Map(),Reduce(),Filter(), Find() And How They Are Different in Nature
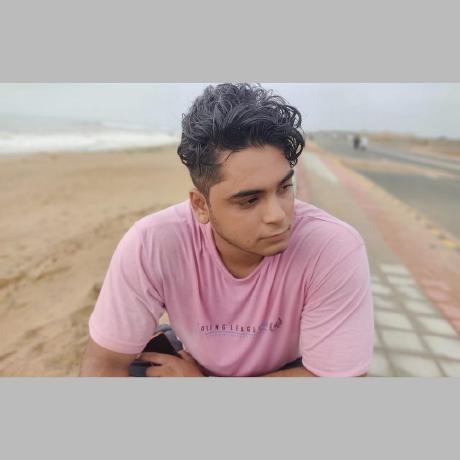
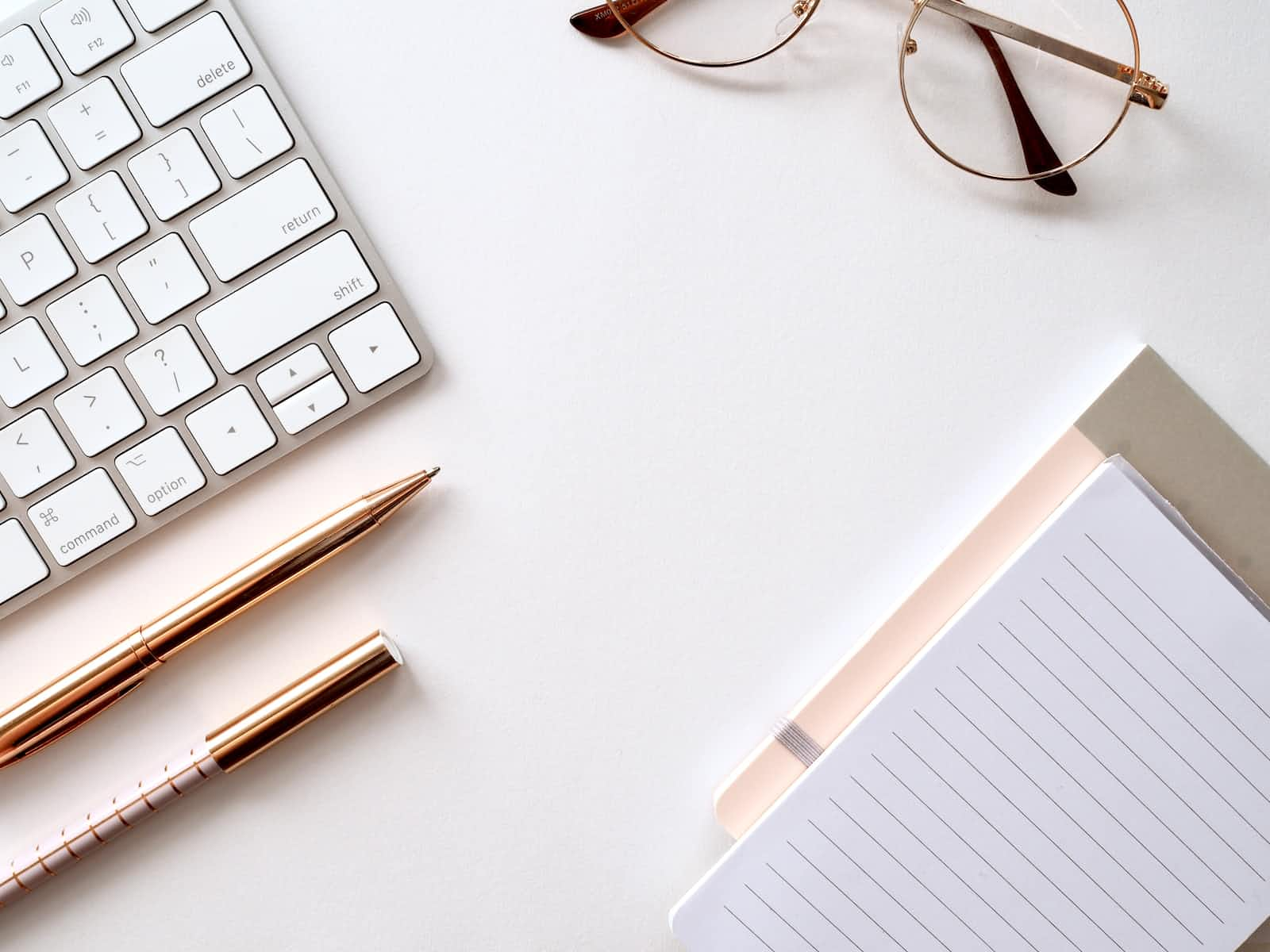
Many people are confused about when to use Map() when to use Filter() and when to use Reduce() and when to use Find(). Don't worry you are not alone.
Overview:-
Map(), reduce(), filter() and find () all are array methods in JavaScript. Each one will iterate over an array and perform a transformation or computation. In this article, you will learn why and how to use each one.
Map():-
The work of Map() is to return a new array out of the existing array after applying desired function to each element of that array. It doesn't change the original array or in other words it doesn't affect the original array instead it creates a new array.
Ex:-
//ES6
const array=[1,2,3,4,5];
const newArray=array.map(element=>element*2);
console.log(newArray);
//output:-[2,4,6,8,10];
Here we use Map() method on an array. inside Map(), we run an ES6 function where we use "element' as a parameter and return that value by multiplying it by 2. Finally, Map() method returns a new array after applying that ES6 function to all the elements of the old array.
Filter():-
Like Map() Filter also returns a new array but the main difference between Map() and Filter() is while Map() returns a new array containing the same amount of elements that are present in the old array filter returns only the elements which we choose to add in a new array. in other words Filter() returns a new array after applying a conditional statement to each element of an array. if the conditional returns true it includes that element in the new array and if it returns false it doesn't include it into a new Array.
Ex:-
//ES6
const array=[1,2,3,4,5];
const newArray=array.filter(element=>element%2===0);
console.log(newArray);
//output:-[2,4]
Here we use Filter() method on an array and inside the filter method we call a function where we take the element as a parameter and return a conditional statement that returns the element into the new array only if the element is divided 2.
Reduce():-
The Reduce() method reduces an array of values down to just one value. To get the output value, it runs a reducer function on each element of the array.
syntax:-
array.reduce(
(accumulator, currentValue) =>( accumulator + currentValue)
,initialValue)
//(accumulator, currentValue) is a callBack function
Ex:-
const array=[1,2,3,4,5,6];
const value=array.reduce((acc,crr)=>(acc+crr),0);
//output:- 21
We can see that here when we perform Reduce() on an array and expect the sum of all the numbers present in that array ,Reduce() does it for us without using Loop. Here inside reduce() we used a callback function where we take two parameters first is accumulator (acc) and second is currentValue(crr) and InitialValue as 0(By default). Inside that callback function, we perform sum.
Find():-
Find() method returns the first element of an array after performing the desired function on that array. Remember unlike Filter() method Find() only returns the first boolean element which is True.
Ex:-
const array=[1,2,3,4,5];
const value=array.find(element=>element%2===0);
//Output:- 2
here we used find() method to find the first element of the array which is an even number. For that, we used a callback function inside the Find() function that will return the element which is a even number.
When to use What:-
Use Map() when you want a new array that contains all the elements of the old array but after applying a certain function on each element of that array.
Use Filter() when you want to create a new array from an old array after filtering out your desired elements by the use of a certain function.
Use Find() when you want to find out a particular element from an array. Remember Find() only returns a single element not an array, unlike Filter() method.
Use Reduce() when you want a particular result out of an array. It could be the sum of all numbers or concatenate strings etc.
Conclusion:-
Map(), Filter(), Reduce(), Find() these array methods make our lives easy as coders beacuse we can do certain things on an array without using Loops. But understanding when to use what is the most important thing and that understanding will come with more and more practice. So keep learning and keep practising.
Subscribe to my newsletter
Read articles from Debashis Kar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
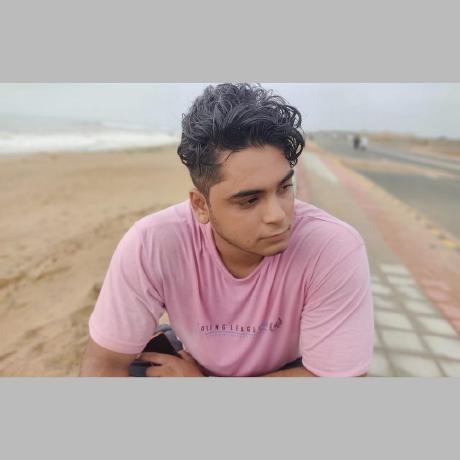