A Flutter Template from ICAPPS: Part 2
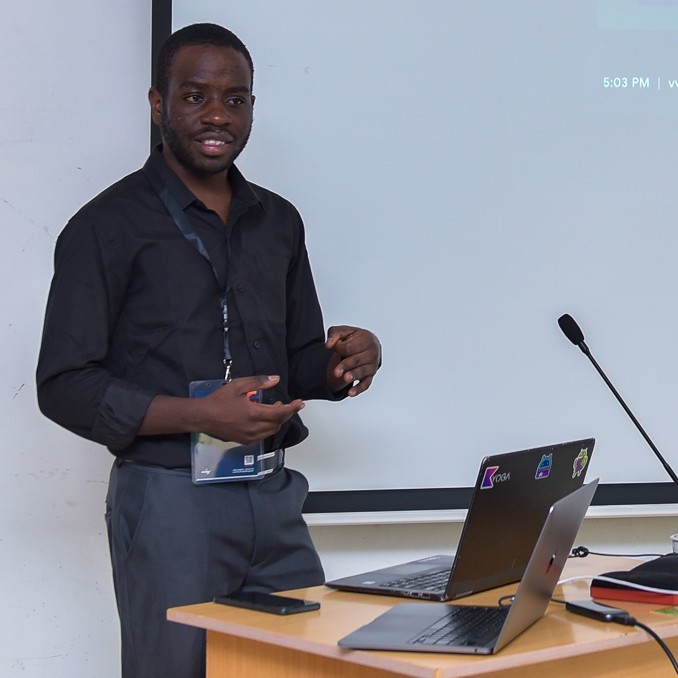
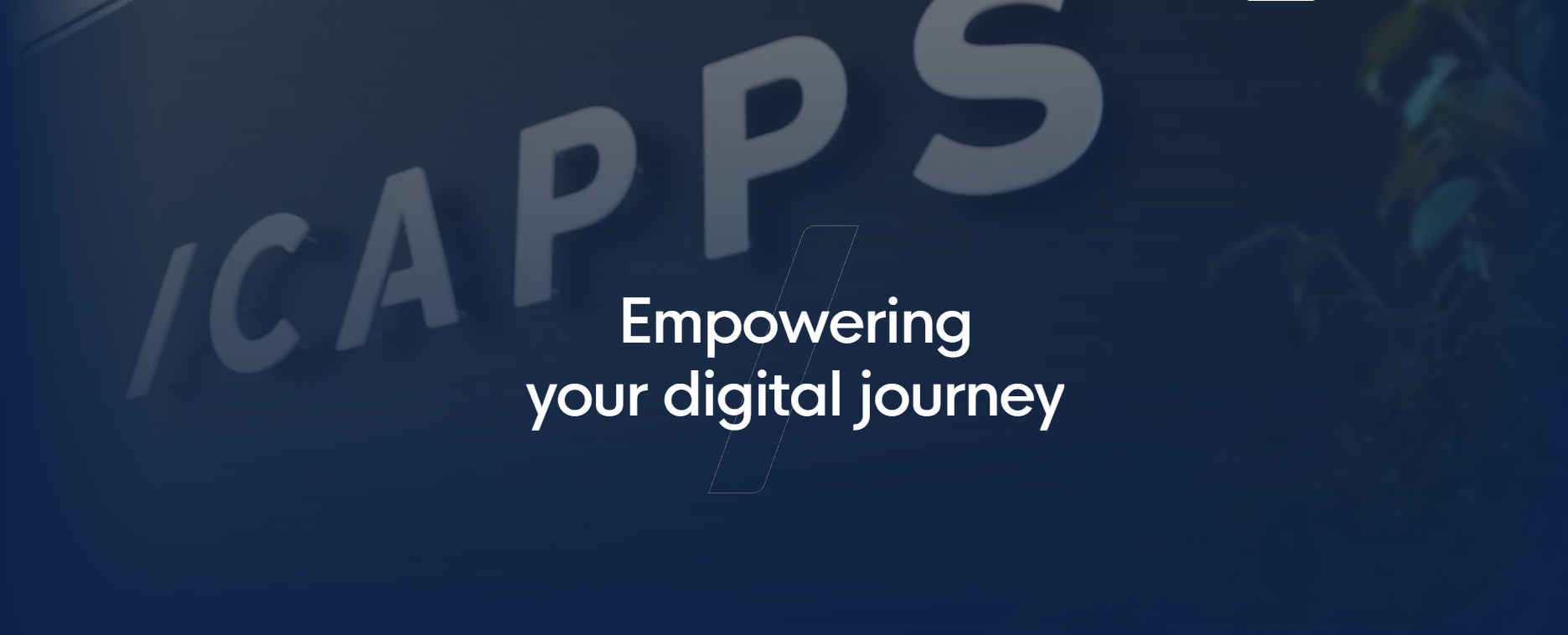
Having introduced you to the ICAPPS Flutter Template in my previous article, I wish to continue talking about the features of the template from where I left off. If you didn't read the article then I politely ask you to read it before proceeding with this.
6. Json Serialization
JSON serialization is a crucial part of data communication in modern applications. The ICAPPS Flutter template provides a simple yet powerful way to serialize and deserialize JSON data using the json_serializable
package. It reduces the risk of runtime errors and improves the efficiency of data communication.
The json_serializable
package is a code generator that generates serialization and deserialization code for Dart classes. It uses annotations to specify which classes should be serialized and how they should be serialized. The package generates code that is efficient and type-safe, reducing the risk of runtime errors.
In the template, the json_serializable
package is used to serialize and deserialize JSON data for the application's models and data classes. The package generates serialization and deserialization code based on annotations in the model classes.
To use json_serializable
, the model classes must be annotated with the @JsonSerializable
annotation. The @JsonSerializable
annotation specifies how the class should be serialized and deserialized, including the property names and data types.
7. Different Environments
The ICAPPS Flutter template supports multiple environments, including dummy, dev (debug), beta, alpha, and prod (release). Each environment has its own set of configurations, such as API endpoints, authentication tokens, and other parameters.
To launch the application in a specific environment, you can use the following commands:
Dev, Debug:
flutter run --flavor dev -t lib/main.dart
Dummy:
flutter run --flavor dev -t lib/main_dummy.dart
Alpha:
flutter run --flavor alpha -t lib/main_alpha.dart
Beta:
flutter run --flavor beta -t lib/main_beta.dart
Prod, Release:
flutter run --flavor prod -t lib/main_prod.dart
These commands specify the flavor and entry point file for the desired environment.
In general, having multiple environments is useful for managing the different stages of development and deployment. Each environment can have its own set of configurations, which allows you to test the application in different scenarios and environments. For example, you can have a dummy environment that simulates server responses, a development environment with different API endpoints, and a production environment with optimized settings.
By using environment-specific configurations, you can ensure that the application behaves correctly in each environment and reduces the risk of errors and bugs in production. It also allows you to manage and test different configurations without modifying the code, which can be useful for debugging and troubleshooting.
Overall, the support for multiple environments in the template provides a flexible and robust way to manage configurations for different stages of development and deployment. It allows you to customize the application behavior based on the environment and provides a better development experience.
8. Themes
The template provides support for themes, which allows you to define a set of styles and colors that can be used throughout the application. Themes are an important part of the design and user experience of an application, and they can help create a consistent and cohesive look and feel across the entire app.
Themes are defined using the ThemeData
class, which allows you to specify the colors and styles for different parts of the application, such as the app bar, text, buttons, and backgrounds.
The template provides a base theme that is used as the default theme for the application. This base theme can be customized by creating a new theme that extends the base theme and overrides specific properties as needed. This approach allows you to create multiple themes for different use cases, such as a dark theme, a light theme, or a theme with custom colors.
To apply the theme to the application, you can use the Theme
widget, which wraps the root widget of the application and provides the theme data to the child widgets. This approach ensures that the theme is applied consistently throughout the entire app.
9. Navigator
In ICAPPS flutter template, navigation within the app is defined using routes, which are essentially mappings between a route name and a specific screen. These routes can be referenced within the MainNavigator
class, which is responsible for managing the app's overall navigation flow, including handling dialogs and animations.
The MainNavigator
class typically contains a MaterialApp
widget that defines the overall structure of the app, including the app's theme, title, initial route, and more. Within this widget, the routes
property is used to define the available routes in the app, and each route is mapped to a specific screen widget.
Once the routes are defined, their different methods for their navigation are defined too in the Navigator class after which they can be navigated to from anywhere within the app using the Navigator
class. The MainNavigator
class is typically accessed within the view models or screen classes and provides methods for navigating to a specific route, pushing new routes onto the stack, and popping routes off the stack.
10. Linting
Linting is a crucial aspect of any software development project, and the ICAPPS Flutter template is no exception. Linting involves analyzing code for potential errors, bugs, and stylistic inconsistencies to ensure that the code is of high quality and follows best practices. In the ICAPPS Flutter template, linting is implemented using the Flutter Lints package, which provides a set of rules and guidelines for writing clean, maintainable code. This package is integrated into the development workflow, and developers can run the linting tool to check their code before committing changes. By using linting in the ICAPPS Flutter template, developers can catch errors early and ensure that their code is consistent and easy to read, leading to a more efficient and effective development process.
11. Local Storage
In the ICAPPS Flutter template, two popular packages used for local storage are Shared Preferences and Drift.
a) shared_preference
Shared Preferences is a package that provides a simple key-value store for storing user preferences or other small amounts of data. It allows developers to store and retrieve data asynchronously and persistently across app launches. The package is easy to use and requires minimal setup, making it a popular choice for local storage in Flutter applications.
b) drift
Drifton the other hand, is a more robust and flexible solution for local storage. It provides a type-safe, object-oriented API for working with local data, and supports both SQL and NoSQL databases. Drift allows developers to define their data models and easily perform CRUD operations on local data. Additionally, it offers features like migrations, indexing, and data querying.
One of the primary differences between drift and the sqflite package is the approach to data management. While sqflite is a lower-level package that provides a SQLite implementation for Flutter, drift offers a higher-level abstraction layer that makes it easier to work with local data. Drift's fluent API allows developers to define data models and perform CRUD (create, read, update, delete) operations in a more concise and readable way.
Another difference is in the use of type-safe data management. Drift's type-safe API ensures that data is stored and retrieved in a way that conforms to the defined data model, reducing the risk of errors or data corruption. Sqflite, on the other hand, requires developers to write raw SQL queries and parse the results manually.
In summary: When deciding which package to use for local storage in the ICAPPS Flutter template, developers must consider the specific needs of their application. Shared Preferences is suitable for simple data storage and retrieval, while Drift is a more robust solution for complex data management. Ultimately, the choice between these two packages will depend on the requirements of the application and the preferences of the development team.
12. Tests
In the ICAPPS Flutter template, two popular packages used for writing tests are mockito and flutter_test.
a) mockito
Mockito is a mocking framework that allows developers to create mock objects and behavior for unit testing. It provides an easy way to create mock objects for dependencies and test the behavior of the system under test in isolation. Mockito is particularly useful when testing complex systems with many dependencies, as it allows developers to isolate and test individual components.
b) flutter_tests
Flutter_test, on the other hand, is a package provided by Flutter for writing widget tests and integration tests. It provides APIs for rendering widgets and interacting with them programmatically, allowing developers to test the UI behavior of their applications. Flutter_test also provides a way to test widget behavior in response to user interactions, making it useful for testing user flows.
When writing tests in the ICAPPS Flutter template, developers can use both Mockito and flutter_test to cover different aspects of their application. Mockito can be used to create and test the behavior of individual components in isolation, while flutter_test can be used to test the UI behavior and user flows. By combining these two packages, developers can ensure that their applications are thoroughly tested and free of bugs before release.
CONCLUSION
The ICAPPS Flutter template is a comprehensive and well-designed starting point for building Flutter applications. It provides a solid foundation for developing high-quality, production-ready applications, with a focus on scalability, maintainability, and performance. The template includes a variety of features and packages to accelerate development, such as dependency injection, local storage, localization support, retrofit, and integration with popular services like Firebase and GraphQL.
One of the strengths of the ICAPPS Flutter template is its emphasis on best practices and modern development techniques. The template follows the Flutter community's recommended architecture, using the Provider for state management. It also includes a robust testing framework and linting tools, enabling developers to ensure that their code is reliable and free of bugs.
Another key benefit of the ICAPPS Flutter template is its flexibility. The template provides a starting point that can be customized to fit a wide range of use cases and business needs. It is easy to add or remove features, adjust the UI design, and integrate with other libraries or services.
Overall, the ICAPPS Flutter template is a solid choice for developers looking to build high-quality, scalable Flutter applications. It provides a well-structured and modern architecture, a rich set of features and packages, and a flexible starting point for customization. With its focus on best practices and performance, the ICAPPS Flutter template is a great foundation for building reliable and feature-rich mobile applications.
Don't fail to Follow me here on Hashnode and On
Twitter @ JacksiroKe | Linked In Jack Siro | Github @ JacksiroKe
Subscribe to my newsletter
Read articles from Siro Daves directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
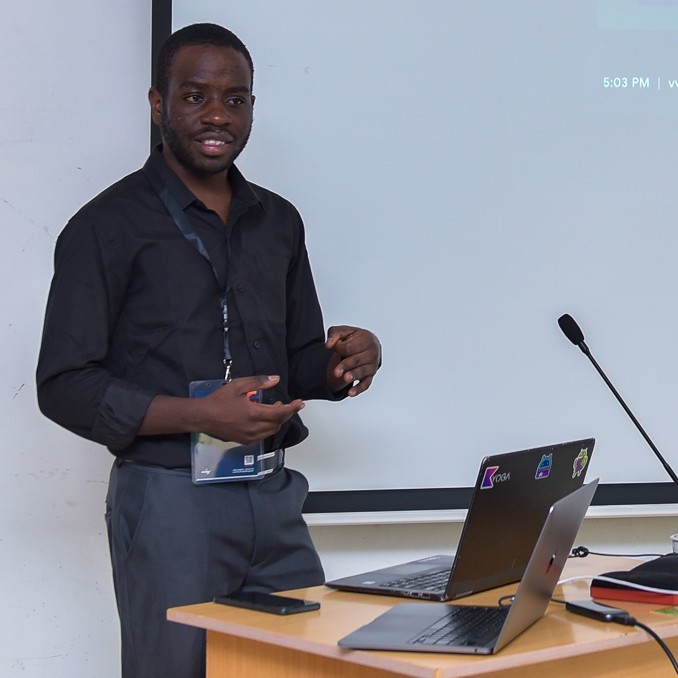
Siro Daves
Siro Daves
Software engineer and a Technical Writer, Best at Flutter mobile app development, full stack development with Mern. Other areas are like Android, Kotlin, .Net and Qt