Getting started with ReactJS: A step-by-step tutorial for beginners
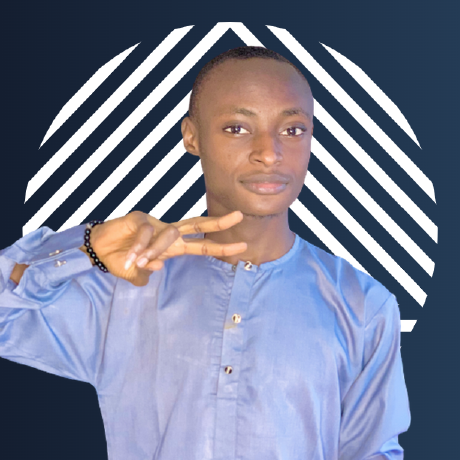
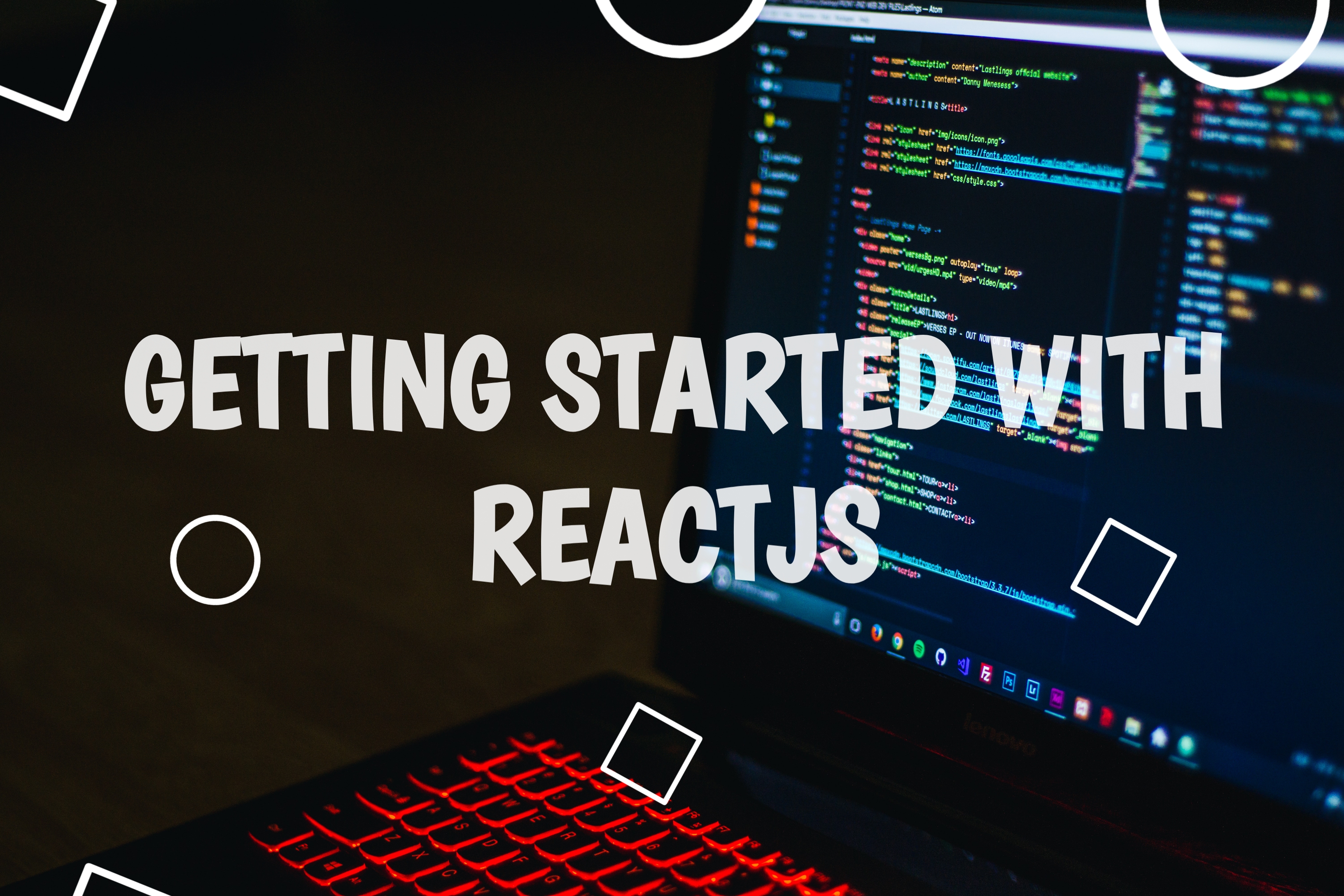
ReactJS is a powerful JavaScript library used for building dynamic and interactive user interfaces. It was created by Facebook and is currently maintained by Facebook and a community of developers. ReactJS has become one of the most popular JavaScript libraries and is widely used by developers worldwide. If you are new to ReactJS, this article will guide you on how to get started with ReactJS.
Prerequisites
Before you start with ReactJS, you need to have a basic understanding of HTML, CSS, and JavaScript. You should also have a good understanding of the concepts of Object-Oriented Programming (OOP). Familiarity with other JavaScript libraries such as jQuery will be an added advantage.
Installation
To use ReactJS, you need to install it first. ReactJS can be installed using Node Package Manager (NPM) or Yarn. If you don't have Node.js installed on your system, you need to install it first.
To install ReactJS using NPM, open your terminal and type the following command:
npm install react react-dom
To install ReactJS using Yarn, open your terminal and type the following command:
yarn add react react-dom
Creating a React Application
Once you have installed ReactJS, you can create a new React application using the Create React App tool. Create React App is a command-line tool that helps you set up a new React project quickly.
To create a new React application, open your terminal and type the following command:
npx create-react-app my-app
Replace my-app with the name of your application. This will create a new React application with the name my-app.
Understanding React Components
ReactJS is all about components. Components are the building blocks of a React application. A component is a JavaScript class or function that returns a piece of HTML (also known as JSX).
There are two types of React components:
Class Components: Class components are ES6 classes that extend the
React.Component
class. They have arender()
method that returns JSX.Function Components: Function components are JavaScript functions that return JSX.
Here's an example of a class component:
import React from 'react';
class App extends React.Component {
render() {
return <h1>Hello, World!</h1>;
}
}
export default App;
Here's an example of a function component:
import React from 'react';
function App() {
return <h1>Hello, World!</h1>;
}
export default App;
Rendering a Component
To render a React component, you need to create an instance of the component and pass it to the ReactDOM.render()
method. The ReactDOM.render()
method takes two parameters: the component to render and the DOM element to render it into.
Here's an example of rendering a component:
import React from 'react';
import ReactDOM from 'react-dom';
function App() {
return <h1>Hello, World!</h1>;
}
ReactDOM.render(<App />, document.getElementById('root'));
This will render the App component into the DOM element with the ID root.
Conclusion
ReactJS is a powerful library for building dynamic and interactive user interfaces. In this article, we have covered the basics of getting started with ReactJS. We have learned how to install ReactJS, create a new React application, understand React components, and render a component. With this knowledge, you can now start building your own React applications.
Subscribe to my newsletter
Read articles from Emmanuel Dalyop directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
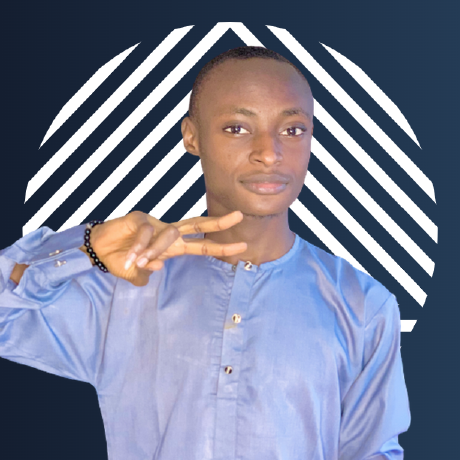
Emmanuel Dalyop
Emmanuel Dalyop
Hello, my name is Emmanuel and I am a software developer with over 2 years of experience. I have a passion for creating innovative and user-friendly applications that solve real-world problems. I am highly skilled in REACTJS,PYTHON,NODEJS,EXPRESSJS,MONGODB,HTML,CSS,JAVASCRIPT and have a deep understanding of software design and development best practices. I am always eager to learn new technologies and techniques to further improve my skills and deliver the best possible solutions for my clients.