Introduction to NumPy: A Beginner's Guide to Numerical Computing in Python
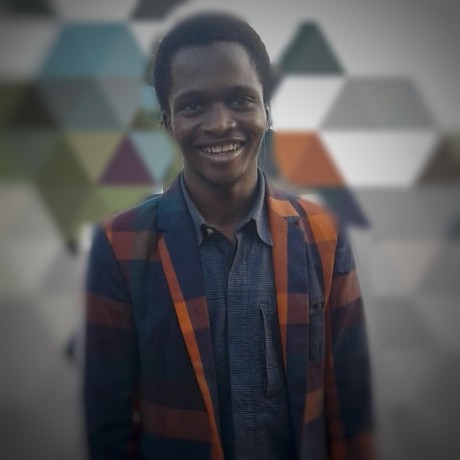
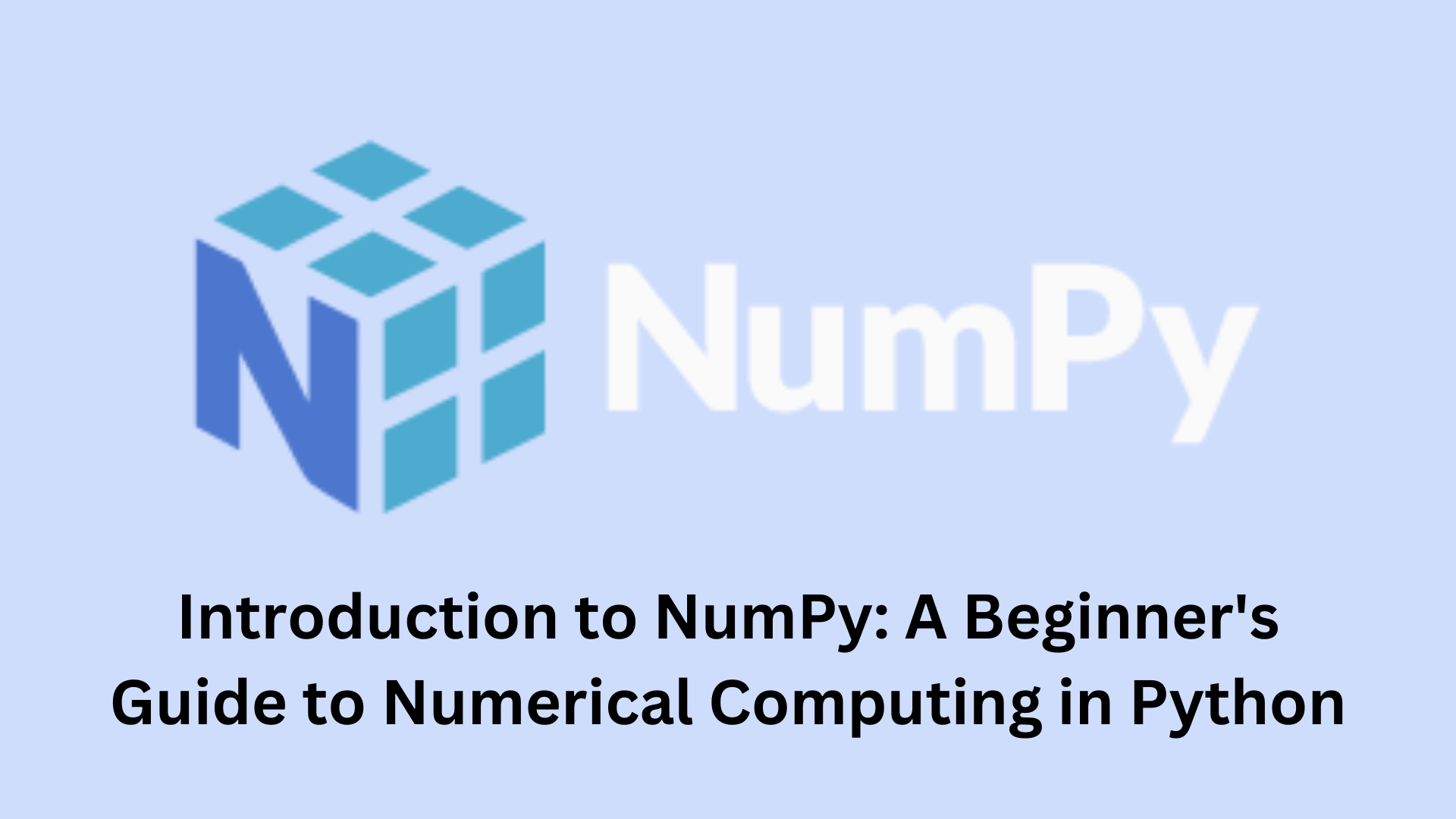
If you're interested in numerical computing or data analysis with Python, you'll need to learn about NumPy. NumPy is a powerful Python library that provides support for large, multi-dimensional arrays and matrices, along with a wide range of mathematical functions to operate on them.
In this article, we'll provide a beginner-friendly guide to NumPy, covering the basics of array creation, indexing, and arithmetic operations. We'll also dive into more advanced topics, including broadcasting and vectorization, and provide tips for writing efficient NumPy code.
Getting Started with NumPy
NumPy stands for "Numerical Python". It is a library that provides a multidimensional array object, various derived objects (such as masked arrays and matrices), and an assortment of routines for fast operations on arrays, including mathematical, logical, shape manipulation, sorting, selecting, I/O, discrete Fourier transforms, basic linear algebra, basic statistical operations, and much more.
Before we dive into the specifics of NumPy, let's start by installing the library and creating our first NumPy array.
To install NumPy, open a terminal or command prompt and run the following command:
pip install numpy
To use NumPy, you first need to import it into your Python script or interactive environment. You can do this by running the following code:
import numpy as np
This imports NumPy and assigns it the alias np
, which is a common convention in the scientific computing community.
Creating NumPy Arrays
The most important feature of NumPy is the ndarray
(n-dimensional array) data structure. This data structure allows you to represent and manipulate numerical data as arrays.
To create a NumPy array, you can use the np.array()
function. This function takes a list or tuple of values and converts it into a NumPy array. For example, the following code creates a NumPy array with the values [1, 2, 3, 4, 5]
:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
print(a)
Output:
[1 2 3 4 5]
We can also create multi-dimensional arrays using the same np.array()
function. For example, to create a two-dimensional array, we can use the following code:
import numpy as np
b = np.array([[1, 2, 3], [4, 5, 6]])
print(b)
Output:
[[1 2 3]
[4 5 6]]
NumPy also provides a number of functions for creating arrays with specific values. For example, the following code creates a 1D array of zeros with the np.zeros()
function:
import numpy as np
c = np.zeros(5)
print(c)
Output:
[0. 0. 0. 0. 0.]
You can also create arrays of ones, or arrays with a specific shape, using the np.ones()
and np.full()
functions, respectively. For example:
import numpy as np
d = np.ones((2, 3))
print(d)
Output:
[[1. 1. 1.]
[1. 1. 1.]]
import numpy as np
e = np.full((2, 3), 5)
print(e)
Output:
[[5 5 5]
[5 5 5]]
NumPy also provides functions for creating arrays with random values, such as np.random.rand()
and np.random.randint()
. These functions can be useful for generating test data or for simulating random events in your code.
Indexing and Slicing NumPy Arrays
Once you have created a NumPy array, you can access its values using indexing and slicing. Indexing allows you to access individual elements of an array, while slicing allows you to access a subset of elements.
To index a NumPy array, you can use square brackets and provide the index of the element you want to access. NumPy arrays are zero-indexed, which means the first element has an index of 0. For example, the following code accesses the first element of the array a
:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
print(a[0])
Output:
1
You can also use negative indexing to access elements from the end of the array. For example, the following code accesses the last element of the array a
:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
print(a[-1])
Output:
5
To access a specific element of a multi-dimensional NumPy array, you can use a comma-separated tuple of indices. For example, to access the element in the second row and third column of a 2D array, you can use the index [1, 2]
:
import numpy as np
b = np.array([[1, 2, 3], [4, 5, 6]])
print(b[1, 2])
Output:
6
You can also slice NumPy arrays using the same syntax as Python lists. For example, to slice the first three elements of a 1D NumPy array, you can use the slice [0:3]
:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
print(a[0:3])
Output:
[1 2 3]
Similarly, to slice the first two rows and first two columns of a 2D NumPy array, you can use the slice [:2, :2]
:
import numpy as np
b = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(b[:2, :2])
Output:
[[1 2]
[4 5]]
Basic Arithmetic Operations with NumPy
NumPy provides a number of functions for performing arithmetic operations on arrays. These operations are performed element-wise, which means that the same operation is applied to each element of the array.
For example, to add two NumPy arrays element-wise, you can use the +
operator:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([5, 4, 3, 2, 1])
c = a + b
print(c)
Output:
[6 6 6 6 6]
Similarly, to multiply two NumPy arrays element-wise, you can use the *
operator:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([5, 4, 3, 2, 1])
c = a * b
print(c)
Output:
[5 8 9 8 5]
You can also perform arithmetic operations on a single NumPy array. For example, to square all elements of a NumPy array, you can use the **
operator:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = a**2
print(b)
Output:
[ 1 4 9 16 25]
NumPy also provides a number of mathematical functions that can be applied to arrays element-wise, such as np.sin()
, np.cos()
, np.exp()
, and more.
Broadcasting in NumPy
Broadcasting is a powerful feature of NumPy that allows you to perform arithmetic operations between arrays of different shapes and sizes. The smaller array is broadcast across the larger array so that they have compatible shapes.
For example, let's say we want to add a constant value to each element of a 1D NumPy array. We can do this by creating a new array with the same shape as the original array, but filled with the constant value. Then, we can simply add the two arrays together:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([10, 10, 10, 10, 10])
c = a + b
print(c)
Output:
[11 12 13 14 15]
However, this approach can be inefficient for large arrays, since it requires creating a new array in memory. Instead, we can use broadcasting to achieve the same result more efficiently:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = 10
c = a + b
print(c)
Output:
[11 12 13 14 15]
In this example, the scalar value b
is broadcast across the entire a
array, so that the shapes of the two arrays are compatible for element-wise addition.
Advanced NumPy Techniques
Fancy Indexing
One of the most powerful features of NumPy is fancy indexing, which allows you to select subsets of an array using boolean masks, integer arrays, or other arrays of indices. For example, let's say we have an array of 10 numbers:
import numpy as np
a = np.arange(10)
We can use a boolean mask to select only the elements of the array that meet a certain condition, like this:
mask = a % 2 == 0
even_numbers = a[mask]
This will select only the even numbers from the array. We can also use an array of indices to select specific elements of the array, like this:
indices = np.array([1, 3, 5])
selected_numbers = a[indices]
This will select only the elements of the array with indices 1, 3, and 5.
Broadcasting with Strides
Another powerful feature of NumPy is broadcasting, which allows you to perform arithmetic operations between arrays of different shapes and sizes. Broadcasting is based on the idea of "strides", which are the number of bytes that must be skipped in memory to move from one element of an array to the next.
For example, let's say we have two arrays:
a = np.array([1, 2, 3])
b = np.array([10])
We can add these two arrays together using broadcasting, like this:
c = a + b
NumPy will automatically "broadcast" the value in the b
array across the a
array, so that the shapes of the two arrays are compatible for element-wise addition. The result is an array of the same shape as a
, with each element increased by 10.
Advanced Array Manipulation
NumPy provides a number of advanced functions for manipulating arrays, including sorting, searching, reshaping, and more. For example, we can use the sort()
method to sort the elements of an array:
a = np.array([3, 1, 4, 2, 5])
a.sort()
This will sort the elements of the array in ascending order, so that a
is now [1, 2, 3, 4, 5]
.
We can also use the reshape()
method to change the shape of an array:
a = np.arange(6)
b = a.reshape((2, 3))
This will reshape the 1D array a
into a 2D array with shape (2, 3)
.
Conclusion
NumPy is a powerful library for performing numerical operations in Python. It provides a fast, efficient, and easy-to-use interface for working with arrays of data, and includes a wide range of functions for performing common mathematical operations.
In this article, we covered the basics of working with NumPy arrays, including creating arrays, indexing and slicing arrays, performing basic arithmetic operations, and using broadcasting. With this knowledge, you should be well-equipped to start using NumPy in your own projects, and to explore its many advanced features and capabilities.
Subscribe to my newsletter
Read articles from Agbeniga Agboola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
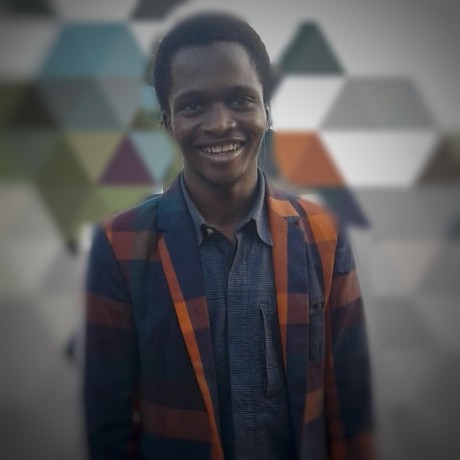