React admin #2 - A step further: Diving into the major elements
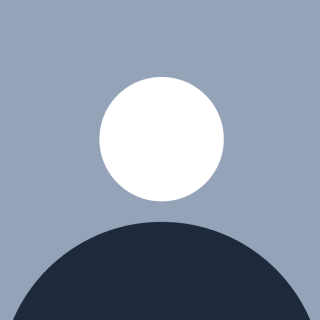
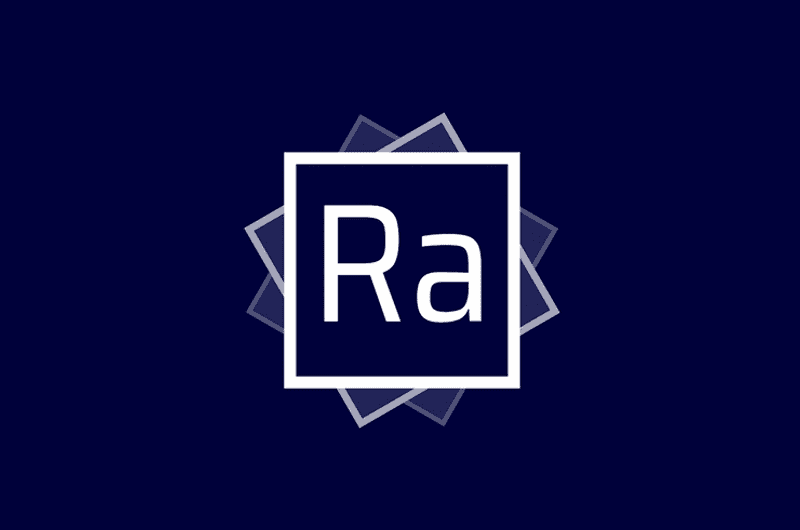
In the previous article, we explored the basics of React Admin and setting up. Let’s now dive more into how we can use React Admin for various applications.
How do we create records in React Admin?
The major goals of creating an admin-side website include creating, editing, viewing, and deleting records. To create a new resource in React Admin, use the <Create> component. Children of the <Create> component should include the form that you’ve created for adding records.
It is also important that you specify the create component while creating the resource in the app.js file. Or you could combine all the major components like edit, show and list inside an object and spread in the resource.
import UserAdd from './UserAdd.js';
import UserEdit from './UserEdit.js';
import UsersList from './UsersList.js';
import UserShow from './UserShow.js';
const Users = {
create: UserAdd,
edit: UserEdit,
list: UsersList,
show: UserShow
}
For creating the form, a multitude of inputs is provided by React Admin. You can choose according to the requirements.
import * as React from 'react';
import { Create, SimpleForm, TextInput, DateInput, SelectInput } from 'react-admin';
export const UserAdd = () => (
<Create>
<SimpleForm>
<TextInput source="name" label="Name" />
<TextInput source="email" label="Email" />
<DateInput source="dob" label="Date of Birth" />
<SelectInput source="jobrole" choices={[
{ id: 'admin', name: 'Admin' },
{ id: 'developer', name: 'Developer' },
{ id: 'tester', name: 'Tester' },
]}/>
</SimpleForm>
</Create>
);
This will generate a form with four inputs to create a new user. Along with creating the form, the API has also to be mentioned in the dataProvider. For create, it automatically calls create function.
For instance,
create: async function (resource, params) {
if (resource === 'users') {
try {
const apiUrl = `/user/create`;
const { data } = await httpClient.post(apiUrl, params.data);
return {
data: { data, id: data.id },
};
} catch (error) {
throw new Error(error.message);
}
}
},
How do we edit records in React Admin?
Edit is similar to create except that it works based on an id. It is important that each of the records should have an id. Make a similar form to that of create with the fields you wish to edit and then provide the API in the update call. In edit, to display the fields to edit, React Admin fetches data from getOne based on the id and resource of the record deduced from the URL.
getOne: async function (resource, params) {
if (resource === 'users') {
try {
const apiUrl = `/user/show`;
const { data } = await httpClient.get(apiUrl, params.data);
return {
data: { data, id: data.id },
};
} catch (error) {
throw new Error(error.message);
}
}
},
One thing to make sure of here is that while providing the source, it should be matching with the field in your API structure. This helps in smoothly prepopulating the data and updating it.
import * as React from 'react';
import { Edit, SimpleForm, TextInput, DateInput } from 'react-admin';
export const UserEdit = () => (
<Edit>
<SimpleForm>
<TextInput source="name" label="Name" />
<TextInput source="email" label="Email" />
<DateInput source="dob" label="Date of Birth" />
</SimpleForm>
</Edit>
);
update: async function (resource, params) {
if (resource === 'users') {
try {
const apiUrl = `/user/edit/${params.id}`;
const { data } = await httpClient.patch(apiUrl, params.data);
return {
data: data,
};
} catch (error) {
throw new Error(error.message);
}
}
},
How do we view records in React Admin?
Viewing records is made with the help of the Show component. The show component also fetches data from the getOne and data is displayed accordingly. It basically creates a ShowContext and a RecordContext. The child component is rendered based on the <Card> from MUI.
import * as React from 'react';
import { Show, SimpleShowLayout, TextField, DateField } from 'react-admin';
export const UserShow = () => (
<Show>
<SimpleShowLayout>
<TextField source="name" label="Name" />
<TextField source="email" label="Email" />
<DateField source="dob" label="Date of Birth" />
</SimpleShowLayout>
</Show>
);
As an alternative, there is also a different layout TabbedShowLayout
which helps in viewing data as a separate tab rather than in a single column.
We discussed how to create, edit and display resources in a React Admin application. We looked at the <Create>, <Edit>, and <Show> components and their usage with examples. In the next post, we will look into listing and other options by React Admin.
Subscribe to my newsletter
Read articles from Shaba K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Shaba K
Shaba K
Frontend developer with expertise in React and a knack for Material UI for designing. Loves to explain concepts in written format. Bibliophile.