Maps in Javascript
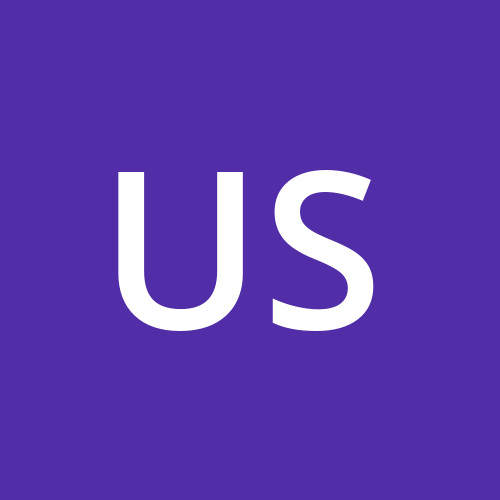
Introduction
Map is a collection of elements where each element stores a key-value pair and remembers the original order of keys.
Map is an object that can hold objects and primitive values as either keys or values.
Syntax :
const map1 = new Map()
map1.set('a',1)
console.log(map1) //Expected output Map(1) { 'a' => 1 }
Description
Map objects are collections of key-value pairs. A key in the map only may occur once; it is unique in the Map's collection. A map can be iterated by its key-value pairs — a for...of
loop returns a 2-member array of [key, value] of each iteration. Iteration happens in insertion order which corresponds to the order in which each key-value pair was first inserted into the map by the set()
Objects Vs Maps
The object is similar to Map
— both let to set keys to values., retrieve those values, delete keys and detect whether is stored something in the key. For this reason (and because there were no built-in alternatives), The object
has been used as Map
historically.
However, there are important differences that make Map
preferable in some cases:
Map | Object | |
Accidental Keys | A map does not contain any keys by default. It only contains what is explicitly put into it. | An Object has a prototype, so it contains default keys that could collide with your own keys if you're not careful. |
Security | A Map is safe to use with user-provided keys and values. | Setting user-provided key-value pairs may allow an attacker to override the object's prototype, which can lead to object injection attacks. |
Key Types | A Map keys can be any value (including functions, objects, or any primitive). | The keys of an Object must be either a String or a Symbol . |
Key Order | Map object iterates entries, keys and values in order of their entry insertion | In an object, no single mechanism iterates all the values of the object; the various mechanisms each include different subsets of properties. (for-in includes only enumerable string-keyed properties; Object. keys include only own, enumerable, string-keyed properties even if non-enumerable; Object.getOwnPropertySymbols does the same for just Symbol-keyed properties, etc. |
Size | The number of items in a Map is easily retrieved from its size property. | Determining the number of items in an Object is more roundabout and less efficient. A common way to do it is through the length of the array returned from Object.keys(). |
Constructor
Creates a new Map object.
Static properties
The function is used to create derived objects.
Instance Properties
These properties are defined on Map. prototype and shared by all the Map instances.
The constructor function that created the instance object. For Map instances, the initial value is the Map constructor.
Example :
const utkarsh = "object";
console.log(utkarsh.constructor===String); //true
const obj = {}
console.log(obj.constructor===Object) //true
const Marks = {
Hindi : 99,
English : 95,
Maths :97
}
console.log(Marks.constructor===Object) //true
Returns the number of key-value pairs in the Map object.
Example :
const map1 = new Map();
map1.set("Hindi",99)
map1.set("English",95)
map1.set("Maths",97)
console.log(map1.size); //3
Map.prototype[@@toStringTag]
The initial value of the @@toStringTag
property is the string "Map"
. This property is used in Object.prototype.toString()
.
Example:
function Dog(name){
this.name = name
}
const dog1 = new Dog("JOJO")
Dog.prototype.toString = function dogToString(){
return `${this.name}`
}
console.log(dog1.toString()) //JOJO
Instance Methods
The clear() method removes all key pairs from the map object.
Example :
map1.clear()
console.log(map1.size) // Expected output : 0
Returns true if an element in the map object existed and has been removed, or false if the element does not exist. map. has(key) will return false afterward.
Example :
const hello = new Map()
hello.set('jojo','tommy')
hello.set('sheru','bruno')
console.log(hello.delete('sheru')) //true
console.log(hello) //Map(1) { 'jojo' => 'tommy' }
Returns the value associated with the passed key. or undefined
if there is none.
Example:
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
console.log(temp.get("Hindi")) //99
console.log(temp.get("English")) //97
console.log(temp.get("Mathematics")) //98
Returns a boolean indicating whether a value is associated with the passed key in the Map
object or not.
Example :
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
console.log(temp.has("Hindi")) //true
console.log(temp.has("English")) //true
console.log(temp.has("Science")) //false
Sets the value to the passed key in the Map
object and returns the object.
Example:
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
console.log(temp) //Map(3) { 'Hindi' => 99, 'English' => 97, 'Mathematics' => 98 }
Returns a new iterator object that contains a two-member array of [key,value] for each element in the Map
object in insertion order.
Example :
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
const iterator1 = temp[Symbol.iterator]()
for(const item of iterator1){
console.log(item)
}
// Output : [ 'Hindi', 99 ]
//[ 'English', 97 ]
//[ 'Mathematics', 98 ]
Returns a new Iterator object that contains the keys for each element in the Map
object in insertion order.
Example :
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
const iterator2 = temp.keys()
console.log(iterator2) //[Map Iterator] { 'Hindi', 'English', 'Mathematics' }
console.log(iterator2.next().value) //Hindi
console.log(iterator2.next().value) //English
Returns a new Iterator object that contains the values for each element in the Map
object in insertion order.
Example :
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
const iterator3= temp.values()
console.log(iterator3) // [Map Iterator] { 99, 97, 98 }
console.log(iterator3.next().value) // 99
console.log(iterator3.next().value) //97
Returns a new Iterator object that contains a two-member array of [key, value]
for each element in the Map
object in insertion order.
Example :
const temp = new Map();
temp.set("Hindi",99)
temp.set("English",97)
temp.set("Mathematics",98)
const iterator4= temp.entries()
console.log(iterator4)
console.log(iterator4.next().value)
console.log(iterator4.next().value)
/* output: [Map Entries] {
[ 'Hindi', 99 ],
[ 'English', 97 ],
[ 'Mathematics', 98 ]
}
[ 'Hindi', 99 ]
[ 'English', 97 ] */
Calls callbackFn
once for each key-value pair present in the Map
object, in insertion order. If a thisArg
parameter is provided to forEach
, it will be used as the this
value for each callback.
Example :
function marks(key,value,temp){
console.log(`m[${key}] = ${value}`)
}
console.log(new Map([['Hindi',99],['English',{}],['Mathematics',undefined]]).forEach(marks))
/* Output : m[99] = Hindi
m[[object Object]] = English
m[undefined] = Mathematics
undefined */
Subscribe to my newsletter
Read articles from Utkarsh Saxena directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
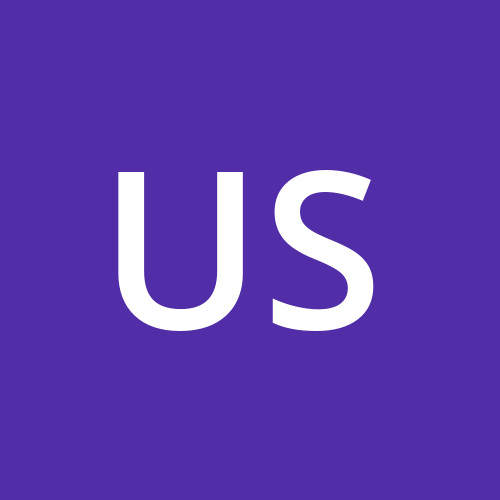