How to extract parameter value from query string in React.js
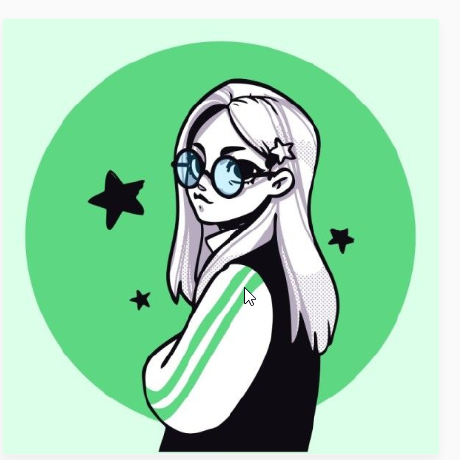
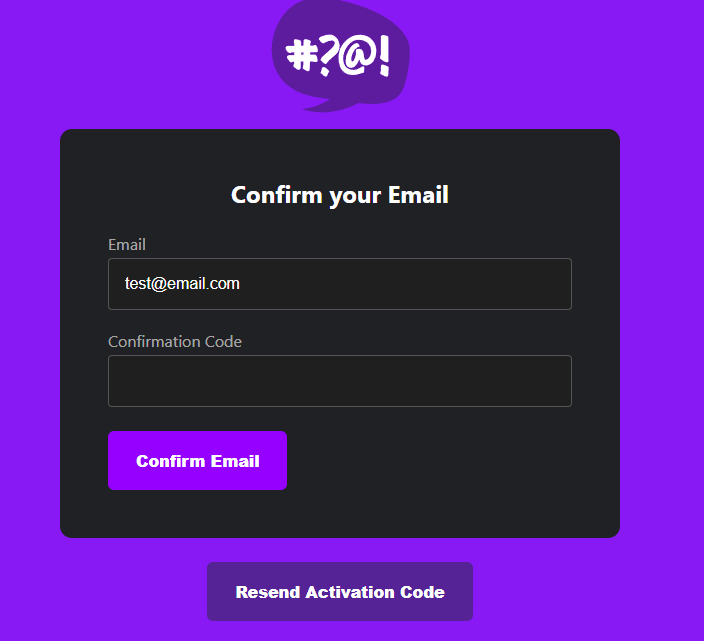
This article is supplemental material for Andrew's Brown AWS Free Cloud Bootcamp for Week 3-Decentralized Authentication.
In the Cruddur app, when a new user signs up, the user is directed to a confirmation page. The URL for this confirmation page already has the user's email address.
The current UI required the user to type their email address and the confirmation code they received.
An improved user experience would be for the Email field to be auto-populated. This can be achieved by extracting the email parameter from the url before rendering the page.
Introducing ReactRouter
React Router is a lightweight library that allows you to manage and handle client-side routing. Hooks allow us to "hook" into React features such as state and lifecycle methods. React Router ships with a few hooks that let you access the state of the router and perform navigation from inside your components.
The useSearchParams
Hook
If you’re working with React, React Router makes the consuming state in the URL, particularly in the form of a query string or search parameters, straightforward. It achieves this with the useSearchParams
Hook:
import { useSearchParams } from "react-router-dom";
const [searchParams, setSearchParams] = useSearchParams();
const message= searchParams.get('messsage');
// ...
setSearchParams({ 'message': 'HelloWorld' }); // will set URL like so https://our-app.com?message=HelloWorld - this value will feed through to anything driven by the URL
Applying Concept to Cruddur
The following changes were introduced to the ConfirmationPage.js
First, import and define the hook
import { useSearchParams } from "react-router-dom"; .. .. .. .. const [searchParams, setSearchParams] = useSearchParams();
Next, extract the parameter value by replacing the following block of code
React.useEffect(()=>{ if (params.email) { setEmail(params.email) } }, [])
with below code
React.useEffect(()=>{ const paramemail = searchParams.get('email'); console.log(paramemail) if (paramemail) { setEmail(paramemail) } }, [])
Hooray!
Now when you hit the url you will see that the email parameter is extracted and prepopulated into the Email field.
Subscribe to my newsletter
Read articles from Supercali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
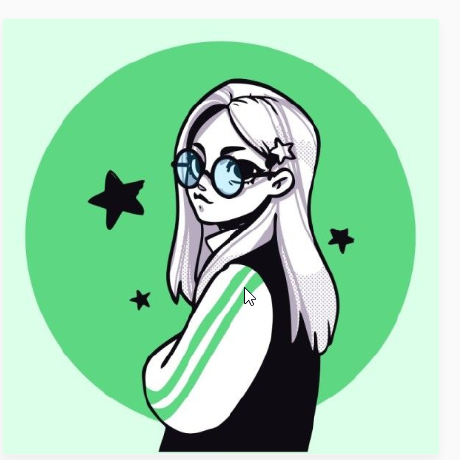