay ahead in the fast-evolving tech landscape.