React MUI Select All Checkbox With Simple Logic
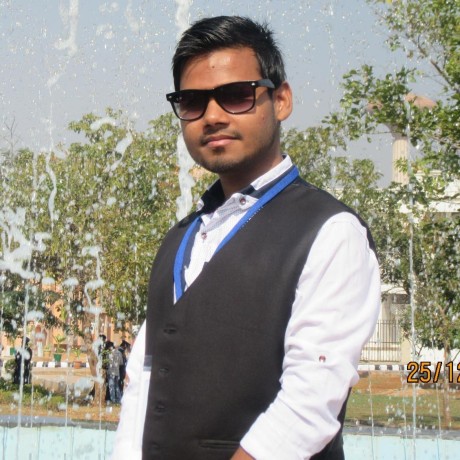
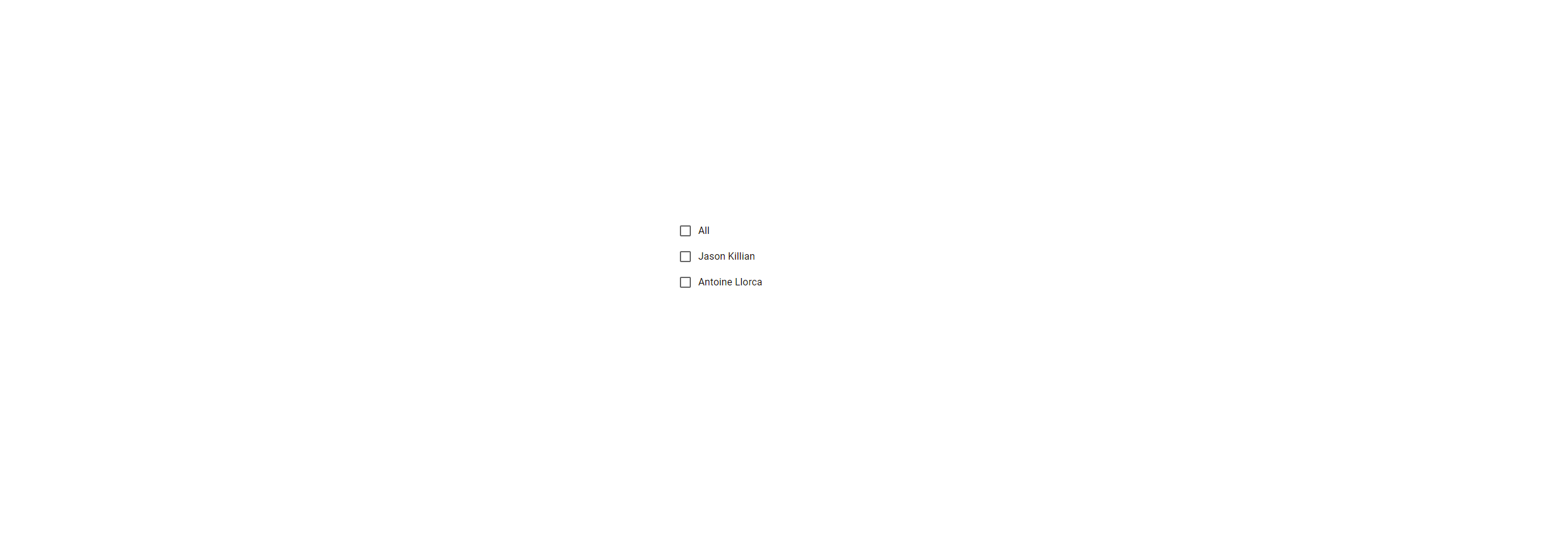
Selecting multiple items from a list is a common feature in many web applications. Often, users need to select all items in the list, instead of selecting them one by one. This is where the "Select All" checkbox comes in handy. In this article, we will explore how to implement the "Select All" functionality with checkboxes in a React application using the Material-UI library.
Material-UI is a popular UI library for React that provides a set of reusable components for building web applications. It includes a checkbox component that we can use to implement the "Select All" functionality. Let's get started!
First, let's create a basic React component that renders a list of items with checkboxes. Here's the code for the CheckBoxWithSelectAll
component:
import * as React from 'react';
import FormGroup from '@mui/material/FormGroup';
import FormControlLabel from '@mui/material/FormControlLabel';
import Checkbox from '@mui/material/Checkbox';
import { FormControl, FormHelperText, FormLabel } from '@mui/material';
export default function CheckBoxWithSelectAll() {
const [state, setState] = React.useState({
jason: false,
antoine: false,
});
const objectMap = (obj, fn) =>
Object.fromEntries(
Object.entries(obj).map(([k, v], i) => [k, fn(v, k, i)])
);
const handleChange = (event: React.ChangeEvent<HTMLInputElement>) => {
if (event.target.name === 'all') {
// const newObj: any = objectMap(state, (value) => event.target.checked);
Object.keys(state).forEach(key => state[key]=event.target.checked);
setState({...state});
} else {
setState({
...state,
[event.target.name]: event.target.checked,
});
}
};
console.log(state);
const { jason, antoine } = state;
const error = false;
return (
<FormControl>
{/* <FormLabel component="legend">Demo </FormLabel> */}
<FormGroup>
<FormControlLabel
control={
<Checkbox
checked={
Object.values(state).filter((value) => value !== true).length <1
}
onChange={handleChange}
name="all"
/>
}
label="All"
/>
<FormControlLabel
control={
<Checkbox checked={jason} onChange={handleChange} name="jason" />
}
label="Jason Killian"
/>
<FormControlLabel
control={
<Checkbox
checked={antoine}
onChange={handleChange}
name="antoine"
/>
}
label="Antoine Llorca"
/>
</FormGroup>
<FormHelperText></FormHelperText>
</FormControl>
);
}
In this example, we are using the useState
hook to maintain the state of the checkboxes. We are also using the FormGroup
, FormControlLabel
, and Checkbox
components from MUI to create the checkboxes. The checked
prop of the Checkbox
component specifies whether the checkbox is checked or not, and the onChange
prop specifies the function to be called when the checkbox is clicked. The name
prop is used to identify the checkbox. The label
prop of the FormControlLabel
component specifies the label of the checkbox.
Full Code
https://stackblitz.com/edit/react-jmpp88?embed=1&file=demo.tsx
Subscribe to my newsletter
Read articles from Alam Jamal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
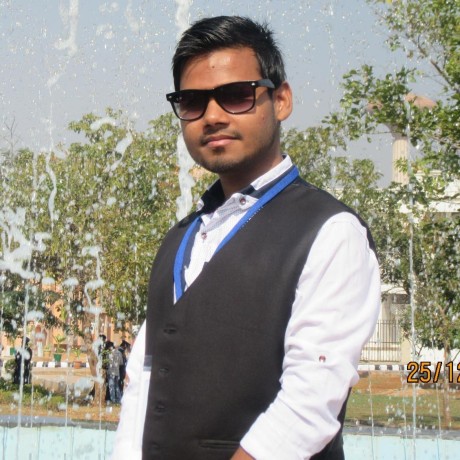
Alam Jamal
Alam Jamal
Building LMS Backend