JavaScript BigInt
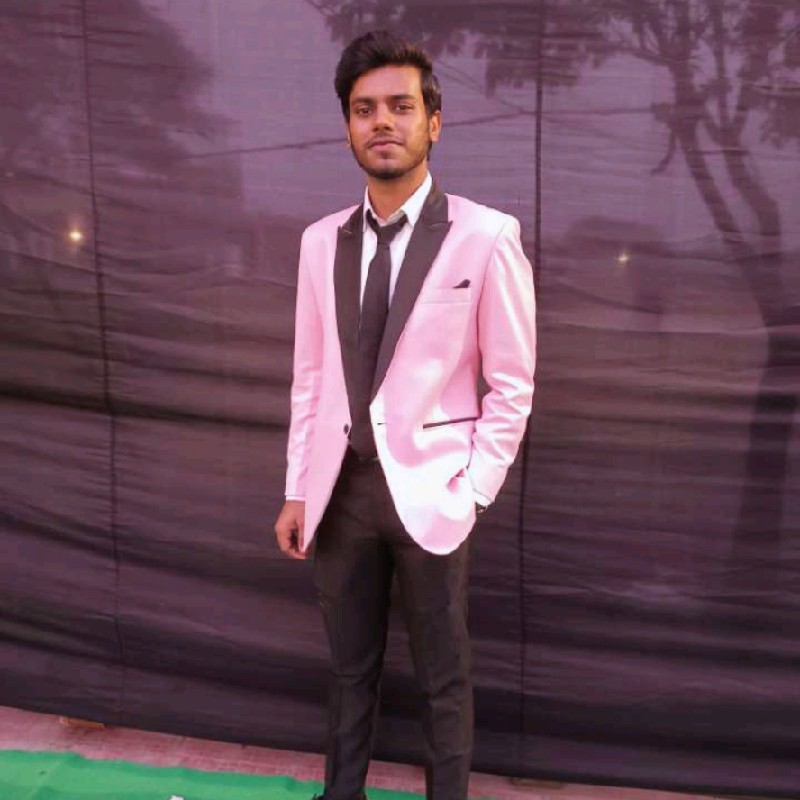
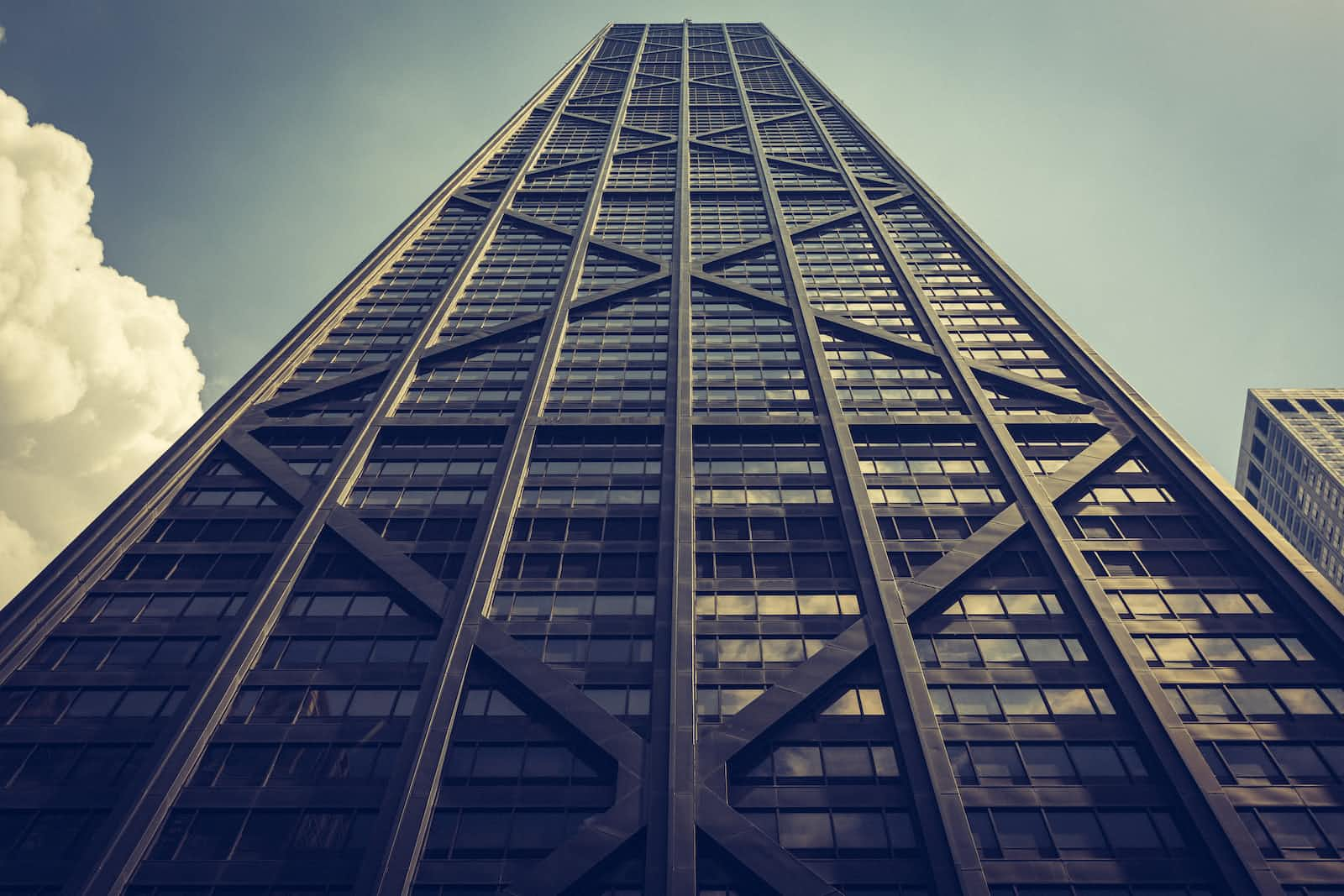
BigInt was introduced in ES2020. BigInt is one of the primitives in JavaScript.
Why We Need BigInt?
In JavaScript, regular numbers are represented internally as 64 bits. that means there are exactly 64 0's and 1's to represent any number. Only 53 out of 64 bits are used to store digits, the rest are used to represent the position of the decimal point and the sign (i.e. positive or negative).
It means, the biggest number that javascript can represent safely is 2^53 - 1, and to get this biggest number we can also use Number.Max_Safe_Integer.
console.log(2 ** 53 - 1); // 9007199254740991
console.log(Number.MAX_SAFE_INTEGER); // 9007199254740991
console.log(2 ** 53 - 1 === Number.MAX_SAFE_INTEGER) // true
To overcome this problem of storing big numbers, ES2020 introduced BigInt, which is just another primitive in JavaScript.
Now using BigInt we can store a number as big as we want.
How To Create A BigInt
//put n at the end of the number to create BigInt
const hugeNumber = 4829243020238349234230282042842429023n;
console.log(typeof hugeNumber); // BigInt
//We can also use BigInt constructor Function to create a BigInt
console.log(typeof BigInt(382392482n)); // BigInt
Operations On BigInt
We can perform all basic operations like (+, -, *, /, etc);
console.log(383473n + 23823723n); // 24207196n //BigInt cuts off the decimal parts console.log(10n / 3n); // 3n console.log(10 / 3) // 3.33333333
We can not perform any Math operations like (Math.sqrt(), Math.round(), etc);
console.log(Math.sqrt(383473n)) // TypeError: Cannot convert a BigInt value to a number
We can not mix a BigInt and a Regular Number;
const hugeNumber = 292392n; const num = 34; console.log(num * hugeNunmber); // TypeError: Cannot mix BigInt and other types, use explicit conversions
Exceptions
We can use a comparison operator by mixing a BigInt and a Regular Number;
console.log(232n > 23) // true console.log(20n === 20) // false console.log(20n == 20) //true console.log(20n > 20) // false
BigInt gets concatenated with strings;
const hugeNumber = 49232n; console.log(hugeNumber + " is a BigInt"); // 49232 is a BigInt
THANKS FOR READING!โค
If you have any questions you can ask me in comment box.๐
Subscribe to my newsletter
Read articles from Akash Deep Chitransh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
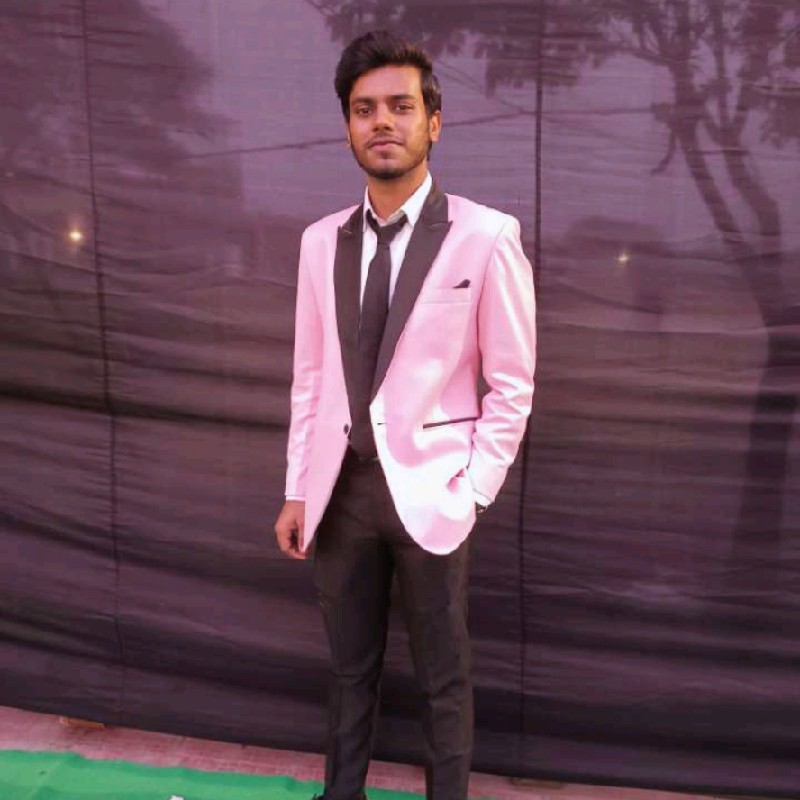
Akash Deep Chitransh
Akash Deep Chitransh
I am a front-end developer, currently working at TCS.