JavaScript Arrays: An introduction for beginners
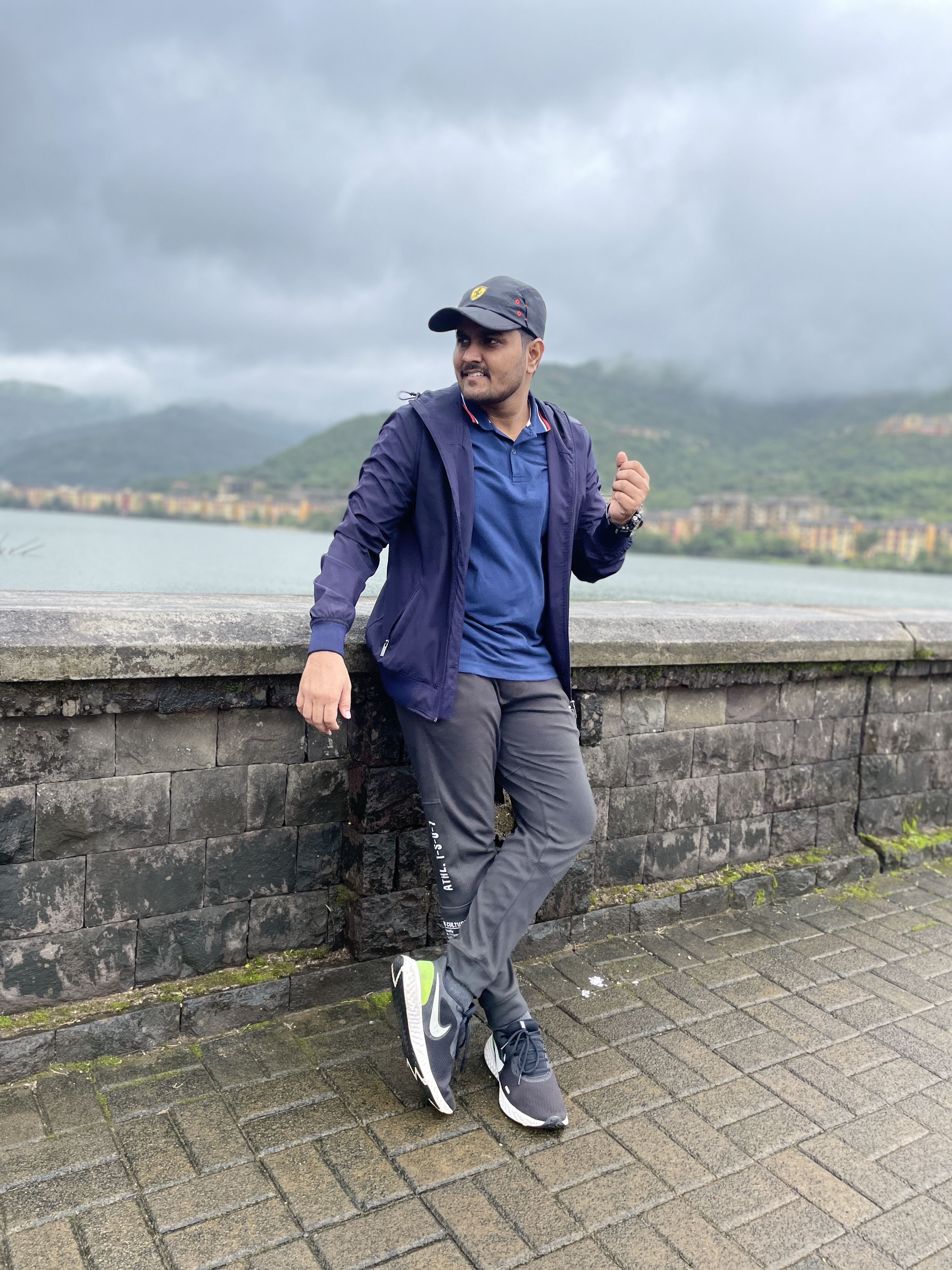
Introduction
Hello Everyone, My Name is Akshay and I am going to share a little bit of my knowledge about javascript arrays which I got from many sources in this vast sea of knowledge.
So what is an array - An array is a collection of different items at a contiguous memory location. In Javascript, Arrays are used to store multiple values in single variables. this multiple values can be on different datatype, each item in the array has a specific number through which we can access it it is called an Index Number.
Array plays important role in JavaScript (umm actually its very very important in every programming language😂)
Declaration of array
To get the array in our program we have to declare it..!
To declare the array we should know the syntax of the Array in JavaScript.
So the Syntax of the array in Javascript is as below
var arrayname=[value1,value2.....valueN];
There are two methods for declaration of array.
//Method-1 --> Preferred
let arr1 = [ "value1", "value2", "value3"...... ];
//Method-2 --> Not Preferred
let arr2 = new Array( "value1", "value2", "value3"...... );
Array Method and its Properties.
array.length()
The length properties tell us about the number of elements in an array.
let arr1 = ["Akshay", "Aditi", "Darshan", "Karan", "Aditya"]
console.log(arr1.length); // 5 i.e arr1 has five elements
Index -
In programming Index starts from 0 not from 1.with the help of index we can target a specific element in an array.
let arr1 = ["Akshay", "Aditi", "Darshan", "Karan", "Aditya"]
console.log(arr1.length); // 5 i.e arr1 has five elements
So for this above code Akshay is at 0 index and Aditya is at 4 index.
now think what is the index of Darshan..!
yeah you are right its 2.
Now we are familiar with the index, I think it's the perfect time to understand that what is mutability.
If we use typeof operator on the array we find that array is an object type i.e. non-primitive data type and non-primitive data types (objects, Arrays) are mutable in JavaScript and the primitive data types ( Number, String, Boolean etc.) are immutable.
But wait, What is Mutability?
Mutability means that a Mutable value or data can be modified or changed without creating a new value.
Let's understand this with an example..!
// We have this array fruits which contains 4 elements
let fruits = ["apple", "bada apple", "chhota apple", "double apple"];
console.log(fruits)
// ["apple", "bada apple", "chhota apple", "double apple"]
//Now mutability means that we can change or modifiy content of this array
fruits[1] = "BADA APPLE";
console.log(fruits)
//[ 'apple', 'BADA APPLE', 'chhota apple', 'double apple']
//Here you can see that 2nd element (at position index 1 ) has changed in the original array.But we can't do this with primitive data types.
let str = "Akshay";
console.log(str[0]) // "A"
str[0] = "H";
console.log(str[0]) //"S"
console.log(str) //"Akshay"
//Here you can see that first character of string str has not changed Because String is immutable
One more thing does not confuse mutability with an assignment to a variable. They both are completely different things.
let str = "Savinder";
str = "Ravinder";
//See here we are not changing or modifying the value or string "Savinder". We are creating a new value "Ravinder" which will now be inside container (Variable) str. It is not mutability because a new value "Ravinder" is created. We haven't modified anything in the "Savinder".
Adding and removing elements in an array.
array. pop()
The pop()
method removes the last element from an array and returns that element. This method changes the length of the array. it updates the array.
Syntax
arrayname.pop()
Example
const plants = ['broccoli', 'cauliflower', 'cabbage', 'kale', 'tomato'];
console.log(plants.pop());
// Expected output: "tomato"
console.log(plants);
// Expected output: Array ["broccoli", "cauliflower", "cabbage", "kale"]
plants.pop();
console.log(plants);
// Expected output: Array ["broccoli", "cauliflower", "cabbage"]
array.push()
The push()
method adds one or more elements to the end of an array and returns the new length of the array.
const animals = ['pigs', 'goats', 'sheep'];
const count = animals.push('cows');
console.log(count);
// Expected output: 4
console.log(animals);
// Expected output: Array ["pigs", "goats", "sheep", "cows"]
animals.push('chickens', 'cats', 'dogs');
console.log(animals);
// Expected output: Array ["pigs", "goats", "sheep", "cows", "chickens", "cats", "dogs"]ssss
array.shift()
The shift()
method removes the first element from an array and returns that removed element. This method changes the length of the array.
const array1 = [1, 2, 3];
array1.shift();
console.log(array1);
// Expected output: Array [2, 3]
array.unshift()
It will add the given item at the start of an array. It also modifies the original array.
let arr = [1,2,3,4,5,6,7]
console.log(arr) // [1,2,3,4,5,6,7]
arr.unshift(0);
console.log(arr) // [0,1,2,3,4,5,6,7
Convert Array to a string.
array.toString()
It will convert the given array into a string then it will return the given array but it will not modify the given array.
let arra = [11, 22, 33, 44, 55, 66];
console.log(arra); //[11, 22, 33, 44, 55, 66]
let str1 = arra.toString();
console.log(str1) //11,22,33,44,55,66
console.log(typeof str1); //string
console.log(arra) //[11, 22, 33, 44, 55, 66]
array.join()
The join method creates and returns a new string by concatenating all of the elements in an array.
const elements = ['Fire', 'Air', 'Water'];
console.log(elements.join());
// Expected output: "Fire,Air,Water"
console.log(elements.join(''));
// Expected output: "FireAirWater"
console.log(elements.join('-'));
// Expected output: "Fire-Air-Water
array.concat()
This method merges two different arrays and it gives output as a new array.it will not modify the previous array.
let arr = [1,2,3,4,5,6,7];
console.log(arr) // [1,2,3,4,5,6,7]
let arr2 = [8,9,10,11,12,13]
let arr3 = [14,15,16,17,18,19,20]
let arr4 = arr.concat(arr2,arr3);
console.log(arr4) // [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20]
console.log(arr) //[1,2,3,4,5,6,7] (original arr is not modified)
array.reverse()
It will reverse the given array in position and it update the original array
let arr = [1,2,3,4,5,6,7];
console.log(arr) // [1,2,3,4,5,6,7]
arr.reverse();
console.log(arr) // [7,6,5,4,3,2,1]
array.slice()
It slices out a piece from an array. It does not modify the original array but returns a new array.
let arr = [1,2,3,4,5,6,7];
console.log(arr) // [1,2,3,4,5,6,7]
console.log(arr.slice(2)) // [3,4,5,6,7] from index 2 to last element of array.
console.log(arr.slice(1,6))// [2,3,4,5,6] from index 1 to 5 (6-1)
console.log(arr) //[1,2,3,4,5,6,7]
array.splice()
Splice() can be used to add items in an array at a given particular position. It can also delete elements. It returns the deleted items and modifies the original array.
let arr = [1,2,3,4,5,6,7];
console.log(arr) // [1,2,3,4,5,6,7]
console.log(arr.splice(2,3,"Ram","Shyam","Mohan")) //[3,4,5], return deleted items
console.log(arr) //[ 1, 2, 'Ram', 'Shyam', 'Mohan', 6, 7 ] modifies original array
array.sort()
If we are using the sort() method on an array. It will first convert each element of the array into a string then comparison happens based on their UTF-16 code and the order of comparison is ascending.
let arr = ["Akshay", "Karan", "Darshan"];
console.log(arr.sort()) //
//Output
//[ 'Akshay', 'Darshan', 'Karan' ]
delete
delete is not an array method but it is an operator which is used to delete array elements.
let arr = [1,2,3,4,5,6,7]
delete arr[3];
console.log(arr) // [ 1, 2, 3, <1 empty item>, 5, 6, 7 ]
Note - Delete operator will delete the element but it will not change the original length of array.
array.fill()
It replaces elements of an array with the given value, from index 0 to end index (array. length). It also modifies the original array and also returns the modified array.
let arr = [1,2,3,4,5,6,7]
console.log(arr.fill("FSJS")) //['FSJS','FSJS','FSJS','FSJS','FSJS','FSJS','FSJS']
console.log(arr)
//['FSJS','FSJS','FSJS','FSJS','FSJS','FSJS','FSJS']
//for specifix index
let arr1 = [1,2,3,4,5,6,7]
//from index 1 till last index
console.log(arr1.fill("FSJS",1));
// [1,'FSJS','FSJS', 'FSJS','FSJS', 'FSJS','FSJS']
//For specific A and Z index
let arr2 = [1,2,3,4,5,6,7];
//from index 1 till index 4 (5-1)
console.log(arr2.fill("FSJS",1,5));
//[1,'FSJS','FSJS', 'FSJS','FSJS', 6,7]
indexOf()
It returns the index of the first occurrence of that element. it returns -1 if element is not in array.
let arr = ["Akshay", "Karan", "Darshan"];
console.log(arr.indexOf("Darshan"));// 2
lastIndexOf()
It returns the index of the last occurrence of that element. It returns -1 if the element is not present in the array.
let arr = ["Akshay", "Karan", "Darshan", "Karan"];
console.log(arr.indexOf("Karan"));// 3
split()
This is a string method. It will take a pattern as an argument and based on that pattern it will convert the string into a substring and put these substrings inside the array and returns that array.
let firstName = "Akshay";
let arr1 = firstName.split("");
console.log(arr1); // ['A', 'k', 's','h', 'a', 'y']
let txt = "This FSJS 2.0 Bootcamp by Hitesh Sir & iNeuron is Awesome";
let arr2 = txt.split(" ");
console.log(arr2)
// ['This','FSJS','2.0','Bootcamp','by','Hitesh','Sir', '&','iNeuron', 'is','Awesome']
Array.isArray()
It tells whether the passed value is an array or not. It returns a boolean value that is either true or false.
let firstName = "Akshay";
console.log(Array.isArray(firstName)) //false
if(Array.isArray(firstName)){
console.log(`${firstName} is an array`)
}else{
console.log(`${firstName} is not an array`)
}
// Akshay is not an array
let lastName = ["Fasale"];
console.log(Array.isArray(lastName)) //true
if(Array.isArray(lastName)){
console.log(`${lastName} is an array`)
}else{
console.log(`${lastName} is not an array`)
}
// Fasale is an array
array.includes()
It takes two arguments first is the element that needs to search in the array & second is the index from where till the last index it will search that element in the array. It returns a boolean-type value if the element is present the return is true, else false. If the second argument i.e. indexFrom is missing then it will search the whole array.
let languages = [ "python", "Ruby", "javascript", "kotlin", "PHP", "java" ];
// Search the whole array
console.log(languages.includes("javascript")) // true
// search from index 1 till last index
console.log(languages.includes("javascript",1)); // true
// search from index 2 till last index
console.log(languages.includes("javascript",2)); //true
// search from index 3 till last index
console.log(languages.includes("javascript",3)); //false
// search from index 4 till last index
console.log(languages.includes("javascript",4)); //false
Array.from()
Array. from() converts an array-like object (which is iterable and uses index & length properties e.g. Map, Set, HTML Collection) to an array. It returns a new array. If the object is not iterable then also it converts into an array. The first argument is an array-like object & second is a callback.
let num = "123456789"
// Although here num is string but is also be any array like iterable object.
let arr = Array.from(num,(element)=>{
return element*element;
})
console.log(num) //123456789
console.log(arr) // [1, 4, 9, 16, 25, 36, 49, 64, 81]
array.map()
It creates a new array. It takes a callback function as an argument and the function to execute for each element in the array. Its return value is added as a single element in the new array.
let arr = [1,2,3,4,5]
let arr2 = arr.map(element=>{
return element*element*element;
})
console.log(arr2) // [ 1, 8, 27, 64, 125 ]
for of loop
Well instead of a traditional for loop we can use for-of-loop on an array for simplicity. The for-of-loop iterate on each array element.
let arr = [1,2,3,4,5]
for(let item of arr){
console.log(`The square of ${item} is ${item*item}`)
}
//The square of 1 is 1
//The square of 2 is 4
//The square of 3 is 9
//The square of 4 is 16
//The square of 5 is 25
Subscribe to my newsletter
Read articles from Akshay Fasale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
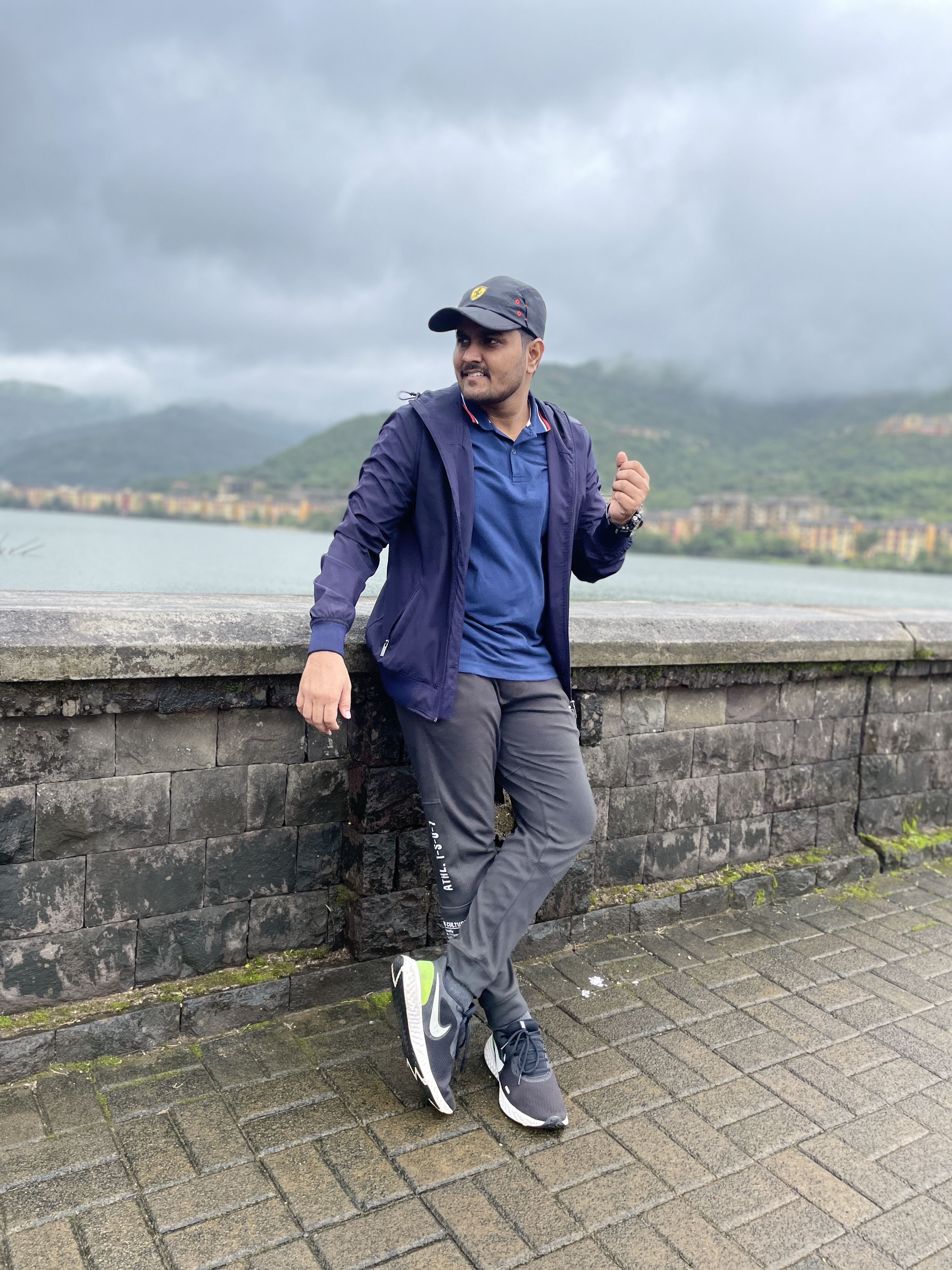