React-Admin #1 - Introduction to building well-managed admin side sites
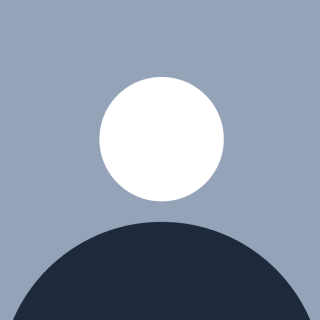
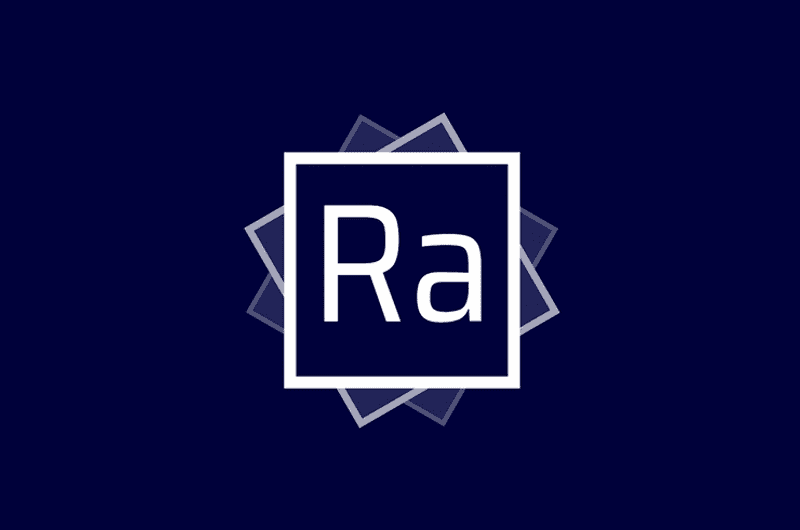
What is React-admin?
A frontend framework that utilizes React, Material-UI, React Router, etc. which facilitates us in building and customizing our admin side needs effortlessly.
Why do we need React-admin?
Most dynamic websites which involve frequent changes in content, are a type of blog/forum, are something like an online store, have multiple people updating the website without knowing much coding, etc require an admin side. Admin-side websites are meant to be simple and not crowded with complex design patterns. They just have to be straightforward: adding, viewing, editing, and deleting are the primary motives.
And React-admin gives you the same seamless experience with lesser lines of code compared to building from scratch with only React.
What are the Key Features of React Admin?
Resource-based routing: React Admin uses resources (e.g. posts, comments, users) for routing. This maps nicely to typical admin interfaces.
List view: For displaying a list of records. Supports filters, sorters, pagination, etc.
Create/Edit forms: For creating/editing individual records. Supports input validation.
Show view: For displaying a single record.
Delete button: For deleting a record.
Search: For searching records by keywords.
Permissions: For restricting access to resources based on roles.
Theming: For customizing the look and feel.
Translation: For supporting multiple languages.
Overall, React Admin covers pretty much everything you need to build a full-featured admin dashboard. The variety of built-in components allows you to develop admin interfaces quickly without re-inventing the wheel. You can simply compose these components to build custom admin panels tailored to your needs.
How do we configure a React-admin app?
Initial procedures for setting up the react-admin app include creating an <Admin> component and wrapping the whole application with the same. It requires at least one child <Resource> and a dataProvider prop. In most cases, custom data providers are created based on the requirements or you could try some REST providers like simpleRestProvider. For setting the routes of the application <CustomRoutes> are used which are not mandatory but will definitely be in use when building apps.
import * as React from "react";
import { Admin, Resource, CustomRoutes } from 'react-admin';
import customDataProvider from './providers';
import { Users } from './users';
import {Profile} from './profile';
const App = () => (
<Admin dataProvider={customDataProvider}>
<CustomRoutes>
<Route path="/profile" element={<Profile />} />
</CustomRoutes>
<Resource name="users" {...Users}/>
</Admin>
);
export default App;
The other major props of <Admin> include authProvider, title, layout, theme, store, etc. More on this here.
Data Providers
For every application, there needs to be data fetching and submitting. This is facilitated by data providers in React-admin. There are built-in methods of data provider that does their work respective to the API calls. These methods include:
getList
- gets a list of records (used in editing and showing)getOne
- gets a single record based on the id providedgetMany
- gets a list of records based on the array of ids providedgetManyReference
- gets the record related to another recordcreate
- create a recordupdate
- editing a record based on the id providedupdateMany
- editing multiple records based on an array of idsdelete
- deleting a recorddeleteMany
- deleting a list of records
const customDataProvider = {
getList: (resource, params) => Promise,
getOne: (resource, params) => Promise,
getMany: (resource, params) => Promise,
getManyReference: (resource, params) => Promise,
create: (resource, params) => Promise,
update: (resource, params) => Promise,
updateMany: (resource, params) => Promise,
delete: (resource, params) => Promise,
deleteMany: (resource, params) => Promise,
}
Another thing to note here is that the returning methods of these should be in the specified format and deviation from this will cause errors.
This doesn’t mean that the methods should strictly be in any one of the methods. In most cases, we may need more than this which is based on our requirements. React-admin also allows us to create custom methods. The useDataProvider hook may be handy when dealing with custom methods. There are also hooks like useGetOne, useGetList, useDelete, etc that can be used whenever your requirement demands.
That’s all for this one, we’ll learn more about listing records and creating records in the next post.
Subscribe to my newsletter
Read articles from Shaba K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Shaba K
Shaba K
Frontend developer with expertise in React and a knack for Material UI for designing. Loves to explain concepts in written format. Bibliophile.