Function Scope, Block Scope and Lexical Scope
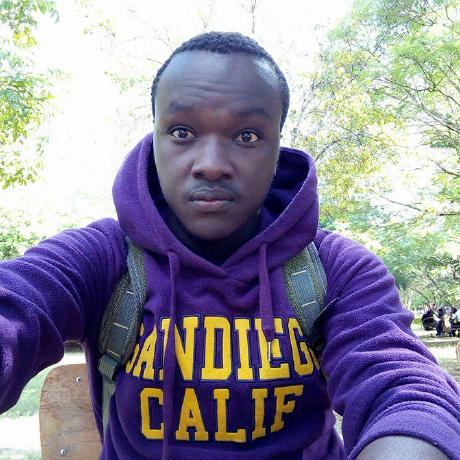
Table of contents
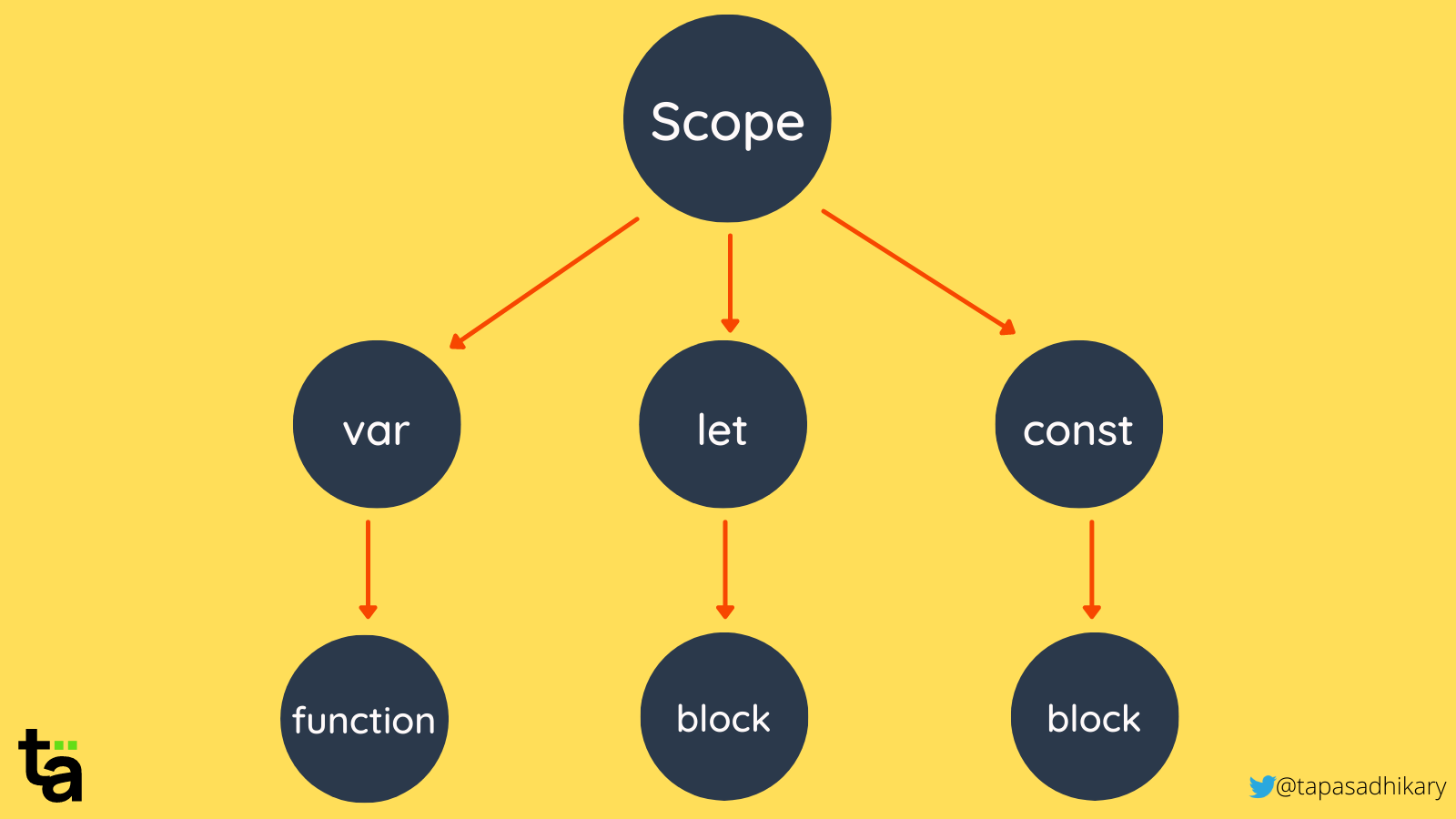
For the last 5 days, we have covered 5 topics in JS that are important for every developer to know. If you are new here you follow this link JS30IN30 to be able to read through the series.
Scope
Today we are going in-depth about the scope in Javascript programming.
In JavaScript, the term scope
refers to the set of variables, functions, and objects that are accessible in a particular context. The context can be a function, a block of code, or the entire program.
JavaScript has two types of scope: global scope and local scope.
Global Scope
Global scope refers to variables, functions, and objects that are available throughout the entire program. They are accessible from any part of the code, including within functions and other blocks.
Example:
// Global variable
var myGlobalVariable = "Hello, world!";
function myFunction() {
// Access global variable
console.log(myGlobalVariable);
}
// Call function
myFunction(); // Output: "Hello, world!"
// Access global variable from outside function
console.log(myGlobalVariable); // Output: "Hello, world!"
Local Scope
Local scope, on the other hand, refers to variables, functions, and objects that are only accessible within a specific block or function. They are not accessible outside of that context.
Local scope is comprised of two types; Function scope and block scope.
Function Scope
In JavaScript, variables defined with the "var" keyword have a function-level scope, meaning they are only accessible within the function they are defined in.
Example:
function myFunction() {
// Local variable
var myLocalVariable = "Hello, world!";
// Access local variable
console.log(myLocalVariable);
}
// Call function
myFunction(); // Output: "Hello, world!"
// Attempt to access local variable from outside function
console.log(myLocalVariable); // Output: ReferenceError: myLocalVariable is not defined
In this example, the variable myLocalVariable
is declared inside the myFunction
function, making it a local variable with function scope. It can only be accessed within the myFunction
function.
The myFunction
function logs the value of the local variable to the console using console.log(myLocalVariable);
.
After defining the function, we call it using myFunction()
, which logs the value of the local variable to the console.
Finally, we attempt to access the local variable from outside the function using console.log(myLocalVariable);
, which results in a ReferenceError
because the variable is not defined outside the function's scope.
Block Scope
Variables defined with the let
and const
keywords have a block-level scope, meaning they are only accessible within the block they are defined in.
Example:
function myFunction() {
// Local variable with block scope
if (true) {
let myLocalVariable = "Hello, world!";
// Access local variable
console.log(myLocalVariable);
}
// Attempt to access local variable from outside block
console.log(myLocalVariable); // Output: ReferenceError: myLocalVariable is not defined
}
// Call function
myFunction();
In this example, the variable myLocalVariable
is declared inside a block of code (an if
statement) using the let
keyword, which gives it block scope. It can only be accessed within the block where it is declared.
The myFunction
function logs the value of the local variable to the console using console.log(myLocalVariable);
.
After defining the function, we call it using myFunction()
, which logs the value of the local variable to the console if the condition is true.
Finally, we attempt to access the local variable from outside the block using console.log(myLocalVariable);
, which results in a ReferenceError
because the variable is not defined outside the block's scope.
Lexical Scope
The lexical scope can refer to both local and global scopes. In JavaScript, the scope of a variable or function is determined by its location within the code's nested hierarchy of functions. The variable or function can be defined in a local scope, which means it is only accessible within the function where it is defined, or it can be defined in a global scope, which means it is accessible from anywhere in the program.
For example; If a variable is defined outside of any function, it has a global scope and can be accessed from any function or block within the program. On the other hand, if a variable is defined within a function, it has local scope and is only accessible within that function.
Here's an example of a variable with global scope and a variable with local scope:
// Global variable with global scope
var globalVariable = "Hello, world!";
function myFunction() {
// Local variable with local scope
var localVariable = "Hello, there!";
console.log(globalVariable); // Output: "Hello, world!"
console.log(localVariable); // Output: "Hello, there!"
}
myFunction();
console.log(globalVariable); // Output: "Hello, world!"
console.log(localVariable); // Output: ReferenceError: localVariable is not defined
In this example, globalVariable
is defined outside of any function, giving it global scope. It can be accessed from within the myFunction
function as well as from outside the function.
On the other hand, localVariable
is defined within the myFunction
function, giving it local scope. It can only be accessed from within the function and not from outside. When we attempt to log localVariable
outside of the function, we get a ReferenceError
because it is not defined in that scope.
Conclusion
Understanding scope is important in JavaScript because it can help prevent naming collisions and improve code organization. By using a local scope, you can limit the accessibility of variables and functions, which can help prevent unintended side effects and make your code easier to understand and maintain.
Please leave a comment if there is anything left out. I may not cover everything but hope this helps.
Subscribe to my newsletter
Read articles from Kiprotich Dominic directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
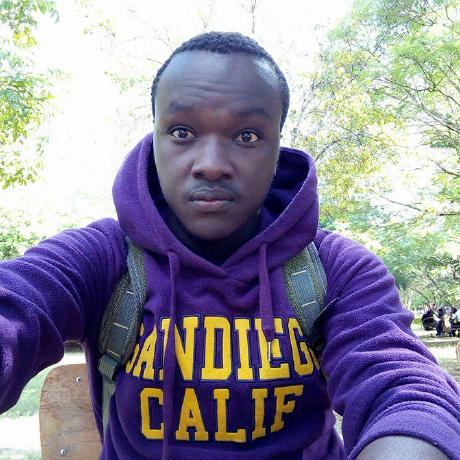