Difference Between "==" and "===" in javaScript

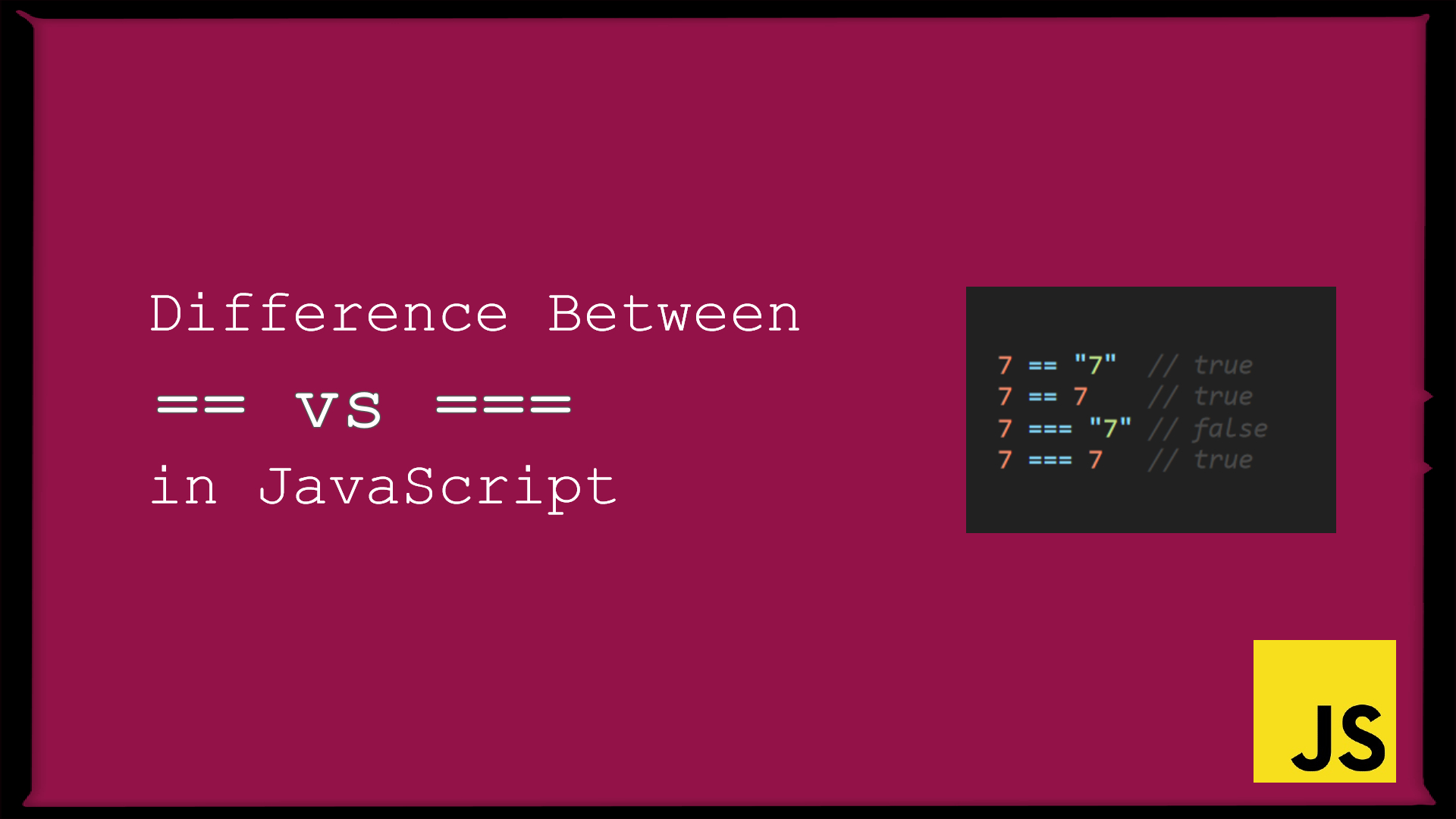
Introduction
In javascript, two comparison operators give the same answers with some code comparison and different answers with some code comparison. we are talking about "==" which is known as an abstract operator or loose equality operator and "===" which is known as a strict equality operator.
''==" takes the value and compare it while "===" takes both value and datatype and compare it.
const firstValue = 10;
const secondValue = "10";
console.log(firstValue == secondValue);
// output : true
console.log(firstValue === secondvalue);
// output : false
In the above example, it can be seen that when we apply the loose equality operator it gives the output true while with the strict equality operator output is false. we will get to know WHY?
Loose equality operator
It's a comparison operator which only takes a value and compares it. why "==" gives output as true the answer is implicit type coercion.
const firstValue = 10;
const secondValue = "10";
console.log(firstValue == secondValue);
// output = 10
console.log (typeof firstValue);
// output = Number;
console.log (typeof secondValue);
// output = String;
firstvalue is a number while secondvalue is a string and during comparing it converts its type if the value is the same.
The == operator does type conversion of operand before comparing and returning an output.
If we are comparing null and undefined, then the \== operator will return true.
Implicit type coercion
Type coercion is the conversion of data types. Implicit type coercion is the automatic conversion from one data type to another data type.
const numberValue = 1;
const booleanValue = true;
console.log(numberValue == booleanValue);
// output = true;
console.log(typeof numberValue);
// output = Number;
console.log(typeof booleanValue);
// output = boolean;
In the above example, both are of different types but during comparison, booleanValue is converted to 1 internally and it gives the output true. If we are comparing a boolean and a number (0 or 1), then it treats 0 as false and 1 as true. booleanValue has true value and it indicates for 1 and during comparing datatypes of operand converted to number. so, in large codebases try to avoid loose equality operator.
const firstNumber = 10;
const firstString = "10";
const secondNumber = 20;
const secondString = "20";
console.log(firstNumber + secondNumber);
//output = 30;
console.log(firstNumber + firstString);
// output = '1010';
console.log(firstNumber + secondNumber + firstString);
// output = '3010';
// firstNumber + secondNumber + string = 10 + 20 + "10" = 3010
// first additon operator works as adding the firstNumber and secondNumber while second addition operator works as concat the string.
console.log(firstString + secondString);
// output = '1020';
console.log(firstString + firstNumber + secondNumber);
// ouput = "101020";
// string + firstNumber + secondNumber = 10 + 10 + 20 = "101020"
// first addition operator works as concat the string and number and it gives output as string as it use number firstNumber as string due to implicit type coercion and same happens with secondNumber.
In the above snippets, it can be seen that during addition when we encounter an operand of string type it concat rather than addition. it also concat the operand of number type instead of adding because of implicit type coercion.
Strict equality operator
It's a comparison operator which compares both value and data type to compare and gives output on that. it returns the output of a boolean value of true or false. \=== doesn't do type conversion.
const firstVar = 1;
const secondVar = "1";
console.log(firstVar === secondVar);
// output : false;
In the above code comparing operand firstVar and operand secondVar output came as false as both operands have equal values. Because for the first operand, its datatype is a number and for the second operand its datatype is a string and the strict equality operator compares its datatype also.
let a = null;
let b;
console.log(a == b);
// output : true;
console.log(a === b);
// output : false;
If we are comparing null and undefined, then the \== operator will return true.
\== follows abstract equality comparison algorithm
If we are comparing null and undefined, then the \=== operator will return false.
\=== follows strict equality comparison algorithm
let name1 = {
name: "Prince",
}
let name2 = {
name: "Raj",
}
console.log(name1 == name1);
//output: true
console.log(name1 == name2);
//output: false
console.log(name1 === name1);
//output: true
console.log(name1 === name2);
//output: false
For non-primitive data types both loose operator and strict operator work same both refer to the same reference.
Type conversion works behind loose equality operator, so avoid using loose equality operator in large codebases. use strict equality operator in a large codebase.
Subscribe to my newsletter
Read articles from prince raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
