Compiling a Node.js Application into an executable file
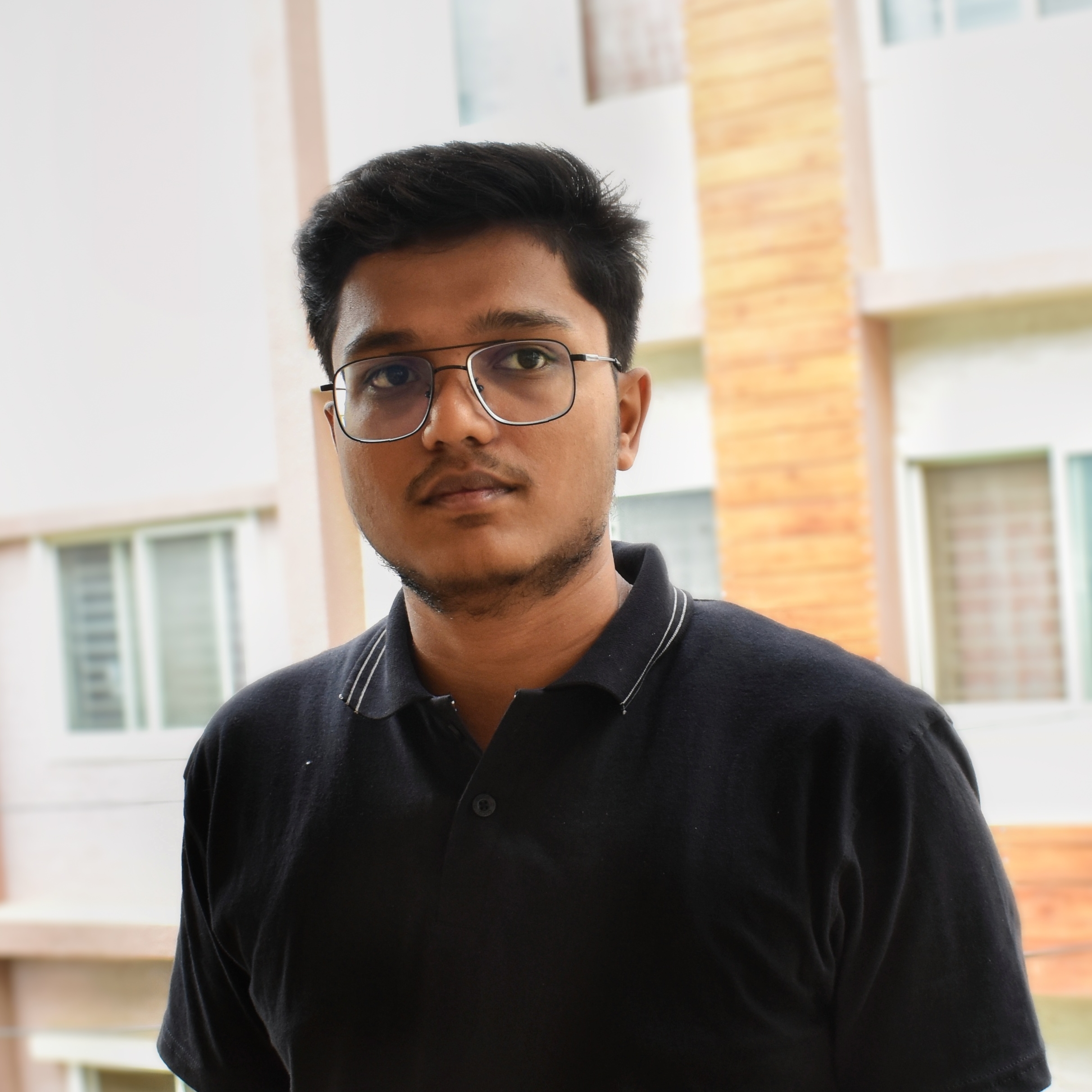
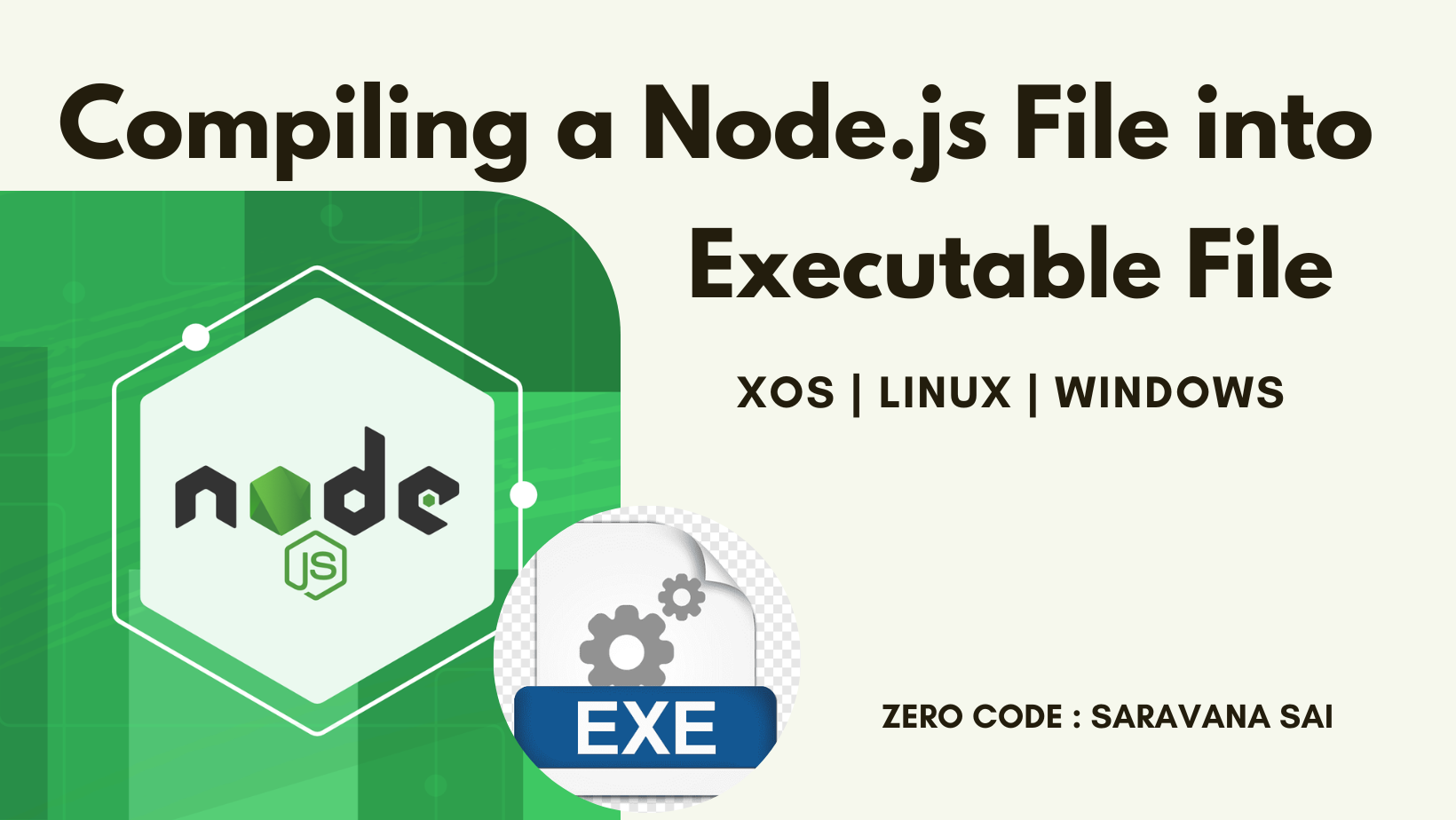
Introduction
Executable files (.exe files): These files contain a set of encoded instructions, executed sequentially once the user clicks on the file or runs it.
.exe
is a Windows extension referring to an executable file? Other operating systems have executable files as well, but with varying extensions. For example, in Linux or Unix-based operating systems, files ending with the .bin
, .elf
extensions are executables, while in MacOS, they lack extensions.
In this article, we are going to learn how to create a .exe binary executable file from JavaScript code.
Prerequisites
Node.js installed in your machine.
A stable internet connection.
pkg - npm package: It is a Node.js package that can convert your Node.js app into several executable files for various operating systems or for an individual operating system.
We shall now get a simple Node.js application that is running and use it as our application.
A Simple Application
Initialize the project with:
npm init -y
For simplicity, we are going to build a basic application that prints the name 10 times in the console.
Folder Structure:
├── node_modules (folder)
├── index.js (file)
└── package.json (file)
index.js:
for (let index = 0; index < 10; index++) {
console.log("hello Dev's");
}
package.json:
{
"name": "Simple node app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"bin": "index.js"
}
Compile file into an executable using pkg:
Run the following command in which we’ll call the pkg module and the entry point to our application.
pkg index.js
This will compile our app’s entry point into three different executable files since no targets were specified. Just wait for some time for the process to complete. Head over to the main directory. You will find three newly generated files.
These are the:
Windows (index-win.exe)
Linux (index-Linux)
mac (index-macOS)
Run the file that’s suitable for your operating system. For example, in Windows, double-click on the “index-win.exe” icon.
It will launch the app and display a console message that will act as an interactive interface between you and the app.
Conclusion
I hope you folks like this article and share & comment with your thoughts. In addition, one prefers quick project distribution. We can solve these by compiling them into Node.js executable files either with or without resources as preferred.
Subscribe to my newsletter
Read articles from Saravana Sai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
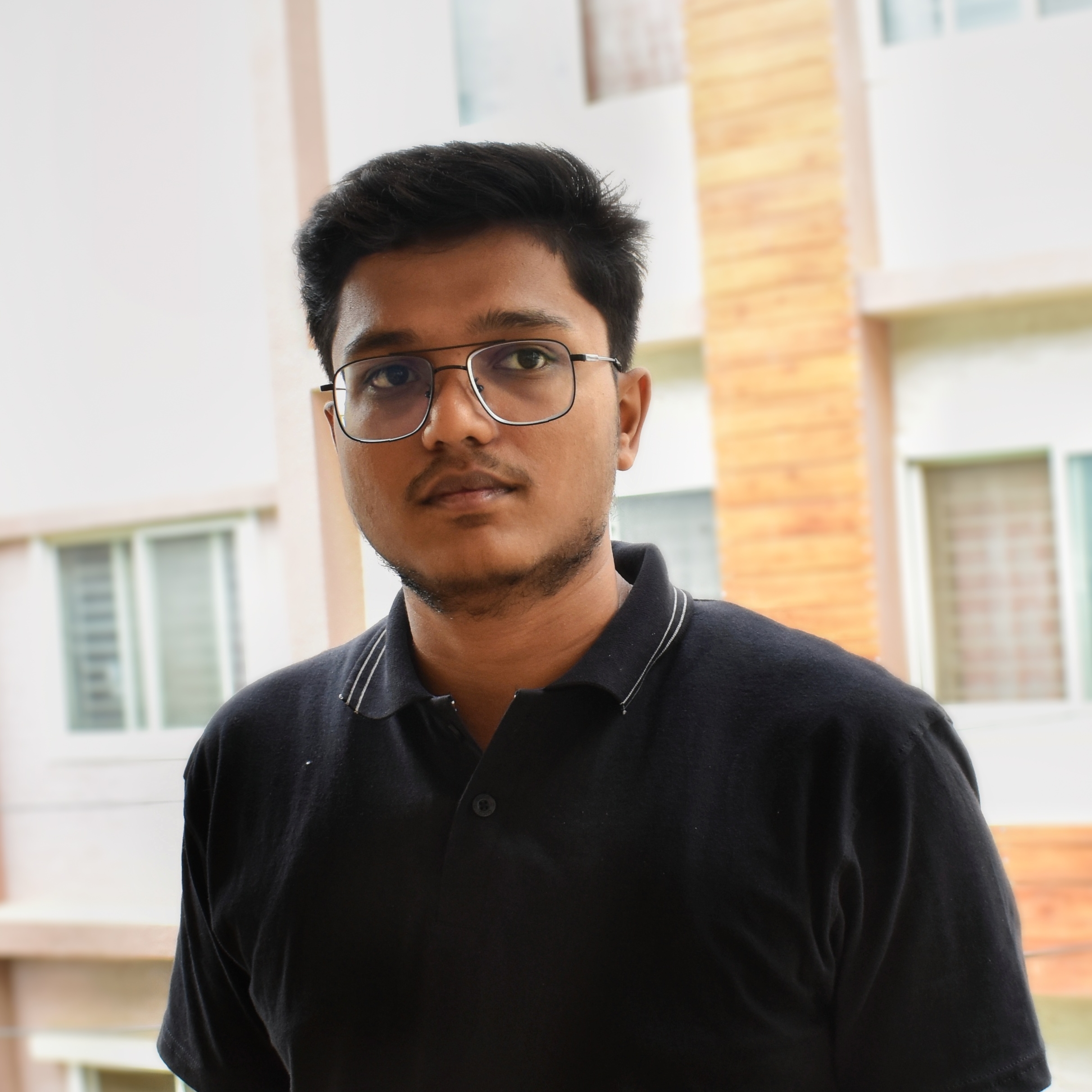
Saravana Sai
Saravana Sai
I am a self-taught web developer interested in building something that makes people's life awesome. Writing code for humans not for dump machine