Functional Programming: Currying And Partial Application
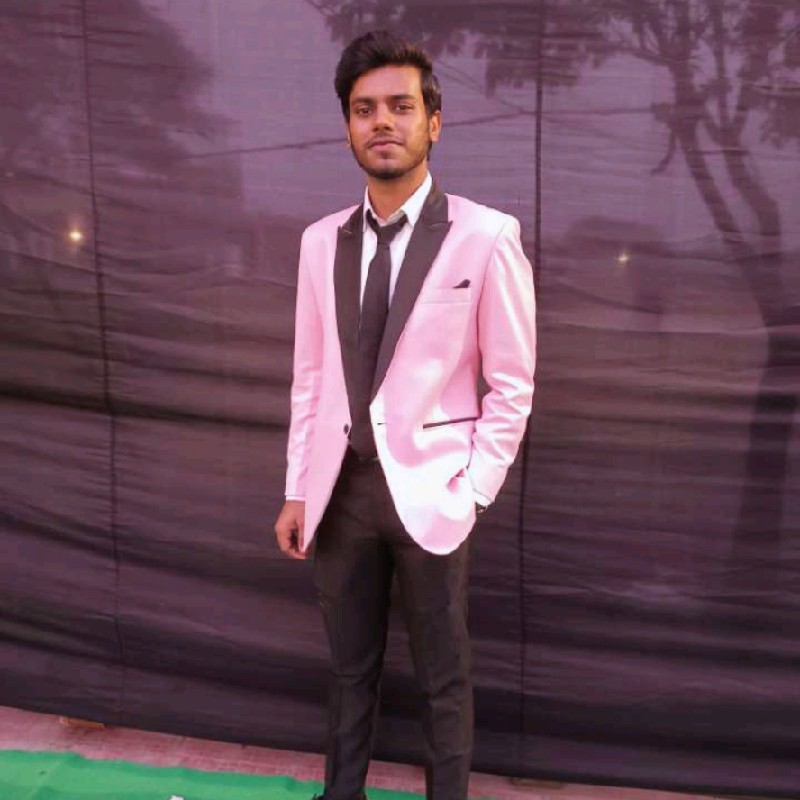
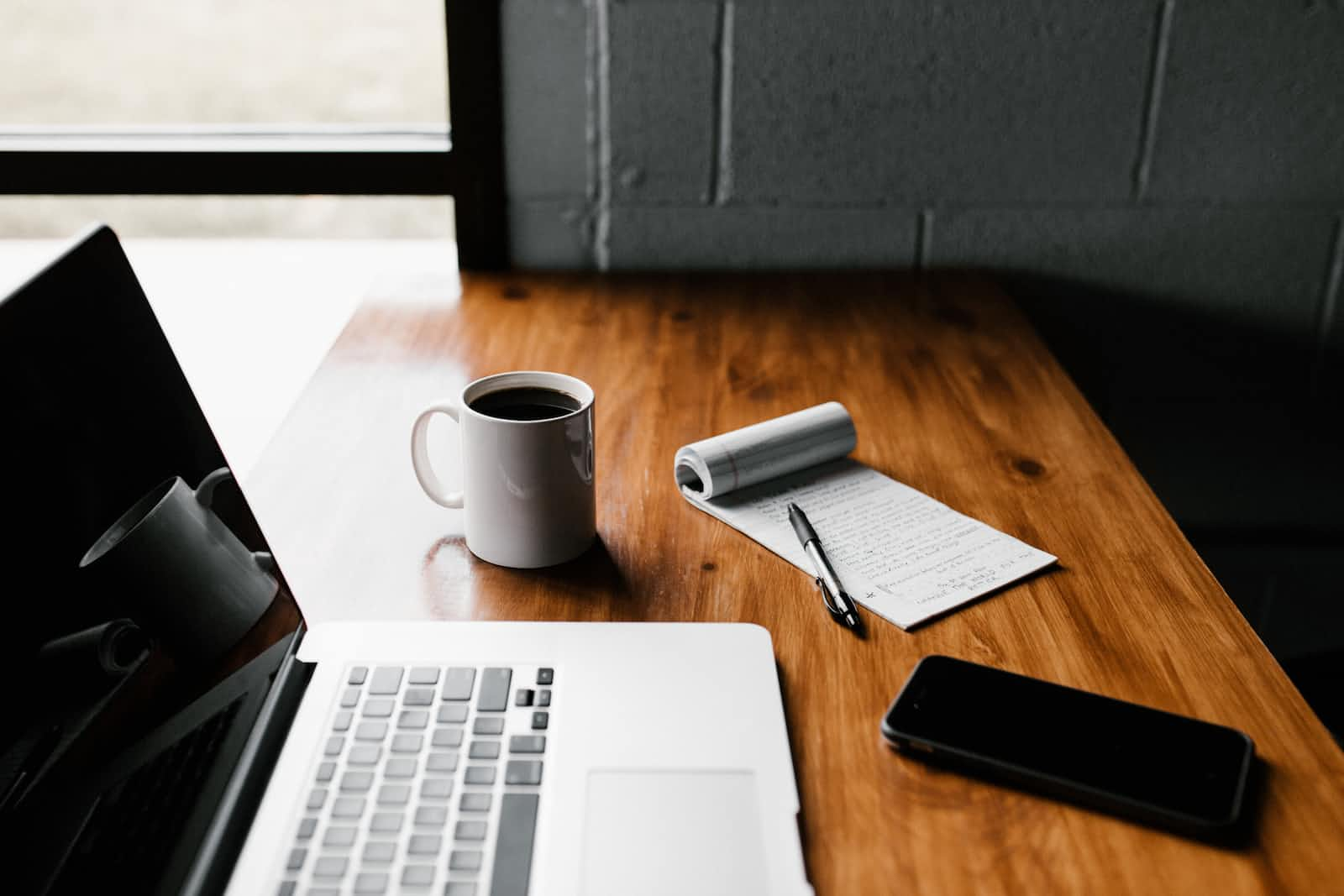
What is Currying?
Currying is a technique in functional programming to transform a function with multiple arguments into a series of function calls with each having only a single argument. Basically, it converts a function call like this f(a, b) to this f(a)(b).
Why are the use cases of Currying?
It helps you to avoid passing the same variable again and again.
It helps you to make function pure.
It helps you to create a higher-order function.
How to make a function Curry?
In JavaScript, currying is possible because of closures. Curry functions are constructed by chaining closures and by defining and returning their inner function simultaneously. I know this hardly makes any sense at first😅, but don't worry you will understand it properly with time.
Let's understand it with an example👇
-->CREATE A FUNCTION TO RETURN THE SUM OF THREE NUMBERS.
Standard Function
function sum(a,b,c){
return a+b+c;
}
const result = sum(1,2,3);
console.log(result); // 6
Curry Function
function sum(a){
return function(b){
return function(c){
return a+b+c;
}
}
}
const result = sum(1)(2)(3);
console.log(result) //6
So now using currying you can make pure functions and also avoid passing the same arguments again and again, for example, 👇
function multiply(a){
return function(b){
return a*b;
}
}
const multiplyBy3 = multiply(3);
console.log(multiplyBy3(2)); // 6
console.log(multiplyBy3(5)); // 15
const multiplyBy5 = multiply(5);
console.log(multiplyBy5(2)); // 10
console.log(multiplyBy5(4)); // 20
Infinite Currying
Infinite currying is when A curry function can take infinite arguments.
e.g. sum(1)(2)(3)......(n);
We achieve this through recursive function calls;
->We return a function until arguments are provided
->If there are no arguments specified anymore, we just return 'a' which contains the total.
function sum(a){
return function(b){
if(b){
return sum(a+b);
}
else{
return a;
}
}
}
console.log(sum(1)(2)(3)()); // 6
console.log(sum(2)(5)(5)(56)(4)()); // 72
Partial Application
Partial Application is a technique in functional programming to make your javascript code more readable and maintainable. The partial application starts with a function. We take this function and create a new one with one or more of its arguments already “set” or partially applied. This sounds odd, but it will reduce the number of parameters needed for our functions. -[freeCodeCamp]
function calculation(operation){
return function(a, b){
switch(operation){
case "add":
return a+b;
case "multiply":
return a*b;
case "divide":
return a/b;
case "substract":
return a-b;
default:
return "Invalid Operation";
}
}
}
//Here as you can see that we are setting one argument (operation) already before calling it with another one or more arguments;
const add = calculation("add");
const divide = calculation("divide");
console.log(add(3, 2));
console.log(divide(10, 5));
THANKS FOR READING!❤
If you have any queries related to web development you can ask me in the comment section.
About Me
Subscribe to my newsletter
Read articles from Akash Deep Chitransh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
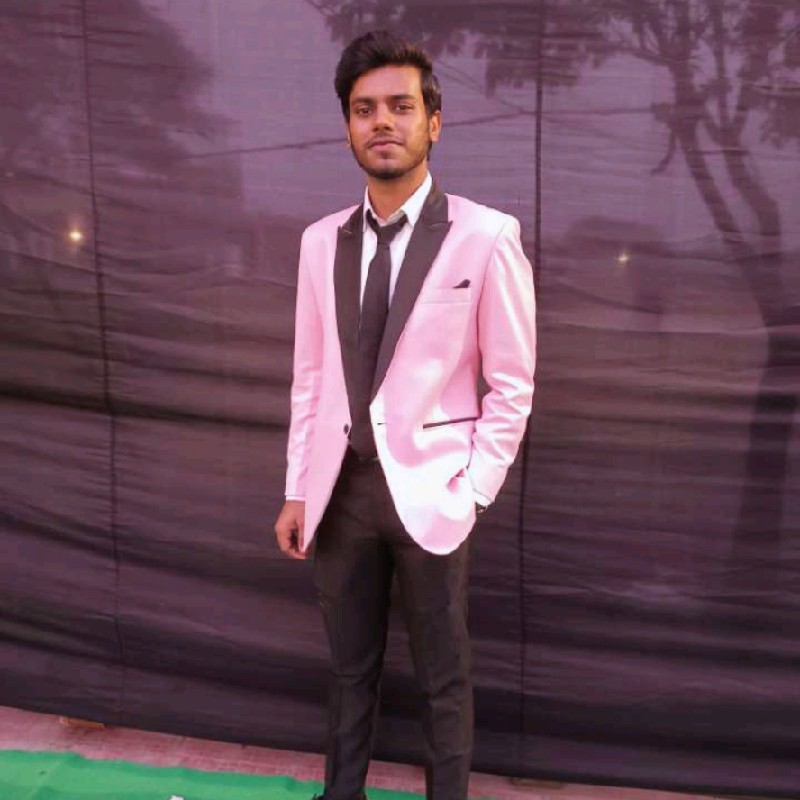
Akash Deep Chitransh
Akash Deep Chitransh
I am a front-end developer, currently working at TCS.