Hangman Game in C!!
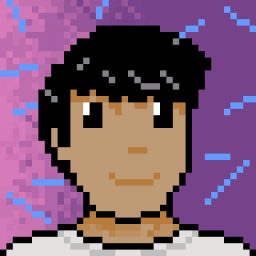
Hangman is a timeless classic that has been enjoyed for generations. The thrill of guessing letters in a word and avoiding the dreaded noose has kept people on the edge of their seats for years. Are you ready to take on the challenge? With just a few lines of code in C, you can bring this exhilarating game to life. Don't miss out on the fun - let's get started!
Flowchart:
A flow chart is an excellent tool that can help us visualize how the game will work. It simplifies the process and makes it easier to understand. With the help of a flow chart, we can easily see how the game will progress and what steps are involved. It's a great way to get a clear picture of the game mechanics and how everything fits together. So let's take a look at the flow chart and get a better understanding of how this exciting game works!
Code:
To program Hangman in C, we will be using the standard libraries that come with most compilers. The main function of our program will be to generate a random word from a predefined list of words and allow the player to guess letters until they either win or lose.
To achieve this, we will need to use several different functions such as rand() to generate a random number, strlen() to get the length of a string, and scanf() to read user input. We will also use loops and conditional statements to control the flow of the game and check for correct or incorrect guesses.
Steps to program the game:
STEP 1: Add the useful headers.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
STEP 2: Make a function that helps the program come up with the words from a predefined array.
#define EASY_WORDS_COUNT 4
#define MEDIUM_WORDS_COUNT 4
#define HARD_WORDS_COUNT 4
void wordchoose(char *a, int b)
{
srand(time(0));
int ind = (rand() % 4);
const char *easy[EASY_WORDS_COUNT] = {"tree", "brim", "club", "blub"};
const char *medium[MEDIUM_WORDS_COUNT] = {"first", "scoop", "eight", "nasty"};
const char *hard[HARD_WORDS_COUNT] = {"school", "circle", "access", "lesson"};
switch (b)
{
case (1):
strcpy(a, easy[ind]);
break;
case (2):
strcpy(a, medium[ind]);
break;
case (3):
strcpy(a, hard[ind]);
break;
default:
printf("Enter a valid option");
exit(1);
}
}
Here char *a is the pointer of the array in which we are going to copy our word for further use and int b is going to be the difficulty of the game chosen by the user.
STEP 3: Now we will start writing code in our main function.
int main()
{
int dif, i = 0, length, win = 0, life = 5, count = 0, limit, found = 0;
char word[10] = {};
char blank[10] = {};
char guess[10] = {};
scanf("%i", &dif);
wordchoose(word, dif);
length = strlen(word);
for(i=0; i<length; i++)
{
blank[i] = '_';
}
STEP 4: Now we need to write a while loop that will run as long as the player does not win or lose.
while(win == 0 && life > 0)
{
printf("%s\n", blank);
printf("Lifes left : %i\t Words found:%i\nEnter the letter\n", life, found);
scanf(" %c", &guess[0]);
count = 0;
for (i = 0; i<length; i++)
{
if (guess[0] == word[i])
{
blank[i] = guess[0];
count++;
found++;
}
}
if (count == 0)
{
life--;
}
if (found == length)
{
win = 1;
}
}
printf("%s\n",blank);
if (win == 0 && life == 0)
{
printf("you lose");
}
else
{
printf("you win");
}
return 0;
}
Problems I faced during writing this code:
Passing an Array as a Function Argument.
Certain usage of scanf caused the while loop to fail in taking input during its first cycle.
Fix: The reason we write
scanf(" %s",&s)
instead ofscanf("%s",&s)
is to prevent the input buffer from reading the newline character that is left in the input buffer after the user hits the enter key.
Subscribe to my newsletter
Read articles from Pratyush Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
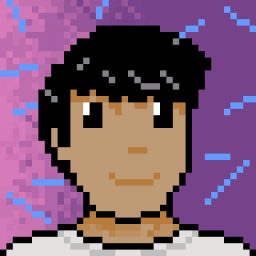