Client-side AJAX using JSON
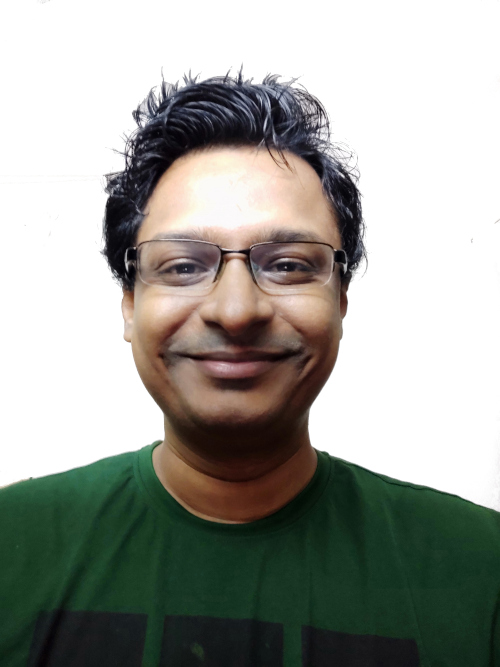
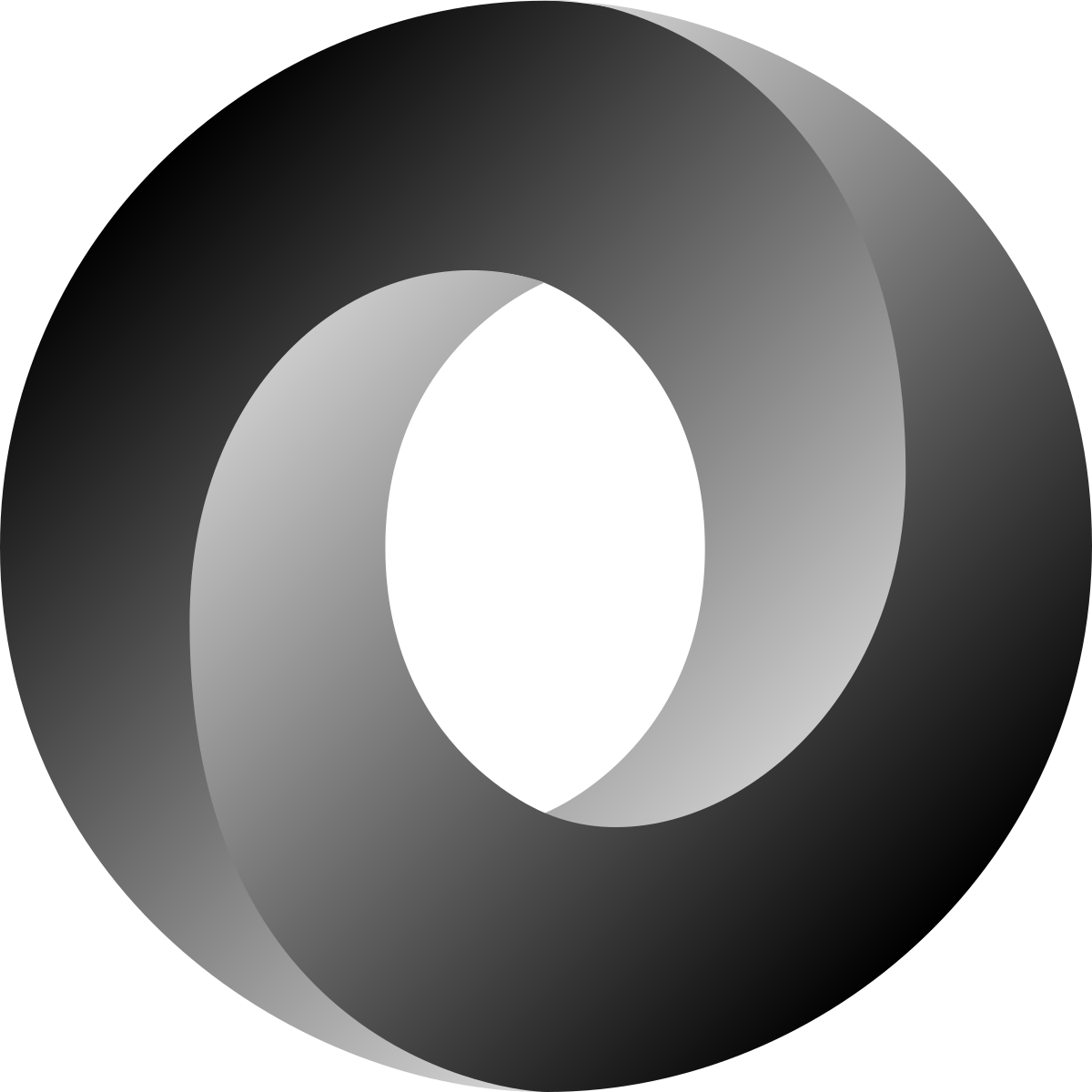
Very often, many developers, including myself in the past, normally all along we were using jQuery's $.ajax
and sending in JSON with dataType: 'json'
without really sending the content-type
as a JSON string which is supposed to be application/json
- instead we were actually sending in a regular POST (application/x-www-urlencoded
) and decoding it from the PHP side as $_POST
.
So, if you have this script, post.php, in a folder :
<?php
$post_body = $_POST;
echo json_encode($post_body);
?>
and in jqurey-ajax.html :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>jQuery AJAX</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
</head>
<body>
<script>
let url = "http://localhost:8989/post.php";
const formData = new FormData();
formData.append("username", "john");
formData.append("password", "a5y6e7Chk65kmFWeK5F5");
const run = async() =>
{
$.ajax({
type: 'ajax',
method: 'post',
url: url,
data: {username: "john", password: "a5y6e7Chk65kmFWeK5F5"},
async: false,
dataType: 'json',
success: function(result) { console.log(result); },
error: function() { console.log("error"); }
});
}
run();
</script>
</body>
</html>
we get :
The Real JSON
For sending in non-JSON requests
Have this script, run.php, in the same folder :
<?php
$post_body = file_get_contents('php://input');
parse_str($post_body, $queryArray);
print_r($queryArray);
?>
If you have this HTML file in the same folder as client.html
let url = "http://localhost:8989/run.php";
const formData = new FormData();
formData.append("username", "john");
formData.append("password", "a5y6e7Chk65kmFWeK5F5");
const run = async() =>
{
let response = await fetch(url,
{
method: "POST",
headers:
{
"Content-Type": "application/x-www-urlencoded",
},
body: new URLSearchParams(formData),
});
}
run();
If you run `php.exe -S localhost:8989`, you should get this in the response of the URL request you'll see in console :
Array
(
[username] => john
[password] => a5y6e7Chk65kmFWeK5F5
)
For sending in JSON requests
run.php :
<?php
$post_body = file_get_contents('php://input');
print_r($post_body);
?>
client.html :
let url = "http://localhost:8989/run.php";
const formData = new FormData();
formData.append("username", "john");
formData.append("password", "a5y6e7Chk65kmFWeK5F5");
const run = async() =>
{
var object = {};
formData.forEach((value, key) => object[key] = value);
let response = await fetch(url,
{
method: "POST",
headers:
{
"Content-Type":"application/json",
},
body: JSON.stringify(object)
});
}
run();
We get :
username "john"
password "a5y6e7Chk65kmFWeK5F5"
I haven't echoed a JSON string to the output in the above 2 examples because I just wanted show how it's communicated - I'll leave it up to you to print a JSON string on the server-side for the client to fetch as response.
Subscribe to my newsletter
Read articles from Anjanesh Lekshminarayanan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
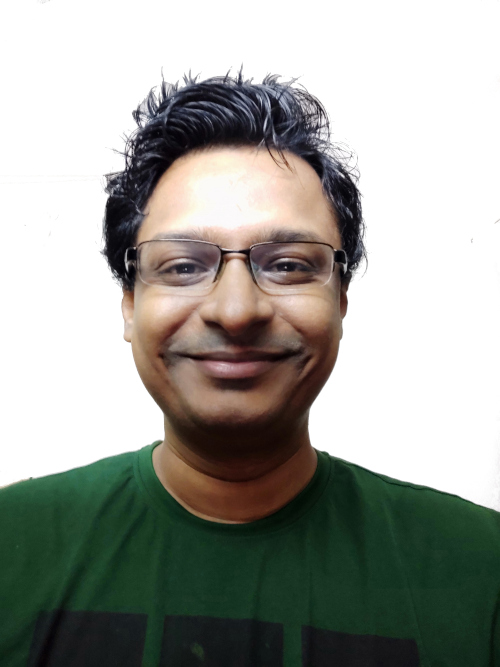
Anjanesh Lekshminarayanan
Anjanesh Lekshminarayanan
I am a web developer from Navi Mumbai. Mainly dealt with LAMP stack, now into Django and getting into Laravel and Google Cloud. TensorFlow in the near future. Founder of nerul.in and gaali.in