Format on Save - How to debug errors you didn't make
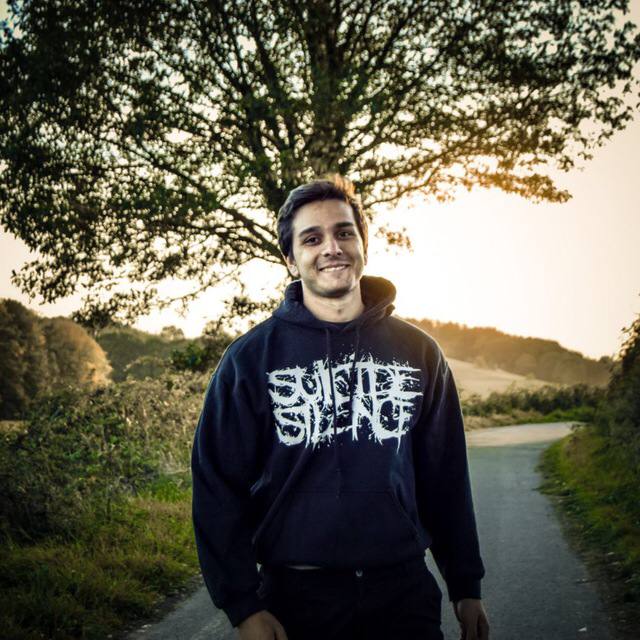
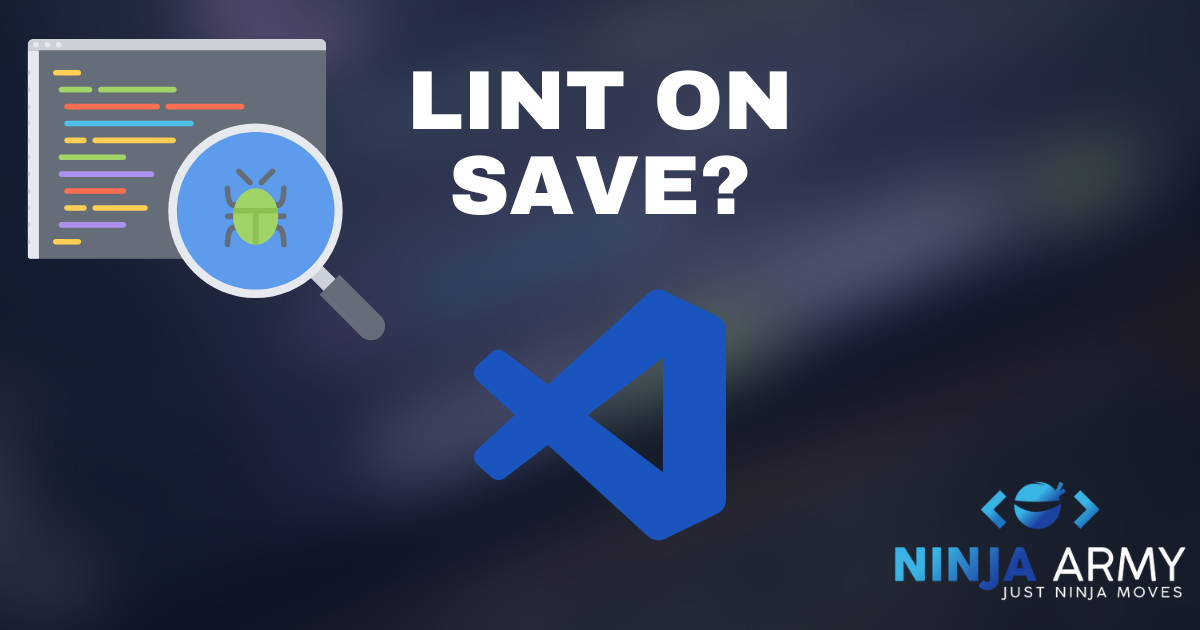
Format on save is one of your most liked features on VSCode? It's pretty cool you just hit save and all the formatting is done for you. You can write your code, however, the f*ck you want and VSCode is doing everything for you.
It's amazing but only if you configure it correctly! I did spend a couple of hours debugging because of this feature. And no surprise it all started with Shopware.
The Shopping Experiences
You already saw a couple of posts about Shopware from me right? A lot of them are about the CMS-Elements or the CMS-Blocks. In my opinion, creating those is a lot of fun. You can just create elements and build your shop with them. Of course, you have a configuration to add the content and settings you want.
The first bug
And there is also my first bug. I took a look at the official Shopware documentation. I have to 2 tabs but none of them is selected or shows content:
Let's take a look at the code which was formatted on save.
{% block sw_cms_el_ninja_cms_button %}
<sw-tabs class="sw-cms-el-config-ninja-cms-button__tabs" defaultitem="content">
<template slot-scope="{ active }">
{% block sw_cms_el_config_ninja_button_tab_content %}
<sw-tabs-item :title="$tc('sw-cms.elements.general.config.tab.content')" name="content" :activetab="active">
{{ $tc('sw-cms.elements.general.config.tab.content') }}
</sw-tabs-item>
{% endblock %}
{% block sw_cms_el_ninja_button_config_tab_options %}
<sw-tabs-item :title="$tc('sw-cms.elements.general.config.tab.settings')" name="settings" :activetab="active">
{{ $tc('sw-cms.elements.general.config.tab.settings') }}
</sw-tabs-item>
{% endblock %}
</template>
<template slot="content" slot-scope="{ active }">
{% block sw_cms_el_ninja_cms_button_config_content %}
<sw-container v-if="active === 'content'" class="sw-cms-el-config-ninja-button__tab-content">
<sw-text-field :label="$tc('sw-cms.elements.ninja-cms-button.config.label.buttonText')" :placeholder="$tc('sw-cms.elements.ninja-cms-button.config.placeholder.buttonText')" v-model="element.config.title.value" @element-update="onElementUpdate" :helptext="$tc('sw-cms.elements.ninja-cms-button.config.helpText.buttonText')"></sw-text-field>
<sw-colorpicker v-model="element.config.textColor.value" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.buttonTextColor')" coloroutput="hex" :zindex="1001" :alpha="true" :helptext="$tc('sw-cms.elements.ninja-cms-button.config.helpText.buttonTextColor')"></sw-colorpicker>
<sw-field v-model="element.config.url.value" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.buttonUrl')" :placeholder="$tc('sw-cms.elements.ninja-cms-button.config.placeholder.buttonUrl')" :helptext="$tc('sw-cms.elements.ninja-cms-button.config.helpText.buttonUrl')"></sw-field>
<sw-field v-model="element.config.newTab.value" type="switch" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.newTab')"></sw-field>
</sw-container>
{% endblock %}
{% block sw_cms_el_ninja_button_config_settings %}
<sw-container v-if="active === 'settings'" class="sw-cms-el-config-ninja-button__tab-settings">
{% block sw_cms_el_cms_ninja_button_config_settings_horizontal_align %}
<sw-select-field :label="$tc('sw-cms.elements.ninja-cms-button.config.label.hAlignment')" v-model="element.config.buttonAlign.value" :placeholder="$tc('sw-cms.elements.ninja-cms-button.config.placeholder.hAlignment')">
<option value="flex-start">left</option>
<option value="center">center</option>
<option value="flex-end">right</option>
</sw-select-field>
<sw-colorpicker v-model="element.config.buttonColor.value" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.buttonColor')" coloroutput="hex" :zindex="1001" :alpha="true" :helptext="$tc('sw-cms.elements.ninja-cms-button.config.helpText.buttonColor')"></sw-colorpicker>
<sw-field v-model="element.config.buttonWidth.value" type="number" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.width')" :placeholder="$tc('sw-cms.elements.ninja-cms-button.config.placeholder.width')">
<template #suffix>px</template>
</sw-field>
<sw-field v-model="element.config.buttonHeight.value" type="number" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.height')" :placeholder="$tc('sw-cms.elements.ninja-cms-button.config.placeholder.height')">
<template #suffix>px</template>
</sw-field>
{% endblock %}
</sw-container>
{% endblock %}
</template>
</sw-tabs>
{% endblock %}
We have our <sw-tabs>
element with a defaultitem
which is content. That looks pretty good to me. It should work.
Small typos can cause bugs
I was pretty new to Shopware when I faced this issue. I copied the code multiple times and tried to fix that bug. In the end, I was just confused why the code works on all elements but did not on mine.
After staring down the code for a couple of hours and trying very stupid things I noticed that defaultitem
actually has to be defaultItem
. It's case-sensitive and I copied it the correct way!
Fixing the bug
To fix that I had to disable the format on save feature from VSCode or configure it correctly. Of course, I just disabled the feature saved the file and activated that feature again because that sounds like a good idea doesn't it?
The fix was very easy. Just write defaultItem the correct way:
<sw-tabs class="sw-cms-el-config-ninja-cms-button__tabs" defaultItem="content">
And there you go. We have a defaultItem now and there is something selected from the first time you go into the configuration.
The second Bug
Of course, we are not done yet. Bugs rarely come alone right? We have a beautiful configuration now and luckily put in our content. Every field is working perfectly fine except for the color field. If you click on that nothing happens. So here we go again.
The <sw-colorpicker>
At that moment it was a very confusing bug. It was possible to add a hex code manually but there was no color picker appearing. So I went through the whole process again. Copied code from the documentation. I also took code from the GitHub Repository where the color picker worked!
The Bugfix
I spent a couple of hours on this bug as well. The sad thing is it was the same bug I had before. Just because I was lazy I lost so much time again. The solution here was to disable format on save or configure it correctly! Let's take a look at the code:
<sw-colorpicker v-model="element.config.buttonColor.value" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.buttonColor')" coloroutput="hex" :zindex="1001" :alpha="true" :helptext="$tc('sw-cms.elements.ninja-cms-button.config.helpText.buttonColor')"></sw-colorpicker>
You have to know that zindex
is case-sensitive and has to be zIndex
. Same for helptext
it has to be helpText
. So this time I fixed two bugs even if I only noticed one. Small letters make big changes. I simply changed the code to:
<sw-colorpicker v-model="element.config.buttonColor.value" :label="$tc('sw-cms.elements.ninja-cms-button.config.label.buttonColor')" coloroutput="hex" :zIndex="1001" :alpha="true" :helpText="$tc('sw-cms.elements.ninja-cms-button.config.helpText.buttonColor')"></sw-colorpicker>
I definitely lost too many hours just because of this. So never forget small letters have a huge impact. The final result looks like this:
Conclusion
Small letters can make your life hard. I lost a couple of hours twice because configuring a linter took too much time for me. Luckily there are a few easy solutions for this:
Don't use VSCode
Don't use a Linter
Don't use the Format on Save Feature
Configure your linter correctly!
I was pretty new to Shopware which made it even harder. I could have configured my linter. Most likely I would have the same bugs because at that time I had no clue that there are a few case-sensitive words.
Subscribe to my newsletter
Read articles from Joschi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
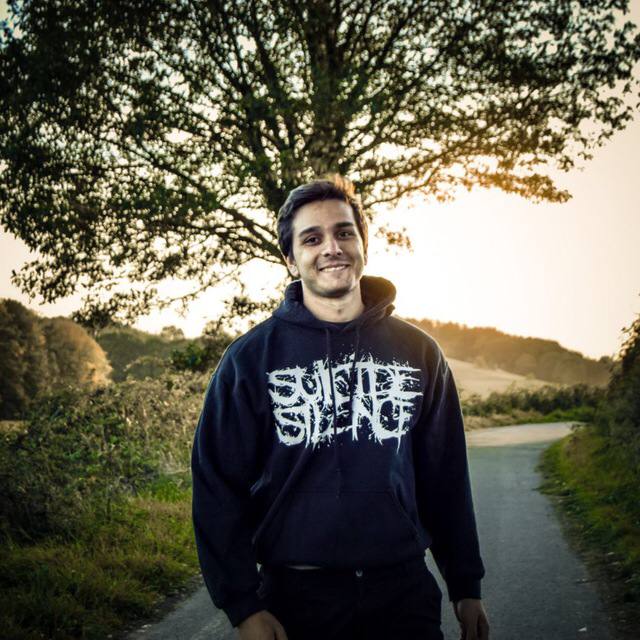