Different ways to import in Python
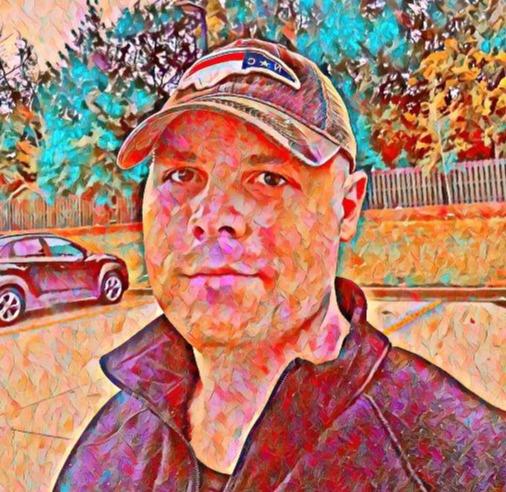
Table of contents
Importing in Python
I was in a Python community on Twitter, and saw someone ask a question. They asked if import math
and from math import *
were the same. They are not.
I decided to write a short post explaining the various ways you can import something in Python, and explain what the differences are.
import math
When you import a module like this, the entire module is imported, but the contents are contained within the module. What do I mean by this? Well, in the math module, there is a function called sin
. In order to access this function, one would do math.sin(argument)
as so.
This standard practice and is useful when you are working with a lot of code but do not want to overwrite the definitions of other code that you have. To help show what I mean, let’s compare this to the other method of importing.
from math import *
When you import a module this way, all of its contents are now present in the global scope. You can prove this with the globals() function. If you simply do import math
and then call globals(), you won’t see all of the math module’s contents in the global scope, but if you do from math import *
and then call the globals function, you WILL see all of its contents show up in global scope. You can also use the sin function by calling it on a variable, you don’t have to do math.sin like we did earlier.
Importing modules this way is considered bad practice, and most of the time you would not want to do this. There are various reasons for this, but one reason is to prevent objects from over-writing each other. For example, remainder is a function in the math module. If you had a variable named “remainder” and then did from math import *
then the value of remainder would be over-written into the function from the math module.
math = __import__(“math”, globals=None, locals=None, fromlist=(), level=0)
This is the __import__()
function, it is probably the rarest form of importing a module that you will see, and it is not recommended. Let me explain the parameters.
__import__() Parameters
name - the name of the module you want to import
globals and locals - determines how to interpret name
fromlist - objects or submodules that should be imported by name
level - specifies whether to use absolute or relative imports
Conclusion
None of these ways of importing are the same. Each are different and have their use cases. Whether you’re a beginner or an expert, you will be able to get by using the import keyword to import what you need.
Subscribe to my newsletter
Read articles from Zian Elijah Smith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
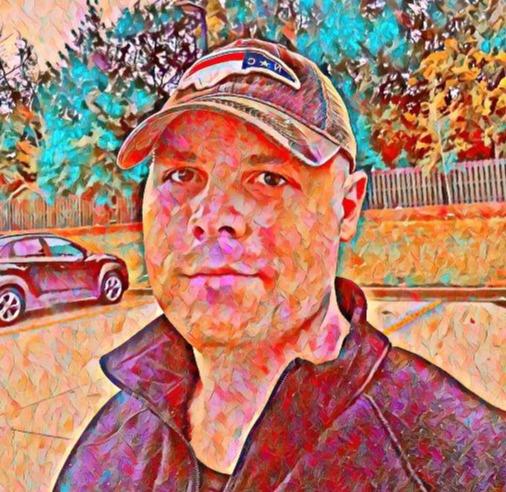